1 概念
框架:大师写好的半成品
spring框架:不是功能性框架(不属于某一层) 用于整合其他功能框架的中间层框架
核心:ioc和aop
ioc:Inversion of Control,控制反转
把程序中需要对象的创建 初始化 维护 分配 销毁等交给spring容器来管理
程序中需要的所有对象 不需要new 而是从spring容器中拿
di: Dependency Injection,依赖注入
在运行时 把需要的对象动态的注入到程序中
2 案例1
2.1 导入jar包
logging-1.1.1.jar:日志jar
log4j-1.2.15.jar:日志jar
asm-3.0.1.RELEASE-A.jar:字节码文件解析jar
RELEASE-A.jar:javabean实体类依赖的jar
context-3.0.1.RELEASE-A.jar:spring功能控制jar---验证/jmail
core-3.0.1.RELEASE-A.jar:springioc核心jar
expression-3.0.1.RELEASE-A.jar:spring表达式依赖的jar
2.2 创建核心配置文件
spring_config.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
</beans>xxxxxxxxxx spring_config.xml<?xml version="1.0" encoding="UTF-8"?><beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> </beans>xml
2.3 创建实体类
1 实现序列化
2 所有属性私有化:提供getset方法
3 提供无参数的构造方法
4 属性包装类类型
public class Student implements Serializable{
private Integer sid;
private String sname;
private Integer sage;
private Boolean sdy;
private Float score;
...
}
2.4 在核心配置文件中通过bean标签来创建对象
<bean id="stu1" class="com.zhiyou100.test01.Student">
<property name="sid" value="1001"/>
<property name="sname" value="韩梅梅"/>
<property name="sage" value="19"/>
<property name="sdy" value="true"/>
<property name="score" value="11.6"/>
</bean>
<bean id="stu2" class="com.zhiyou100.test01.Student">
<constructor-arg name="sid" value="1002"/>
<constructor-arg name="sname" value="韩寒"/>
<constructor-arg name="sage" value="19"/>
<constructor-arg name="sdy" value="false"/>
<constructor-arg name="score" value="33.5"/>
</bean>
<bean id="stu3" class="com.zhiyou100.test01.Student">
<constructor-arg index="0" value="1003"/>
<constructor-arg index="1" value="韩非子"/>
<constructor-arg index="2" value="29"/>
<constructor-arg index="3" value="false"/>
<constructor-arg index="4" value="35.5"/>
</bean>
2.5 测试类
ClassPathXmlApplicationContext context=
new ClassPathXmlApplicationContext("com/zhiyou100/test01/spring_config.xml");
Student s1=(Student)context.getBean("stu1");
System.out.println(s1);
Student s2=(Student)context.getBean("stu2");
System.out.println(s2);
Student s3=(Student)context.getBean("stu3");
System.out.println(s3);
context.close();
2.6 结果
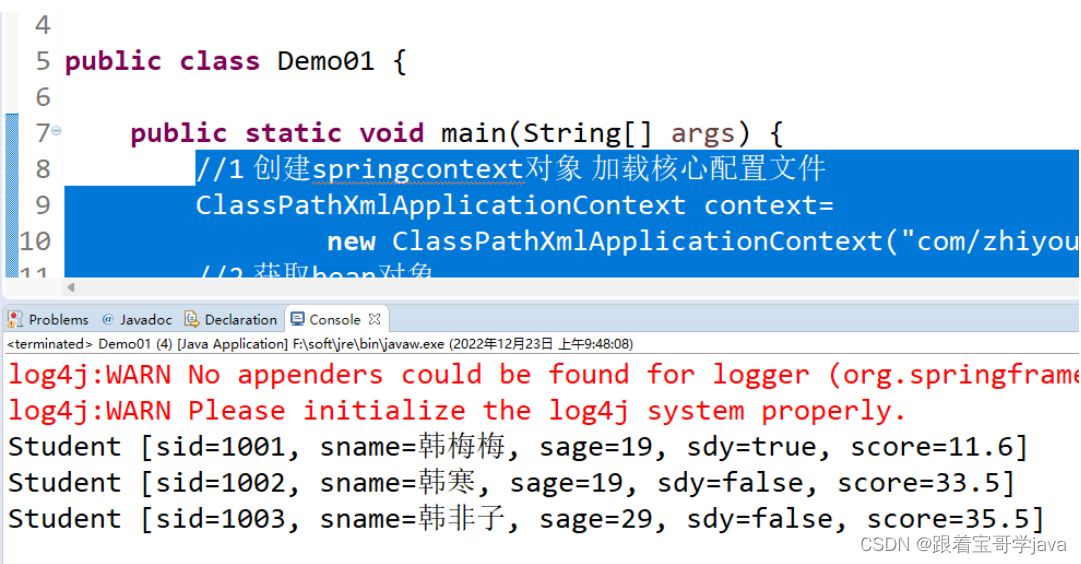
3 注意事项
1:默认情况下:项目一启动,springcontext上下文对象就加载核心配置文件 立刻为每个bean标签创建对象
2:默认情况下:一个bean标签只对应一个对象:每个bean都是一个单例对象
3:property标签给属性赋值:必须提供无参数的构造方法:否则报错:NoSuchMethodException
对象创建后 提供调用set方法给属性赋值
4:constructor-arg标签给属性赋值:调用对应的构造方法 通过构造方法的参数列表给属性赋值
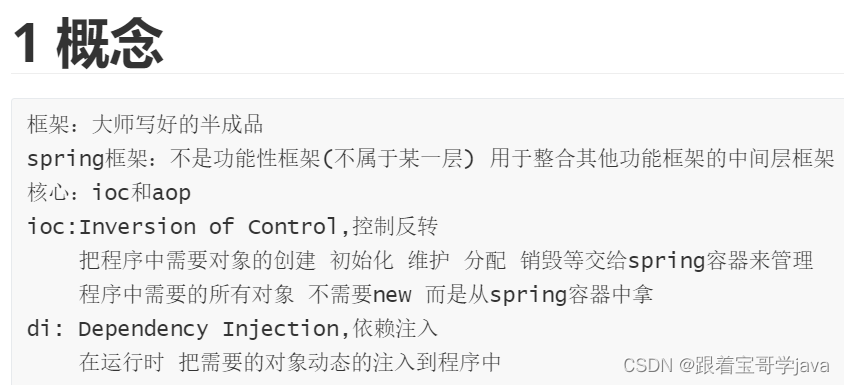