一、IO流概述
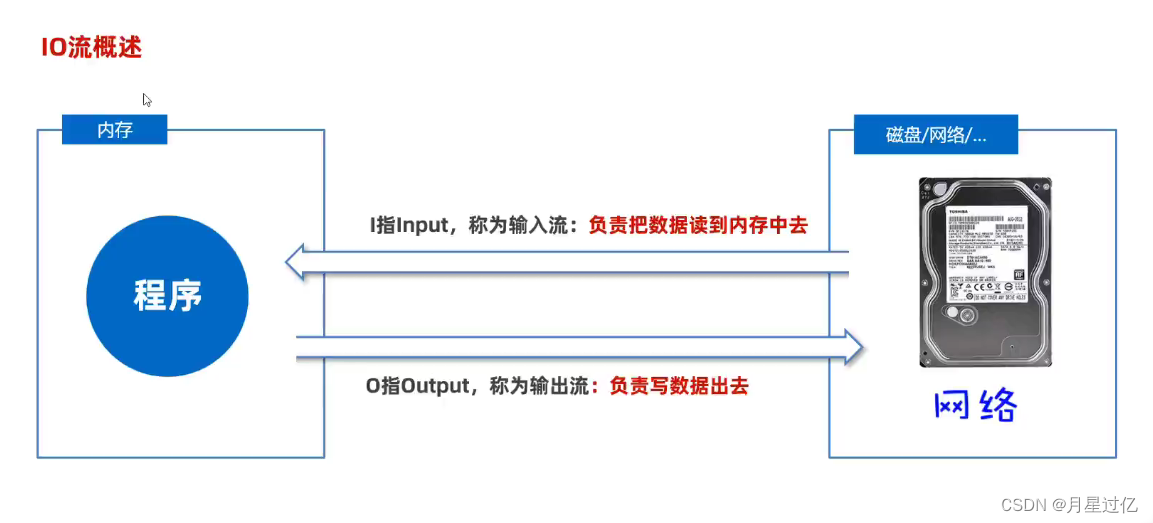
二、IO流的分类
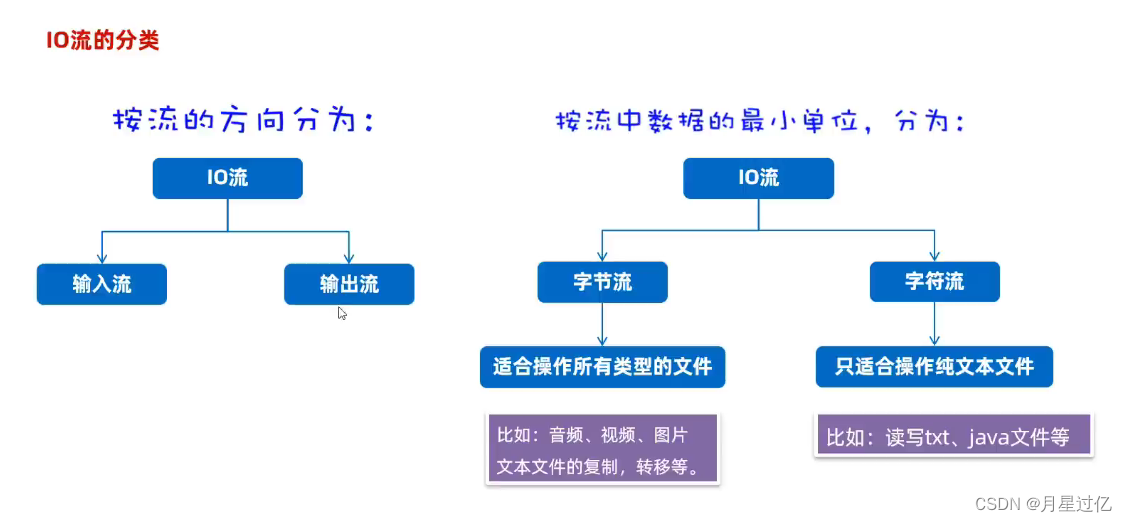
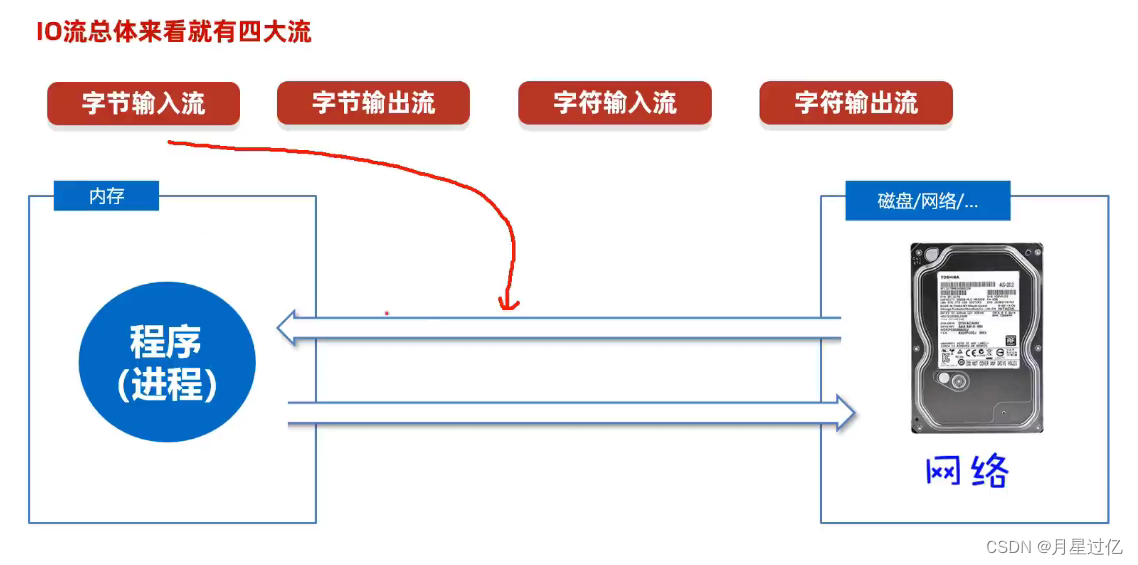
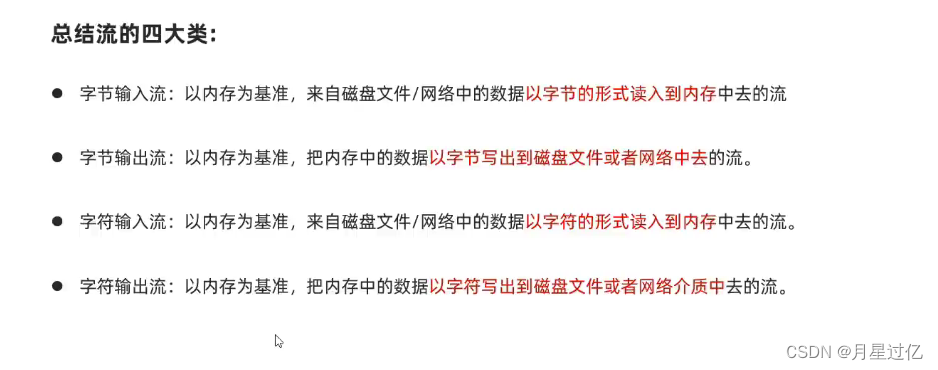
三、IO流的体系
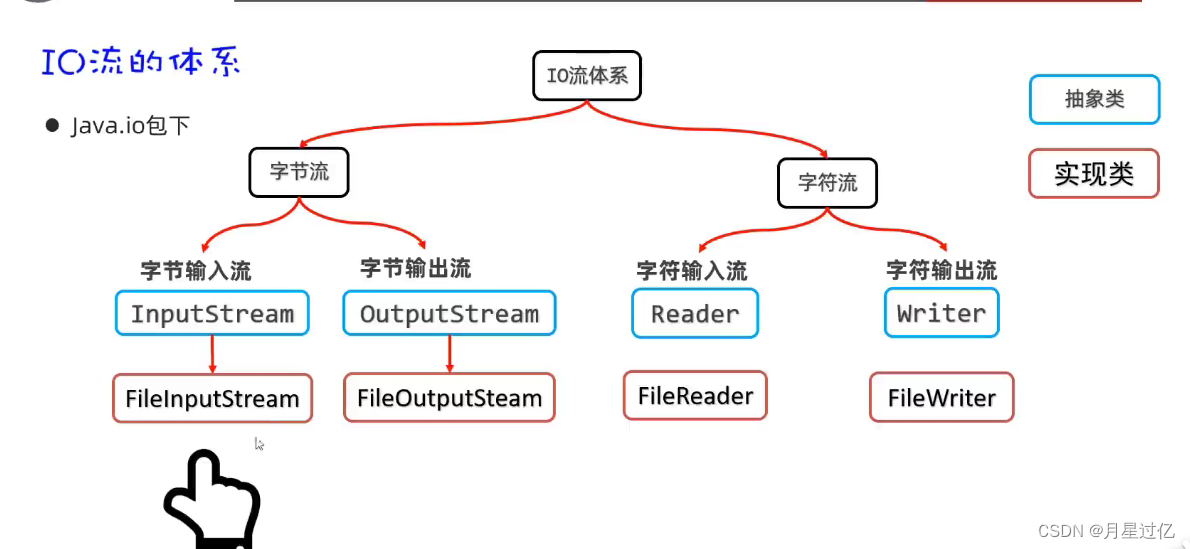
四、IO流的具体实现类
1.FileInputStream(文件字节输入流)
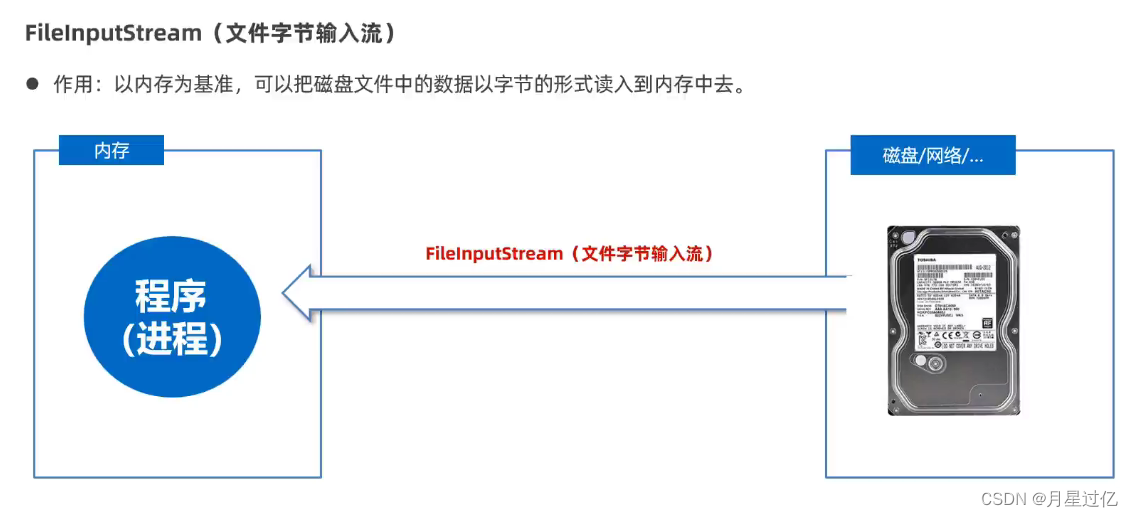
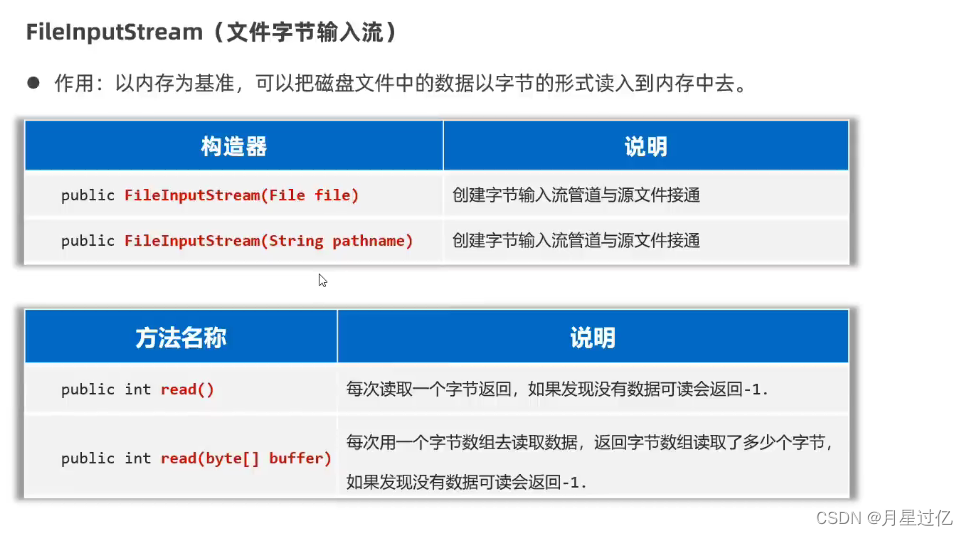
(1)每次读取一个字节
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class FileInputStreamTest {
public static void main(String[] args) throws Exception {
//1.创建文件字节输入流管道,与原文件接通
// FileInputStream is = new FileInputStream (new File ( "xuexi\\src\\zzz.txt" ) );
