需要用到的jar包
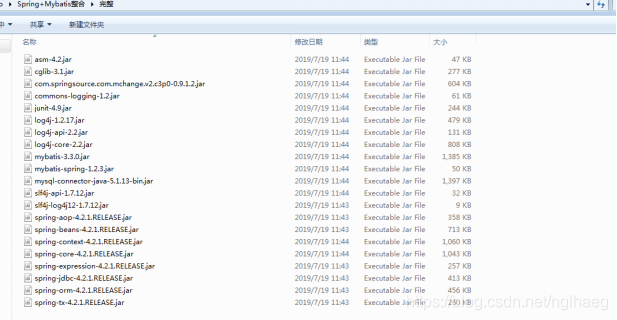
建如下包和类以及xml
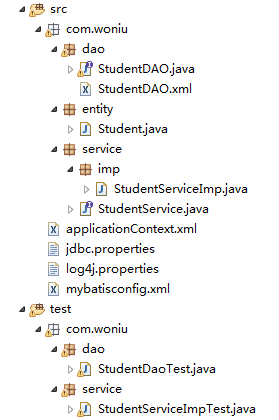
mybatisconfig.xml配置文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<package name="com.woniu.entity"/>
</typeAliases>
<mappers>
<package name="com.woniu.dao"/>
</mappers>
</configuration>
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="configLocation" value="classpath:mybatisconfig.xml" />
<property name="dataSource" ref="dataSource" />
</bean>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driver}" />
<property name="jdbcUrl" value="${jdbc.url}" />
<property name="user" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
</bean>
<context:property-placeholder location="classpath:jdbc.properties" />
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.woniu.dao"/>
<property name="sqlSessionFactoryBeanName" value="sqlSessionFactory" />
</bean>
<bean id="studentService" class="com.woniu.service.imp.StudentServiceImp">
<property name="studentDao" ref="studentDAO"/>
</bean>
</beans>
Student.java
public class Student {
private Integer sid;
private String sname;
private Integer sage;
private Character ssex;
private Integer cid;
public Student() {
super();
}
public Student(String sname, Integer sage, Character ssex, Integer cid) {
super();
this.sname = sname;
this.sage = sage;
this.ssex = ssex;
this.cid = cid;
}
public Student(Integer sid, String sname, Integer sage, Character ssex, Integer cid) {
super();
this.sid = sid;
this.sname = sname;
this.sage = sage;
this.ssex = ssex;
this.cid = cid;
}
public Integer getSid() {
return sid;
}
public void setSid(Integer sid) {
this.sid = sid;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public Integer getSage() {
return sage;
}
public void setSage(Integer sage) {
this.sage = sage;
}
public Character getSsex() {
return ssex;
}
public void setSsex(Character ssex) {
this.ssex = ssex;
}
public Integer getCid() {
return cid;
}
public void setCid(Integer cid) {
this.cid = cid;
}
@Override
public String toString() {
return "Student [sid=" + sid + ", sname=" + sname + ", sage=" + sage + ", ssex=" + ssex + ", cid=" + cid + "]";
}
}
StudentDAO.java
public interface StudentDAO {
void insertStudent(Student student);
}
StudentDAO.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.woniu.dao.StudentDAO">
<insert id="insertStudent">
insert into student values(null,#{sname},#{sage},#{ssex},#{cid},0)
</insert>
</mapper>
StudentService.java
public interface StudentService {
void addStudent(Student student);
}
StudentServiceImp.java
public class StudentServiceImp implements StudentService{
StudentDAO studentDao;
public void setStudentDao(StudentDAO studentDao) {
this.studentDao = studentDao;
}
@Override
public void addStudent(Student student) {
studentDao.insertStudent(student);
}
}
jdbc.properties
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql:///infobase
jdbc.username=root
jdbc.password=123456
StudentServiceImpTest.java
public class StudentServiceImpTest {
StudentService studentService;
@Before
public void setUp() {
ApplicationContext ac = new ClassPathXmlApplicationContext("applicationContext.xml");
studentService = (StudentService) ac.getBean("studentService");
}
@Test
public void testInsertStudent() {
Student student = new Student("张三",20,'男',2);
studentService.addStudent(student);
}
}