1. 概述
2. 固件库
gpio_af_set(); //pin复用模式
gpio_mode_set(); //IO模式:输入输出、上下拉
gpio_output_options_set(); //推拉、速率
3. 工程配置
3.1 选择型号
gd32f4xx.h中要选择对应型号的定义:GD32F450
/* define GD32F4xx */
#if !defined (GD32F450) && !defined (GD32F405) && !defined (GD32F407)
/* #define GD32F450 */
#define GD32F450
/* #define GD32F405 */
/* #define GD32F407 */
#endif /* define GD32F4xx */
3.2 网络
lwip的mac地址和芯片mac寄存器中的mac地址要一致。
(1)lwip的mac地址在drv_enet.c中的rt_hw_gd32_eth_init(void)函数中定义:
// OUI 00-00-0E FUJITSU LIMITED
gd32_emac_device0.dev_addr[0] = 0x00; //mac地址
gd32_emac_device0.dev_addr[1] = 0x00;
gd32_emac_device0.dev_addr[2] = 0x0E;
/* set mac address: (only for test) */
gd32_emac_device0.dev_addr[3] = 0x12;
gd32_emac_device0.dev_addr[4] = 0x34;
gd32_emac_device0.dev_addr[5] = 0x56;
(2)程序寄存器的mac地址:
drv_enet.c中的rt_hw_gd32_eth_init(void)函数中调用EMAC_MAC_Addr_config():
//drv_enet.c
rt_hw_gd32_eth_init(void)
EMAC_MAC_Addr_config(ETHERNET_MAC, EMAC_MAC_Address0, (uint8_t*)&gd32_emac_device->dev_addr[0]);
//synopsys_emac.c
void EMAC_MAC_Addr_config(struct rt_synopsys_eth * ETHERNET_MAC, rt_uint32_t MacAddr, rt_uint8_t *Addr)
3.3 lwip
3.3.0 设置固定IP
注意要把lwip的DCHP功能去掉,并把网关的初始值也去掉。分别去掉“Disable alloc ip address through DCHP”、“Static IPv4 Address”中设置。
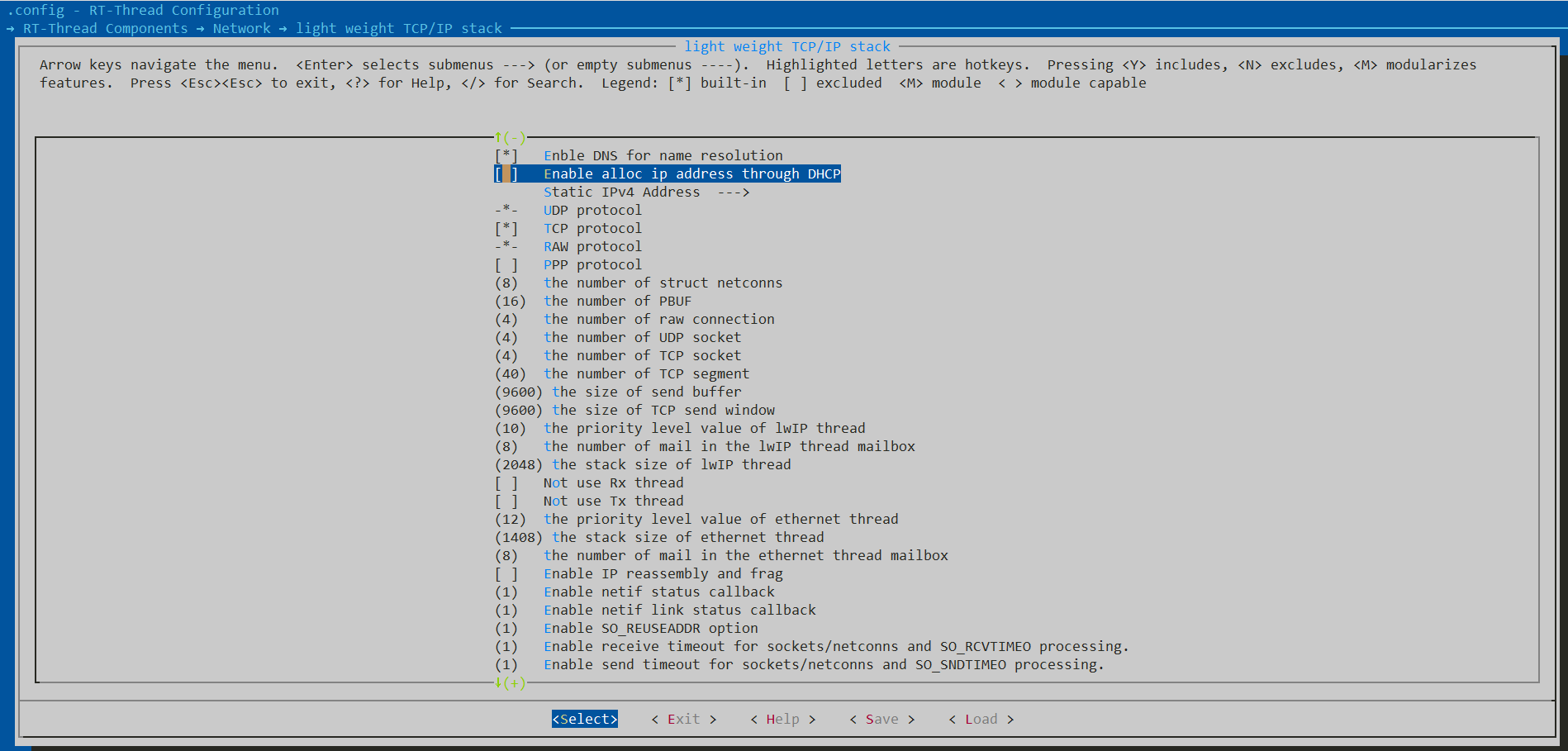
3.3.1 移植修改
//gd32f4xx_enet.h中
#if(PHY_TYPE == LAN8700)
#define PHY_SR 31U /*!< tranceiver status register */
#define PHY_SPEED_STATUS ((uint16_t)0x0004) /*!< configured information of speed: 10Mbit/s */
#define PHY_DUPLEX_STATUS ((uint16_t)0x0010) /*!< configured information of duplex: full-duplex */
#elif(PHY_TYPE == DP83848)
#define PHY_SR 16U /*!< tranceiver status register */
#define PHY_SPEED_STATUS ((uint16_t)0x0002) /*!< configured information of speed: 10Mbit/s */
#define PHY_DUPLEX_STATUS ((uint16_t)0x0004) /*!< configured information of duplex: full-duplex */
#elif(PHY_TYPE == YT8512)
#define PHY_SR 17U /*!< tranceiver status register */
#define PHY_SPEED_STATUS ((uint16_t)0x4000) /*!< configured information of speed: 10Mbit/s */
#define PHY_DUPLEX_STATUS ((uint16_t)0x2000) /*!< configured information of duplex: full-duplex */
#endif /* PHY_TYPE */
//gd32f4xx_enet.c中的
ErrStatus enet_init(enet_mediamode_enum mediamode, enet_chksumconf_enum checksum, enet_frmrecept_enum recept)
