高亮颜色说明:突出重点
个人觉得,:待核准个人观点是否有误
本篇博客涵盖以下内容:
- 起步
- 变量和简单数据类型
- 列表简介
- 操作列表
- if语句
- 字典
- 用户输入和while循环
文章目录
打印输出python版本号
打印输出python版本号
# 通过使用platform模块
import platform
print(platform.python_version())
# 通过使用sys模块
import sys
print(sys.version)
print(sys.version_info)
Python中的下划线命名规则
(待阅读) 详解 Python 中的下划线命名规则 - leejun2005的个人页面 - OSCHINA 20150315
Python中的常用操作
range(start, stop[, step])
# 当step取正值或为空时, start的取值应该小于stop;
>>> list(range(0, 10))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> list(range(-10, 0))
[-10, -9, -8, -7, -6, -5, -4, -3, -2, -1]
# 错误示例
>>> list(range(10, 0))
[]
>>> list(range(0, -10))
[]
# 当step取负值时, start的取值应该大于stop;
>>> list(range(10, 0, -1))
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
>>> list(range(0, -10, -1))
[0, -1, -2, -3, -4, -5, -6, -7, -8, -9]
# 错误示例
>>> list(range(0, 10, -1))
[]
>>> list(range(-10, 0, -1))
[]
tqdm 进度可视化
- tqdm介绍及常用方法_qq60cc3533dc52a的技术博客_51CTO博客 20210618
- 使用python Tqdm进度条库让你的python进度可视化 - pytorch中文网 20180513
Python运算符
通配符*
的用法
通配符「*
」,英文叫 wildcard,在计算机语言中代表一个或多个元素。
*args
,元组args前面加个通配符*
是拆散元组,把元组中的元素传入函数中;**kwargs
,字典kwargs前面加两个通配符**
是拆散字典,把字典中的元素(键值对)传入函数中;- 解压元组时,用
*
c就是把多个元素都丢给了 c 变量:
t = 1, 2, 3, 4, 5
a, b, *c, d = t # 解压元组时,用*c就是把多个元素都丢给了 c 变量
print(a, b, d)
>>> 1 2 5
print(c)
>>> [3, 4]
+=和=+
(待阅读)Python中的+= - 云+社区 - 腾讯云 20191218
/ 和 //
/
表示浮点数除法, 返回浮点数结果;//
表示整数除法, 返回向下取整的整数结果;
列表list
list的复制
Python 正确复制列表的方法 - iFantasticMe - 博客园 20140627
Python中List的复制(直接复制、浅拷贝、深拷贝)_今晚打老虎-CSDN博客 20200205
嵌套列表的创建
python 嵌套列表创建_hello_program_world的博客-CSDN博客 20201226
元组tuple
集合set
字典dict
字典.get()方法
[dict() for _ in range(3)]
和 [dict(),] * 3
之间的区别
tmp_a = [dict() for _ in range(3)]
tmp_b = [dict(),] * 3
tmp_a_id = [id(x) for x in tmp_a]
tmp_b_id = [id(x) for x in tmp_b]
tmp_a_id
[3023482152584, 3023482225432, 3023482225912]
tmp_b_id
[3023482193304, 3023482193304, 3023482193304]
Python中字符串相关的操作
Python中打印输出时的格式控制
output_1 = 'one1two2three3four4'
output_1 = 'one1two2three3four4'
output_2 = 1234
# {!r}需要与.format()配合使用, 且打印输出的字符串包含单引号, 但数字不包含引号
print('this is {!r}, {!r}'.format(output_1, output_1)) # this is 'one1two2three3four4', 'one1two2three3four4'
print('this is {!r}, {!r}'.format(output_2, output_2)) # this is 1234, 1234
# 打印输出的值不包含单引号
print('this is', output_1) # this is one1two2three3four4
print('this is {}, {}'.format(output_1, output_1)) # this is one1two2three3four4, one1two2three3four4
print(f'this is {output_1}, {output_1}') # this is one1two2three3four4, one1two2three3four4
print(f'this is %s, %d' % (output_1, output_2)) # this is one1two2three3four4, 1234
print(f'this is %(dict_key1)s, %(dict_key2)d' % {'dict_key1':'dict_val1', 'dict_key2':1234}) # this is dict_val1, 1234
# %r需要与%配合使用, 且打印输出的字符串包含单引号, 但数字不包含引号
print(f'this is %r, %d' % (output_1, output_2)) # this is 'one1two2three3four4', 1234
print(f'this is %(dict_key1)r, %(dict_key2)d' % {'dict_key1':'dict_val1', 'dict_key2':1234}) # this is 'dict_val1', 1234
