结构伪类选择器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>结构伪类选择器</title>
<style>
li:first-child {
background-color: pink;
}
li:last-child {
background-color: red;
}
li:nth-child(4) {
background-color: rebeccapurple;
}
li:nth-child(6) {
background-color: green;
}
</style>
</head>
<body>
<h3>结构伪类选择器</h3>
<strong>作用:</strong>根据元素的结构关系查找元素。 <br>
<img src="./img/结构伪类选择器.png" alt="">
<ul>
<li>E:first-child 查找第一个E元素</li>
<li>E:last-child 查找最后一个E元素</li>
<li>E:nth-child(N) 查找第N个E元素</li>
</ul>
<br><br>
<ul>
<li>这是li标签1,背景粉色</li>
<li>这是li标签2</li>
<li>这是li标签3</li>
<li>这是li标签4,背景紫色</li>
<li>这是li标签5</li>
<li>这是li标签6,背景绿色</li>
<li>这是li标签7</li>
<li>这是li标签8背景红色</li>
</ul>
</body>
</html>
结构伪类选择器公式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>结构伪类选择器-公式</title>
<style>
li:nth-child(2n){
background-color: green;
}
li:nth-child(2n+1) {
background-color: red;
}
li:nth-child(5n) {
background-color: rebeccapurple;
}
li:nth-child(n+5) {
background-color: pink;
}
li:nth-child(-n+5) {
background-color: blue;
}
</style>
</head>
<body>
<h3>结构伪类选择器-公式</h3>
<strong>作用:</strong>根据元素的结构关系多个元素<br>
<img src="./img/结构伪类选择器-公式.png" alt=""><br>
<ul>
<li>偶数标签 2n</li>
<li>奇数标签 2n+1;2n-1</li>
<li>找到5的倍数的标签 5n</li>
<li>找到第5个以后的标签 n+5</li>
<li>找到第5个以前的标签 -n+5</li>
</ul>
<br><br>
<ul>
<li>这是li标签1</li>
<li>这是li标签2</li>
<li>这是li标签3</li>
<li>这是li标签4</li>
<li>这是li标签5</li>
<li>这是li标签6</li>
<li>这是li标签7</li>
<li>这是li标签8</li>
</ul>
</body>
</html>
伪元素选择器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>伪元素选择器</title>
<style>
div {
width: 400px;
height: 300px;
background-color: pink;
}
div::before {
content: "这是div前面";
width: 100px;
height: 100px;
background-color: red;
display: block;
}
div::after {
content: "这是div后面";
width: 100px;
height: 100px;
background-color: blue;
display: inline-block;
}
</style>
</head>
<body>
<h3>伪元素选择器</h3>
<strong>作用:</strong>创建虚拟元素(伪元素),用来摆放装饰性内容。<br>
<ul>
<li>E::before 在E元素里面最前面添加一个伪元素</li>
<li>E::after 在E元素里面最后面添加一个伪元素</li>
</ul><br><strong>注意点</strong>
<ul>
<li>必须设置content:" "属性,用来 设置伪元素的内容,如果没有内容,则引号留空即可</li>
<li>伪元素默认是行内显示模式</li>
<li>权重和标签选择器相同</li>
</ul>
<br><br>
<div>这是div标签</div>
</body>
</html>
PxCook
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PxCook</title>
</head>
<body>
<h3>PxCook</h3>
<strong>PxCook(像素大厨)</strong> 是一款切图设计工具软件。支持PSD文件的文字、颜色、距离自动智能识别<br>
<ul>
<li>开发面板(自动智能识别)</li>
<li>设计面板(手动测量尺寸和颜色)</li>
</ul>
<br><br>
</body>
</html>
盒子模型-组成
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-组成</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
padding: 20px;
margin: 20px;
border: 1px solid #000;
}
</style>
</head>
<body>
<h3>盒子模型-组成</h3>
<strong>作用:</strong>布局网页,摆放盒子和内容<br>
<strong>重要组成部分:</strong>
<ul>
<li>内容区域:width&height</li>
<li>内边距:padding(内容和盒子中间)</li>
<li>边框线:border</li>
<li>外边框:margin(出现在盒子外面)</li>
</ul><br>
<img src="./img/盒子模型-组成.png" alt="">
<div>这是div标签</div>
</body>
</html>
盒子模型-边框线
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-边框线</title>
