树的高度
方式1
public class Node {
//树的高度
public int Height1() {
return Math.max(this.left == null ? 0 : this.left.Height1(), this.right == null ? 0 : this.right.Height1()) + 1;
}
}
public class BinarytShortTree {
Node root;
public int Height1() {
return root.Height1();
}
}
public class BinarytShortTreeDemo {
public static void main(String[] args) {
System.out.println(bst.Height1());
}
}
方式2
public class BinarytShortTree {
Node root;
public int Height2(Node node) {
return Math.max(node.left == null ? 0 : Height2(node.left), node.right == null ? 0 : Height2(node.right)) + 1;
}
}
public class BinarytShortTreeDemo {
public static void main(String[] args) {
System.out.println(bst.Height2(bst.root));
}
}
方式3
public class BinarytShortTree {
Node root;
public int Height3(Node node) {
if (node == null) {
return 0;
}
int left = Height3(node.left);
int right = Height3(node.right);
return Math.max(left, right) + 1;
}
}
public class BinarytShortTreeDemo {
public static void main(String[] args) {
System.out.println(bst.Height3(bst.root));
}
}
方式4是错误的 NullPointerException
//NullPointerException
// 这个是不对的,因为null不能调方法
// 但是null可以作为参数传递,所以这个方法只能写在BinaryShortTree里面
public class Node {
public int Height4() {
if (this == null) {
return 0;
}
int left = this.left.Height4();
int right = this.right.Height4();
return Math.max(left, right) + 1;
}
}
public class BinarytShortTree {
public int Height4() {
return root.Height4();
}
}
public class BinarytShortTreeDemo {
public static void main(String[] args) {
System.out.println(bst.Height4());
}
}
左旋转
右旋转
双旋转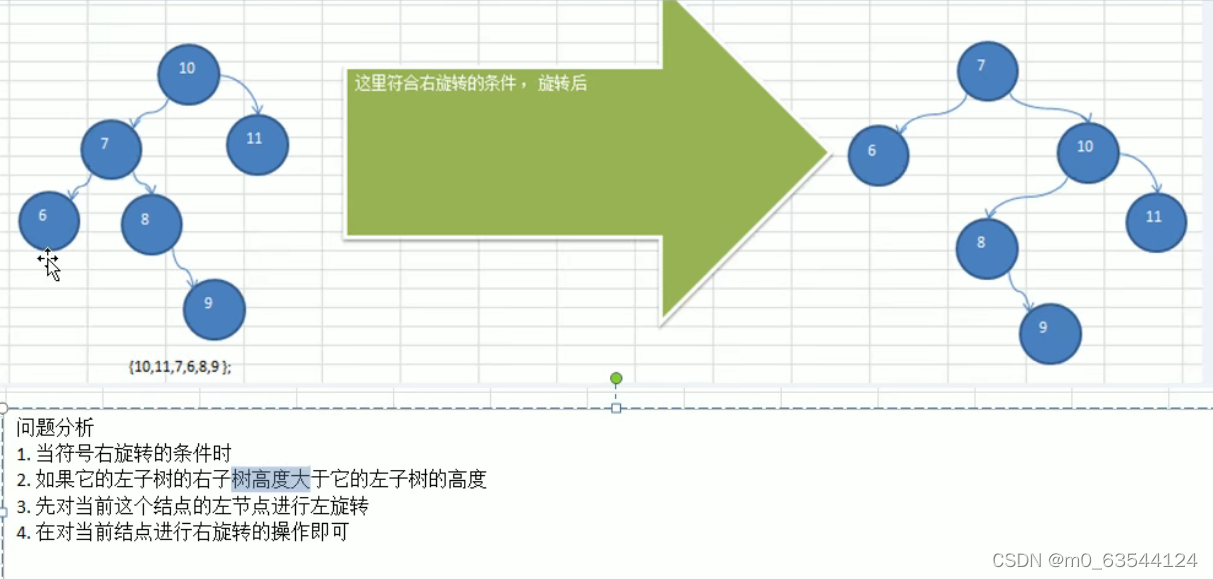
public class Node {
int no;
Node left;
Node right;
public Node() {
}
public Node(int no) {
this.no = no;
}
@Override
public String toString() {
return "Node{" +
"no=" + no +
'}';
}
//添加
public void add(Node node) {
if (node == null) {
return;
}
if (node.no < this.no) {
if (this.left == null) {
this.left = node;
} else {
this.left.add(node);
}
} else {
if (this.right == null) {
this.right = node;
} else {
this.right.add(node);
}
}
//右子树的高度比左子树的高,且相差大于1,左旋转,降低右子树的高度
if (this.rightHeight() - this.leftHeight() > 1) {
if (this.right.leftHeight() > this.right.rightHeight()) {
//如果右子树的左子树的高度大于右子树的右子树的高度
//需要先把右子树向右旋转,降低右子树的左子树的高度
this.right.turnright();
//然后把当前节点左旋转
this.turnLeft();
} else {
//否则直接左旋转
this.turnLeft();
}
}
//左子树的高度比右子树的高,且相差大于1,右旋转,降低左子树的高度
if (this.leftHeight() - this.rightHeight() > 1) {
if (this.left.rightHeight() > this.left.leftHeight()) {
//如果左子树的右子树的高度大于左子树的左子树的高度
//先将左子树向左旋转,降低左子树的右子树的高度
this.left.turnLeft();
//然后把当前节点右旋转
this.turnright();
}
}
}
public void turnLeft() {
Node newNode = new Node(this.no);
newNode.left = this.left;
newNode.right = this.right.left;
this.no = this.right.no;
this.right = this.right.right;
this.left = newNode;
}
public void turnright() {
Node newNode = new Node(this.no);
newNode.right = this.right;
newNode.left = this.left.right;
this.no = this.left.no;
this.left = this.left.left;
this.right = newNode;
}
//左子树的高度
public int leftHeight() {
if (this.left != null) {
return this.left.treeHeight();
} else {
return 0;
}
}
//右子树的高度
public int rightHeight() {
if (this.right != null) {
return this.right.treeHeight();
} else {
return 0;
}
}
//树的高度
public int treeHeight() {
return Math.max(this.left == null ? 0 : this.left.treeHeight(), this.right == null ? 0 : this.right.treeHeight()) + 1;
}
public void infixOrder() {
if (this.left != null) {
this.left.infixOrder();
}
System.out.println(this);
if (this.right != null) {
this.right.infixOrder();
}
}
}
public class AVLTree {
Node root;
public AVLTree() {
}
//添加
public void add(Node node) {
if (root == null) {
root = node;
} else {
root.add(node);
}
}
//左子树的高度
public int leftHeight() {
if (root != null) {
return root.leftHeight();
} else {
return 0;
}
}
//右子树的高度
public int rightHeight() {
if (root != null) {
return root.rightHeight();
} else {
return 0;
}
}
//统计树的高度
public int treeHeight() {
if (root != null) {
return root.treeHeight();
} else {
return 0;
}
}
public int height2(Node node) {
if (node == null) {
return 0;
}
int left = height2(node.left);
int right = height2(node.right);
return Math.max(left, right) + 1;
}
//中序遍历
public void infixOrder() {
if (root != null) {
root.infixOrder();
}
}
}
public class AVLTreeDemo {
public static void main(String[] args) {
int[] arr = {4, 3, 6, 5, 7, 8};
AVLTree avl = new AVLTree();
for (int x = 0; x < arr.length; x++) {
avl.add(new Node(arr[x]));
}
System.out.println(avl.treeHeight());
System.out.println(avl.leftHeight());
System.out.println(avl.rightHeight());
avl.infixOrder();
}
}