<html>
<head>
<meta content="charset=utf-8" />
<meta name="viewport" content="initial-scale=1, maximun-scale=1 user-scalable=no" />
<title>geometry</title>
<style>
html,
body,
#viewDiv {
padding: 0;
margin: 0;
height: 100%;
width: 100%;
}
</style>
<link rel="stylesheet" href="https://js.arcgis.com/4.23/esri/themes/light/main.css">
<script src="https://js.arcgis.com/4.23/"></script>
<script>7
require(["esri/Map", "esri/views/MapView", "esri/Graphic", "esri/layers/GraphicsLayer", "esri/widgets/Sketch/SketchViewModel"], (Map, MapView, Graphic, Graphicslayer, SketchViewModel) => {
/*新建绘图层*/
const tempGraphicsLayer = new Graphicslayer({
id: "tempGraphics"
});
/*创建地图*/
const map = new Map({
basemap: "hybrid",
layers:[tempGraphicsLayer] /*添加绘图层,如果要绘图层可以不要该属性*/
});
const view = new MapView({
center: [-80, 35],
container: "viewDiv",
map: map,
zoom: 3
});
/*************************
* Create a 绘图工具 begin
*************************/
var sketchViewModel = new SketchViewModel({
view: view,
layer: tempGraphicsLayer,
pointSymbol: {
type: "simple-marker",
style: "square",
color: "#8A2BE2",
size: "16px",
outline: {
color: [255, 255, 255],
width: 3
}
},
polylineSymbol: {
type: "simple-line",
color: "#8A2BE2",
width: "4",
style: "dash"
},
polygonSymbol: {
type: "simple-fill",
color: "rgba(138,43,226,0.8)",
style: "solid",
outline: {
color: "white",
width: 1
}
}
})
sketchViewModel.on("create-complete", function (event) {
const graphic = new Graphic({
geometry: event.geometry,
symbol: sketchViewModel.graphic.symbol
});
tempGraphicsLayer.add(graphic);
});
var drawPointButton = document.getElementById("pointButton");
drawPointButton.onclick = function () {
sketchViewModel.create("point");
setActiveButton(this);
};
var drawlineButton = document.getElementById("polylineButton");
drawlineButton.onclick = function () {
sketchViewModel.create("polyline");
setActiveButton(this);
};
var drawpolygonButton = document.getElementById("polygonButton");
drawpolygonButton.onclick = function () {
sketchViewModel.create("polygon");
setActiveButton(this);
};
var drawrectangleButton = document.getElementById("rectangleButton");
drawrectangleButton.onclick = function () {
sketchViewModel.create("rectangle");
setActiveButton(this);
};
var drawcircleButton = document.getElementById("circleButton");
drawcircleButton.onclick = function () {
sketchViewModel.create("circle");
setActiveButton(this);
};
sketchViewModel.on("create-complete", addGraphic);
function addGraphic(event) {
const graphic = new Graphic({
geometry: event.geometry,
symbol: sketchViewModel.graphic.symbol
});
tempGraphicsLayer.add(graphic);
};
document.getElementById("resetButton").onclick = function () {
tempGraphicsLayer.removeAll();
sketchViewModel.reset();
}
/*************************
* Create a 绘图工具 end
*************************/
/*************************
* Create a point graphic
*************************/
// First create a point geometry (this is the location of the Titanic)
const point = {
type: "point", // autocasts as new Point()
longitude: -49.97,
latitude: 41.73
};
// Create a symbol for drawing the point
const markerSymbol = {
type: "simple-marker", // autocasts as new SimpleMarkerSymbol()
color: [226, 119, 40],
outline: {
// autocasts as new SimpleLineSymbol()
color: [255, 255, 255],
width: 2
}
};
// Create a graphic and add the geometry and symbol to it
const pointGraphic = new Graphic({
geometry: point,
symbol: markerSymbol
});
/****************************
* Create a polyline graphic
****************************/
// First create a line geometry (this is the Keystone pipeline)
const polyline = {
type: "polyline", // autocasts as new Polyline()
paths: [[-111.3, 52.68], [-98, 49.5], [-93.94, 29.89]]
};
// Create a symbol for drawing the line
const lineSymbol = {
type: "simple-line", // autocasts as SimpleLineSymbol()
color: [226, 119, 40],
width: 4
};
// Create an object for storing attributes related to the line
const lineAtt = {
Name: "Keystone Pipeline",
Owner: "TransCanada",
Length: "3,456 km"
};
/*******************************************
* Create a new graphic and add the geometry,
* symbol, and attributes to it. You may also
* add a simple PopupTemplate to the graphic.