<style>
.div1 {
width: 200px;
height: 200px;
background-color: red;
padding: 20px;
margin: 20px;
border: 5px solid green;
}
.div2 {
width: 200px;
height: 200px;
background-color: red;
padding: 20px;
margin: 20px;
border: 5px dashed orange;
}
.div3 {
width: 200px;
height: 200px;
background-color: red;
padding: 20px;
margin: 20px;
border: 5px dotted blue;
}
</style>
</head>
<body>
<h3>盒子模型-边框线</h3>
<strong>属性名:</strong>border (bd) <br>
<strong>属性值:</strong>边框线粗细 线条样式 颜色 (不区分顺序) <br>
<strong>常用线条样式</strong>
<ul>
<li>solid 实线</li>
<li>dashed 虚线</li>
<li>dotted 点线</li>
</ul>
<br>
<div class="div1">这是实线div标签</div>
<div class="div2">这是虚线div标签</div>
<div class="div3">这是点线div标签</div>
</body>
</html>
盒子模型-单方向边框线
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-单方向边框线</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
padding: 20px;
margin: 20px;
border-top: 5px dotted pink;
border-bottom: 5px dashed bisque;
border-left: 5px solid #170202;
border-right: 5px solid #3014ae;
}
</style>
</head>
<body>
<h3>盒子模型-单方向边框线</h3>
<strong>属性名:</strong>border-方位名词 (bd+方位名词首字母,例如:bdl) <br>
<strong>属性值:</strong>边框线粗细 线条样式 颜色 (不区分顺序) <br>
<strong>常用线条样式</strong>
<ul>
<li>solid 实线</li>
<li>dashed 虚线</li>
<li>dotted 点线</li>
</ul>
<br>
<div>这是div标签</div>
</body>
</html>
盒子模型-内边距
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-内边距</title>
<style>
div {
width: 200px;
height: 200px;
background-color: red;
padding-top: 10px;
padding-bottom: 20px;
padding-left: 30px;
padding-right: 80px;
margin: 20px;
border-top: 5px dotted pink;
border-bottom: 5px dashed bisque;
border-left: 5px solid #170202;
border-right: 5px solid #3014ae;
}
</style>
</head>
<body>
<h3>盒子模型-内边距</h3>
<strong>作用:</strong>设置内容与盒子边缘距离<br>
<strong>属性名:</strong>padding/padding-方位名词 <br>
<strong>属性值:</strong>边框线粗细 线条样式 颜色 (不区分顺序) <br>
<img src="./img/盒子模型-内边距.png" alt="">
<br>
<div>这是div标签</div>
</body>
</html>
盒子模型-尺寸计算
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-尺寸计算</title>
<style>
.div1 {
width: 200px;
height: 200px;
background-color: pink;
padding: 20px;
}
.div2 {
width: 160px;
height: 160px;
background-color: pink;
padding: 20px;
}
.div3 {
width: 200px;
height: 200px;
background-color: pink;
padding: 20px;
box-sizing: border-box;
}
</style>
</head>
<body>
<h3>盒子模型-尺寸计算</h3>
<strong>默认情况:</strong>盒子尺寸 =内容尺寸 + border 尺寸 +内边距尺寸<br>
<strong>结论:</strong>给盒子加border/padding会撑大盒子<br>
<strong>解决:</strong>
<ul>
<li>手动做减法,减掉 border /padding 的尺寸</li>
<li>内减模式: box-sizing: border-box</li>
</ul>
<img src="./img/盒子模型-尺寸计算.png" alt="">
<br>
<strong>原图放大</strong><br>
<div class="div1">这是div标签200+20+20</div>
<strong>手动计算</strong><br>
<div class="div2">这是div标签160+20+20</div>
<strong>内建模式</strong><br>
<div class="div3">这是div标签,内减模式:(box-sizing: border-box;)</div>
</body>
</html>
盒子模型-外边距
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-外边距</title>
<style>
.div1 {
width: 1000px;
height: 200px;
background-color: pink;
margin: 50px;
}
.div2 {
