C++:专题八实验(继承)
Tips:如果你有看不懂的地方,欢迎<私信我> 或者<百度搜索c++菜鸟教程>,提问是解决问题的最好办法!
实验目的:
- 学习类的继承概念和实现方法,掌握基本的继承语法,理解父类与子类之间的关系。
- 学习派生类对基类不同权限的成员的访问权限,掌握公有派生、保护派生和私有派生三种继承方式对基类成员在派生类中访问权限的影响。
- 理解并掌握子类向父类的向上转型。
- 学习多继承的基本方法和访问控制。
实验内容:
- 定义一个表示盒子的类Box,盒子的底面为正方形,宽度为width,高度为height。定义一个Box的子类ColoredBox,在Box基础上增加了颜色属性。分别定义一个Box对象和一个ColoredBox对象,并访问输出两个对象的所有属性。
- 在实验一的基础上,在两个类的构造函数中均添加提示语句,其中Box的构造函数中,对height和width设置费0的初始值,在新建对象后输出属性,判断Box和ColoredBox在逻辑上的关系。
- 编写一个程序,设计一个汽车类Vehicle,包好的数据成员有:车轮个数wheels和车重weight。小车类Car是它的派生类,其中包含的新属性为载人数passenger。卡车类Truck也是Vehicle的派生类,包含的新属性为载人数passenger和载重量payload。要求实现3个类的相关函数(包括构造函数和析构函数,构造函数和析构函数中要输出提示信息),并至少个建立一个对象,输出其属性。
- 对第一个实验任务中构造的两个类,各添加一个show()函数,输出其所有属性。在主函数中定义一个子类(ColoredBox)对象,调用其show()函数查看输出结果使用向上转换,转换为Box类的对象后,再次调用show()函数,比较函数运行结果。
- 定义一个Circle类,包含一个数据成员radius;定义一个Table类,包含一个数据成员height;两个类的数据成员都是私有的。定义一个RoundTable类表示圆桌,采用公有继承的方式同时继承Circle和Table类,此外包含一个新属性Color。定义一个RoundTable对象,以适当的方式输出其属性。
- 在实验五任务所有类的构造函数中,添加一条输出语句,以提示这个类的构造函数被调用,再次运行程序,观察结果思考多继承的机制。
(一)定义一个表示盒子的类Box,盒子的底面为正方形,宽度为width,高度为height。定义一个Box的子类ColoredBox,在Box基础上增加了颜色属性。分别定义一个Box对象和一个ColoredBox对象,并访问输出两个对象的所有属性。
#include <iostream>
using namespace std;
class Box
{
protected:
float width, height;
public:
void setBox(float w, float h)
{
width = w, height = h;
}
void showBox()
{
cout << "box width:" << width << " height:" << height << endl;
}
};
class ColoredBox : public Box
{
protected:
int* color;
public:
void setBox(float w, float h, int c[3])
{
width = w, height = h, color = c;
}
void showBox()
{
cout << "coloredbox width:" << width << " height:" << height << " color:" << color[0] << "," << color[1] << "," << color[2] << endl;
}
};
int main()
{
int c[3] = {255, 0, 125};
Box box;
ColoredBox coloredbox;
box.setBox(9.1f, 8.4f);
coloredbox.setBox(10.3f, 6.2f, c);
box.showBox();
coloredbox.showBox();
return 0;
}

