p716 回顾list集合需要掌握什么
ArrayList
package com.bjpowernode.day24.review;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ArrayListTest01 {
public static void main(String[] args) {
List list = new ArrayList();//不区分集合中的元素
list.add(12);;
list.add("zhangsan");;
list.add(33.144);;
list.add(true);;
System.out.println(list.get(2));
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i));
}
//使用泛型约束元素类型
List<String> list2 = new ArrayList<>();
list2.add("zhangsab");
list2.add("wwawngwqu");
list2.add("lisi");
list2.add("maliu");
System.out.println(list.get(2));
//使用下标遍历
// for (int i = 0; i < list2.size(); i++) {
// System.out.println(list2.get(i));
//
// }
//使用迭代器方式遍历,通用的,所有的collection都用能用
// Iterator<String> it = list2.iterator();
//
// while (it.hasNext()){
// System.out.println(it.next());
// }
//while循环修改为for循环
for(Iterator<String> it2 = list2.iterator(); it2.hasNext();){
System.out.println("=====>"+it2.next());
}
//使用增强for循环遍历
for (String s : list2){
System.out.println(s);
}
}
}
LinkedList
package com.bjpowernode.day24.review;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
public class LinkedListTest01 {
public static void main(String[] args) {
//使用泛型约束元素类型
LinkedList<String> list = new LinkedList();//不区分集合中的元素
list.add("zhangsab");
list.add("wwawngwqu");
list.add("lisi");
list.add("maliu");
System.out.println(list.get(2));
//使用下标遍历
// for (int i = 0; i < list2.size(); i++) {
// System.out.println(list2.get(i));
//
// }
//使用迭代器方式遍历,通用的,所有的collection都用能用
// Iterator<String> it = list2.iterator();
//
// while (it.hasNext()){
// System.out.println(it.next());
// }
//while循环修改为for循环
for(Iterator<String> it2 = list.iterator(); it2.hasNext();){
System.out.println("=====>"+it2.next());
}
//使用增强for循环遍历
for (String s : list){
System.out.println(s);
}
}
}
p717 回顾HashSet集合需要掌握什么
package com.bjpowernode.day24.review;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Objects;
public class HashSetTest {
public static void main(String[] args) {
HashSet<String> set = new HashSet<>();
set.add("king");
set.add("hello");
set.add("kitty");
set.add("pop");
//set中的元素没有下标,所以不能单独取出
//遍历迭代器
// Iterator<String> it = set.iterator();
// while(it.hasNext()){
// System.out.println(it.next());
//
// }
//foreach方法
for(String s : set){
System.out.println(s);
}
//无序不可重复、测试
System.out.println(set.size());
set.add("king");
set.add("king");
set.add("king");
System.out.println(set.size());
//创建Set集合,存储Student
HashSet<Student> students = new HashSet<>();
students.add(new Student(14,"zhangsan"));
students.add(new Student(15,"lisi"));
students.add(new Student(12,"wangwu"));
students.add(new Student(78,"maliu"));
students.add(new Student(78,"maliu"));
System.out.println(students.size());
Iterator<Student> it = students.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
}
}
class Student{
int no ;
String name ;
public Student(int no, String name) {
this.no = no;
this.name = name;
}
@Override
public String toString() {
return "Student{" +
"no=" + no +
", name=" + name +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Student)) return false;
Student student = (Student) o;
return no == student.no && name == student.name;
}
@Override
public int hashCode() {
return Objects.hash(no, name);
}
}
p718 回顾TreeSet集合需要掌握什么
集合之间的相互转换,可以使用集合的构造方法,直接使用将待转换集合作为参数传进去
Map转换为keySet方法。
package com.bjpowernode.day24.Collection;
import com.sun.source.tree.Tree;
import java.util.Comparator;
import java.util.Iterator;
import java.util.TreeSet;
public class TreeSetTest {
public static void main(String[] args) {
//集合的创建<Integer>
//TreeSet<Integer> ts = new TreeSet<>();
/**
* 重写比较规则,使其按照从大到小排序,传入比较器,使用匿名内部类.
