通过实训案例来了解
1.建立安卓项目
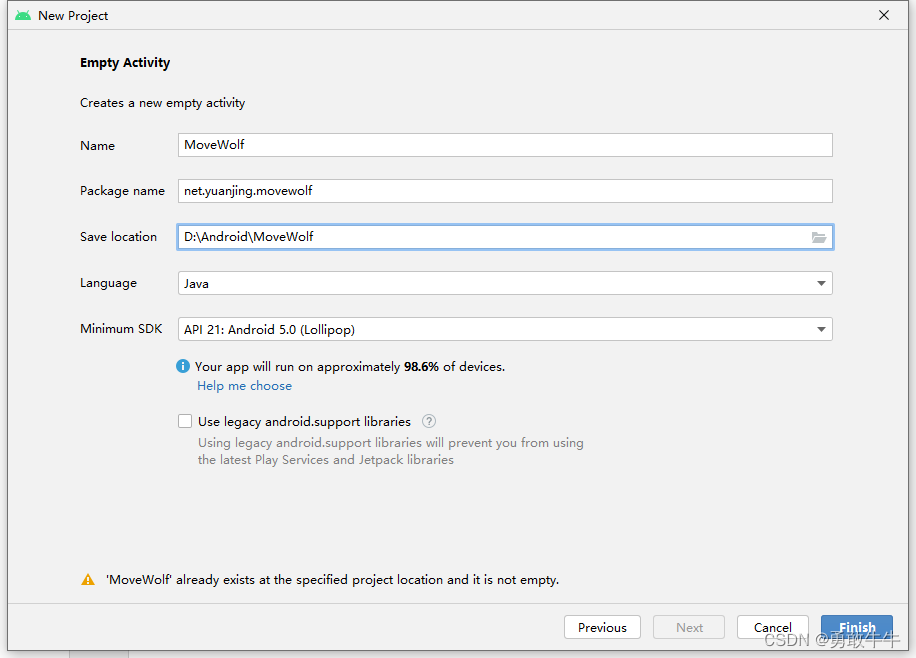
2.准备图片资源
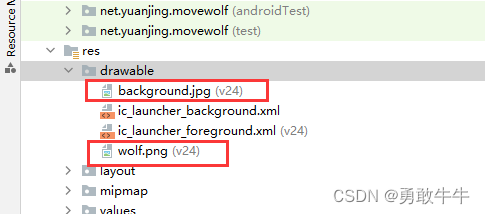
3.布局资源文件activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/root"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@drawable/background" >
<ImageView
android:id="@+id/ivMickey"
android:layout_width="100dp"
android:layout_height="120dp"
android:scaleType="fitXY"
android:src="@drawable/wolf" />
</LinearLayout>
4.主界面类MainActivity.java
package net.yuanjing.movewolf;
import android.os.Bundle;
import android.util.Log;
import android.view.MotionEvent;
import android.view.View;
import android.widget.ImageView;
import android.widget.LinearLayout;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
protected static final String TAG = "move_mickey_by_touch";
private ImageView ivWolf;
private LinearLayout root;
private LinearLayout.LayoutParams LayoutParams;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ivWolf = (ImageView) findViewById(R.id.ivWolf);
root = (LinearLayout) findViewById(R.id.root);
root.setFocusable(true);
root.requestFocus();
LayoutParams = (LinearLayout.LayoutParams) ivWolf.getLayoutParams();
root.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
Log.d(TAG, "ACTION_DOWN(" + event.getX() + ", " + event.getY() + ")");
break;
case MotionEvent.ACTION_MOVE:
Log.d(TAG, "ACTION_MOVE(" + event.getX() + ", " + event.getY() + ")");
break;
case MotionEvent.ACTION_UP:
Log.d(TAG, "ACTION_UP(" + event.getX() + ", " + event.getY() + ")");
}
ivWolf.setX(event.getX() - ivWolf.getWidth() / 2);
ivWolf.setY(event.getY() - ivWolf.getHeight() / 2);
return true;
}
});
}
}
5.运行的效果
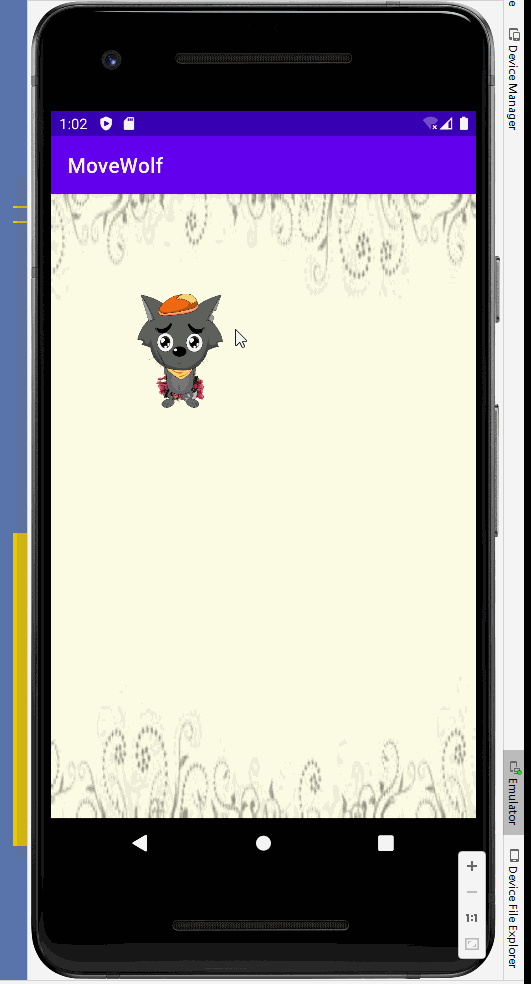