示例
SSM + PageHelper
紧接上篇内容:
1.创建Dao接口
CountryDao.java
/**
* @author Ray
* @date 2018/6/2 0002
*/
public interface CountryDao{
/**
* 查询所有国家信息
*/
List<Country> selectByCountryQueryModel(Country country);
/**
* 根据id查询所有国家信息
*/
Country selectByKey(@Param("id") Integer id);
/**
* 修改国家信息
*/
void update(Country country);
/**
* 新增国家信息
*/
void insert(Country country);
/**
* 删除国家信息
*/
void delete(@Param("id") Integer id);
}
2.创建完Dao层,一般都会测试是否有误
BastTest.java
PageHelperTest.java
public class PageHelperTest extends BaseTest{
private Logger logger = LoggerFactory.getLogger(this.getClass());
@Autowired
private CountryDao countryDao;
@Test
public void selectByKey(){
Country country = countryDao.selectByKey(6);
System.out.println(country);
}
}
3.创建Service和Impl
CountryService.java
/**
* @author Ray
* @date 2018/6/2 0002
*/
public interface CountryService {
Country selectByKey(Integer id);
List<Country> selectByCountryQueryModel(Country country, int page, int rows);
void insert(Country country);
void update(Country country);
void delete(Integer id);
}
CountryServiceImpl.java
/**
* @author Ray
* @date 2018/6/2 0002
*/
@Service //@Service用于标注业务层组件
public class CountryServiceImpl implements CountryService {
@Autowired //@Autowired按byType自动注入
private CountryDao countryDao;
@Override
public Country selectByKey(Integer id) {
return countryDao.selectByKey(id);
}
@Override
public List<Country> selectByCountryQueryModel(Country country, int page, int rows) {
// 分页查询,从第page页开始,每页显示rows条记录
PageHelper.startPage(page, rows);
// 只有紧跟在 PageHelper.startPage 方法后的第一个 Mybatis 的查询(Select)方法会被分页(重点)
return countryDao.selectByCountryQueryModel(country);
}
@Override
public void insert(Country country) {
countryDao.insert(country);
}
@Override
public void update(Country country) {
countryDao.update(country);
}
@Override
public void delete(Integer id) {
countryDao.delete(id);
}
}
4.创建Controller
CountryController.java
/**
* @author Ray
* @date 2018/6/2 0002
*/
@Controller //@Controller用于标注控制层组件(如struts中的action)
public class CountryController {
@Autowired
private CountryService countryService;
private String page_list = "list"; //分页页面
private String page_view = "view"; //修改页面
private String redirect_list = "redirect:list"; //重定向,地址栏的url变成了新的url
/**
* 分页视图
*/