FileInputStream is = new FileInputStream ( "/xuexi/src/zzz.txt" );//简化写法 推荐使用
//2.读取文件的字节数据
// int b1 = is.read ();//每次只能读取一个字节
// System.out.println ( b1 );//97
// int b2 = is.read ();
// System.out.println ( b2 );//98
// int b4 = is.read ();
// System.out.println ( b4 );//-1 没有数据返回-1
//3.使用循环改造上述代码
int b;//用于记住读取的字节 读取数据的性能很差
while ((b= is.read ())!=-1){//读到-1停止
System.out.print ( (char) b );
}
//读取数据的性能很差
//无法避免中文乱码
//流使用完必须关闭 释放系统资源
is.close ();
}
}
(2)每次读取多个字节
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
public class FileInputStreamTest1 {
public static void main(String[] args) throws Exception {
//1.创建一个字节输入流管道连接文件
InputStream is= new FileInputStream ( "\\xuexi\\src\\zzz.txt" );
//2.开始读取文件中的数据,每次读取多个字节
byte[] bytes = new byte[3];
int len = is.read ( bytes );//返回每次所读的字节数
String rs = new String ( bytes );
System.out.println ( rs );
System.out.println ( len );//3
int len2 = is.read ( bytes );//读取会覆盖之前的数据
//读取多少,倒出多少
String rs1 = new String ( bytes ,0,len2);
System.out.println ( rs1 );//66
System.out.println ( len2 );//2
is.close();
}
}
循环改造
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
public class FileInputStreamTest1 {
public static void main(String[] args) throws Exception {
//1.创建一个字节输入流管道连接文件
InputStream is= new FileInputStream ( "\\xuexi\\src\\zzz.txt" );
// //2.开始读取文件中的数据,每次读取多个字节
// byte[] bytes = new byte[3];
// int len = is.read ( bytes );//返回每次所读的字节数
// String rs = new String ( bytes );
// System.out.println ( rs );
// System.out.println ( len );//3
//
// int len2 = is.read ( bytes );//读取会覆盖之前的数据
// //读取多少,倒出多少
// String rs1 = new String ( bytes ,0,len2);
// System.out.println ( rs1 );//66
// System.out.println ( len2 );//2
//
//
//3.使用循环改造
byte[] buffer = new byte[3];
int len;//记录读取了多少个字节
while ((len=is.read (buffer))!=-1){
String s = new String ( buffer, 0, len );
System.out.print ( s );
}
//性能得到了明显的提升!!