* 重写方法可以实现降序排序
*/
TreeSet<Integer> ts = new TreeSet<>(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2 -o1;//自动拆箱,Integer类型自动拆成int数字
}
});
//添加元素
ts.add(1);
ts.add(1);
ts.add(2);
ts.add(3);
ts.add(178);
ts.add(35);
ts.add(35);
ts.add(67);
System.out.println(ts.size());//size为6.重复的不算
//遍历,使用迭代器遍历
// Iterator<Integer> it = ts.iterator();
// while(it.hasNext()){
// System.out.println(it.next());
// }
//增强for循环遍历
for(Integer i : ts){
System.out.println(i);
}
//集合的创建<String>
TreeSet<String> ts2 = new TreeSet<>();
ts2.add("mazhongjie");
ts2.add("mazhongjie");
ts2.add("hajinwei");
ts2.add("heshuai");
ts2.add("mazhongjie");
ts2.add("fanhengrui");
// //使用迭代器遍历
// Iterator<String> it2 = ts2.iterator();
//
// while(it2.hasNext()){
// String s = it2.next();
// System.out.println(s);
// }
//使用foreach遍历
for (String s : ts2){
System.out.println(s);
}
TreeSet<A> atree = new TreeSet<>();
atree.add(new A(100));
atree.add(new A(34));
atree.add(new A(45));
atree.add(new A(565));
atree.add(new A(2334));
//遍历,查看是否能给自定义类型自动排序
//方法1使用迭代器遍历
Iterator<A> it = atree.iterator();
while(it.hasNext()){
A a = it.next();
System.out.println(a);
}
}
}
class A implements Comparable<A>{
int i;
public A(int i) {
this.i = i;
}
@Override
public String toString() {
return "A{" +
"i=" + i +
'}';
}
@Override
public int compareTo(A o) {
return this.i - o.i;
}
}
package com.bjpowernode.day24.Collection;
import com.sun.source.tree.Tree;
import java.util.Comparator;
import java.util.Iterator;
import java.util.TreeSet;
public class TreeSetTest {
public static void main(String[] args) {
//集合的创建<Integer>
//TreeSet<Integer> ts = new TreeSet<>();
/**
* 重写比较规则,使其按照从大到小排序,传入比较器,使用匿名内部类.
* 重写方法可以实现降序排序
*/
TreeSet<Integer> ts = new TreeSet<>(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2 -o1;//自动拆箱,Integer类型自动拆成int数字
}
});
//添加元素
ts.add(1);
ts.add(1);
ts.add(2);
ts.add(3);
ts.add(178);
ts.add(35);
ts.add(35);
ts.add(67);
//System.out.println(ts.size());//size为6.重复的不算
//遍历,使用迭代器遍历
// Iterator<Integer> it = ts.iterator();
// while(it.hasNext()){
// System.out.println(it.next());
// }
//增强for循环遍历
// for(Integer i : ts){
// System.out.println(i);
// }
//集合的创建<String>
TreeSet<String> ts2 = new TreeSet<>();
ts2.add("mazhongjie");
ts2.add("mazhongjie");
ts2.add("hajinwei");
ts2.add("heshuai");
ts2.add("mazhongjie");
ts2.add("fanhengrui");
// //使用迭代器遍历
// Iterator<String> it2 = ts2.iterator();
//
// while(it2.hasNext()){
// String s = it2.next();
// System.out.println(s);
// }
//使用foreach遍历
// for (String s : ts2){
// System.out.println(s);
// }
//
TreeSet<A> atree = new TreeSet<>();
atree.add(new A(100));
atree.add(new A(34));
atree.add(new A(45));
atree.add(new A(565));
atree.add(new A(2334));
//遍历,查看是否能给自定义类型自动排序
//方法1使用迭代器遍历
// Iterator<A> it = atree.iterator();
//
// while(it.hasNext()){
// A a = it.next();
// System.out.println(a);
// }
///
//一定要记得把自己定义的比较器类传进去
TreeSet<B> btree = new TreeSet<>(new BComparator());
btree.add(new B(233));
btree.add(new B(34));
btree.add(new B(4565));
btree.add(new B(343));
btree.add(new B(3223));
//迭代器遍历
//Iterator<B> it3 = btree.iterator();
// while(it3.hasNext()){
// B b = it3.next();
// System.out.println(b);
//
// }
///
System.out.println("=========================================");
//使用匿名内部类方式
TreeSet<B> btree2 = new TreeSet<>(new Comparator<B>() {
@Override
public int compare(B o1, B o2) {
return o1.i - o2.i;
}
});
btree2.add(new B(34));
btree2.add(new B(34));
btree2.add(new B(456));
btree2.add(new B(456));
