-
在exe路径的生成固定字节数据的二进制文件a.out,命令行输入:
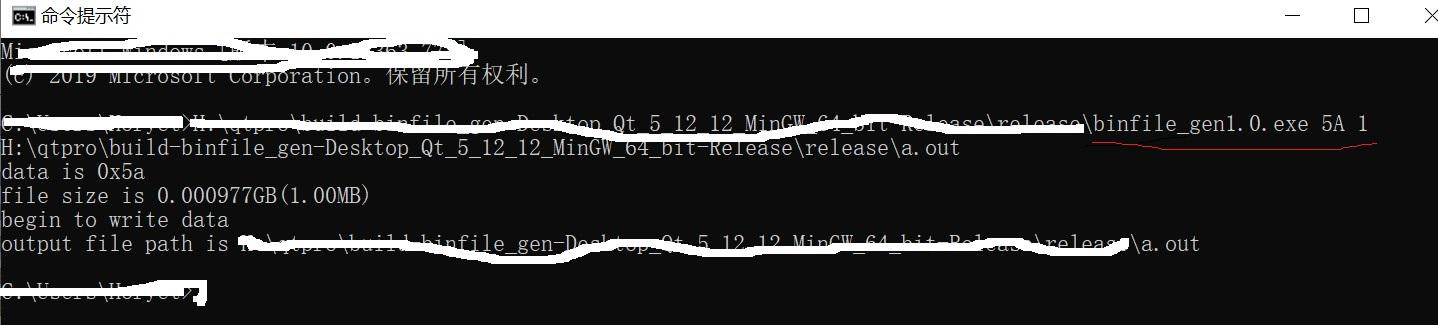
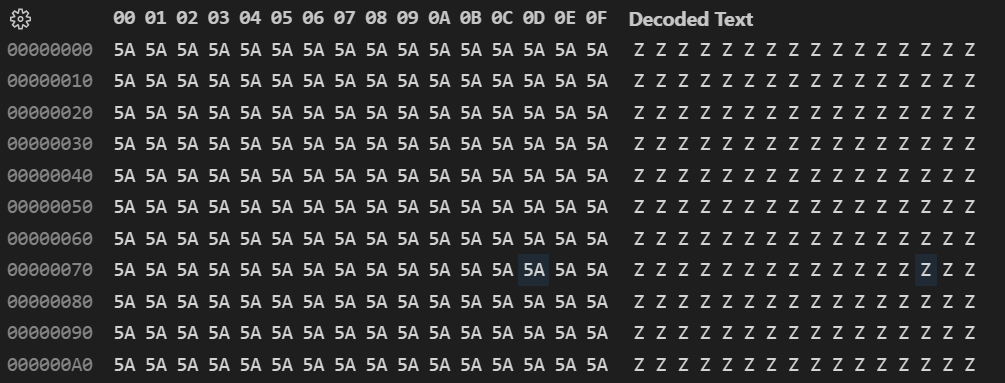
-
源代码:
//#include <QCoreApplication>
#include <iostream>
#include <fstream>
#include <string.h>
#include <direct.h>
#include <stdlib.h>
#include <stdio.h>
#include <iomanip>
#include <windows.h>
//#include <libloaderapi.h>
using namespace std;
char convertHexChart(char ch)
{
if((ch >= '0') && (ch <= '9'))
return ch-0x30; // 0x30 对应 ‘0’
else if((ch >= 'A') && (ch <= 'F'))
return ch-'A'+10;
else if((ch >= 'a') && (ch <= 'f'))
return ch-'a'+10;
else return ch-ch;//不在0-f范围内的会发送成0
}
//获取到cmd的当前路径
char* getcurpath(){
char* path;
path = getcwd(NULL,0);
// cout<<"path is "<<path<<endl;
if(path == NULL){
cout<<"get path error!"<<endl;
}
else{
}
return path;
}
//获取到exe所在路径
string getExepath(){
char szFilePath[MAX_PATH+1]={0};
GetModuleFileNameA(NULL,szFilePath,MAX_PATH);
(strrchr(szFilePath,'\\'))[0]=0;
string path = szFilePath;
return path;
}
int main(int argc,char *argv[]){ //char *argv[]指针数组
// cout<<argc<<endl;
uint64_t str;
uint64_t offset;
sscanf(*(argv+1),"%0llx",&str);
// cout<<strlen(argv[1])<<endl;
int byte_num=(strlen(argv[1])+1)/2;
printf("data is 0x%0llx\n",str);
// uint64_t data=stoll(*(argv+1));
if(argc<3){
cout<<"usage:gen_binfile.exe data(DEC) size(MB)"<<endl;
return 0;
}
else if(argc==4){
sscanf(*(argv+3),"%0llx",&offset);
}
string file_suffix=".bin";
string ExePath=argv[1]+file_suffix;
const char* fname = ExePath.data();
ofstream fout(fname,ios::binary);
long double size = stold(argv[2])*1024*1024; //size MByte to Byte
printf("file size is %0.6fGB(%0.2fMB)\n",stoi(argv[2])/1024.0,stof(argv[2])/1.0);
cout << "begin to write data" << endl;
cout<<"output file path is "<<fname<<endl;
cout<<"Please wait a minute ... "<<endl;
long long i=0;
while(i<size){
//for(int m=3;m>=0;m--){ //address
for(int m=0;m<=3;m++){ //address
uint8_t u8_data=i>>(m*8);
fout.write((char *)&u8_data,sizeof(uint8_t));
}
i+=4;
for(int j=0;j<=3;j++){ //data
uint8_t u8_data=str>>(j*8);
fout.write((char *)&u8_data,sizeof(uint8_t));
i++;
}
// for(int j=byte_num-1;j>=0;j--){ //data
}
cout<<"write data down "<<endl;
/***************check file structure*******************/
uint8_t *recvarr=(uint8_t*) malloc(16*sizeof(uint8_t));
FILE *rdFile;
if((rdFile=fopen(fname,"rb"))==NULL){
printf("read bin file error");
}
//rewind(rdFile);
fseek(rdFile,offset,SEEK_SET);
fread(recvarr,sizeof(uint8_t),16,rdFile);
printf("print the data of address:\n%dMB:",offset/1024/1024);
printf("offset %08x:",offset);
for(int i=0;i<16;i++){
printf("%02x ",*(recvarr+i));
}
printf("\n");
return 0;
}
-
在写入过程中加入代码进度条会很炫酷,但是会让程序异常的慢。。。(不推荐使用)。
static const char *ponit[]={"\x20", "\xA8\x87", "\xA8\x86", "\xA8\x84", "\xA8\x83", "\xA8\x80"}; ▏▎▍▊█
//int per 百分比范围(0 ~ 100)
void progress_bar(int per,int whole)
{
int i=0;
int num0=0;
printf("\r [");
//空格占多少
num0 = (whole-per)/(whole/20);
while(per>(whole/20)) //完整单元格
{
printf("%s", ponit[5]);
per -= whole/20;
}
if((per)&&(per/(whole/20/5))) //不完整的单元格
{
printf("%s", ponit[i]);
}
for(i=0;i<num0;i++) //填充空格
{
printf("%s", ponit[0]);
}
printf("] %2d%% %d %d", per/whole,per,whole);
if(per == whole)
{
printf("\n");
}
}
void DoProgress(int t, int n) {
putchar('[');
for (int i = 0; i < n; i++) {
putchar(i < t ? '>' : ' '); // 输出> 或者 ' '
}
putchar(']');
printf("%3d%%",(int)((double(t)/n) *100));
// 光标回退,实现刷新
for (int i = 0; i != n + 6; i++) {
putchar('\b');
}
}