Container_of在Linux内核中是一个常用的宏,用于从包含在某个结构中的指针获得结构本身的指针,通俗地讲就是通过结构体变量中某个成员的首地址进而获得整个结构体变量的首地址。
Container_of的定义如下:
- #define container_of(ptr, type, member) ({ \
- const typeof( ((type *)0)->member ) *__mptr = (ptr); \
- (type *)( (char *)__mptr - offsetof(type,member) );})
- const typeof( ((type *)0)->member ) *__mptr = (ptr); \
创建一个类型为const typeof( ((type *)0)->member ) *,即类型为type结构的member域所对应的对象类型的常指针__mptr,并用ptr初始化之,这样一来,__mptr就指向了某一个type的member域。因为数据结构是顺序存储的,此时如果知道member在type结构中的相对偏移,那么用__mptr减去此偏移便是ptr所属的type的地址,因此宏的第二条语句应运而生:
- (type *)( (char *)__mptr - offsetof(type,member) );})
另一个主角出场了---offsetof,返回一个数据域在它所属的数据结构中的相对偏移,单位是size_t,宏定义如下:
- #define offsetof(TYPE, MEMBER) ((size_t) &((TYPE *)0)->MEMBER)
将地址0强制转换为type *,那么0指向某一个type类型的对象,也就是此type对象的地址,那么(TYPE *)0)->MEMBER就是type的member域,现在取址后&((TYPE *)0)->MEMBER 就是member域的地址,因为type的地址为0,则member的地址实质上就是它相对于type地址的偏移。这里为什么可以这样实现而不会出错呢?有两点原因,其一,地址0是在编译器编译时已经指定好了的,其二,这里全部都是取址操作,并没内存数据访问,因此不会存在非法访问内存的问题。
这样贯穿起来看,其实container_of的实现其实也是蛮清晰的,记得某位兄台说过一句话:一切事物在你看清楚它的本质之前,对你而言总是朦胧的。
其实它的语法很简单,只是一些指针的灵活应用,它分两步:
第一步,首先定义一个临时的数据类型(通过typeof( ((type *)0)->member )获得)与ptr相同的指针变量__mptr,然后用它来保存ptr的值。
第二步,用(char *)__mptr减去member在结构体中的偏移量,得到的值就是整个结构体变量的首地址(整个宏的返回值就是这个首地址)。
其中的语法难点就是如何得出成员相对结构体的偏移量?
通过例子说明,如清单1:
-
- #define __compiler_offsetof(a,b) __builtin_offsetof(a,b)
-
-
- #undef offsetof
- #ifdef __compiler_offsetof
- #define offsetof(TYPE,MEMBER) __compiler_offsetof(TYPE,MEMBER)
- #else
- #define offsetof(TYPE, MEMBER) ((size_t) &((TYPE *)0)->MEMBER)
- #endif
-
- #include <stdio.h>
-
- struct test_struct {
- int num;
- char ch;
- float fl;
- };
-
- int main(void)
- {
- printf("offsetof(struct test_struct, num) = %d\n",
- offsetof(struct test_struct, num));
-
- printf("offsetof(struct test_struct, ch) = %d\n",
- offsetof(struct test_struct, ch));
-
- printf("offsetof(struct test_struct, fl) = %d\n",
- offsetof(struct test_struct, fl));
-
- return 0;
- }
说明,__builtin_offsetof(a,b)是GCC的内置函数,可认为它的实现与((size_t) &((TYPE *)0)->MEMBER)这段代码是一致的。
例子输出结果:
- offsetof(struct test_struct, num) = 0
- offsetof(struct test_struct, ch) = 4
- offsetof(struct test_struct, fl) = 8
其中代码难以理解的地方就是它灵活地运用了0地址。如果觉得&( (struct test_struct *)0 )->ch这样的代码不好理解,那么我们可以假设在0地址分配了一个结构体变量struct test_struct a,然后定义结构体指针变量p并指向a(struct test_struct *p = &a),如此我们就可以通过&p->ch获得成员ch的地址。由于a的首地址为0x0,所以成员ch的首地址为0x4。
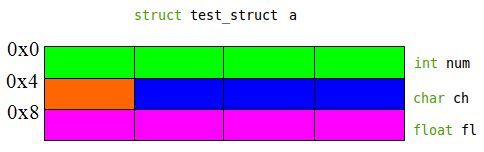
最后通过强制类型转换(size_t)把一个地址值转换为一个整数。
分析完container_of的定义,接下来举两个例子来体会一下它的使用方法。
正确的例子,如清单2:
-
- #define __compiler_offsetof(a,b) __builtin_offsetof(a,b)
-
-
- #undef offsetof
- #ifdef __compiler_offsetof
- #define offsetof(TYPE,MEMBER) __compiler_offsetof(TYPE,MEMBER)
- #else
- #define offsetof(TYPE, MEMBER) ((size_t) &((TYPE *)0)->MEMBER)
- #endif
-
-
-
-
-
-
-
-
- #define container_of(ptr, type, member) ({ \
- const typeof( ((type *)0)->member ) *__mptr = (ptr); \
- (type *)( (char *)__mptr - offsetof(type,member) );})
-
- #include <stdio.h>
-
- struct test_struct {
- int num;
- char ch;
- float fl;
- };
-
- int main(void)
- {
- struct test_struct init_test_struct = { 99, 'C', 59.12 };
-
- char *char_ptr = &init_test_struct.ch;
-
- struct test_struct *test_struct = container_of(char_ptr, struct test_struct, ch);
-
- printf(" test_struct->num = %d\n test_struct->ch = %c\n test_struct->fl = %f\n",
- test_struct->num, test_struct->ch, test_struct->fl);
-
- return 0;
- }
例子输出结果:
- test_struct->num = 99
- test_struct->ch = C
- test_struct->fl = 59.119999
不适当的例子,如清单3:
-
- #define __compiler_offsetof(a,b) __builtin_offsetof(a,b)
-
-
- #undef offsetof
- #ifdef __compiler_offsetof
- #define offsetof(TYPE,MEMBER) __compiler_offsetof(TYPE,MEMBER)
- #else
- #define offsetof(TYPE, MEMBER) ((size_t) &((TYPE *)0)->MEMBER)
- #endif
-
-
-
-
-
-
-
-
- #define container_of(ptr, type, member) ({ \
- const typeof( ((type *)0)->member ) *__mptr = (ptr); \
- (type *)( (char *)__mptr - offsetof(type,member) );})
-
- #include <stdio.h>
-
- struct test_struct {
- int num;
- char ch;
- float fl;
- };
-
- int main(void)
- {
- char real_ch = 'A';
- char *char_ptr = &real_ch;
-
- struct test_struct *test_struct = container_of(char_ptr, struct test_struct, ch);
-
- printf(" char_ptr = %p test_struct = %p\n\n", char_ptr, test_struct);
-
- printf(" test_struct->num = %d\n test_struct->ch = %c\n test_struct->fl = %f\n",
- test_struct->num, test_struct->ch, test_struct->fl);
-
- return 0;
- }
例子输出结果:
- char_ptr = 0xbfb72d7f test_struct = 0xbfb72d7b
-
- test_struct->num = -1511000897
- test_struct->ch = A
- test_struct->fl = 0.000000
注意,由于这里并没有一个具体的结构体变量,所以成员num和fl的值是不确定的。