学习时间:
2023年1月20日
题目描述:
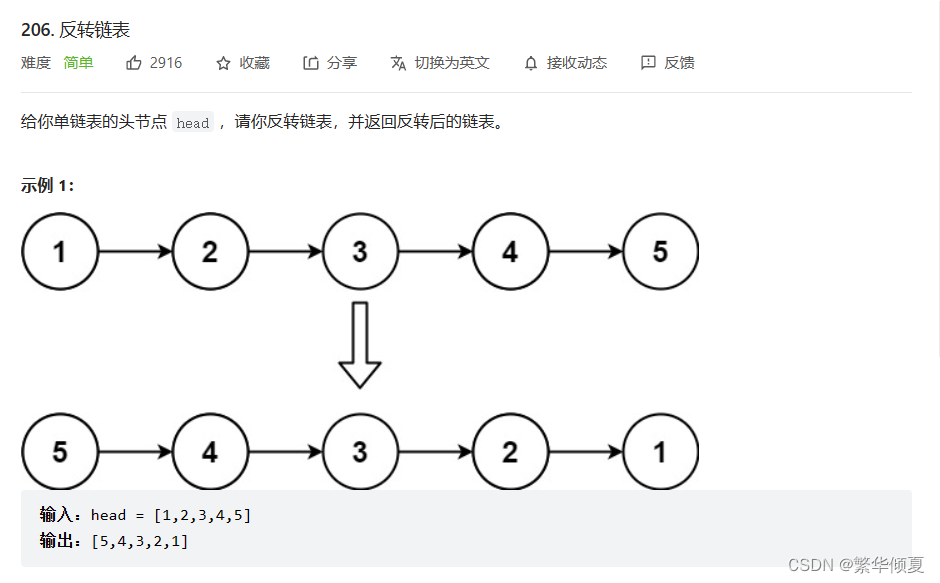
题解分享:
// 作 者 : 繁 华 倾 夏
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h> // 调用malloc函数
// 力扣(LeetCode):206. 反转链表
// Definition for singly-linked list. 单向链表的定义
struct ListNode {
int val;
struct ListNode *next;
};
// 方法一:改变链表指针
// head:链表头节点
struct ListNode* reverseList1(struct ListNode* head) {
if (head == NULL) { // 判断是否传入的空链表
return NULL;
}
struct ListNode* n1, * n2, * n3; // 新建三个链表指针
n1 = NULL;
n2 = head;
n3 = head->next;
while (n2) { // n2判断链表是否有数据
n2->next = n1;
n1 = n2;
n2 = n3;
if (n3) { // 当n3为NULL时,没有下一个next了,不能再指向next
n3 = n3->next;
}
}
return n1;
}
// 方法二:建立new链表
// head:链表头节点
struct ListNode* reverseList2(struct ListNode* head) {
struct ListNode* cur = head;
struct ListNode* newhead = NULL; // 新链表
while (cur) { // 遍历旧链表
struct ListNode* next = cur->next; // 因为cur每回都会变动,所以新建指针指向cur的下一个节点
// 头插法
cur->next = newhead; // next指向新节点
newhead = cur; // 复制节点
// 迭代
cur = next; // 间接使用next节点,指向cur的下一个节点
}
return newhead; // 返回新链表
}
// 从尾部插入数据
void SListPushBack(struct ListNode** pphead, int x) {
struct ListNode* newnode = (struct ListNode*)malloc(sizeof(struct ListNode));
newnode->val = x;
newnode->next = NULL;
if (*pphead == NULL) {
*pphead = newnode;
}
else {
// 找到尾节点
struct ListNode* tail = *pphead;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = newnode;
}
}
// 打印链表
void SListPrint(struct ListNode* phead)
{
struct ListNode* cur = phead;
while (cur != NULL) {
printf("%d->", cur->val);
cur = cur->next;
}
printf("NULL\n");
}
// 测试用例
// 输入 head = [1, 2, 3, 4, 5]
// 输出 [5, 4, 3, 2, 1]
int main() {
struct ListNode* plist = NULL; // 建立空链表
SListPushBack(&plist, 1); // 为链表插入数据
SListPushBack(&plist, 2);
SListPushBack(&plist, 3);
SListPushBack(&plist, 4);
SListPushBack(&plist, 5);
struct ListNode* newhead = reverseList1(plist);
//struct ListNode* newhead = reverseList2(plist);
SListPrint(newhead); // 打印链表
return 0;
}
【繁华倾夏】【每日力扣题解分享】【Day6】