Python 装饰器
1. 简单的装饰器
下面是一个简单的装饰器示例,它记录被装饰函数的调用信息:
def my_decorator(func):
""" 中层函数:接收被装饰的函数 """
def wrapper():
""" 内层函数:执行具体功能 """
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
def my_decorator(func):
def wrapper(x):
print("Something is happening before the function is called.")
func(x)
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello(x):
print(f"Hello! {x}")
say_hello('xiao ming ')
# 这样更统一
def my_decorator(func):
def wrapper(*args, **kwargs):
print("Something is happening before the function is called.")
func(*args, **kwargs)
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello(x):
print(f"Hello! {x}")
say_hello('xiao ming ')
带参数直接在内层函数添加
2. 装饰器带参数
def repeat(num_times):
def my_decorator(func):
def wrapper(x):
for i in range(num_times):
print(f"Something is happening before the function is called.{i}")
func(x)
print(f"Something is happening after the function is called.{i}", end='\n\n')
return wrapper
return my_decorator
@repeat(3)
def say_hello(x):
print(f"Hello! {x}")
say_hello('xiao ming ')
问题:定义一个装饰器,可以计算函数的执行时间
import time
def times(num_times):
def decorated(func):
def wrapper(x):
times = 0
for _ in range(num_times):
start = time.time()
ret = func(x)
times += time.time() - start
print(f"Average execution time over {num_times} runs: {times:.6f} seconds")
times /= num_times
return ret
return wrapper
return decorated
@times(5)
def func(n):
cnt = 0
for i in range(1, n+1):
cnt += i
return cnt
func(1000000)
3. 类调用
class CountCalls:
def __init__(self, func):
self.func = func
self.num_calls = 0
def __call__(self, *args, **kwargs):
self.num_calls += 1
print(f"Call {self.num_calls} of {self.func.__name__}")
return self.func(*args, **kwargs)
@CountCalls
def say_hello():
print("Hello!")
say_hello()
say_hello()
return self.func(*args, **kwargs)
@CountCalls
def say_hello():
print(“Hello!”)
say_hello()
say_hello()
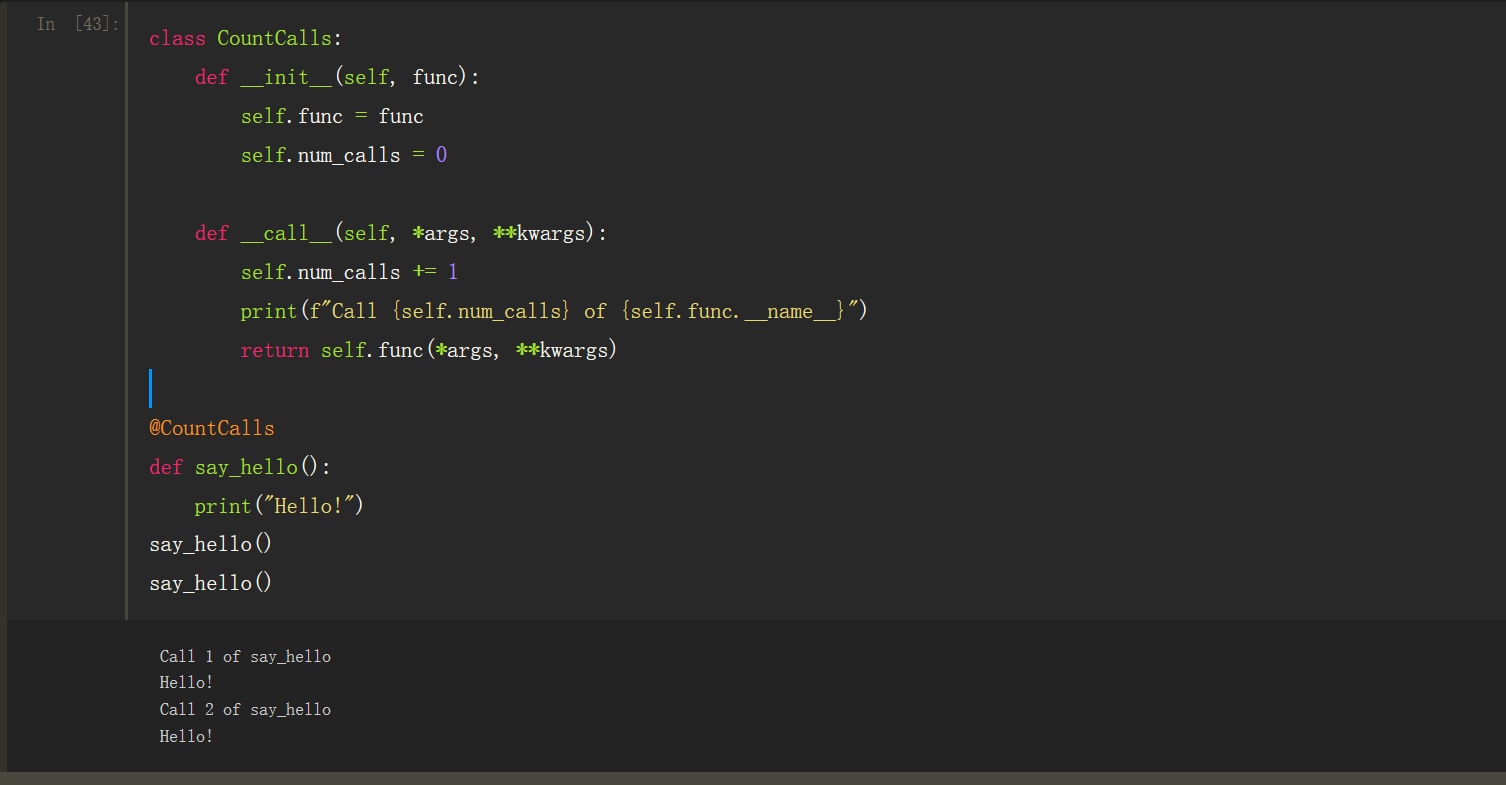