print('this is %r' % output_1) # this is 'one1two2three3four4'
print('this is %r' % output_2) # this is 1234
print('this is %r', output_1) # this is %r one1two2three3four4
python字符串格式化 %操作符 {}操作符—总结 - 目标120 - 博客园 20161010
.format()方法的格式控制
print(“{ <参数序号> : <格式控制标记>}”.format())
: | <填充> | <对齐> | <宽度> | < , > | < . 精度> | <类型> |
---|---|---|---|---|---|---|
引导符号 | 用于填充的单个字符 | < 左对齐 > 右对齐 ^居中对齐 | 槽设定的输出宽度 | 数字的千位分隔符 | 浮点数小数精度 或 字符串最大输出长度 | 整数类型 b, c, d, o, x, X 浮点数类型 e, E, f, % |
# example1
print("{0:=^20}".format("PYTHON"))
=======PYTHON=======
# example2
print("{0:*>20},".format("BIT"))
*****************BIT,
# example3
print("{:20},".format("BIT"))
BIT ,
# example4:
print("{0:,.2f}".format(12345.6789))
‘12,345.68’
# example5
print("{0:b}\n{0:c}\n{0:d}\n{0:o}\n{0:x}\n{0:X}".format(425))
110101001
Ʃ
425
651
1a9
1A9
# example6
print("{0:e}\n{0:E}\n{0:f}\n{0:%}".format(3.14))
3.140000e+00
3.140000E+00
3.140000
314.000000%
Python中的正则表达式(regular expression)
python正则表达式 re (一)_Winterto1990的博客 20150808
python正则表达式 re (二)compile_Winterto1990的博客 20150808
python里使用正则的finditer()函数_大坡3D软件开发 20171007
re — Regular expression operations — Python 3.9.4 documentation
re — Regular expression operations
This module provides regular expression matching operations similar to those found in Perl.
一些术语
- regular expression patterns;
- strings to be searched;
- module-level functions and methods on compiled regular expressions;
- compiled regular expression objects support the following methods and attributes;
一些摘录
- similarly, when asking for a substitution, the replacement string must be of the same type as both the pattern and the search string.
- each backslash must be expressed as \ inside a regular Python string literal; 在匹配字符串字母值中的1个反斜杠字符时, 正则表达式中应该也写为’\';
- 建议to use Python’s raw string notation for regular expression patterns; backslashes are not handled in any special way in a string literal prefixed with
'r'
. Sor"\n"
is a two-character string containing'\'
and'n'
, while"\n"
is a one-character string containing a newline. Usually patterns will be expressed in Python code using this raw string notation.
Regular Expression Syntax
A regular expression (or RE) specifies a set of strings that matches it; the functions in this module let you check if a particular string matches a given regular expression (or if a given regular expression matches a particular string, which comes down to the same thing).
A brief explanation of the format of regular expressions follows. For further information and a gentler presentation, consult the Regular Expression HOWTO.
Module Contents
- 如果某个正则表达式用到的次数比较少, 可以直接调用模块级的函数/方法, 类似于
result = re.match(pattern, string)
这种形式; - 如果某个正则表达式需要多次重复使用, 建议先创建a regular expression object, 再调用正则表达式对象的方法, 类似于
prog = re.compile(pattern) result = prog.match(string)
这种形式;
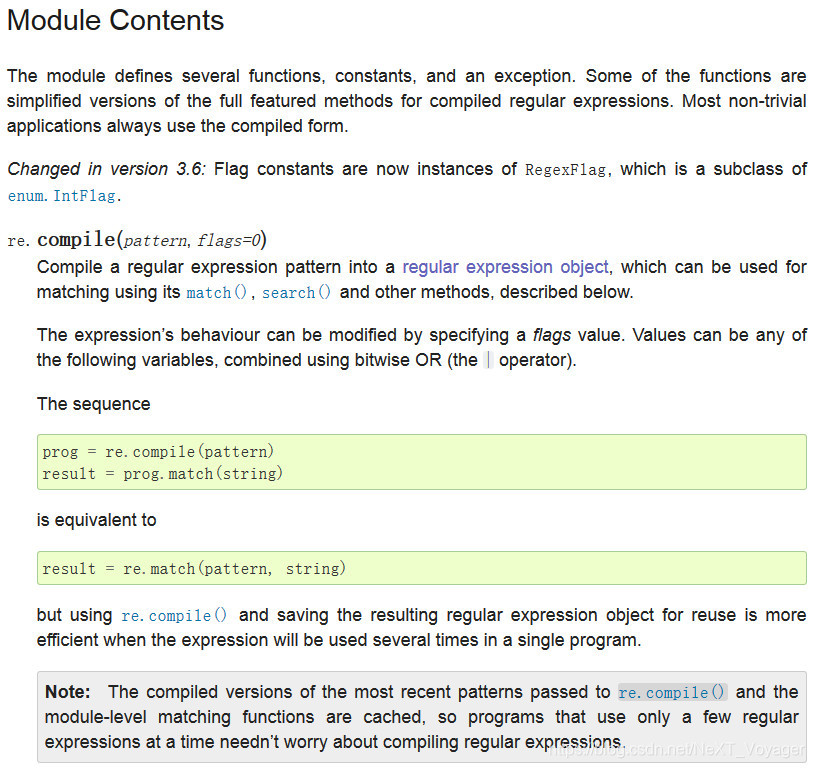
Python字符串分割