//这种方案也不能避免汉字乱码的问题
is.close ();
}
}
(3)一次性读完全部字节
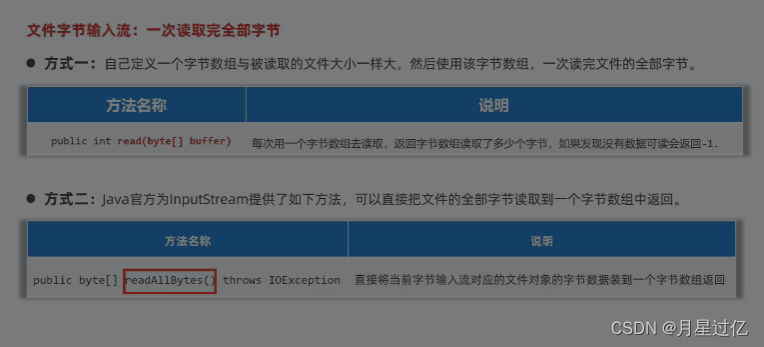
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
public class FileInputStreamTest3 {
public static void main(String[] args) throws Exception {
//1.创建一个字节输入流对象与文件连通
InputStream is=new FileInputStream ( "\\xuexi\\src\\zzz.txt" );
//2.准备一个字节数组,大小与文件的大小一样大
File f1 = new File ( "\\xuexi\\src\\zzz.txt" );
long size = f1.length ();
byte[] bytes = new byte[(int) size];
int len = is.read ( bytes );
String s = new String ( bytes );
System.out.println ( s );
System.out.println ( len );
System.out.println ( size );
is.close ();
}
}
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
public class FileInputStreamTest3 {
public static void main(String[] args) throws Exception {
//1.创建一个字节输入流对象与文件连通
InputStream is =new FileInputStream ( "\\xuexi\\src\\zzz.txt" );
//2.准备一个字节数组,大小与文件的大小一样大
// File f1 = new File ( "\\xuexi\\src\\zzz.txt" );
// long size = f1.length ();
// byte[] bytes = new byte[(int) size];
// int len = is.read ( bytes );
// String s = new String ( bytes );
// System.out.println ( s );
// System.out.println ( len );
// System.out.println ( size );
// is.close ();
//可以有效避免中文乱码
byte[] bytes=is.readAllBytes();
System.out.println (new String (bytes));
}
}
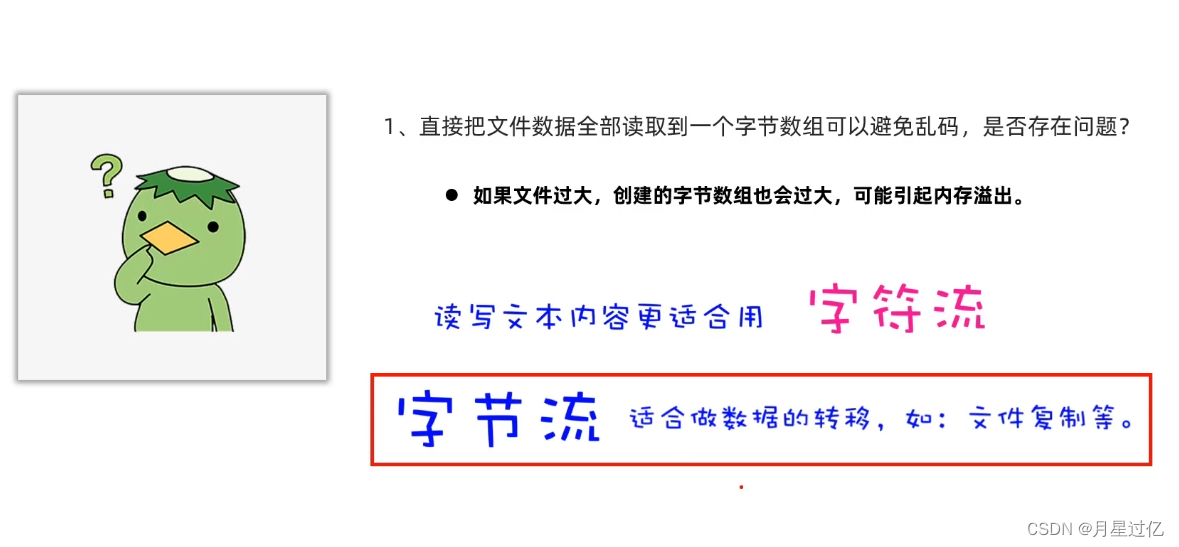
