-
打印输出:
pythonCopy code
print("Hello, world!")
-
变量赋值:
pythonCopy code
x = 10
-
基本数学运算:
pythonCopy code
addition = 5 + 5 subtraction = 10 - 5 multiplication = 4 * 3 division = 8 / 2 modulus = 7 % 3 exponentiation = 2 ** 3
-
字符串拼接:
pythonCopy code
greeting = "Hello" + " " + "World!"
-
列表创建:
pythonCopy code
my_list = [1, 2, 3, 4, 5]
-
列表添加元素:
pythonCopy code
my_list.append(6)
-
列表访问元素:
pythonCopy code
first_element = my_list[0]
-
列表切片:
pythonCopy code
sublist = my_list[1:3]
-
循环遍历列表:
pythonCopy code
for item in my_list: print(item)
-
条件语句:
pythonCopy code
if x > 5: print("x is greater than 5") elif x == 5: print("x is 5") else: print("x is less than 5")
-
字典创建和访问:
pythonCopy code
my_dict = {"name": "John", "age": 30} name = my_dict["name"]
-
字典添加或修改元素:
pythonCopy code
my_dict["city"] = "New York"
-
字典中的循环:
pythonCopy code
for key, value in my_dict.items(): print(f"{key}: {value}")
-
使用range函数:
pythonCopy code
for i in range(5): print(i)
-
列表推导式:
pythonCopy code
squares = [x**2 for x in range(10)]
-
函数定义和调用:
pythonCopy code
def greet(name): return f"Hello, {name}!" greeting = greet("Alice")
-
使用*args和kwargs**:
pythonCopy code
def function_with_args(*args, **kwargs): print(args) print(kwargs) function_with_args(1, 2, 3, key1="value1", key2="value2")
-
类定义和实例化:
pythonCopy code
class MyClass: def __init__(self, name): self.name = name def greet(self): return f"Hello, {self.name}!" my_object = MyClass("Bob") print(my_object.greet())
-
文件读写:
pythonCopy code
# 写入文件 with open("myfile.txt", "w") as file: file.write("Hello, file world!") # 读取文件 with open("myfile.txt", "r") as file: content = file.read() print(content)
-
错误和异常处理:
pythonCopy code
try: result = 10 / 0 except ZeroDivisionError: print("Divided by zero!") finally: print("This code block is always executed")
-
使用enumerate:
pythonCopy code
for index, value in enumerate(["a", "b", "c"]): print(index, value)
-
集合创建和操作:
pythonCopy code
my_set = {1, 2, 3} my_set.add(4) my_set.remove(2)
-
集合运算:
pythonCopy code
set_a = {1, 2, 3} set_b = {
100个Python代码(一)
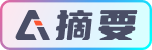