A - ABC/ARC
Time limit : 2sec / Memory limit : 256MB
Score : 100 points
Problem Statement
Smeke has decided to participate in AtCoder Beginner Contest (ABC) if his current rating is less than 1200, and participate in AtCoder Regular Contest (ARC) otherwise.
You are given Smeke's current rating, x. Print ABC
if Smeke will participate in ABC, and print ARC
otherwise.
Constraints
- 1≦x≦3,000
- x is an integer.
Input
The input is given from Standard Input in the following format:
x
出力
Print the answer.
Sample Input 1
1000
Sample Output 1
ABC
Smeke's current rating is less than 1200, thus the output should be ABC
.
Sample Input 2
2000
Sample Output 2
ARC
Smeke's current rating is not less than 1200, thus the output should be ARC
.
1200作为一个标杆,1200以下的参加ABC,1200(包括1200)以上的参加ARC.输入一个值x,让你输出参加的场次。
Ac代码:
#include<stdio.h>
#include<string.h>
using namespace std;
int main()
{
int n;
while(~scanf("%d",&n))
{
if(n<1200)printf("ABC\n");
else printf("ARC\n");
}
}
B - A to Z String
Time limit : 2sec / Memory limit : 256MB
Score : 200 points
Problem Statement
Snuke has decided to construct a string that starts with A
and ends with Z
, by taking out a substring of a string s (that is, a consecutive part of s).
Find the greatest length of the string Snuke can construct. Here, the test set guarantees that there always exists a substring of s that starts with A
and ends withZ
.
Constraints
- 1≦|s|≦200,000
- s consists of uppercase English letters.
- There exists a substring of s that starts with
A
and ends withZ
.
Input
The input is given from Standard Input in the following format:
s
Output
Print the answer.
Sample Input 1
QWERTYASDFZXCV
Sample Output 1
5
By taking out the seventh through eleventh characters, it is possible to construct ASDFZ
, which starts with A
and ends with Z
.
Sample Input 2
ZABCZ
Sample Output 2
4
Sample Input 3
HASFJGHOGAKZZFEGA
Sample Output 3
12
思路:
维护最左边的A的位子,以及最右边Z的位子即可。
Ac代码:
#include<stdio.h>
#include<string.h>
using namespace std;
char a[200050];
int main()
{
while(~scanf("%s",a))
{
int pre=-1;
int back=-1;
int n=strlen(a);
for(int i=0;i<n;i++)
{
if(a[i]=='A'&&pre==-1)pre=i;
if(a[i]=='Z')back=i;
}
if(pre==-1||back==-1)
{
printf("0\n");
}
else printf("%d\n",back-pre+1);
}
}
C - X: Yet Another Die Game
Time limit : 2sec / Memory limit : 256MB
Score : 300 points
Problem Statement
Snuke has decided to play with a six-sided die. Each of its six sides shows an integer 1 through 6, and two numbers on opposite sides always add up to 7.
Snuke will first put the die on the table with an arbitrary side facing upward, then repeatedly perform the following operation:
- Operation: Rotate the die 90° toward one of the following directions: left, right, front (the die will come closer) and back (the die will go farther). Then, obtain ypoints where y is the number written in the side facing upward.
For example, let us consider the situation where the side showing 1 faces upward, the near side shows 5 and the right side shows 4, as illustrated in the figure. If the die is rotated toward the right as shown in the figure, the side showing 3 will face upward. Besides, the side showing 4 will face upward if the die is rotated toward the left, the side showing 2 will face upward if the die is rotated toward the front, and the side showing 5 will face upward if the die is rotated toward the back.
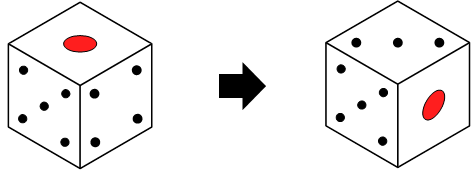
Find the minimum number of operation Snuke needs to perform in order to score at least x points in total.
Constraints
- 1≦x≦1015
- x is an integer.
Input
The input is given from Standard Input in the following format:
x
Output
Print the answer.