btree2.add(new B(333));
//迭代器遍历
Iterator<B> it4 = btree2.iterator();
while(it4.hasNext()){
B b = it4.next();
System.out.println(b);
}
///
///
}
}
/**
* 第一种方式:实现Comparable接口
*/
class A implements Comparable<A>{
int i;
public A(int i) {
this.i = i;
}
@Override
public String toString() {
return "A{" +
"i=" + i +
'}';
}
@Override
public int compareTo(A o) {
return this.i - o.i;
}
}
/**
* 第二种方式:单独写一个类,下面写一个比较器类
*/
class B{
int i;
public B(int i) {
this.i = i;
}
@Override
public String toString() {
return "B{" +
"i=" + i +
'}';
}
}
class BComparator implements Comparator<B>{
@Override
public int compare(B o1, B o2) {
return o1.i - o2.i;
}
}
p719 回顾HashMap集合需要掌握什么
Properties
package com.bjpowernode.day24.review;
import java.util.Properties;
public class PropertiesTest {
public static void main(String[] args) {
Properties pro = new Properties();
pro.setProperty("username","zhangsan");
pro.setProperty("password","123456");
//取
String username = pro.getProperty("username");
String passwprd = pro.getProperty("password");
System.out.println(username);
System.out.println(passwprd);
}
}
集合中只需要掌握review里面的5个例子就行。别的正常学习就可以,抓住重点。
——————————————————————————————集合完结
p720 I/O流概述
p721 I/O流分类
p722 I/O流该怎么学习
p723 流的四大家族
p724 流的close()和flush()方法
所有的输出流都实现了java.io.Flushable接口,都是可刷新的
p725 需要掌握哪些流
p726 FileInputStream初步
p727 FileInputStream循环读
package com.bjpowernode.java.io;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileInputStreamTest02 {
public static void main(String[] args) {
//文件路径:C:\Users\ZhongjieMa\Desktop
//IDEA会自动变成两个斜杠,因为一个斜杠表示转义
//其实也可以写成这个路径:C:/Users/ZhongjieMa/Desktop【单个左斜杠】
//所有的流使用之后必须要关闭,在finally里面关闭
FileInputStream fl = null;
try {
fl = new FileInputStream("G:/Study/JAVA/java/powerNode/javase/Chapter23_IOStream/temp.txt");
// while(true){
// int readData = fl.read();
// if (readData == -1){
// break;
// }
// System.out.println(readData);
// }
//改造while循环
int readData = 0;
while((readData = fl.read() )!= -1){
System.out.println(readData);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
//在finally里面关闭流,但是一定要确保流不为空
if (fl != null){
try {
fl.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
p728 IDEA中的当前路径
p729 往byte数组中读
package com.bjpowernode.java.io;
/**
* 一次读取多个字节,读取到byte[]数组中
*/
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileInputStreamTest03 {
public static void main(String[] args) {
FileInputStream fl = null;
try {
fl = new FileInputStream("temp.txt");
//开始读取,byte[],最多读取数组.length个字节
byte[] bytes = new byte[4];
int readCount = fl.read(bytes);
System.out.println(readCount);//4返回读到的字节数
//System.out.println(new String(bytes));
System.out.println(new String(bytes,0,readCount));
readCount = fl.read(bytes);
System.out.println(readCount);
//System.out.println(new String(bytes));
System.out.println(new String(bytes,0,readCount));
readCount = fl.read(bytes);
System.out.println(readCount);
//System.out.println(new String(bytes));
System.out.println(new String(bytes,0,readCount));
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fl != null){
try {
fl.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
p730 FileInputStream的最终版
package com.bjpowernode.java.io;
/**
* 最终版
*/
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class FileInputStreamTest04 {
public static void main(String[] args) {
FileInputStream fl = null;
try {
fl = new FileInputStream("Chapter23_IOStream\\src\\tempfiles3.java");
byte[] bytes = new byte[4];
// while (true){
// int readCount = fl.read(bytes);
// if (readCount == -1) {
