分别创建了zhigonng类和manage类。
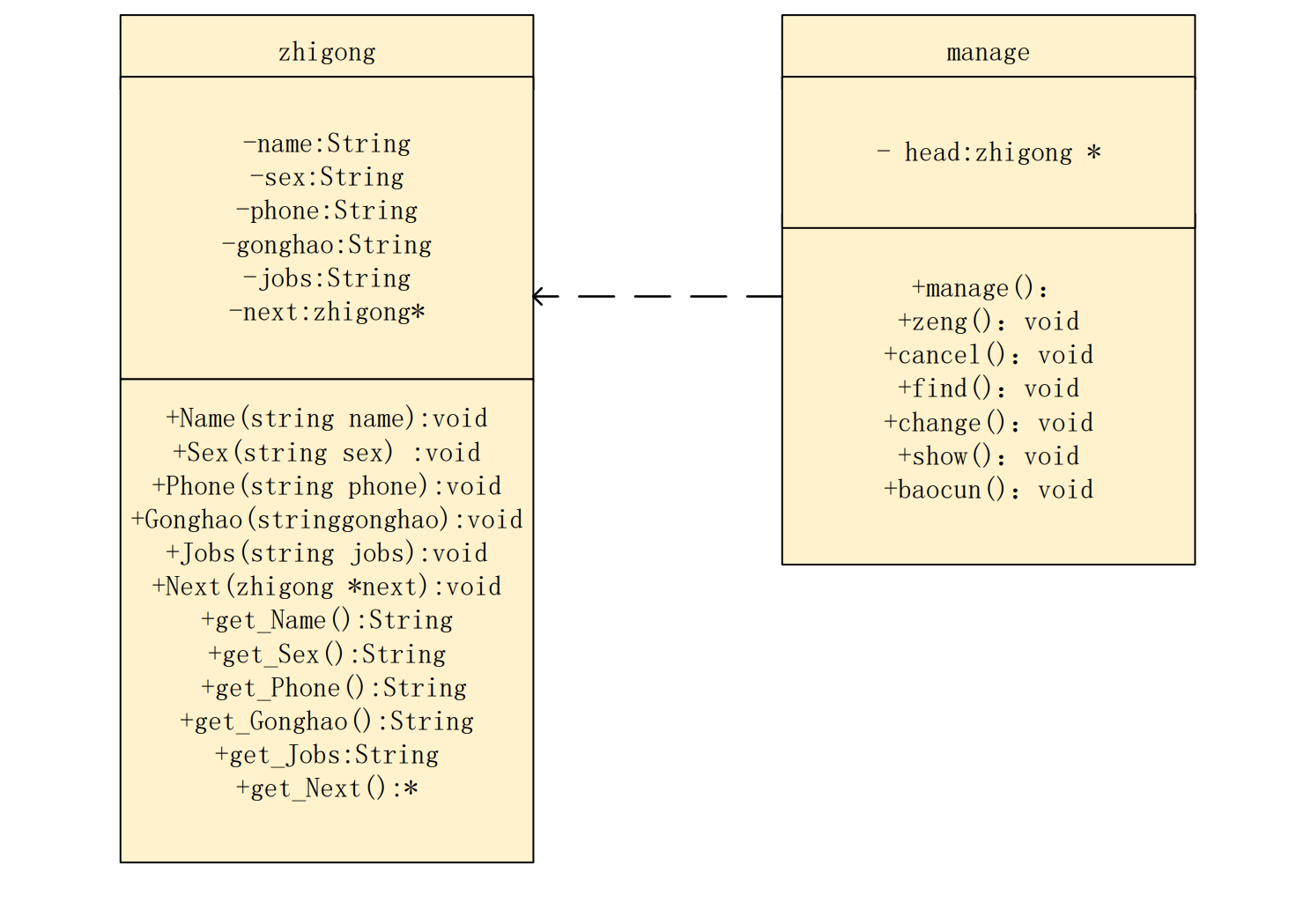
zhigong类存储职工姓名、性别、工号、电话、工种信息,有以下成员:
class zhigong
{
public:
void Name(string name) { //姓名
this->name=name;
}
void Sex(string sex) { //性别
this->sex=sex;
}
void Phone(string phone){ //电话
this->phone=phone;
}
void Gonghao(string gonghao){ //工号
this->gonghao=gonghao;
}
void Jobs(string jobs){ //工种
this->jobs=jobs;
}
void Next(zhigong *next) { //next指针
this->next=next;
}
string get_Name(){
return name;
}
string get_Sex(){
return sex;
}
string get_Phone(){
return phone;
}
string get_Gonghao(){
return gonghao;
}
string get_Jobs(){
return jobs;
}
zhigong *get_Next() {
return next;
}
private:
string name,sex,phone,gonghao,jobs;
zhigong *next;
};
manage类用来操作,有以下成员,具体每个成员函数怎么实现由后面的整体源代码展示
class manage
{
public:
manage(); //为头指针创建空间
void zeng(); //新增职工信息
void cancel(); //删除职工信息
void find(); //查找职工信息
void change(); //修改职工信息
void show(); //显示所有职工信息
void baocen(); //以文件保存所有信息
private:
zhigong *head;
};
源代码:
#include<iostream>
#include<stdlib.h>
#include<conio.h>
#include<time.h>
#include<string>
#include<fstream>
#include<windows.h>
using namespace std;
class zhigong
{
public:
void Name(string name) { //姓名
this->name=name;
}
void Sex(string sex) { //性别
this->sex=sex;
}
void Phone(string phone){ //电话
this->phone=phone;
}
void Gonghao(string gonghao){ //工号
this->gonghao=gonghao;
}
void Jobs(string jobs){ //工种
this->jobs=jobs;
}
void Next(zhigong *next) { //next指针
this->next=next;
}
string get_Name(){
return name;
}
string get_Sex(){
return sex;
}
string get_Phone(){
return phone;
}
string get_Gonghao(){
return gonghao;
}
string get_Jobs(){
return jobs;
}
zhigong *get_Next() {
return next;
}
private:
string name,sex,phone,gonghao,jobs;
zhigong *next;
};
class manage
{
public:
manage(); //为头指针创建空间
void zeng(); //新增职工信息
void cancel(); //删除职工信息
void find(); //查找职工信息
void change(); //修改职工信息
void show(); //显示所有职工信息
void baocen(); //以文件保存所有信息
private:
zhigong *head;
};
manage::manage() //为头指针创建空间
{
head=new zhigong;
head->Next(NULL);
}
void manage::zeng() //新增职工信息
{
while(1)
{
string name,sex,phone,gonghao,jobs;
zhigong *p,*tail,*q;
tail=head;
q=NULL;
while(tail->get_Next() != NULL){ //使结点循环到最后
tail=tail->get_Next();
}
p=new zhigong;
cout<<"请输入职工姓名:";
cin>>name;
p->Name(name);
cout<<"请输入该职工性别:";
cin>>sex;