@RequestMapping(value = {"list", "index", "index.html"})
public ModelAndView getList(Country country,
@RequestParam(required = false, defaultValue = "1") int page,
@RequestParam(required = false, defaultValue = "10") int rows){
ModelAndView modelAndView = new ModelAndView(page_list);
// 执行查询操作
List<Country> countryList = countryService.selectByCountryQueryModel(country, page, rows);
// 使用参数名生成向模型添加属性
modelAndView.addObject("pageInfo", new PageInfo<Country>(countryList)); // 分页控制(重点)
modelAndView.addObject("country", country); // country对象
modelAndView.addObject("page", page); // 页码
modelAndView.addObject("rows", rows); // 行数
return modelAndView;
}
/**
* 修改视图
*/
@RequestMapping(value = "view", method = RequestMethod.GET)
public ModelAndView getView(Country country){
ModelAndView modelAndView = new ModelAndView(page_view);
if(country.getId() != null){
// 查询操作
country = countryService.selectByKey(country.getId());
}
// 使用参数名生成向模型添加属性
modelAndView.addObject("country", country);
return modelAndView;
}
/**
* 新增视图
*/
@RequestMapping(value = "save", method = RequestMethod.POST)
public ModelAndView save(Country country){
ModelAndView modelAndView = new ModelAndView(redirect_list);
if(country.getId() != null){
// 修改操作
countryService.update(country);
}else{
// 新增操作
countryService.insert(country);
}
return modelAndView;
}
/**
* 删除操作
*/
@RequestMapping(value = "delete")
public String delete(Integer id){
countryService.delete(id);
return redirect_list;
}
}
5.创建视图页面
list.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<html>
<head>
<title>Mybatis分页插件 - 测试页面</title>
<script src="${pageContext.request.contextPath}/static/js/jquery-1.11.1.min.js"></script>
<link href="${pageContext.request.contextPath}/static/css/style.css" rel="stylesheet" type="text/css"/>
<style type="text/css">
.pageDetail {
display: none;
}
.show {
display: table-row;
}
</style>
<script>
$(function () {
$('#list').click(function () {
$('.pageDetail').toggleClass('show');
});
});
</script>
</head>
<body>
<div class="wrapper">
<div class="middle">
<h1 style="padding: 50px 0 20px;">国家(地区)列表</h1>
<form action="${pageContext.request.contextPath}/list" method="post">
<table class="gridtable" style="width: 100%;">
<tr>
<th>国家(地区)名称:</th>
<td><input type="text" name="countryname" value="${country.countryname}"/></td>