Python去除字符串中空格
Python判断字符串中是否包含指定子字符串
- Python判断一个字符串是否包含子串的几种方法_三五月儿的专栏-CSDN博客 20160731
- Python如何判断一个字符串是否包含指定子字符串-Python教程-PHP中文网 20181117
- Python判断一个字符串是否包含指定字符串的方法 - lu11 - 博客园 20181212
Python将列表转换为字符串
Python中将列表转换为字符串的方法:
-
使用join()函数
join()函数将列表中的每个元素合并成一个字符串,并以指定的字符串为分隔符将它们连接起来。list = ['a', 'b', 'c'] str = ''.join(list) # 空字符串''作为分隔符,则在列表元素之间不插入任何字符; print(str) # 输出: abc str = ' '.join(list) # 一个空格字符' '作为分隔符,则在列表元素之间插入一个空格字符; print(str) # 输出: a b c
-
使用map()函数和join()函数
map()函数可以将一个列表中的元素应用到一个函数中,并返回一个新的列表。我们可以使用map()函数将列表中的元素转换为字符串类型,然后使用join()函数将它们连接起来。list = ['1', '2', '3'] str = ''.join(map(str, list)) # map()函数中的str表示将列表元素转换为字符串类型; print(str) # 输出: 123
-
使用列表生成式和join()函数
列表生成式是一种简洁的语法,可以将一个列表中的元素转换为另一个列表。我们可以使用列表生成式将列表中的元素转换为字符串类型,然后使用join()函数将它们连接起来。list = [1, 2, 3] str = ''.join([str(i) for i in list]) # 列表生成式中的str(i)表示将列表元素i转换为字符串类型; print(str) # 输出: 123
-
使用reduce()函数和join()函数
reduce()函数是Python中的一个内置函数,可以将一个列表中的元素逐个应用到一个函数中,并返回一个汇总结果。我们可以使用reduce()函数将列表中的元素逐个连接起来。from functools import reduce list = ['1', '2', '3'] str = reduce(lambda x, y: x + y, list) # reduce()函数中的lambda表达式表示将两个字符串相加,从而将列表中的所有字符串连接起来; print(str) # 输出: 123
Python数字转字符串 前补零
20240128记:百度检索"python数字转字符串 前补零"
- python int转字符前面补零_mob64ca12e1881c的技术博客_51CTO博客 20231111
- python的数字与字符串相互转换-腾讯云开发者社区-腾讯云 20200721
- python数字转字符串 前补零 - 优草派 20231208
Python获取秒级时间戳与毫秒级时间戳
*** Python获取秒级时间戳与毫秒级时间戳 - 北方卧龙 - 博客园 20190924
datetime库 日期与时间 - pywjh - 博客园 20180823
本博文包含以下内容:
- datetime是一个关于时间的库,主要包含的类有:
- date 日期对象,常用的属性有year,month,day;
- time 时间对象,hour,minute,second,microsecond;
- datetime 日期时间对象,常用的属性有hour,minute,second,microsecond;
- timedelta 时间间隔,即两个时间点之间的长度;
- 主要的操作有:
- 返回当前系统时间(now);
- 解析时间(strptime):按一定的格式输出时间(字符串时间转化为datetime格式);
- 格式化时间(strftime):完全自定义时间格式(将时间格式转化为自定义字符串格式);
- 关于时间格式的汇总;
- fromtimestamp(t)将时间戳浮点数转换成易读的日期时间;
- timestamp(t)将日期时间转换成时间戳浮点数;
将字符串转换为时间戳
20230222,谷歌检索"python yy/mm/dd hh:mm:ss string to datetime",
- Convert datetime string to YYYY-MM-DD-HH:MM:SS format in Python - GeeksforGeeks 20221219
strptime()
is used to convert the DateTime string to DateTime in the format of year-month-day hours minutes and seconds.
datetime.strptime(my_date, “%d-%b-%Y-%H:%M:%S”)
strftime()
is used to convert DateTime into required timestamp format.
datetime.strftime(“%Y-%m-%d-%H:%M:%S”) - Convert String to datetime in Python – thisPointer
python - Using datetime.strptime with microseconds (ValueError: unconverted data remains: :00) - Stack Overflow 20211228
That’s not microseconds, but a time zone offset in hours and minutes. - python 2.7 - datetime strptime method for format HH:MM:SS.MICROSECOND - Stack Overflow 20190226
if语句
使用案例 any(b in a for b in list_b)
案例一,
# 如果 list_exclude 中的任何一个元素都不在 tmp_a 中, 则怎么怎么样...
if not any(x in tmp_a for x in list_exclude):
pass
案例二,
for a in list_a:
# 如果 a 包含了 list_b 中的任何一个元素, 则怎么怎么样...
if any(b in a for b in list_b):
pass
待补充
待补充