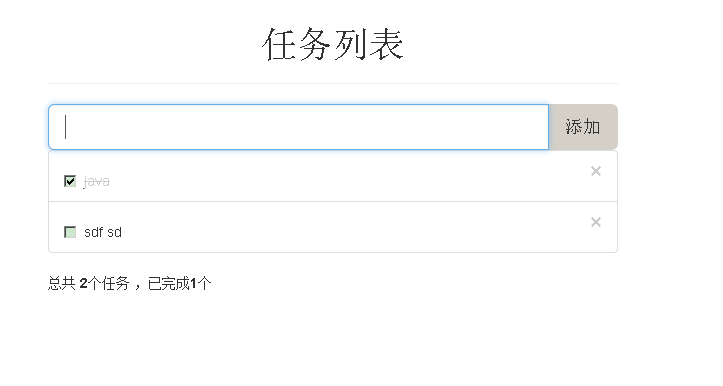
<!DOCTYPE html>
<html ng-app="task-app">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>angualJS</title>
<link rel="stylesheet" href="./bootstrap/css/bootstrap.min.css">
<style type="text/css" media="screen">
body{
max-width: 600px;
}
.checkbox{
margin-bottom: 0;
margin-right: 20px;
}
.done{
color: #ccc;
}
.done > .checkbox>label>span{
text-decoration: line-through;
}
</style>
</head>
<body class="container text-center">
<div class="page-header">
<h1>任务列表</h1>
</div>
<section ng-controller="myControl">
<form class="input-group input-group-lg">
<input class="form-control" ng-model="text" />
<span class="input-group-btn">
<button type="button" class="btn btn-secondary" ng-click="addTask()">添加</button>
</span>
</form>
<ul class="list-group text-left">
<li class="list-group-item" ng-class="{'done':item.done}" ng-repeat ="item in taskList" >
<button type="button" class="close" aria-label="Close" ng-click="delete()"><span aria-hidden="true">×</span></button>
<div class="checkbox">
<label>
<input type="checkbox" ng-model="item.done">
<span>{{item.text}} </span>
</label>
</div>
</li>
</ul>
<p class="text-left">
总共 <strong>{{taskList.length}}</strong>个任务
,已完成<strong>{{doneCount()}}</strong>个
</p>
</section>
<script src="./js/angular.min.js"></script>
<script src="./js/index.js"></script>
</body>
</html>
(function() {
window.angular.module('task-app', []);
window.angular.module('task-app').
controller('myControl', ['$scope', function($scope) {
$scope.text = '';
$scope.taskList = [{
text: 'java',
done: false
}];
$scope.addTask = function() {
var text = $scope.text.trim();
if (text) {
$scope.taskList.push({
text: text,
done: false
});
$scope.text = '';
}
};
$scope.delete = function(todo) {
var index = $scope.taskList.indexOf(todo);
$scope.taskList.splice(index, 1);
}
$scope.doneCount = function() {
var temp = $scope.taskList.filter(function(item) {
return item.done;
})
return temp.length;
}
}]);
})(window)