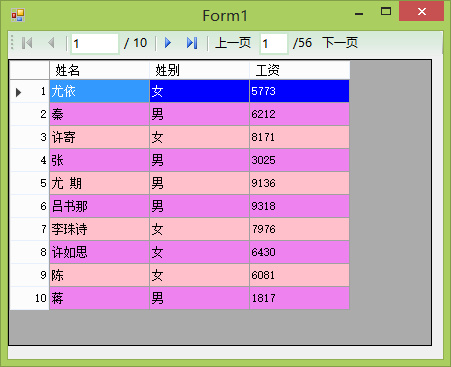
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Windows.Forms;
- namespace DataGridViewLastTest
- {
- public partial class Form1 : Form
- {
- public Form1()
- {
- InitializeComponent();
- }
- DataTable dt;
- int valTotal = 500;//总数量
- int valPerPage = 9;//每页条数
- int pageNum = 0;//总页数
- int currentPage = 1;//当前页
- int valCurrent = 0;//当前条数
- int valStartIndex = 0;//每页起始条数
- int valEndIndex = 0;//当前页终止条数
- //生成数据表
- private void GenerageData()
- {
- dt = new DataTable("ClerkSalary");
- dt.Columns.Add("姓名", Type.GetType("System.String"));
- dt.Columns.Add("姓别", Type.GetType("System.String"));
- dt.Columns.Add("工资", Type.GetType("System.Int32"));
- string familyName = "赵钱孙李周吴郑冯陈褚卫蒋沈韩杨朱秦尤许何吕施张";
- string lastName=@"那日丹桂梢头缀黄花绽竹篱抛情引墨顿成诗情也依依爱也依依
- 天涯两相望月人独伤悲红笺小字寄相思情也难追爱也难追
- 聊期花共月闲看绿着珠滴水涌泉歌诗儒词也狂书笔也狂书
- 瑶琴闲置久知音日渐疏往事思来总不如词也成枯笔也成枯";
- string gender = "男女";
- Random random = new Random();
- for (int i = 0; i < valTotal; i++)
- {
- //新增行方法一:
- //DataRow dr = dt.NewRow();
- //dr[0] = familyName[random.Next(0, familyName.Length)].ToString()
- // + lastName[random.Next(0, lastName.Length)].ToString()
- // + lastName[random.Next(0, lastName.Length)].ToString();
- //dr[1] = gender[random.Next(0, gender.Length)].ToString();
- //dr[2] = random.Next(1800, 10000);
- //dt.Rows.Add(dr);
- //新增行方法二:
- string name = familyName[random.Next(0, familyName.Length)].ToString()
- +lastName[random.Next(0, lastName.Length)].ToString()
- +lastName[random.Next(0, lastName.Length)].ToString();
- string Gender=gender[random.Next(0,gender.Length)].ToString();
- int salary = random.Next(1800,10000);
- dt.Rows.Add(new object[] { name,Gender,salary});
- }
- }
- //加载当前页的数据
- private void LoadData()
- {
- DataTable dtTemp = dt.Clone();
- //只有一页
- if (currentPage == pageNum) valEndIndex = valTotal - 1;
- //不止一页
- else valEndIndex = currentPage * valPerPage;
- valStartIndex = valCurrent;
- //更新显示
- txtCurrentPage.Text = currentPage.ToString();
- lblTotalPage.Text = "/" + Convert.ToString(pageNum);
- //从数据表中读取当前数据
- for (int i = valStartIndex; i <= valEndIndex; i++)
- {
- dtTemp.ImportRow(dt.Rows[i]);
- valCurrent++;
- }
- //通过bindingSource1作为桥梁绑定数据
- bindingSource1.DataSource = dtTemp;
- bindingNavigator1.BindingSource = bindingSource1;
- dataGridView1.DataSource = bindingSource1;
- //设置不同行显示背景
- dataGridView1.RowsDefaultCellStyle.BackColor = Color.Pink;
- dataGridView1.AlternatingRowsDefaultCellStyle.BackColor = Color.Violet;
- }
- private void Form1_Load(object sender, EventArgs e)
- {
- try
- {
- GenerageData();
- pageNum = (valTotal % valPerPage == 0) ? (valTotal / valPerPage) : (valTotal / valPerPage + 1);
- LoadData();
- }
- catch (Exception ex)
- {
- throw;
- }
- }
- private void dataGridView1_RowPostPaint(object sender, DataGridViewRowPostPaintEventArgs e)
- {
- //绘制行号
- Rectangle rect = new Rectangle(e.RowBounds.Location.X,e.RowBounds.Location.Y,dataGridView1.RowHeadersWidth,e.RowBounds.Height);
- TextRenderer.DrawText(e.Graphics,(e.RowIndex+1+valStartIndex).ToString(),dataGridView1.RowHeadersDefaultCellStyle.Font,rect,dataGridView1.RowHeadersDefaultCellStyle.ForeColor,TextFormatFlags.VerticalCenter|TextFormatFlags.Right);
- //选中时显示不同的背景
- if (e.RowIndex >= dataGridView1.Rows.Count - 1) return;
- var row =dataGridView1.Rows[e.RowIndex];
- Color oldForeColor = new Color();
- Color oldBackColor = new Color();
- if (row == dataGridView1.CurrentRow)
- {
- if (row.DefaultCellStyle.ForeColor != Color.White)
- {
- oldForeColor = row.DefaultCellStyle.ForeColor;
- row.DefaultCellStyle.ForeColor = Color.White;
- }
- if (row.DefaultCellStyle.BackColor != Color.Blue)
- {
- oldBackColor = row.DefaultCellStyle.BackColor;
- row.DefaultCellStyle.BackColor = Color.Blue;
- }
- }
- else
- {
- row.DefaultCellStyle.ForeColor = oldForeColor;
- row.DefaultCellStyle.BackColor = oldBackColor;
- }
- }
- private void bindingNavigator1_ItemClicked(object sender, ToolStripItemClickedEventArgs e)
- {
- if (e.ClickedItem.Text == "上一页")
- {
- currentPage--;
- if (currentPage <= 0)
- {
- MessageBox.Show("已经是第一页");
- currentPage++;
- return;
- }
- else
- {
- valCurrent=valPerPage*(currentPage-1);
- }
- LoadData();
- }
- if (e.ClickedItem.Text =="下一页")
- {
- currentPage++;
- if (currentPage > pageNum)
- {
- currentPage--;
- MessageBox.Show("已经是最后一页");
- return;
- }
- else
- {
- valCurrent = valPerPage * (currentPage - 1);
- }
- LoadData();
- }
- }
- }
- }