// break;
// }
// //把byte数组转换为字符串,读到多少个转换多少个
// System.out.print(new String(bytes,0,readCount));
// }
int readCount = fl.read(bytes);
while((readCount = fl.read(bytes)) != -1){
System.out.println(new String(bytes,0,readCount));
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fl != null){
try {
fl.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
p731 FileInputStream的其他方法【avaiable()方法】
package com.bjpowernode.java.io;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/*
int avaiable() 获取当前可读的字节数量
*/
public class FileInputStreamTest05 {
public static void main(String[] args) {
FileInputStream fls = null;
try {
fls = new FileInputStream("temp.txt");
System.out.println("当前文件一共有多少字节:"+fls.available());
// int readByte = fls.read();
// System.out.println("剩下多少个字节没有读:"+fls.available());
byte[] bytes = new byte[6];
int readCount = fls.read(bytes);
System.out.println(new String(bytes));//abcdef
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally{
if (fls == null) {
try {
fls.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
p732 FileInputStream的其他方法【skip()方法】
package com.bjpowernode.java.io;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/*
int avaiable() 获取当前可读的字节数量
*/
public class FileInputStreamTest05 {
public static void main(String[] args) {
FileInputStream fls = null;
try {
fls = new FileInputStream("temp.txt");
System.out.println("当前文件一共有多少字节:"+fls.available());
// int readByte = fls.read();
// System.out.println("剩下多少个字节没有读:"+fls.available());
// byte[] bytes = new byte[fls.available()];
//
// int readCount = fls.read(bytes);
// System.out.println(new String(bytes));//abcdef
fls.skip(3);
System.out.println("跳过之后剩下多少个字节没有读:"+fls.available());
System.out.println(fls.read());
// byte[] bytes = new byte[fls.available()];
// int readCount = fls.read(bytes);
// System.out.println(new String(bytes));
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally{
if (fls == null) {
try {
fls.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
p733 FileOutStream的使用
package com.bjpowernode.java.io;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class FileOutputStreamTest01 {
public static void main(String[] args) throws IOException {
FileOutputStream fos = null;
try {
//没有myfile文件时,会自动新建文件
//这种方式要谨慎使用,它会将原文件全部清空后重新写入
//fos = new FileOutputStream("myfile.txt");
//fos = new FileOutputStream("Chapter23_IOStream\\src\\tempfiles3.java");
/*
想要保留原文件的内容,不去清空,而是追加在最后面,可以使用append参数
*/
fos = new FileOutputStream("Chapter23_IOStream\\src\\tempfiles3.java",true);
//开始写
//准备一个byte数组
byte[] bytes = {97,98,99,100};
//将byte数组全部写入
fos.write(bytes);//abcd
//写入数组的一部分
fos.write(bytes,0,2);//ab
//最后一定要刷新
String s = "我是一个中国人,我骄傲!!!";
//不能直接将s字符串传进去,但是可以调用getBytes方法转为byte数组
byte[] myBytes = s.getBytes();
fos = new FileOutputStream("Chapter23_IOStream\\src\\tempfiles3.java",true);
fos.write(myBytes);
fos.write(myBytes);
fos.write(myBytes);
fos.write(myBytes);
fos.flush();
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
if (fos == null) {
fos.close();
}
}
}
}
p734 文件复制
package com.bjpowernode.java.io;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
/*
使用FileInputStream和FileOutSteven完成文件的拷贝
*/
public class Copy01 {
public static void main(String[] args) {
FileInputStream fls = null;
FileOutputStream fos = null;
try {
//创建输入流对象
fls = new FileInputStream("G:\\图片摄影\\电脑壁纸头像\\fisherman-5970480.jpg");
//创建输出流对象
fos = new FileOutputStream("C:\\Users\\ZhongjieMa\\Desktop\\copy.jpg");
/*
最核心的部分就是一边读一边写
*/