if((uint16_t)RESET != (phy_value & PHY_DUPLEX_STATUS)){
media_temp = ENET_MODE_FULLDUPLEX;
}else{
media_temp = ENET_MODE_HALFDUPLEX;
}
/* configure the communication speed of MAC following the auto-negotiation result */
if((uint16_t)RESET !=(phy_value & PHY_SPEED_STATUS)){ //设置速率---
media_temp |= ENET_SPEEDMODE_100M; //为1时
}else{
media_temp |= ENET_SPEEDMODE_10M;
}
3.3.2 启动流程
- 1、lwip初始化在:int lwip_system_init(void)
- 2、使用lwip注册设备:
int rt_hw_gd32_eth_init(void)
{
rt_kprintf("rt_gd32_eth_init...\n");
/* enable ethernet clock */
rcu_periph_clock_enable(RCU_ENET);
rcu_periph_clock_enable(RCU_ENETTX);
rcu_periph_clock_enable(RCU_ENETRX);
nvic_configuration(); //中断配置
/* configure the GPIO ports for ethernet pins */
enet_gpio_config(); //管脚配置
/* set autonegotiation mode */
gd32_emac_device0.phy_mode = EMAC_PHY_AUTO;
gd32_emac_device0.ETHERNET_MAC = ETHERNET_MAC0; //寄存器地址
gd32_emac_device0.ETHER_MAC_IRQ = ENET_IRQn;
// OUI 00-00-0E FUJITSU LIMITED
gd32_emac_device0.dev_addr[0] = 0x00; //mac地址
gd32_emac_device0.dev_addr[1] = 0x00;
gd32_emac_device0.dev_addr[2] = 0x0E;
/* set mac address: (only for test) */
gd32_emac_device0.dev_addr[3] = 0x12;
gd32_emac_device0.dev_addr[4] = 0x34;
gd32_emac_device0.dev_addr[5] = 0x56;
gd32_emac_device0.parent.parent.init = gd32_emac_init; //mac初始化
gd32_emac_device0.parent.parent.open = gd32_emac_open;
gd32_emac_device0.parent.parent.close = gd32_emac_close;
gd32_emac_device0.parent.parent.read = gd32_emac_read;
gd32_emac_device0.parent.parent.write = gd32_emac_write;
gd32_emac_device0.parent.parent.control = gd32_emac_control;
gd32_emac_device0.parent.parent.user_data = RT_NULL;
gd32_emac_device0.parent.eth_rx = gd32_emac_rx;
gd32_emac_device0.parent.eth_tx = gd32_emac_tx;
/* init tx buffer free semaphore */
rt_sem_init(&gd32_emac_device0.tx_buf_free, "tx_buf0", EMAC_TXBUFNB, RT_IPC_FLAG_FIFO);
eth_device_init(&(gd32_emac_device0.parent), "e0"); //lwip向系统注册网卡e0-----
/* change device link status */
eth_device_linkchange(&(gd32_emac_device0.parent), RT_TRUE);
return 0;
}
INIT_DEVICE_EXPORT(rt_hw_gd32_eth_init);
管脚配置要根据板子实际来初始化:
- 3、netif_set_status_callback()函数可以注册NETIF_STATUS_CALLBACK回调函数,有3个地方调用:
netif_set_up、netif_set_down、netif_set_ipaddr