2.文件字节输出流:写字节出去
(1)概念
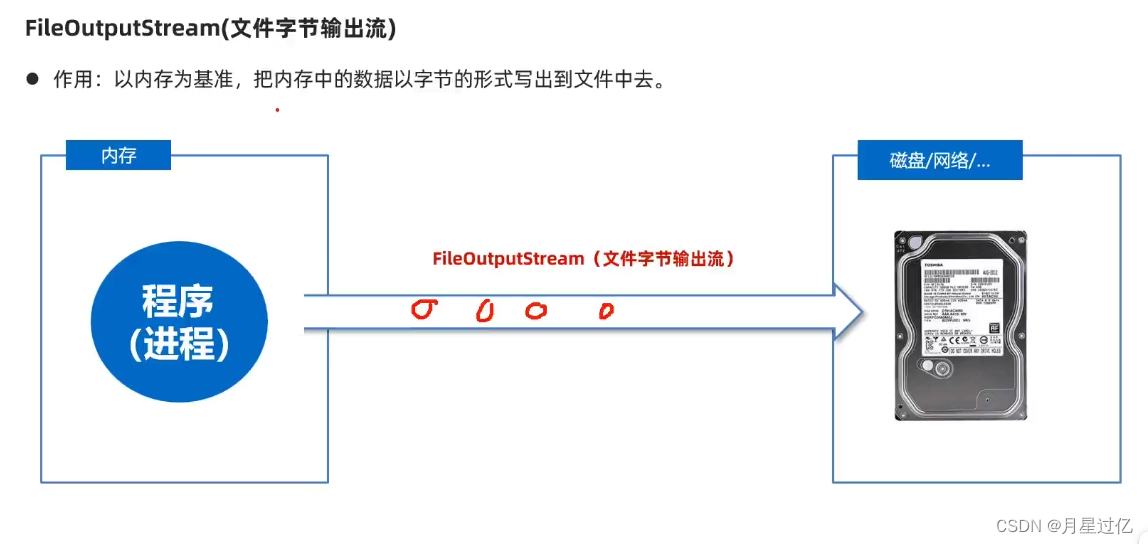
(2)常用方法
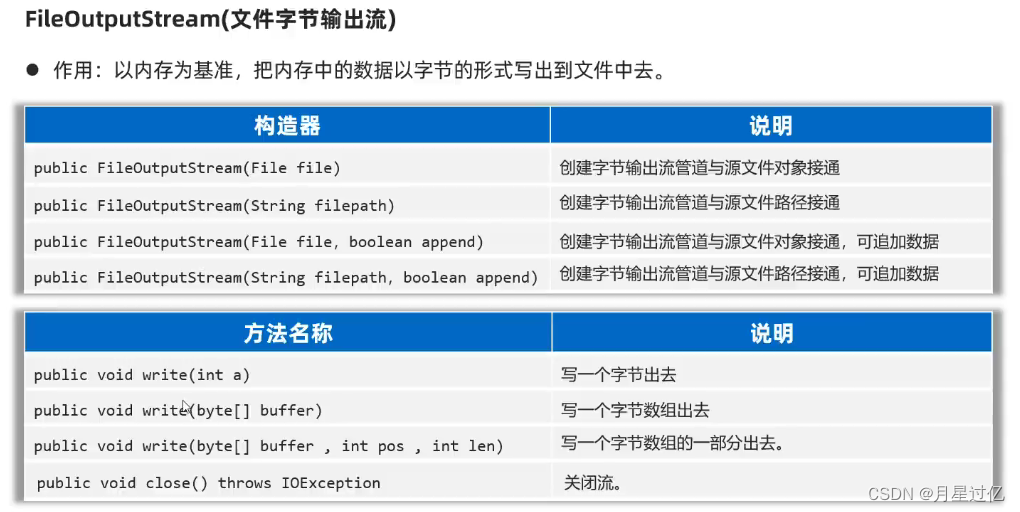
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.OutputStream;
import java.nio.charset.StandardCharsets;
public class FileOutputStreamTest {
public static void main(String[] args) throws Exception {
//1.创建字节输出流管道,与目标文件接通(不需要提前创建文件 会自动创建)
OutputStream os = new FileOutputStream ( "\\xuexi\\src\\hhh.txt" ,true);
//2.开始写字节数据出去
os.write ( 97 );//97----a
os.write ( 'b' );
//3.写入一组数据
byte[] bytes = "我爱你中国abc".getBytes ();
os.write ( bytes );
os.write ( bytes,0,15 );
//换行符
os.write ( "\r\n".getBytes( ) );
os.close ();
//每次写入前都会清空文件数据
//public FileOutputStream(String filepath,boolean append) append 表示是否追加 默认为false
// OutputStream os = new FileOutputStream ( "\\xuexi\\src\\hhh.txt" ,true); 表示不清空追加数据
}
}
五、文件复制案例
import java.io.*;
public class FileOutputStreamTest1 {
public static void main(String[] args) throws Exception {
//1.创建一个文件输入流通道 连接文件
InputStream is=new FileInputStream ( "E:\\新建文件夹\\shuaige.jpg" );
//2.创建一个文件输出流通道 输出目标文件
OutputStream os=new FileOutputStream ( "E:\\新建文件夹\\新建文件夹\\dashuaige.jpg" );
int len;
byte[] bytes = new byte[2048];
while((len=is.read ())!=-1){
os.write ( bytes,0,len );
}
os.close ();
is.close ();
System.out.println ("复制完成");
}
}