* This allows users to view the graphic's
* attributes when it is clicked.
******************************************/
const polylineGraphic = new Graphic({
geometry: polyline,
symbol: lineSymbol,
attributes: lineAtt,
popupTemplate: {
// autocasts as new PopupTemplate()
title: "{Name}",
content: [
{
type: "fields",
fieldInfos: [
{
fieldName: "Name"
},
{
fieldName: "Owner"
},
{
fieldName: "Length"
}
]
}
]
}
});
/***************************
* Create a polygon graphic
***************************/
// Create a polygon geometry
const polygon = {
type: "polygon", // autocasts as new Polygon()
rings: [[-64.78, 32.3], [-66.07, 18.45], [-80.21, 25.78], [-64.78, 32.3]]
};
// Create a symbol for rendering the graphic
const fillSymbol = {
type: "simple-fill", // autocasts as new SimpleFillSymbol()
color: [227, 139, 79, 0.8],
outline: {
// autocasts as new SimpleLineSymbol()
color: [255, 255, 255],
width: 1
}
};
// Add the geometry and symbol to a new graphic
const polygonGraphic = new Graphic({
geometry: polygon,
symbol: fillSymbol
});
// Add the graphics to the view's graphics layer
view.graphics.addMany([pointGraphic, polylineGraphic, polygonGraphic]);
var drawPointButton = document.getElementById("pointButton");
drawPointButton.onclick = function () {
sketchViewModel.create("point");
setActiveButton(this);
};
var drawlineButton = document.getElementById("polylineButton");
drawlineButton.onclick = function () {
sketchViewModel.create("polyline");
setActiveButton(this);
};
var drawpolygonButton = document.getElementById("polygonButton");
drawpolygonButton.onclick = function () {
sketchViewModel.create("polygon");
setActiveButton(this);
};
var drawrectangleButton = document.getElementById("rectangleButton");
drawrectangleButton.onclick = function () {
sketchViewModel.create("rectangle");
setActiveButton(this);
};
var drawcircleButton = document.getElementById("circleButton");
drawcircleButton.onclick = function () {
sketchViewModel.create("circle");
setActiveButton(this);
};
document.getElementById("resetButton").onclick = function () {
tempGraphicsLayer.removeAll();
sketchViewModel.reset();
}
});
</script>
</head>
<body>
<div id="viewDiv">
<div id="topbar">
<button class="action-button esri-icon-blank-map-pin" id="pointButton" type="button" title="Draw point"></button>
<button class="action-button esri-icon-polyline" id="polylineButton" type="button" title="Draw polyline"></button>
<button class="action-button esri-icon-polygon" id="polygonButton" type="button" title="Draw polygon"></button>
<button class="action-button esri-icon-checkbox-unchecked" id="rectangleButton" type="button"
title="Draw rectangle"></button>
<button class="action-button esri-icon-radio-unchecked" id="circleButton" type="button"
title="Draw circle"></button>
<button class="action-button esri-icon-trash" id="resetButton" type="button" title="Clear graphics"></button>
</div>
</div>
</body>
</html>
arcgis 绘图及显示图形例子
最新推荐文章于 2024-08-22 19:46:07 发布
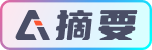