byte[] bytes = new byte[1024 * 1024];//创建一个1024*1024的数组,一次最多拷贝1M
int readCount = 0;
while((readCount = fls.read(bytes)) != -1){
fos.write(bytes,0,readCount);
}
//输出流一定要刷新
fos.flush();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
//fos和fls要分开try、catch
//一起try的话,其中一个出问题就会影响另一个流的关闭
if (fos == null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fls == null) {
try {
fls.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}q
}
p735 FileReader的使用
package com.bjpowernode.java.io;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class FileReaderTest {
public static void main(String[] args) throws IOException {
FileReader reader = null;
try {
//创建文件字符输入流
reader = new FileReader("temp.txt");
//开始读
char[] chars = new char[4];//一次读取4个字符
reader.read(chars);//按照字符的方式进行读取
//对字符数组进行遍历
for (int i = 0; i < chars.length; i++) {
System.out.println(chars[i]);
}
//进行字符串的读
// int readCount = 0;
// while((readCount = reader.read(chars)) != -1){
//
// System.out.print(new String(chars,0,readCount));
// }
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally {
if (reader == null) {
reader.close();
}
}
}
}
p736 FileWriter的使用
package com.bjpowernode.java.io;
/*
写入文件,创建文件
*/
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterTest {
public static void main(String[] args) {
FileWriter out = null;
try {
//创建文件字符输出流对象
out = new FileWriter("tempfile.txt",true);//true表示不清空,在原文件后面追加
//开始写
char[] chars = {'我','是','中','国','人'};
out.write(chars);
out.write(chars,2,3);//中国人
out.write("我是一名java软件工程师!");
out.write('\n');
out.write("hello world");
//进行刷新
out.flush();
} catch (IOException e) {
e.printStackTrace();
}finally {
if (out == null) {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
需要注意的是FileReader和FileWriter只能读普通文本
FileInputStream和FileOutputStream可以读取任何数据
p737 FileReader和FileWriter进行拷贝
package com.bjpowernode.java.io;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
/*
使用FileReader和FileWriter只能拷贝普通文本文档
*/
public class Copy02 {
public static void main(String[] args) throws IOException {
FileReader in = null;
FileWriter out = null;
try {
//读
in = new FileReader("tempfile.txt");
//写
out = new FileWriter("C:\\Users\\ZhongjieMa\\Desktop\\copy.txt");
//一边读一边写
char[] chars = new char[1024*512];
int readCount = 0;
while((readCount = in.read(chars)) != -1){
out.write(chars,00,readCount);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}finally{
if (in != null) {
in.close();
}
if (out != null) {
out.close();
}
}
}
}
p738 带有缓冲区的字符流
package com.bjpowernode.java.io;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
/*
BufferedReader:
带有缓冲区的字符输入流
使用这个流的时候不需要自定义char数组,或者不需要自定义byte数组。自带缓冲
*/
public class BufferedReaderTest01 {
public static void main(String[] args) throws Exception {
FileReader reader = new FileReader("temp.txt");
//当一个流的构造方法需要一个流的时候,被传进来的叫做节点流
//外部负责包装的流叫做包装流,还叫做处理流
//当前的程序中,FileReader就是节点流,BufferedReader是包装流
BufferedReader br = new BufferedReader(reader);
// //读一行,返回一个字符串
//
// String line1 = br.readLine();
// System.out.println(line1);
//
// //再读一行
// String line2 = br.readLine();
// System.out.println(line2);
//循环读
String s = null;
while ((s = br.readLine() ) != null){
System.out.println(s);
}
//关闭流
//对于包装流来说,只需要关闭包装流,里面的字节流会自动关闭
br.close();
}
}
p739 节点流和包装流
package com.bjpowernode.java.io;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStreamReader;
public class BufferedReaderTest02 {
public static void main(String[] args) throws Exception {
// //字节流
// FileInputStream in = new FileInputStream("javase.iml");