<th>国家(地区)代码:</th>
<td><input type="text" name="countrycode" value="${country.countrycode}"/></td>
<td rowspan="2"><input type="submit" value="查询"/></td>
</tr>
<tr>
<th>页码:</th>
<td><input type="text" name="page" value="${page}"></td>
<th>页面大小:</th>
<td><input type="text" name="rows" value="${rows}"></td>
</tr>
</table>
<table class="gridtable" style="width:100%;">
<tr>
<th colspan="2">分页信息 - [<a href="javascript:;" id="list">展开/收缩</a>]</th>
</tr>
<tr class="pageDetail">
<th style="width: 300px;">当前页号-pageNum</th>
<td>${pageInfo.pageNum}</td>
</tr>
<tr class="pageDetail">
<th>页面大小-pageSize</th>
<td>${pageInfo.pageSize}</td>
</tr>
<tr class="pageDetail">
<th>起始行号(>=)-startRow</th>
<td>${pageInfo.startRow}</td>
</tr>
<tr class="pageDetail">
<th>终止行号(<=)-endRow</th>
<td>${pageInfo.endRow}</td>
</tr>
<tr class="pageDetail">
<th>总结果数-total</th>
<td>${pageInfo.total}</td>
</tr>
<tr class="pageDetail">
<th>总页数-pages</th>
<td>${pageInfo.pages}</td>
</tr>
<tr class="pageDetail">
<th>第一页-firstPage</th>
<td>${pageInfo.firstPage}</td>
</tr>
<tr class="pageDetail">
<th>前一页-prePage</th>
<td>${pageInfo.prePage}</td>
</tr>
<tr class="pageDetail">
<th>下一页-nextPage</th>
<td>${pageInfo.nextPage}</td>
</tr>
<tr class="pageDetail">
<th>最后一页-lastPage</th>
<td>${pageInfo.lastPage}</td>
</tr>
<tr class="pageDetail">
<th>是否为第一页-isFirstPage</th>
<td>${pageInfo.isFirstPage}</td>
</tr>
<tr class="pageDetail">
<th>是否为最后一页-isLastPage</th>
<td>${pageInfo.isLastPage}</td>
</tr>
<tr class="pageDetail">
<th>是否有前一页-hasPreviousPage</th>
<td>${pageInfo.hasPreviousPage}</td>
</tr>
<tr class="pageDetail">
<th>是否有下一页-hasNextPage</th>
<td>${pageInfo.hasNextPage}</td>
</tr>
</table>
<table class="gridtable" style="width: 100%;">
<thead>
<tr>
<td colspan="4">查询结果 - [<a href="${pageContext.request.contextPath}/view">新增国家(地区)</a> ]</td>
</tr>
<tr>
<th>ID</th>
<th>国家(地区)名</th>
<th>国家(地区)代码</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${pageInfo.list}" var="country">
<tr>
<td>${country.id}</td>
<td>${country.countryname}</td>
<td>${country.countrycode}</td>
<td style="text-align: center">
[<a href="${pageContext.request.contextPath}/view?id=${country.id}">修改</a> ]
[<a href="${pageContext.request.contextPath}/delete?id=${country.id}">删除</a> ]
</td>
</tr>
</c:forEach>
</tbody>
