前言
首先,数据结构这个东西并不针对某一种编程语言,但是很多初学者在学习过c语言后先接触的是有关c语言实现的基础数据结构(例如链表,栈,队列等),后面学会了C++之后反而有些束手束脚,用C++的类去实现链栈时反而造成了内存泄漏。
这是因为,我们知道,如果在类内创建对象,那么他在主函数结束后就会自动调用类的析构函数(没写的话会调用默认析构函数),所以有同学就会以为在类中写链式结构时不需要delete或者free,这是错误的想法,因为类的析构只是释放类的内部空间,对于new和malloc所建立的堆区空间仍旧需要调用delete或free。
好的那么废话就不再多说了,我这篇文章已经把源码放到文章末尾了,有需要的直接拿走不谢!当然作者很希望各位看官能给本人点个小赞,谢谢!
Indent:订单类
那么首先是基类订单类,有订单编号account,餐品名称food_name,下一级next,然后我去掉了拷贝构造和移动构造函数,不允许赋值和移动赋值,在基类中添加一个printindent输出函数,输出订单信息。
class Indent {
public:
int account;
string food_name;
Indent* next;
Indent(int a, string fn, Indent* n = nullptr) :account(a), food_name(fn), next(n) { cout << "Creat a indent" << endl; }
~Indent() { cout << "Delete a indent" << endl; }
Indent(Indent& x) = delete;
Indent(Indent&& x) = delete;
Indent operator=(Indent& x) = delete;
Indent operator=(Indent&& x) = delete;
void printindent() const { cout << "订单号:" << account << " 餐品名称:" << food_name << endl; }
};
Restaurant:餐厅类
按照队列结构,设计队头head指针,队尾tail指针,num为订单数量。
push函数即入队操作,因为在构造函数里我默认队列为空,因此在入队第一个节点时,使队头队尾都指向它;当队列不为空后,从队尾入队,队尾指针自动向下一级移动。
Pop函数即出队操作,在确认队列不为空的条件下,从队头出队并打印输出订单信息。这里要注意,如果队列中只剩下一个节点时,务必要将head和tail变成nullptr,否则会出现空悬指针问题。
class Restaurant {
private:
Indent* head;
Indent* tail;
int num;
public:
Restaurant() :head(nullptr), tail(nullptr), num(0) { cout << "Creat a restaurant" << endl; }
~Restaurant() { cout << "Delete a restaurant" << endl; }
Restaurant(Restaurant& x) = delete;
Restaurant(Restaurant&& x) = delete;
Restaurant operator=(Restaurant& x) = delete;
Restaurant operator=(Restaurant&& x) = delete;
void printRestaurant() const {
Indent* p = head;
while (p != nullptr) {
p->printindent();
p = p->next;
}
}
bool push(int account, string food_name) {
Indent* t = new Indent(account, food_name);
if (head == nullptr && tail == nullptr) {
head = tail = t;
}
else {
tail->next = t;
tail = tail->next;
}
num++;
return true;
}
bool Pop() {
if (head == nullptr) {
return false;
}
if (head != tail) {
Indent* p = head;
p->printindent();
head = head->next;
delete p;
num--;
}
else {
head->printindent();
delete head;
head = tail = nullptr;
num = 0;
}
return true;
}
bool Delete_Restaurant() {