width: 1000px;
height: 200px;
background-color: pink;
margin-left: 60px;
margin-top: 80px;
margin-right: 50px;
margin-bottom: 70px;
}
.div3 {
width: 1000px;
height: 200px;
background-color: pink;
margin: 80px 90px 100px 110px;
}
</style>
</head>
<body>
<h3>盒子模型-外边距</h3>
<strong>作用:</strong>拉开两个盒子之间的距离<br>
<strong>属性名:</strong>margin (m)<br>
<strong>属性值:</strong>边框线粗细 线条样式 颜色 (不区分顺序) <br>
<strong>与paddind属性值写法含义相同</strong><br>
--------------------------------------------------
<div class="div1">这是div标签1000*200,外边距 margin: 50px;</div>
--------------------------------------------------
<div class="div2">这是div标签1000*200,外边距margin-left: 60px;margin-top: 80px; margin-right: 50px; margin-bottom: 70px;</div>
--------------------------------------------------
<div class="div3">这是div标签1000*200,外边距margin: 80px 90px 100px 110px;</div>
</body>
</html>
版心居中
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-版心居中</title>
<style>
div {
width: 1000px;
height: 200px;
background-color: pink;
margin: 0px auto;
}
</style>
</head>
<body>
<h3>盒子模型-版心居中</h3>
<strong>要求:</strong>盒子有要求<br>
margin: 0px auto;<br>
<div>这是</div>
</body>
</html>
清除默认样式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除默认样式</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
li {
list-style: none;
}
</style>
</head>
<body>
<h3>清除默认样式</h3>
清除默认样式,比如:默认的内外边距<br>
<strong>去掉列表的点 </strong>list-style: none;<br>
<h1>这是h1标签</h1>
<p>这是p标签</p>
<ul>
<li>这是li标签</li>
</ul>
</body>
</html>
盒子模型-元素溢出
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-元素溢出</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
.div1 {
overflow: hidden;
}
.div2 {
overflow: scroll;
}
.div3 {
overflow: auto;
}
</style>
</head>
<body>
<h3>盒子模型-元素溢出</h3>
<strong>作用:</strong>控制溢出元素内容的显示模式<br>
<strong>属性名:</strong>overflow <br>
<strong>属性值:</strong><br>
<ul>
<li>hidden 溢出隐藏</li>
<li>scroll 溢出滚动(无论是否溢出,都显示浪动条位置)</li>
<li>auto 溢出滚动(溢出才显示滚动条位置)</li>
</ul>
<img src="./img/盒子模型-元素溢出.png" alt=""><br>
<br>
<div class="div1"><strong>hidden 溢出隐藏</strong>这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签
这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签</div><br>
<div class="div2"><strong>scroll 溢出滚动(无论是否溢出,都显示浪动条位置)</strong>这是div标签这是div标签
这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签</div><br>
<div class="div3"><strong>auto 溢出滚动(溢出才显示滚动条位置)</strong>这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签
这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签这是div标签</div><br>
</body>
</html>
外边距问题-合并现象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>外边距问题-合并现象</title>
<style>
div {
width: 100px;
height: 100px;
}
.div1 {
background-color: red;
margin-bottom: 50px;
}
.div2 {
background-color: orange;
margin-top: 20px;
}
</style>
</head>
<body>
<h3>外边距问题-合并现象</h3>
<strong>场景:</strong>垂直排列的兄弟元素,上下 margin 会合并<br>
<strong>现象:</strong>取两个 margin 中的较大值生效<br>
<br>
<strong>以下情况外边距50px</strong>
<div class="div1">这是div1,下边距50px</div>
<div class="div2">这是div2,上边距20px</div>