p->Sex(sex);
cout<<"请输入该职工工号:";
zhigong *r=head;
int y=0;
cin>>gonghao;
while(r->get_Next()!=NULL){
if(r->get_Next()->get_Gonghao()==gonghao){
y=2;
cout<<"已有该工号,请重新输入:"<<endl;
break;
}
r=r->get_Next();
}
if(y==2){
continue;
}
p->Gonghao(gonghao);
cout<<"请输入该职工电话号码:";
cin>>phone;
p->Phone(phone);
cout<<"请输入该职工工种:";
cin>>jobs;
p->Jobs(jobs);
tail->Next(p); //与链表连接
p->Next(q); //
int a;
cout<<"继续添加请输入1,结束请输入0";
while(1){
cin>>a;
if(a==1||a==0)
{
break;
}else
{
cout<<"请输入0或1";
continue;
}
}
if(a==1){
continue;
}else if(a==0)
{
system("pause");
system("cls");
break;
}
}
}
void manage::cancel()
{
int x=1;
string gonghao;
zhigong *p;
while(x==1)
{
p=head;
int y=1;
cout<<"请输入你所需要删除的工号:";
cin>>gonghao;
while(p->get_Next()!=NULL)
{
if(p->get_Next()->get_Gonghao()==gonghao)
{
y=2;
zhigong *q=NULL;
q=p->get_Next();
p->Next(q->get_Next());
delete q;
q=NULL;
break;
}
p=p->get_Next();
}
if(y==2){
cout<<"删除成功"<<endl;
cout<<"继续删除输入1 结束删除输入任意数字";
cin>>x;
}else{
cout<<"无此工号"<<endl;
cout<<"继续删除输入1 结束删除输入任意数字";
cin>>x;
}
if(x!=1)
{
system("pause");
system("cls");
break;
}
}
}
void manage::find()
{
int x=1;
string name;
zhigong *p;
while(1)
{
int y=0;
p=head;
cout<<"请输入需要查询的工人姓名:";
cin>>name;
while(p->get_Next()!=NULL)
{
if(p->get_Next()->get_Name()==name)
{
y=1;
cout<<"姓名:"<<p->get_Next()->get_Name()<<endl;
cout<<"性别:"<<p->get_Next()->get_Sex()<<endl;
cout<<"工号:"<<p->get_Next()->get_Gonghao()<<endl;
cout<<"电话:"<<p->get_Next()->get_Phone()<<endl;
cout<<"工种:"<<p->get_Next()->get_Jobs()<<endl;
break;
}
p=p->get_Next();
}
if(y==0){
cout<<"查无此人"<<endl;
cout<<"继续查询请输入1 结束查询请输入任意数字";
cin>>x;
}else{
cout<<"查询成功"<<endl;
cout<<"继续查询请输入1 结束查询请输入任意数字";
cin>>x;
}
if(x!=1)
{
system("pause");
system("cls");
break;
}
}
}
void manage::change()
{
string name,sex,phone,gonghao,jobs;
int x=1;
string name1;
zhigong *p;
while(1)
{
int y=0;
p=head;
cout<<"请输入需要修改的工人姓名:";
cin>>name1;
while(p->get_Next()!=NULL)
{
if(p->get_Next()->get_Name()==name1)
{
y=1;
cout<<"姓名:"<<p->get_Next()->get_Name()<<endl;
cout<<"性别:"<<p->get_Next()->get_Sex()<<endl;
cout<<"工号:"<<p->get_Next()->get_Gonghao()<<endl;
cout<<"电话:"<<p->get_Next()->get_Phone()<<endl;
cout<<"工种:"<<p->get_Next()->get_Jobs()<<endl;
cout<<"请输入修改后的信息"<<endl;
cout<<"请输入职工姓名:";
cin>>name;
p->get_Next()->Name(name);
cout<<"请输入该职工性别:";
cin>>sex;
