1、Find All Anagrams in a String
Given a string s and a non-empty string p, find all the start indices of p's anagrams in s.
Strings consists of lowercase English letters only and the length of both strings s and p will not be larger than 20,100.
The order of output does not matter.
Example 1:
Input: s: "cbaebabacd" p: "abc" Output: [0, 6] Explanation: The substring with start index = 0 is "cba", which is an anagram of "abc". The substring with start index = 6 is "bac", which is an anagram of "abc".
Example 2:
Input: s: "abab" p: "ab" Output: [0, 1, 2] Explanation: The substring with start index = 0 is "ab", which is an anagram of "ab". The substring with start index = 1 is "ba", which is an anagram of "ab". The substring with start index = 2 is "ab", which is an anagram of "ab".
找出字符串中,字串与p字符分布相同,但是顺序可以不同的字串的开始下标,核心思想是使用滑动窗口
2、Find All Numbers Disappeared in an Array
Given an array of integers where 1 ≤ a[i] ≤ n (n = size of array), some elements appear twice and others appear once.
Find all the elements of [1, n] inclusive that do not appear in this array.
Could you do it without extra space and in O(n) runtime? You may assume the returned list does not count as extra space.
Example:
Input: [4,3,2,7,8,2,3,1] Output: [5,6]
找出数组中缺失的数字,不使用外部的存储空间,数组中值的范围在长度以内
注意看这题数组元素的值全都在数组索引的范围内,那么肯定就是套用那个经典的给元素值赋正负来表达额外信息的套路了。而这题就是遍历每个元素,把与元素值相等(实际差个1)的那个索引对应的元素赋值为负数,然后遍历一遍完成后,再次遍历,如果某个索引对应的元素值是正数,就说明与这个索引相同的值在数组中不存在,就是结果了。
拿[4,3,2,7,8,2,3,1]
演示一遍过程:
[ 4, 3, 2, 7, 8, 2, 3, 1] -> [ 4, 3, 2, -7, 8, 2, 3, 1]
[ 4, 3, 2, -7, 8, 2, 3, 1] -> [ 4, 3, -2, -7, 8, 2, 3, 1]
[ 4, 3, -2, -7, 8, 2, 3, 1] -> [ 4, -3, -2, -7, 8, 2, 3, 1]
[ 4, -3, -2, -7, 8, 2, 3, 1] -> [ 4, -3, -2, -7, 8, 2, -3, 1]
[ 4, -3, -2, -7, 8, 2, -3, 1] -> [ 4, -3, -2, -7, 8, 2, -3, -1]
[ 4, -3, -2, -7, 8, 2, -3, 1] -> [ 4, -3, -2, -7, 8, 2, -3, -1]
[ 4, -3, -2, -7, 8, 2, -3, 1] -> [ 4, -3, -2, -7, 8, 2, -3, -1]
[ 4, -3, -2, -7, 8, 2, -3, 1] -> [-4, -3, -2, -7, 8, 2, -3, -1]
output: [5, 6]
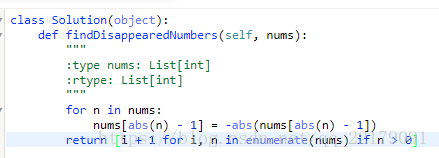
3、Hamming Distance
The Hamming distance between two integers is the number of positions at which the corresponding bits are different.
Given two integers x
and y
, calculate the Hamming distance.
Note:
0 ≤ x
, y
< 231.
Example:
Input: x = 1, y = 4 Output: 2 Explanation: 1 (0 0 0 1) 4 (0 1 0 0) ↑ ↑ The above arrows point to positions where the corresponding bits are different.
4、