//
// //使用InputStreamReader将字节流转换为字符流
// InputStreamReader reader = new InputStreamReader(in);
//
// //这个构造方法只能传一个字符流,不能传字节流,所以要将字节流转换为字符流
// //包装流,此时reader是节点流,br是包装流(处理流)
// BufferedReader br = new BufferedReader(reader);
/*
将上述套娃过程合并
*/
BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream("javase.iml")) );
String line =null;
while((line = br.readLine()) != null){
System.out.println(line);
}
}
}
p740带缓冲区的字符输出流
package com.bjpowernode.java.io;
import java.io.*;
import java.nio.Buffer;
public class BufferedWriterTest {
public static void main(String[] args) throws Exception {
//BufferedWriter out = new BufferedWriter(new FileWriter("javase.iml"));
BufferedWriter out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("myfile.txt",true)));
out.write("hello world");
out.write("\n");
out.write("hello kitty");
out.flush();
out.close();
}
}
p741 数据流
数据专属流【DataOutputStream写入】
package com.bjpowernode.java.io;
import java.io.DataOutputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class DataOutputStreamTest {
public static void main(String[] args) throws Exception {
DataOutputStream dos = new DataOutputStream(new FileOutputStream("data"));
//写数据
byte b = 100;
short s = 200;
int i = 300;
long l = 4000l;
float f = 3.0f;
double d = 3.14;
boolean sex =false;
char c = 'a';
dos.writeByte(b);
dos.writeShort(s);
dos.writeInt(i);
dos.writeLong(l);
dos.writeFloat(f);
dos.writeDouble(d);
dos.writeBoolean(sex);
dos.writeChar(c);
//刷新
dos.flush();
//关闭
dos.close();
}
}
数据专属流【DataInputStream读取】
package com.bjpowernode.java.io;
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class DataInputStreamTest {
public static void main(String[] args) throws Exception {
DataInputStream dis = new DataInputStream(new FileInputStream("data"));
//开始读
byte b = dis.readByte();
short s = dis.readShort();
int i = dis.readInt();
long l = dis.readLong();
float f = dis.readFloat();
double d = dis.readDouble();
boolean sex = dis.readBoolean();
char c = dis.readChar();
//输出
System.out.println(b);
System.out.println(s);
System.out.println(i+1000);
System.out.println(l);
System.out.println(f);
System.out.println(d);
System.out.println(sex);
System.out.println(c);
dis.close();
}
}
p741 标准输出流
printStream
p742 标准输出流【记录日志】
工具类
package com.bjpowernode.java.io;
import javax.xml.crypto.Data;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Logger {
public static void log(String msg){
PrintStream out = null;
try {
out = new PrintStream(new FileOutputStream("log.txt",true));
System.setOut(out);//改变输出的位置到新建文件log.txt中
//获取系统当前时间
Date time = new Date();
//定义时间显示格式
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss SSS");
String strTime = sdf.format(time);
System.out.println(strTime+":"+msg);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
测试
package com.bjpowernode.java.io;
public class LoggerTest {
public static void main(String[] args) {
//测试工具类
Logger.log("调用了System类的gc()方法,建议启动垃圾回收");
Logger.log("调用了UserService的doSome()方法");
Logger.log("用户正在尝试进行登录,验证失败");
Logger.log("我非常喜欢这个记录日志的工具");
}
}
p743 File类的理解以及常用方法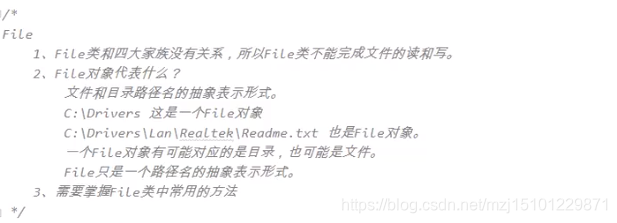
package com.bjpowernode.java.io;
import java.io.File;
import java.io.IOException;
public class FileTest01 {
public static void main(String[] args) throws Exception {
//创建File对象
// File f1 = new File("C:\\Users\\ZhongjieMa\\Desktop\\mamamam");
//
// //判断File是否存在
// System.out.println(f1.exists());
//如果File不存在,则以文件的形式创建出来
// if(!f1.exists()){
// f1.createNewFile();
// }