p->get_Next()->Sex(sex);
cout<<"请输入该职工工号:";
cin>>gonghao;
p->get_Next()->Gonghao(gonghao);
cout<<"请输入该职工电话号码:";
cin>>phone;
p->get_Next()->Phone(phone);
cout<<"请输入该职工工种:";
cin>>jobs;
p->get_Next()->Jobs(jobs);
break;
}
p=p->get_Next();
}
if(y==0){
cout<<"查无此人"<<endl;
cout<<"继续查询请输入1 结束查询请输入任意数字";
cin>>x;
}else{
cout<<"修改成功"<<endl;
cout<<"继续查询请输入1 结束查询请输入任意数字";
cin>>x;
}
if(x!=1)
{
system("pause");
system("cls");
break;
}
}
}
void manage::show()
{
zhigong *p=head;
while(p->get_Next()!=NULL)
{
cout<<"姓名:"<<p->get_Next()->get_Name()<<endl;
cout<<"性别:"<<p->get_Next()->get_Sex()<<endl;
cout<<"工号:"<<p->get_Next()->get_Gonghao()<<endl;
cout<<"电话:"<<p->get_Next()->get_Phone()<<endl;
cout<<"工种:"<<p->get_Next()->get_Jobs()<<endl<<endl;
p=p->get_Next();
}
system("pause");
system("cls");
}
void manage::baocen()
{
zhigong *p=head->get_Next();
ofstream outfile;
outfile.open("职工信息.txt",ios::out);
while(p!=NULL)
{
outfile<<"姓名:"<<p->get_Name()<<"\t"<<"性别:"<<p->get_Sex()<<"\t"
<<"工号:"<<p->get_Gonghao()<<"\t"<<"电话:"<<p->get_Phone()<<"\t"<<"工种:"<<p->get_Jobs()<<endl;
p=p->get_Next();
}
outfile.close();
cout<<"职工信息保存成功"<<endl;
cout<<"正在退出请稍等......";
Sleep(1000);
}
int main()
{
manage M;
int tui=0;
// system("pause");
// system("cls");
while(1)
{
cout<<" =============================================================================\n";
cout<<"|| 职工基本信息管理系统 ||\n";
cout<<"||============================================================================||\n";
cout<<"||============================================================================||\n";
cout<<"|| 【1】--添加职工的信息 ||\n";
cout<<"|| 【2】--删除职工的信息 ||\n";
cout<<"|| 【3】--修改职工的信息 ||\n";
cout<<"|| 【4】--按工号查询职工信息 ||\n";
cout<<"|| 【5】--查看全部职工的信息 ||\n";
cout<<"|| 【0】--生成文件保存后退出系统 ||\n";
cout<<" ==============================================================================\n";
cout<<"请输入数字来选择对应的服务种类:";
int n;
if(!(cin>>n)) {
system("CLS");
cout<<"输入格式错误,请重新输入!"<<endl;
cin.clear();
cin.sync();
cin.ignore(); //清空缓存区
} else {
switch(n) {
case 1 :
M.zeng();
break;
case 2 :
M.cancel();
break;
case 3 :
M.change();
break;
case 4 :
M.find();
break;
case 5 :
M.show();
break;
case 0 :
M.baocen();
tui=1;
break;
default:
system("CLS");
cout<<"输入错误!没有此服务!请重新输入!"<<endl;
}
}
if(tui==1)
{
break;
}
}
}
本系统用链表类来实现,有别于结构体的链表,它体现出c++中面向对象的思想。