将QT项目中每个文件的每行代码自行注释一遍
#项目过程管理文件
QT += core gui
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
#所需的类库,超过4版本要加widgets
CONFIG += c++11
#支持c++11
# The following define makes your compiler emit warnings if you use
# any Qt feature that has been marked deprecated (the exact warnings
# depend on your compiler). Please consult the documentation of the
# deprecated API in order to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
#源文件
SOURCES += \
main.cpp \
widget.cpp
#头文件
HEADERS += \
widget.h
#图形化界面文件
FORMS += \
widget.ui
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; } //命名空间的声明
QT_END_NAMESPACE
//派生的自定义图形化界面,基类是QWidget
class Widget : public QWidget
{
Q_OBJECT //信号和槽操作有关
signals:
void change(); //定义了一个信号,但是没有
public slots:
void on_change_slot(); //自定义的槽函数 是一个完整的函数,既有声明也有定义
public:
Widget(QWidget *parent = nullptr); //自定义的构造函数的声明,参数是一个QWidget的指针,依附于父类组件
~Widget(); //析构函数
private:
Ui::Widget *ui; //UI指针,通过UI指针可以完成对窗口属性的设置和调整,与使用Designer工具
//的效果是一样的
};
#endif // WIDGET_H
#include "widget.h" //引入本工程下的头文件
#include "ui_widget.h" //ui文件生产的头文件
Widget::Widget(QWidget *parent) //定义了自定义类
: QWidget(parent) //调用父类的有参构造,来完成子类继承父类成员的初始化
, ui(new Ui::Widget) //给UI指针,来完成实例化空间
{
ui->setupUi(this); //调用ui中的成员函数
}
Widget::~Widget() //定义自定义的析构函数
{
delete ui; //释放UI指针的空间
}
#include "widget.h" //引入本工程的头文件
#include <QApplication> //引入应用程序的头文件
int main(int argc, char *argv[])
{
QApplication a(argc, argv); //实例化一个QApplication类的对象
Widget w; //在栈区申请一片空间
w.show(); //调用展示应用程序的函数
return a.exec(); //等待被唤醒
}
手动实现对象对象树模型
#include <iostream>
#include <list>
//命名空间的声明
using namespace std;
//自定义对象树类
class my_object
{
public:
list<my_object *> child; //子组件链表
public:
my_object(my_object *parent = nullptr) //带默认参数的有参构造,使用的时候也可以无参,无参时使用默认参数
{
if(parent != nullptr) //如果传入了参数就执行分支语句中的语句块
{
//说明创建对象的时候,给定了父组件,说明要将自己的地址放到父组件的孩子链表中
parent->child.push_back(this);
}
}
virtual ~my_object() //将父类的析构函数设置为虚函数,可以将子组件一起释放
{
for(auto p = child.begin(); p!=child.end(); p++) //循环遍历析构子组件,直至全部析构完
{
delete *p;
}
}
};
//派生类A
class A : public my_object
{
public:
A(my_object *parent = nullptr)
{
if(parent != nullptr)
{
parent->child.push_back(this);
}
cout<< "A::构造函数" <<endl;
}
virtual ~A()
{
cout<< "A::析构函数" <<endl;
}
};
//派生类B
class B : public my_object
{
public:
B(my_object *parent = nullptr)
{
if(parent != nullptr)
{
parent->child.push_back(this);
}
cout<< "B::构造函数" <<endl;
}
virtual ~B()
{
cout<< "B::析构函数" <<endl;
}
};
int main()
{
A w; //在栈区申请空间,相当于自己的MyWnd
B *btn = new B(&w);
return 0;
}
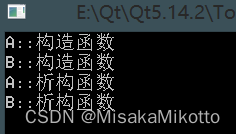