(二)在实验一的基础上,在两个类的构造函数中均添加提示语句,其中Box的构造函数中,对height和width设置费0的初始值,在新建对象后输出属性,判断Box和ColoredBox在逻辑上的关系。
#include <iostream>
using namespace std;
class Box
{
public:
float width, height;
Box()
{
width = 0, height = 0;
cout << "a box has been created." << endl;
}
};
class ColoredBox : public Box
{
public:
int* color;
ColoredBox()
{
cout << "a coloredbox has been created." << endl;
}
};
int main()
{
Box box;
ColoredBox coloredbox;
cout << "box width:" << box.width << " height:" << box.height << endl;
cout << "coloredbox width:" << coloredbox.width << " height:" << coloredbox.height << " color:" << endl;
return 0;
}
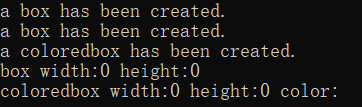
(三)编写一个程序,设计一个汽车类Vehicle,包好的数据成员有:车轮个数wheels和车重weight。小车类Car是它的派生类,其中包含的新属性为载人数passenger。卡车类Truck也是Vehicle的派生类,包含的新属性为载人数passenger和载重量payload。要求实现3个类的相关函数(包括构造函数和析构函数,构造函数和析构函数中要输出提示信息),并至少个建立一个对象,输出其属性。
#include <iostream>
using namespace std;
class Vehicle
{
public:
int wheels;
float weight;
Vehicle(int Wheels = 4, float Weight = 10)
{
wheels = Wheels, weight = Weight;
cout << "a Vehicle has been created." << endl;
}
~Vehicle()
{
cout << "a Vehicle has been destoryed." << endl;
cout << endl;
}
void show()
{
cout << "the Vehicle:" << " wheels:" << wheels << " weight:" << weight << endl;
}
};
class Car: public Vehicle
{
public:
int passenger;
Car(int Wheels = 4, float Weight = 10, int Passenger = 0)
{
wheels = Wheels, weight = Weight, passenger = Passenger;
cout << "a Car has been created." << endl;
}
~Car()
{
cout << "a Car has been destoryed." << endl;
}
void show()
{
cout << "the Car:" << " wheels:" << wheels << " weight:" << weight << " passengers:" << passenger << endl;
}
};
class Truck: public Vehicle
{
public:
int passenger;
float payload;
Truck(int Wheels = 4, float Weight = 10, int Passenger = 0, float Payload = 0)
{
wheels = Wheels, weight = Weight, passenger = Passenger, payload = Payload;
cout << "a Truck has been created." << endl;
}
~Truck()
{
cout << "a Truck has been destoryed." << endl;
}
void show()
{
cout << "the Car:" << " wheels:" << wheels << " weight:" << weight << " passengers:" << passenger << " payload:" << payload << endl;
}
};
int main()
{
Vehicle vehicle(4, 12);
vehicle.show();
cout << endl;
Car car(6, 24, 8);
car.show();
cout << endl;
Truck truck(8, 50, 4, 80);
truck.show();
cout << endl;
}
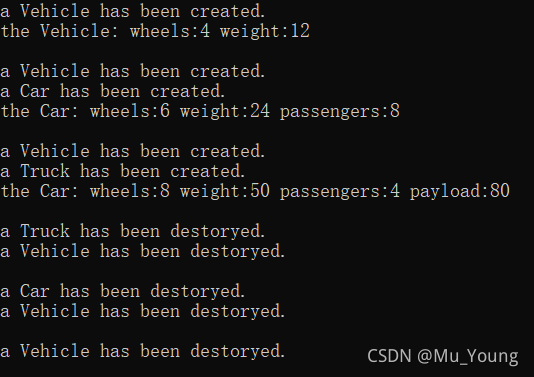
(四)对第一个实验任务中构造的两个类,各添加一个show()函数,输出其所有属性。在主函数中定义一个子类(ColoredBox)对象,调用其show()函数查看输出结果使用向上转换,转换为Box类的对象后,再次调用show()函数,比较函数运行结果。。
#include <iostream>
using namespace std;
class Box
{
protected:
float width, height;
public:
void setBox(float w, float h)
{
width = w, height = h;
}
void show()
{
cout << "box width:" << width << " height:" << height << endl;
}
};
class ColoredBox : public Box
{
protected:
int* color;
public:
void setBox(float w, float h, int c[3])
{
width = w, height = h, color = c;
}
void show()
{
cout << "coloredbox width:" << width << " height:" << height << " color:" << color[0] << "," << color[1] << "," << color[2] << endl;
}
};
int main()
{
int c[3] = {255, 0, 125};
ColoredBox coloredbox;
coloredbox.setBox(10.3f, 6.2f, c);
coloredbox.show();
Box(coloredbox).show();
return 0;
}

(五)定义一个Circle类,包含一个数据成员radius;定义一个Table类,包含一个数据成员height;两个类的数据成员都是私有的。定义一个RoundTable类表示圆桌,采用公有继承的方式同时继承Circle和Table类,此外包含一个新属性Color。定义一个RoundTable对象,以适当的方式输出其属性。。
#include <iostream>
using namespace std;
class Circle
{
private:
float radius;
public :
void setradius(float r)
{
radius = r;
}
float getradius()
{
return radius;
}
};
class Table
{
private:
float height;
public :
void setheight(float h)
{
height = h;
}
float getheight()
{
return height;
}
};
class RoundTable: public Circle, public Table
{
private:
int *color;
public:
RoundTable(int c[3] = {0})
{
color = c;
}
int *getcolor()
{
return color;
}
};
int main()
{
int c[3] = {255, 125, 100};
RoundTable roundtable(c);
roundtable.setradius(3.1415);
roundtable.setheight(10);
cout<<"roundtable:"<<" radius:"<<roundtable.getradius()<<\
" height:"<<roundtable.getheight()<<
" color:"<<*(roundtable.getcolor())<<","<<\
*(roundtable.getcolor()+1)<<","<<*(roundtable.getcolor()+2)<<endl;
}

(六)在实验五任务所有类的构造函数中,添加一条输出语句,以提示这个类的构造函数被调用,再次运行程序,观察结果思考多继承的机制。
#include <iostream>
using namespace std;
class Circle
{
private:
float radius;
public :
Circle()
{
cout << "The constructor of class \"Circle\" is called." << endl;
}
void setradius(float r)
{
radius = r;
}
float getradius()
{
return radius;
}
};
class Table
{
private:
float height;
public :
Table()
{
cout << "The constructor of class \"Table\" is called." << endl;
}
void setheight(float h)
{
height = h;
}
float getheight()
{
return height;
}
};
class RoundTable: public Circle, public Table
{
private:
int *color;
public:
RoundTable(int c[3] = {0})
{
color = c;
cout << "The constructor of class \"RoundTable\" is called." << endl;
}
int *getcolor()
{
return color;
}
};
int main()
{
int c[3] = {255, 125, 100};
RoundTable roundtable(c);
roundtable.setradius(3.1415);
roundtable.setheight(10);
cout << "roundtable:" << " radius:" << roundtable.getradius() << \
" height:" << roundtable.getheight() <<
" color:" << *(roundtable.getcolor()) << "," << \
*(roundtable.getcolor() + 1) << "," << *(roundtable.getcolor() + 2) << endl;
}
