/**
* 获取客户端ip地址(可以穿透代理)
*
* @param request
* @return
*/
public static String getRemoteAddr(HttpServletRequest request) {
String ip = request.getHeader("X-Forwarded-For");
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
return ip;
}
private static final String[] HEADERS_TO_TRY = {
"X-Forwarded-For",
"Proxy-Client-IP",
"WL-Proxy-Client-IP",
"HTTP_X_FORWARDED_FOR",
"HTTP_X_FORWARDED",
"HTTP_X_CLUSTER_CLIENT_IP",
"HTTP_CLIENT_IP",
"HTTP_FORWARDED_FOR",
"HTTP_FORWARDED",
"HTTP_VIA",
"REMOTE_ADDR",
"X-Real-IP"};
/***
* 获取客户端ip地址(可以穿透代理)
* @param request
* @return
*/
public static String getClientIpAddress(HttpServletRequest request) {
for (String header : HEADERS_TO_TRY) {
String ip = request.getHeader(header);
if (ip != null && ip.length() != 0 && !"unknown".equalsIgnoreCase(ip)) {
return ip;
}
}
return request.getRemoteAddr();
}
/***
* 获取客户端ip地址(可以穿透代理)
* @param request
* @return
*/
public static String getClientIpAddr(HttpServletRequest request) {
String ip = request.getHeader("X-Forwarded-For");
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_FORWARDED");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_CLUSTER_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_FORWARDED");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_VIA");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("REMOTE_ADDR");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
return ip;
}
public static String getIpAddr(HttpServletRequest request) {
String ip = request.getHeader("X-Real-IP");
if (null != ip && !"".equals(ip.trim())
&& !"unknown".equalsIgnoreCase(ip)) {
return ip;
}
ip = request.getHeader("X-Forwarded-For");
if (null != ip && !"".equals(ip.trim())
&& !"unknown".equalsIgnoreCase(ip)) {
int index = ip.indexOf(',');
if (index != -1) {
return ip.substring(0, index);
} else {
return ip;
}
}
return request.getRemoteAddr();
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
我使用的是spring MVC框架,测试的控制器代码如下:
package com.web.controller
import java.util.HashMap
import java.util.Map
import javax.servlet.http.HttpServletRequest
import org.springframework.stereotype.Controller
import org.springframework.web.bind.annotation.RequestMapping
import org.springframework.web.bind.annotation.ResponseBody
import com.common.util.SystemHWUtil
import com.common.util.WebServletUtil
import com.string.widget.util.ValueWidget
import com.util.JSONPUtil
@Controller
@RequestMapping("/cors")
public class CORSController {
@ResponseBody
@RequestMapping(value = "/simple",produces=SystemHWUtil.RESPONSE_CONTENTTYPE_JSON_UTF)
public String corsJsonSimple(HttpServletRequest request,String callback){
String content
Map map=new HashMap()
map.put("username", "黄威")
map.put("age", "27")
map.put("address", "beijing")
content=JSONPUtil.getJsonP(map, callback)
System.out.println("getIpAddr:"+WebServletUtil.getIpAddr(request))
System.out.println("getRemoteAddr:"+WebServletUtil.getRemoteAddr(request))
System.out.println("getClientIpAddr:"+WebServletUtil.getClientIpAddr(request))
System.out.println("getClientIpAddress:"+WebServletUtil.getClientIpAddress(request))
return content
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
测试结果:
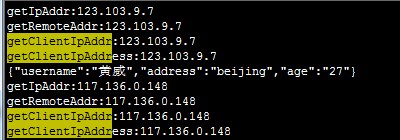
获取登录客户端ip和mac
-
-
-
-
-
-
- public String getIpAddr(HttpServletRequest request) throws Exception {
- String ip = request.getHeader("x-forwarded-for");
- if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
- ip = request.getHeader("Proxy-Client-IP");
- }
- if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
- ip = request.getHeader("WL-Proxy-Client-IP");
- }
- if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
- ip = request.getHeader("HTTP_CLIENT_IP");
- }
- if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
- ip = request.getHeader("HTTP_X_FORWARDED_FOR");
- }
- if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
- ip = request.getRemoteAddr();
- }
- return ip;
- }
-
-
-
-
-
-
- public String getMACAddress(String ip) throws Exception {
- String line = "";
- String macAddress = "";
- final String MAC_ADDRESS_PREFIX = "MAC Address = ";
- final String LOOPBACK_ADDRESS = "127.0.0.1";
-
- if (LOOPBACK_ADDRESS.equals(ip)) {
- InetAddress inetAddress = InetAddress.getLocalHost();
-
- byte[] mac = NetworkInterface.getByInetAddress(inetAddress).getHardwareAddress();
-
- StringBuilder sb = new StringBuilder();
- for (int i = 0; i < mac.length; i++) {
- if (i != 0) {
- sb.append("-");
- }
-
- String s = Integer.toHexString(mac[i] & 0xFF);
- sb.append(s.length() == 1 ? 0 + s : s);
- }
-
- macAddress = sb.toString().trim().toUpperCase();
- return macAddress;
- }
-
- try {
- Process p = Runtime.getRuntime().exec("nbtstat -A " + ip);
- InputStreamReader isr = new InputStreamReader(p.getInputStream());
- BufferedReader br = new BufferedReader(isr);
- while ((line = br.readLine()) != null) {
- if (line != null) {
- int index = line.indexOf(MAC_ADDRESS_PREFIX);
- if (index != -1) {
- macAddress = line.substring(index + MAC_ADDRESS_PREFIX.length()).trim().toUpperCase();
- }
- }
- }
- br.close();
- } catch (IOException e) {
- e.printStackTrace(System.out);
- }
- return macAddress;
- }