</body>
</html>
外边距问题-塌陷问题
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>外边距问题-塌陷问题</title>
<style>
.div1 {
width: 300px;
height: 300px;
background-color: red;
padding-top: 0px;
}
.div2 {
width: 100px;
height: 100px;
background-color: orange;
margin-top: 50px;
}
.div3 {
width: 300px;
height: 300px;
background-color: red;
overflow: hidden;
}
.div4 {
width: 300px;
height: 300px;
background-color: red;
border-top: 1px solid #000;
}
</style>
</head>
<body>
<h3>外边距问题-塌陷问题</h3>
<strong>场景:</strong>父子级的标签,子级的添加 上外边距 会产生塌陷问题<br>
<strong>现象:</strong>导致父级一起向下移动<br>
<strong>解决办法:</strong>
<ul>
<li>取消父级margin,父级设置padding(推荐这个)</li>
<li>父级设置overflow:hidden</li>
<li>父级设置border-top</li>
</ul>
<br>
<strong>以下情况外边距50px</strong>
<div class="div1">
<div class="div2">这是div2,上边距50px</div>
</div>
<hr>
<div class="div3">
<div class="div2">这是div2,上边距50px</div>
</div>
<hr>
<div class="div4">
<div class="div2">这是div2,上边距50px</div>
</div>
</body>
</html>
行内元素-内外边距问题
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>行内元素-内外边距问题</title>
<style>
span {
margin: 50px;
padding: 50px;
background-color: pink;
}
.span1 {
line-height: 30px;
}
</style>
</head>
<body>
<h3>行内元素-内外边距问题</h3>
<strong>场景:</strong>行内元素添加 margin 和 padding,无法改变元素垂直位置<br>
<strong>解决办法:</strong>给行内元素添加 line-height 可以改变垂直位置 <br>
<br>
<span>这是span标签</span><br><br><br><br><br><br>
<span class="span1">这是span标签</span><br>
</body>
</html>
盒子模型-圆角
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-圆角</title>
<style>
div {
margin: 50px auto;
width: 200px;
height: 200px;
background-color: pink;
}
.div1 {
border-radius: 10px 20px 30px 40px;
}
.div2 {
border-radius: 20px 30px 40px;
}
.div3 {
border-radius: 30px 40px;
}
.div4 {
border-radius: 40px;
}
</style>
</head>
<body>
<h3>盒子模型-圆角</h3>
<strong>作用: </strong>设置元素的外边框为圆角。<br>
<strong>属性名: </strong>border-radius <br>
<strong>属性值: </strong>数字+px / 百分比<br>
多值:
<ul>
<li>四值:border-radius: 10px 20px 30px 40px;,左上,右上,右下,左下</li>
<li>三值:border-radius: 20px 30px 40px;,左上,右上左下,右下</li>
<li>两值:border-radius: 30px 40px;,左上右下,右上左下</li>
<li>一值:border-radius: 40px;,左上右下右上左下</li>
</ul>
<br>
<div class="div1">这是div标签(border-radius: 10px 20px 30px 40px;)</div>
<div class="div2">这是div标签(border-radius: 20px 30px 40px;)</div>
<div class="div3">这是div标签(border-radius: 30px 40px;)</div>
<div class="div4">这是div标签(border-radius: 40px;)</div>
</body>
</html>
盒子模型-圆角常见应用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-圆角常见应用</title>
<style>
.img1 {
width: 200px;
height: 200px;
border-radius: 100px;
}
div {
width: 200px;
height: 80px;
background-color: pink;
border-radius: 40px;
text-align: center;
line-height: 80px;
}
</style>
</head>
<body>
<h3>盒子模型-圆角常见应用</h3>
<strong>常见应用</strong>
<ul>
<li>常见应用-正圆形状:给正方形盒子设置圆角属性值为 宽高的一半/50% <br>
最大值50% <br>
<img src="./img/盒子模型-圆角常见应用-头像.png" alt=""><img src="./img/盒子模型-圆角常见应用-头像2.png" alt="">
</li>
<li>常见应用 - 胶囊形状:给长方形盒子设置圆角属性值为 盒子高度的一半 <br>