</table>
<table class="gridtable" style="width: 100%;text-align: center;">
<tr>
<c:if test="${pageInfo.hasPreviousPage}">
<td>
<a href="${pageContext.request.contextPath}/list?page=1&rows=${pageInfo.pageSize}&countryname=${country.countryname}&countrycode=${country.countrycode}">首页</a>
</td>
<td>
<a href="${pageContext.request.contextPath}/list?page=${pageInfo.prePage}&rows=${pageInfo.pageSize}&countryname=${country.countryname}&countrycode=${country.countrycode}">上一页</a>
</td>
</c:if>
<c:forEach items="${pageInfo.navigatepageNums}" var="nav">
<c:if test="${nav == pageInfo.pageNum}">
<td style="font-weight: bold;">${nav}</td>
</c:if>
<c:if test="${nav != pageInfo.pageNum}">
<td>
<a href="${pageContext.request.contextPath}/list?page=${nav}&rows=${pageInfo.pageSize}&countryname=${country.countryname}&countrycode=${country.countrycode}">${nav}</a>
</td>
</c:if>
</c:forEach>
<c:if test="${pageInfo.hasNextPage}">
<td>
<a href="${pageContext.request.contextPath}/list?page=${pageInfo.nextPage}&rows=${pageInfo.pageSize}&countryname=${country.countryname}&countrycode=${country.countrycode}">下一页</a>
</td>
<td>
<a href="${pageContext.request.contextPath}/list?page=${pageInfo.pages}&rows=${pageInfo.pageSize}&countryname=${country.countryname}&countrycode=${country.countrycode}">末页</a>
</td>
</c:if>
</tr>
</table>
</form>
</div>
<div class="push"></div>
</div>
</body>
view.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>国家(地区)信息</title>
<link href="${pageContext.request.contextPath}/static/css/style.css" rel="stylesheet" type="text/css"/>
</head>
<body style="margin-top: 50px;overflow: hidden;">
<form action="${pageContext.request.contextPath}/save" method="post">
<input type="hidden" name="id" value="${country.id}">
<table class="gridtable" style="width: 800px;">
<tr>
<th colspan="5">国家(地区)信息 - [<a href="${pageContext.request.contextPath}/list">返回首页</a> ]</th>
</tr>
<tr>
<th>国家(地区)名称:</th>
<td><input type="text" name="countryname" value="${country.countryname}"></td>
<th>国家(地区)代码:</th>
<td><input type="text" name="countrycode" value="${country.countrycode}"></td>
<td><input type="submit" value="保存"></td>
</tr>
</table>
</form>
</body>
style.css
jquery.js
6.测试页面
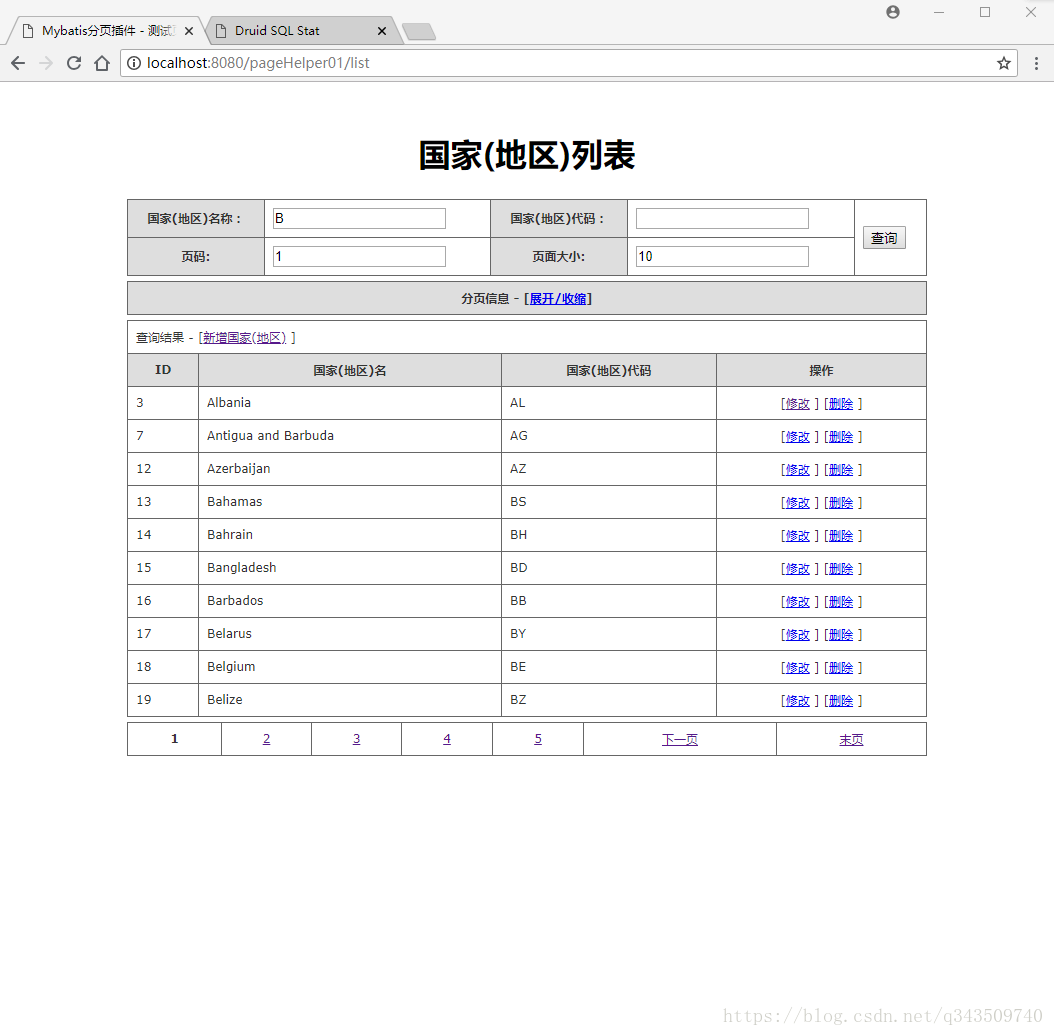
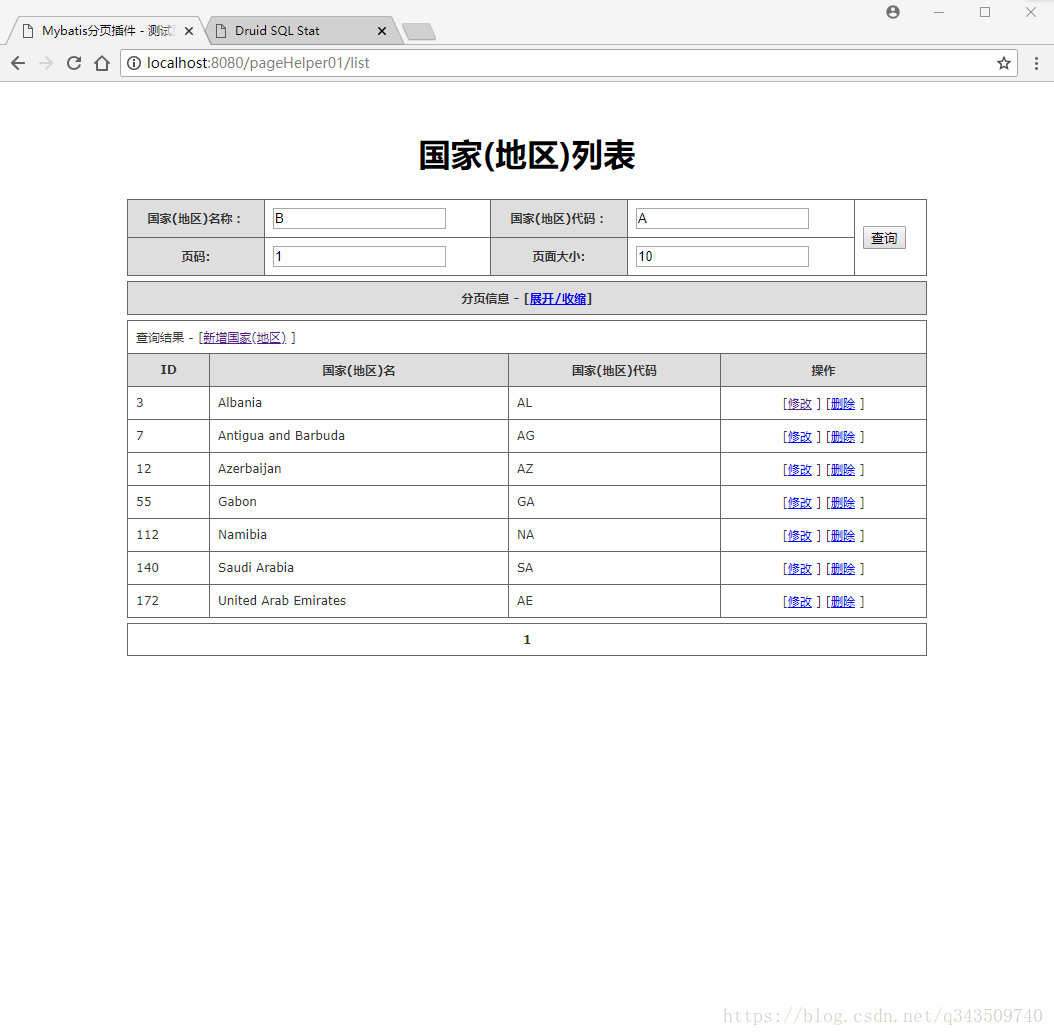