while (num != 0) {
Pop();
}
return true;
}
};
main:测试代码
int main() {
Restaurant 楞敲小青年的餐厅;
楞敲小青年的餐厅.push(1, "香辣鸡腿堡");
楞敲小青年的餐厅.push(2, "香辣鸡肉卷");
楞敲小青年的餐厅.push(3, "香辣鸡翅");
楞敲小青年的餐厅.printRestaurant();
楞敲小青年的餐厅.Pop();
楞敲小青年的餐厅.printRestaurant();
楞敲小青年的餐厅.Delete_Restaurant();
return 0;
}
运行结果
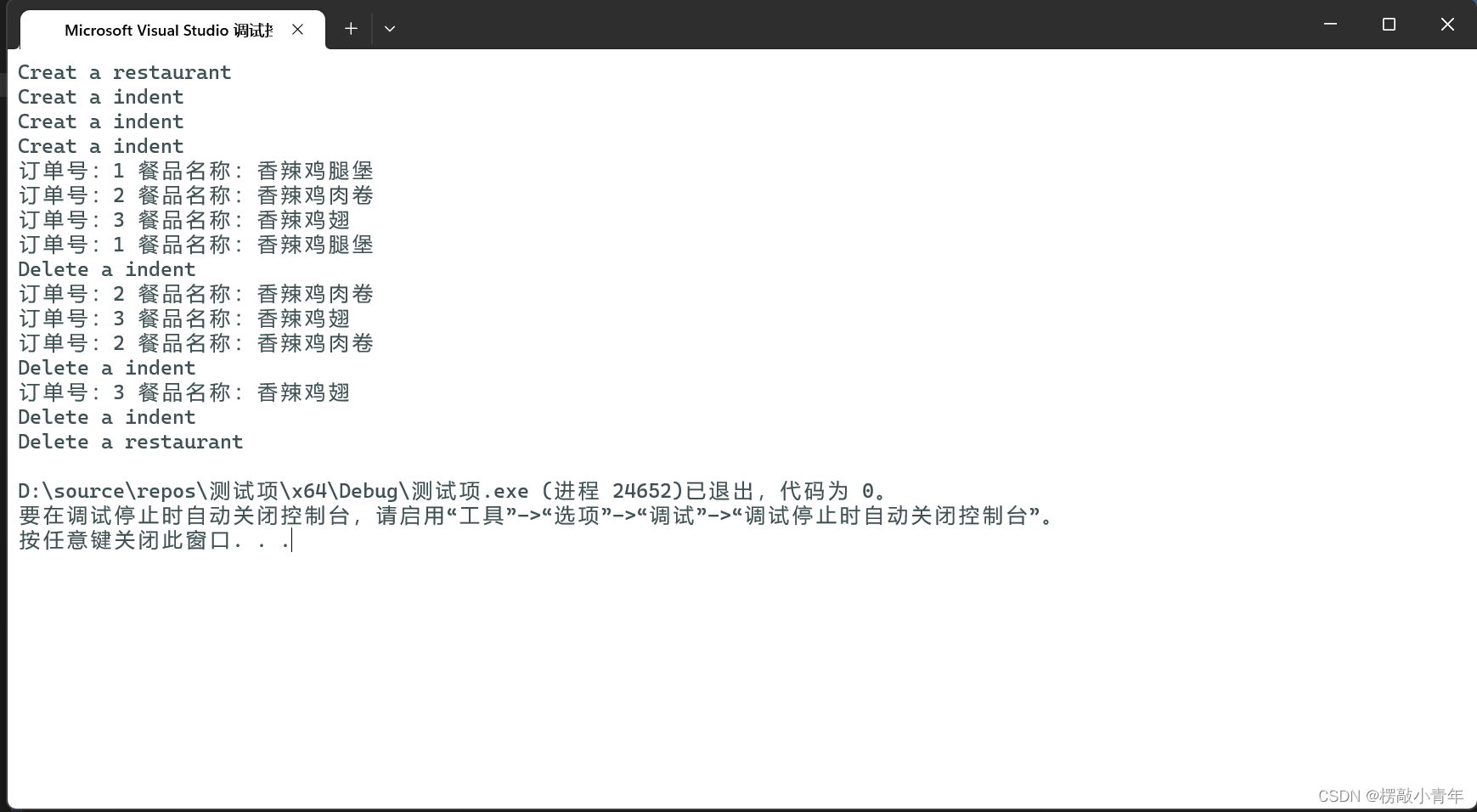
源代码
#include<iostream>
#include<string>
using namespace std;
class Indent {
public:
int account;
string food_name;
Indent* next;
Indent(int a, string fn, Indent* n = nullptr) :account(a), food_name(fn), next(n) { cout << "Creat a indent" << endl; }
~Indent() { cout << "Delete a indent" << endl; }
Indent(Indent& x) = delete;
Indent(Indent&& x) = delete;
Indent operator=(Indent& x) = delete;
Indent operator=(Indent&& x) = delete;
void printindent() const { cout << "订单号:" << account << " 餐品名称:" << food_name << endl; }
};
class Restaurant {
private:
Indent* head;
Indent* tail;
int num;
public:
Restaurant() :head(nullptr), tail(nullptr), num(0) { cout << "Creat a restaurant" << endl; }
~Restaurant() { cout << "Delete a restaurant" << endl; }
Restaurant(Restaurant& x) = delete;
Restaurant(Restaurant&& x) = delete;
Restaurant operator=(Restaurant& x) = delete;
Restaurant operator=(Restaurant&& x) = delete;
void printRestaurant() const {
Indent* p = head;
while (p != nullptr) {
p->printindent();
p = p->next;
}
}
bool push(int account, string food_name) {
Indent* t = new Indent(account, food_name);
if (head == nullptr && tail == nullptr) {
head = tail = t;
}
else {
tail->next = t;
tail = tail->next;
}
num++;
return true;
}
bool Pop() {
if (head == nullptr) {
return false;
}
if (head != tail) {
Indent* p = head;
p->printindent();
head = head->next;
delete p;
num--;
}
else {
head->printindent();
delete head;
head = tail = nullptr;
num = 0;
}
return true;
}
bool Delete_Restaurant() {
while (num != 0) {
Pop();
}
return true;
}
};
int main() {
Restaurant 楞敲小青年的餐厅;
楞敲小青年的餐厅.push(1, "香辣鸡腿堡");
楞敲小青年的餐厅.push(2, "香辣鸡肉卷");
楞敲小青年的餐厅.push(3, "香辣鸡翅");
楞敲小青年的餐厅.printRestaurant();
楞敲小青年的餐厅.Pop();
楞敲小青年的餐厅.printRestaurant();
楞敲小青年的餐厅.Delete_Restaurant();
return 0;
}