<img src="./img/盒子模型-圆角常见应用-胶囊.png" alt=""><img src="./img/盒子模型-圆角常见应用-胶囊2.png" alt="">
</li>
</ul><br><br>
<img src="./img/beijing.png" class="img1">
<div>这是div标签</div>
</body>
</html>
盒子模型-阴影
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>盒子模型-阴影(拓展)</title>
<style>
div {
margin: 50px auto;
width: 200px;
height: 80px;
background-color: orange;
box-shadow: 2px 5px 10px 1px rgba(0, 0, 0, 0.5) ;
border-radius: 40px;
text-align: center;
line-height: 80px;
}
</style>
</head>
<body>
<h3>盒子模型-阴影(拓展)</h3>
<strong>作用:</strong>给元素设置阴影效果<br>
<strong>属性名:</strong>box-shadow <br>
<strong>属性值:</strong>X轴偏移量 Y轴偏移量 模糊半径 扩散半径 颜色 内外阴影 <br>
<strong>注意:</strong><ul>
<li>X轴偏移量 和Y 轴偏移量必须书写</li>
<li>默认是外阴影,内阴影需要添加 inset</li>
</ul><br>
<br>
<div>这是div标签</div>
</body>
</html>
案例
产品卡片
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>案例-产品卡片</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
background-color: #f1f1f1;
}
.product {
width: 270px;
height: 253px;
background-color: #fff;
margin: 50px auto;
padding-top: 40px;
text-align: center;
border-radius: 10px;
}
img {
margin: auto;
}
.product h4 {
margin-top: 20px;
margin-bottom: 12px;
font-size: 18px;
color: #333;
font-weight: 400;
}
.product p {
font-size: 12px;
color: #555;
}
</style>
</head>
<body>
<div class="product">
<img src="./img/liveSDK.svg" alt=""><br>
<h4>抖音直播SDK</h4>
<p>包含抖音直播看播功能</p>
</div>
</body>
</html>
案例-新闻列表
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>案例-新闻列表</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
li {
list-style: none;
}
a {
text-decoration: none;
}
.news {
width: 360px;
height: 200px;
margin: 100px auto;
}
.news .hd {
height: 34px;
background: #eee;
border: 1px solid #dbdee1;
border-left: none ;
}
.news .hd a {
margin: -1px;
display: block;
border-top: 3px solid #ff8400;
border-right: 1px solid #dbdee1;
width: 48px;
height: 34px;
background-color: #fff;
text-align: center;
line-height: 32px;
font-size: 14px;
color: #333;
}
.news .bd {
padding: 5px;
}
.news .bd li {
background-image: url(./img/square.png);
background-repeat: no-repeat;
background-position: 0 center;
padding-left: 15px;
}
.news .bd li a {
background-image: url(./img/img.gif);
background-repeat: no-repeat;
background-position: 0 center;
padding-left: 20px;
font-size: 12px;
color: #666;
line-height: 24px;
}
.news .bd li a:hover {
color: #ff8400;
}
</style>
</head>
<body>
<div class="news">
<div class="hd"><a href="#">新闻</a></div>
<div class="bd">
<ul>
<li><a href="#">点赞“新农人” 温暖的伸手</a></li>
<li><a href="#">在希望的田野上...</a></li>
<li><a href="#">“中国天眼”又有新发现 已在《自然》杂志发表</a></li>
<li><a href="#">急!这个领域,缺人!月薪4万元还不好招!啥情况?</a></li>
<li><a href="#">G9“带货”背后:亏损面持续扩大,竞争环境激烈</a></li>
<li><a href="#">多地力推二手房“带押过户”,有什么好处?</a></li>
</ul>
</div>
</div>
</body>
</html>
CSS书写顺序
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS书写顺序</title>
</head>
<body>
<h3>CSS书写顺序</h3>
<ul>
<li>盒子模型属性</li>
<li>文字样式</li>
<li>圆角、阴影等修饰属性</li>
</ul>
</body>
</html>
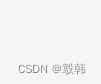