10.8. Serializers
From the framework perspective, the data stored in Redis is only
bytes. While Redis itself supports various types, for the most part,
these refer to the way the data is stored rather than what it
represents. It is up to the user to decide whether the information
gets translated into strings or any other objects.
In Spring Data, the conversion between the user (custom) types and raw
data (and vice-versa) is handled Redis in the
org.springframework.data.redis.serializer package.
This package contains two types of serializers that, as the name
implies, take care of the serialization process:
- Two-way serializers based on RedisSerializer.
- Element readers and writers that use RedisElementReader and RedisElementWriter.
The main difference between these variants is that RedisSerializer
primarily serializes to byte[] while readers and writers use
ByteBuffer
Multiple implementations are available (including two that have been already mentioned in this documentation):
JdkSerializationRedisSerializer, which is used by default for RedisCache and RedisTemplate.
the StringRedisSerializer.
However one can use OxmSerializer for Object/XML mapping through
Spring OXM support or Jackson2JsonRedisSerializer or
GenericJackson2JsonRedisSerializer for storing data in JSON format.
译文
从框架的角度来看,Redis中存储的数据只是字节。
虽然Redis本身支持多种类型,但在大多数情况下,这些类型指的是数据存储的方式,而不是它所代表的内容。
由用户决定是将信息转换为字符串还是任何其他对象。
在Spring Data中,用户(自定义)类型和原始数据(反之亦然)之间的转换是在org.springframework.data.redis.serializer包中处理的。
这个包包含两种类型的序列化器,顾名思义,它们负责序列化过程:
基于RedisSerializer的双向序列化器。
使用RedisElementReader和RedisElementWriter的元素读取器和写入器。
这些变体之间的主要区别是RedisSerializer主要序列化为byte[],而读取器和写入器使用ByteBuffer。
有多种实现(包括本文档中已经提到的两种):
JdkSerializationRedisSerializer,默认情况下用于RedisCache和RedisTemplate。
StringRedisSerializer。
然而,我们可以使用OxmSerializer通过Spring OXM支持进行对象/XML映射,或者使用Jackson2JsonRedisSerializer或GenericJackson2JsonRedisSerializer以JSON格式存储数据。
实践
package com.cjh.example;
import com.alibaba.fastjson.support.spring.GenericFastJsonRedisSerializer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.GenericToStringSerializer;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.JdkSerializationRedisSerializer;
import org.springframework.data.redis.serializer.RedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
/**
* 序列化配置
*
* @author cjh
* @date 2021/7/13
*/
@Configuration
public class Config {
/**
* 自定义StringRedisTemplate的序列化
* @param redisConnectionFactory 注入springboot的 {@link RedisConnectionFactory}
* @return 配置后的 {@link StringRedisTemplate},会覆盖springboot的自动装配
*/
@Bean
public StringRedisTemplate stringRedisTemplate(RedisConnectionFactory redisConnectionFactory) {
StringRedisTemplate stringRedisTemplate = new StringRedisTemplate(redisConnectionFactory);
//setKeySerializer
stringRedisTemplate.setKeySerializer(new StringRedisSerializer());
// stringRedisTemplate.setKeySerializer(RedisSerializer.string());
//setValueSerializer
stringRedisTemplate.setValueSerializer(new GenericToStringSerializer<>(Object.class));
// stringRedisTemplate.setValueSerializer(new GenericFastJsonRedisSerializer());
// stringRedisTemplate.setValueSerializer(new Jackson2JsonRedisSerializer<>(Object.class));
// stringRedisTemplate.setValueSerializer(new JdkSerializationRedisSerializer());
return stringRedisTemplate;
}
/**
* 自定义RedisTemplate的序列化,或指定泛型
* @param springbootRedisTemplate 注入springboot的 {@link RedisTemplate}
* @return 配置后的 {@link RedisTemplate},会覆盖springboot的自动装配
*/
@Bean
public RedisTemplate redisTemplate(RedisTemplate springbootRedisTemplate) {
RedisTemplate redisTemplate = springbootRedisTemplate;
//默认
// redisTemplate.setKeySerializer(RedisSerializer.string());
//setValueSerializer
// redisTemplate.setValueSerializer(new GenericFastJsonRedisSerializer());
redisTemplate.setValueSerializer(new Jackson2JsonRedisSerializer<>(Object.class));
// redisTemplate.setValueSerializer(new GenericToStringSerializer<>(Object.class));
// redisTemplate.setValueSerializer(new JdkSerializationRedisSerializer());
return redisTemplate;
}
}
GenericFastJsonRedisSerializer序列化
GenericToStringSerializer序列化
源码
StringRedisTemplate 序列化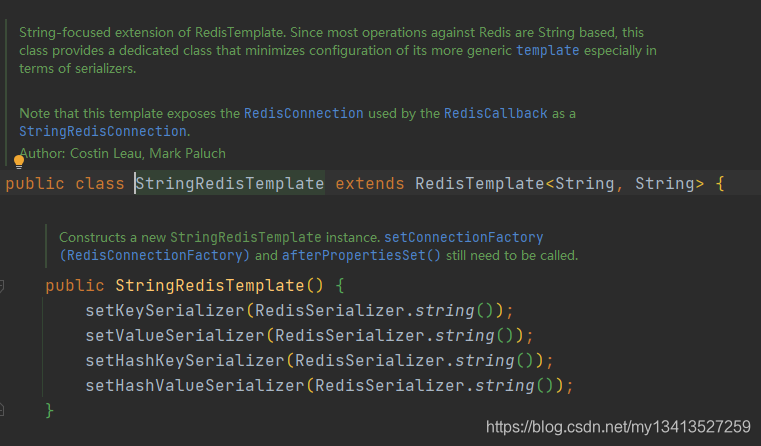
RedisTemplate 序列化