//如果File不存在,则以目录的形式创建出来
// if (!f1.exists()){
// f1.mkdir();
// }
//可以创建多重目录吗
File f2 = new File("C:/Users/ZhongjieMa/Desktop/mamamam/a/b/d");
if (!f2.exists()){
f2.mkdirs();
}
File f3 = new File("C:/Users/ZhongjieMa/Desktop/copy.jpg");
//获取该文件的父路径
System.out.println(f3.getParent());
//获取绝对路径
System.out.println(f3.getAbsolutePath());
File f4 = new File("temp.txt");
System.out.println("绝对路径:"+f4.getAbsolutePath());
}
}
p744 File类常用方法
package com.bjpowernode.java.io;
import java.io.File;
import java.text.SimpleDateFormat;
import java.util.Date;
public class FileTest02 {
public static void main(String[] args) {
File f1 = new File("D:\\ZhongjieMa\\Documents\\学习思维导图.pdf");
//获取文件名
System.out.println("文件名:"+ f1.getName());
//判断是否是一个目录
System.out.println(f1.isDirectory());
//判断是否是一个文件
System.out.println(f1.isFile());
//获取文件最后一次修改时间
long haoMiao = f1.lastModified();//返回的是从1970年1月1日到现在的总毫秒数
//将毫秒数转换为日期
Date time = new Date(haoMiao);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss SSS");
String strTime = sdf.format(time);
System.out.println("最后的修改时间为:"+strTime);
//获取文件大小
System.out.println(f1.getName()+"的大小为:"+f1.length()+"字节");
}
}
p745 File类常用方法
package com.bjpowernode.java.io;
import java.io.File;
public class FileTest03 {
public static void main(String[] args) {
File f1 = new File("C:\\Users\\ZhongjieMa\\Desktop");
//判断是否是一个目录
System.out.println(f1.isDirectory());
File[] files = f1.listFiles();
for (File file : files){
//获取目录下的所有子文件
System.out.println(file.getAbsolutePath());
//获取当前目录下的所有文件名
System.out.println(file.getName());
}
}
}
p746 作业布置
p747 拷贝目录1-p748 拷贝目录2
package com.bjpowernode.java.io.homework;
import java.io.*;
public class CopyAll {
public static void main(String[] args) {
//拷贝源
File srcFile = new File("G:\\Study\\JAVA\\java\\JAVA基础视频\\动力节点\\001-JavaSE课堂笔记+思维导图");
//拷贝目录
File tarFile = new File("C:\\Users\\ZhongjieMa\\Desktop\\测试");
copyDir(srcFile,tarFile);
}
/*
拷贝方法
*/
private static void copyDir(File srcFile, File tarFile) {
if (srcFile.isFile()){
//如果是一个文件的话,需要拷贝
//一边读一边写
FileInputStream fls = null;
FileOutputStream fos = null;
try {
fls = new FileInputStream(srcFile);
//fos = new FileOutputStream(tarFile);//在这里不能使用tarDir,要随时更新
String path = tarFile.getAbsolutePath()+srcFile.getAbsolutePath().substring(2);
fos = new FileOutputStream(path);
//System.out.println(path);
//一边读一边写
byte[] bytes = new byte[1024 *1024];
int readCount = 0;
while((readCount = fls.read(bytes)) != -1){
fos.write(bytes,0,readCount);
}
fos.flush();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if (fos != null) {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fls != null) {
try {
fls.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//获取源下面的子目录
File[] files = srcFile.listFiles();
for (File file : files){
//获取所有文件(文件和目录)的绝对路径
//System.out.println(file.getAbsolutePath());
if (file.isDirectory()){
//System.out.println(file.getAbsolutePath());
//G:\Study\JAVA\java\JAVA基础视频\动力节点\001-JavaSE课堂笔记+思维导图\02-JavaSE零基础每日复习与笔记 源目录
//C:\Users\ZhongjieMa\Desktop\Study\JAVA\java\JAVA基础视频\动力节点\001-JavaSE课堂笔记+思维导图\02-JavaSE零基础每日复习与笔记 目标目录
//得出源目录
String srcDir = file.getAbsolutePath();
System.out.println(srcDir);
//得出目标目录
String tarDir = (tarFile.getAbsolutePath().endsWith("\\") ? tarFile.getAbsolutePath() : tarFile.getAbsolutePath() + "\\")+srcDir.substring(2);
System.out.println(tarDir);
//创建新目录
File newFile = new File(tarDir);
if (! newFile.exists()){
newFile.mkdirs();
}
}
//递归调用
copyDir(file,tarFile);
}
}
}
程序目前还是有点问题,等下次在改