简单实例
主程序
package com.test.dukang.mysharedpreferrence;
import android.app.Activity;
import android.content.SharedPreferences;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends Activity implements View.OnClickListener{
private Button mButton_write;
private Button mButton_read;
private EditText mEditText;
private TextView mTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mButton_read= (Button) findViewById(R.id.button_read);
mButton_write= (Button) findViewById(R.id.button_write);
mEditText= (EditText) findViewById(R.id.editText);
mTextView= (TextView) findViewById(R.id.textView);
mButton_read.setOnClickListener(this);
mButton_write.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.button_read:
SharedPreferences sharedPreferences=getSharedPreferences("nihao",MODE_PRIVATE);
String content=sharedPreferences.getString("hao","keng");
mTextView.setText(content);
break;
case R.id.button_write:
write();
break;
}
}
private void write() {
SharedPreferences sharedPreferences=getSharedPreferences("nihao",MODE_PRIVATE);
SharedPreferences.Editor editor=sharedPreferences.edit();
editor.putString("hao",mEditText.getText().toString());
editor.commit();
}
}
布局文件
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:orientation="vertical">
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="nihao"/>
<Button
android:id="@+id/button_write"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="写入数据"/>
<Button
android:id="@+id/button_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取数据"/>
</LinearLayout>
效果图
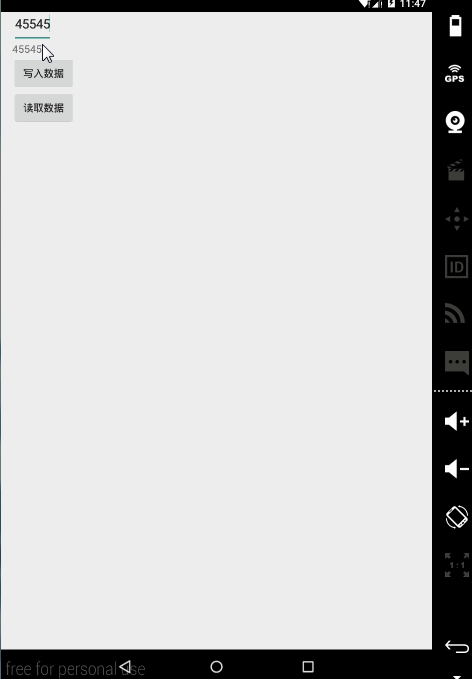
数据存储集锦
主程序
package com.test.dukang.mysharedpreferrence;
import android.app.Activity;
import android.content.SharedPreferences;
import android.os.Environment;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.util.Printer;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
public class MainActivity extends Activity implements View.OnClickListener {
private Button mButton_write;
private Button mButton_read;
private Button mButtonCacheWrite;
private Button mButtonCacheRead;
private Button mButtonCacheWriteDir;
private Button mButtonCacheReadDir;
private Button mButtonCacheWriteSd;
private Button mButtonCacheReadSd;
private EditText mEditText;
private TextView mTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mButton_read = (Button) findViewById(R.id.button_read);
mButton_write = (Button) findViewById(R.id.button_write);
mButtonCacheWrite = (Button) findViewById(R.id.button_cache_write);
mButtonCacheRead = (Button) findViewById(R.id.button_cache_read);
mButtonCacheWriteDir = (Button) findViewById(R.id.button_cache_write_dir);
mButtonCacheReadDir = (Button) findViewById(R.id.button_cache_read_dir);
mButtonCacheWriteSd = (Button) findViewById(R.id.button_cache_write_sd);
mButtonCacheReadSd = (Button) findViewById(R.id.button_cache_read_sd);
mEditText = (EditText) findViewById(R.id.editText);
mTextView = (TextView) findViewById(R.id.textView);
mButton_read.setOnClickListener(this);
mButton_write.setOnClickListener(this);
mButtonCacheWrite.setOnClickListener(this);
mButtonCacheRead.setOnClickListener(this);
mButtonCacheWriteDir.setOnClickListener(this);
mButtonCacheReadDir.setOnClickListener(this);
mButtonCacheWriteSd.setOnClickListener(this);
mButtonCacheReadSd.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.button_read:
SharedPreferences sharedPreferences = getPreferences(MODE_PRIVATE);
String content = sharedPreferences.getString("hao", "keng");
mTextView.setText(content);
break;
case R.id.button_write:
write();
break;
case R.id.button_cache_write:
FileOutputStream fileOutputStream = null;
try {
fileOutputStream = openFileOutput("data", MODE_PRIVATE);
PrintWriter printWriter = new PrintWriter(new OutputStreamWriter(fileOutputStream));
printWriter.write("你好");
printWriter.flush();
printWriter.close();
fileOutputStream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
break;
case R.id.button_cache_read:
try {
FileInputStream fis = openFileInput("data");
BufferedReader br = new BufferedReader(new InputStreamReader(fis));
String line = br.readLine();
while (line != null) {
Log.d("share", line);
line = br.readLine();
}
br.close();
fis.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
break;
case R.id.button_cache_write_dir:
writeDir();
break;
case R.id.button_cache_read_dir:
break;
case R.id.button_cache_write_sd:
File file1 = new File(Environment.getExternalStorageDirectory(), "nimei.txt");
if (!file1.exists()) {
try {
file1.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
FileOutputStream fos = null;
try {
fos = new FileOutputStream(file1);
PrintWriter printWriter = new PrintWriter(new OutputStreamWriter(fos));
printWriter.write("你好,你妹。");
printWriter.flush();
printWriter.close();
fos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
break;
case R.id.button_cache_read_sd:
break;
}
}
private void writeDir() {
File file = new File(getCacheDir(), "dukang");
if (!file.exists()) {
try {
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
FileOutputStream fos = null;
try {
fos = new FileOutputStream(file);
PrintWriter printWriter = new PrintWriter(new OutputStreamWriter(fos));
printWriter.write("你好");
printWriter.flush();
printWriter.close();
fos.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private void write() {
SharedPreferences sharedPreferences = getPreferences(MODE_PRIVATE);
;
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putString("hao", mEditText.getText().toString());
editor.commit();
}
}
布局文件
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:orientation="vertical">
<EditText
android:id="@+id/editText"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="nihao"/>
<Button
android:id="@+id/button_write"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="写入数据"/>
<Button
android:id="@+id/button_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读取数据"/>
<Button
android:id="@+id/button_cache_write"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="写缓存"/>
<Button
android:id="@+id/button_cache_read"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读缓存"/>
<Button
android:id="@+id/button_cache_write_dir"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="写cache缓存"/>
<Button
android:id="@+id/button_cache_read_dir"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读cache缓存"/>
<Button
android:id="@+id/button_cache_write_sd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="写sd缓存"/>
<Button
android:id="@+id/button_cache_read_sd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="读sd缓存"/>
</LinearLayout>