Sample Input 1
7
Sample Output 1
2
Sample Input 2
149696127901
Sample Output 2
27217477801
给你一个色子,初始的时候任意一个面朝上,每次操作可以将色子翻一下,并且得到此时面朝上的点数,
问你想要得到至少X分,需要多少次操作。
思路:
5和6两个数字是色子中数字最大的两个,那么每次旋转都按照6-5-6-5-6-5.........的顺序即可。
那么操作次数就很好计算了。
Ac代码:
#include<stdio.h>
#include<string.h>
using namespace std;
#define ll long long int
int main()
{
ll a;
while(~scanf("%lld",&a))
{
ll mod=11;
ll output=a/mod*2;
a%=mod;
if(a>=1&&a<=6)output++;
if(a>=7&&a<=10)output+=2;
printf("%lld\n",output);
}
}
D - Card Eater
Time limit : 2sec / Memory limit : 256MB
Score : 400 points
Problem Statement
Snuke has decided to play a game using cards. He has a deck consisting of N cards. On the i-th card from the top, an integer Ai is written.
He will perform the operation described below zero or more times, so that the values written on the remaining cards will be pairwise distinct. Find the maximum possible number of remaining cards. Here, N is odd, which guarantees that at least one card can be kept.
Operation: Take out three arbitrary cards from the deck. Among those three cards, eat two: one with the largest value, and another with the smallest value. Then, return the remaining one card to the deck.
Constraints
- 3≦N≦105
- N is odd.
- 1≦Ai≦105
- Ai is an integer.
Input
The input is given from Standard Input in the following format:
N A1 A2 A3 ... AN
Output
Print the answer.
Sample Input 1
5 1 2 1 3 7
Sample Output 1
3
One optimal solution is to perform the operation once, taking out two cards with 1 and one card with 2. One card with 1 and another with 2 will be eaten, and the remaining card with 1 will be returned to deck. Then, the values written on the remaining cards in the deck will be pairwise distinct: 1, 3 and 7.
Sample Input 2
15 1 3 5 2 1 3 2 8 8 6 2 6 11 1 1
Sample Output 2
7
给你N个卡片,每次操作可以任意拿三个卡片出来,去掉最大值和最小值的卡片,中间值卡片放回去。
问最多可以剩余几张卡片,并且使得不重复。
思路:
1、我们统计每种卡片都出现了多少次。接下来我们从小到大枚举卡片,如果一种卡片的数量大于1,那么我们再从大到小枚举一个卡片,也找到一个卡片数量大于1的数字,然后将两种卡片各减少一个(随意将一种卡片拿出来两个,另一种卡片拿出来一个,那么删除的两个其实就是一种卡片删除了一个);
一直到不能处理为止。
2、接下来我们继续处理残余问题,枚举每一种卡片,如果其数量为奇数,那么就可以每次拿三张自己这种卡片出来删除两个,总会剩下一个,所以这种卡片是不会重复的,
那么同理,如果是偶数剩余量,很显然这种残余卡片就要被彻底删除掉了。
3、模拟整个过程维护即可。
Ac代码:
#include<stdio.h>
#include<string.h>
#include<queue>
#include<algorithm>
using namespace std;
int a[100006];
int vis[100006];
int main()
{
int n;
while(~scanf("%d",&n))
{
memset(vis,0,sizeof(vis));
for(int i=0;i<n;i++)
{
scanf("%d",&a[i]);
vis[a[i]]++;
}
int j=100002;
for(int i=1;i<=100002;i++)
{
if(vis[i]>1)
{
while(vis[j]<=1&&j>i)j--;
if(j>i)
{
if(vis[i]==vis[j])vis[i]=1,vis[j]=1;
else if(vis[i]>vis[j])
{
vis[i]-=vis[j]-1;
vis[j]=1;
i--;
}
else if(vis[i]<vis[j])
{
vis[j]-=vis[i]-1;
vis[i]=1;
j++;
}
}
}
}
int output=0;
for(int i=1;i<=100002;i++)
{
if(vis[i]==1)output++;
if(vis[i]>1)
{
if(vis[i]%2==0)continue;
else output++;
}
}
printf("%d\n",output);
}
}