实现方式一:
#ifndef BSTREE_H_INCLUDED
#define BSTREE_H_INCLUDED
#include <iostream>
#include <deque>
#include <stdio.h>
using namespace std;
class BSTNode
{
public:
int key;
BSTNode* left;
BSTNode* right;
BSTNode* parent;
/*构造方法*/
BSTNode():parent(NULL){}
BSTNode(int key,BSTNode* left,BSTNode* right,BSTNode* parent):key(key),left(left),right(right),parent(parent){}
};
class BSTree
{
private:
BSTNode* root;
public:
/*构造函数*/
BSTree():root(NULL){}
/*返回根结点*/
BSTNode* getRoot(){return root;}
/*插入键值为key的结点*/
void insert(int key);
/*插入结点node*/
void insert(BSTNode* node,BSTNode*& root);
/*前序遍历*/
void preOrder(BSTNode* root);
/*中续遍历*/
void inOrder(BSTNode* root);
/*后续遍历*/
void postOrder(BSTNode* root);
/*广度遍历*/
void printFromTopToBottom(BSTNode* root);
/*查找某个键值key*/
BSTNode* search(int k,BSTNode* root);
/*获得最小结点*/
BSTNode* minimum(BSTNode* root);
/*获得最大结点*/
BSTNode* maximum(BSTNode* root);
/*查找前驱结点*/
BSTNode* precessor(int key,BSTNode* root);
/*查找后继结点*/
BSTNode* successor(int key,BSTNode* root);
/*删除结点*/
BSTNode* remove(int key,BSTNode*& root);
};
void BSTree::insert(int key)
{
BSTNode* node = new BSTNode(key,NULL,NULL,NULL);
insert(node,root);
}
void BSTree::insert(BSTNode* node,BSTNode*& root)
{
BSTNode* x = root;
BSTNode* y = NULL;
/*查找插入位置*/
while(x != NULL)
{
y = x;
if(x->key > node->key)
{
x = x->left;
}
else
{
x = x->right;
}
}
/*插入*/
node->parent = y;
if(y == NULL)
{
root = node;
}
else if(y->key > node->key)
{
y->left = node;
}
else
{
y->right = node;
}
}
void BSTree::preOrder(BSTNode* root)
{
if(root!=NULL)
{
cout<<root->key;
preOrder(root->left);
preOrder(root->right);
}
}
void BSTree::inOrder(BSTNode* root)
{
if(root != NULL)
{
inOrder(root->left);
cout<<root->key;
inOrder(root->right);
}
}
void BSTree::postOrder(BSTNode* root)
{
if(root != NULL)
{
postOrder(root->left);
postOrder(root->right);
cout<<root->key;
}
}
void BSTree::printFromTopToBottom(BSTNode* root)
{
if(root == NULL)
return ;
deque<BSTNode* > dequeTree;
dequeTree.push_back(root);
// BSTNode* pnode = dequeTree.front();
// cout<<dequeTree.size()<<"对应的数据是:"<<pnode->key;
while(dequeTree.size())
{
// cout<<dequeTree.size()<<endl;
BSTNode* pnode = dequeTree.front();
dequeTree.pop_front();
cout<<pnode->key<<" ";
// printf("%d ",pnode->key);
if(pnode->left)
{
dequeTree.push_back(pnode->left);
}
if(pnode->right)
{
dequeTree.push_back(pnode->right);
}
}
}
BSTNode* BSTree::search(int key,BSTNode* root)
{
if(root == NULL || key == root->key)
{
return root;
}
else if(key < root->key)
{
search(key,root->left);
}
else
{
search(key,root->right);
}
}
BSTNode* BSTree::minimum(BSTNode* root)
{
BSTNode* pnode = root;
while(root)
{
pnode = root;
root = root->left;
}
if(pnode)
{
return pnode;
}
}
BSTNode* BSTree::maximum(BSTNode* root)
{
if(root->right == NULL)
{
return root;
}
maximum(root->right);
}
BSTNode* BSTree::precessor(int key,BSTNode* root)
{
BSTNode* find = search(key,root);
if(find == NULL)
{
return find;
}
/*1.如果结点存在左子树,那么左孩子中最大的结点即为其前驱结点*/
if(find->left != NULL)
{
return maximum(find->left);
}
/*2.如果结点不存在左子树*/
/* 2.1如果是右孩子,那么它的父结点即为其前驱结点*/
if(find == find->parent->right)
{
return find->parent;
}
/* 2.2如果是左孩子,那么它的父结点中第一个成为其左孩子结点的父节点即为前驱结点*/
else
{
while(find->parent != NULL && find->parent->left == find)
{
find = find->parent;
}
return find->parent;
}
}
BSTNode* BSTree::successor(int key,BSTNode* root)
{
BSTNode* find = search(key,root);
if(find == NULL)
{
return find;
}
/*1.如果有右子树*/
if(find->right != NULL)
{
return minimum(find->right);
}
/*2.如果没有右子树*/
/* 2.1如果是左孩子*/
if(find->parent->left == find)
{
return find->parent;
}
/* 2.2如果是右孩子*/
else
{
while(find->parent != NULL && find->parent->right == find)
{
find = find->parent;
}
return find->parent;
}
}
BSTNode* BSTree::remove(int key,BSTNode*& root)
{
BSTNode* node = search(key,root);
if(node == NULL)
{
return NULL;
}
/*1.如果删除叶子结点*/
if(node->left == NULL && node->right == NULL)
{
/*删除的是根结点*/
if(node == root)
{
return NULL;
}
/*删除左叶子结点*/
if(node->parent->left == node)
{
node->parent->left = NULL;
}
/*删除右叶子结点*/
if(node->parent->right == node)
{
node->parent->right = NULL;
}
}
/*2.如果删除的结点没有左子树或没有右子树*/
/* 2.1没有左子树*/
else if(node->left == NULL)
{
if(node == root)
{
root = root->right;
}
if(node->parent->left == node)
{
node->parent->left = node->right;
node->right->parent = node->parent;
}
else
{
node->left->parent == node->parent;
node->parent->right = node->left;
}
}
/* 2.2没有右子树*/
else if(node->right == NULL)
{
if(node == root)
{
root = root->left;
}
if(node->parent->left == node)
{
node->parent->left = node->left;
node->left->parent = node->parent;
}
else
{
node->right->parent = node->parent;
node->parent->left = node->right;
}
}
/*3.如果既有左子树又有右子树*/
else if(node->left != NULL && node->right != NULL)
{
BSTNode* post_node = successor(key,root->right);
if(node == root)
{
if(post_node->parent->left = post_node)
{
post_node->parent->left = NULL;
}
else
{
post_node->parent->right = NULL;
}
post_node->parent = NULL;
post_node->left = node->left;
post_node->right = node->right;
node->left->parent = post_node;
node->right->parent = post_node;
root = post_node;
}
else
{
BSTNode* pnode = node->parent;
if(post_node->parent->left == post_node)
{
post_node->parent->left = NULL;
}
else
{
post_node->parent->right = NULL;
}
post_node->parent = NULL;
if(pnode->left == node)
{
}
else
{
post_node->parent = pnode;
pnode->right = post_node;
post_node->parent = pnode;
post_node->left = node->left;
post_node->right = node->right;
node->left->parent = post_node;
node->right->parent = post_node;
}
}
}
return root;
}
#endif // BSTREE_H_INCLUDED
#include <iostream>
#include "BSTree.h"
using namespace std;
int main()
{
int a[] = {3,2,6,4,5};
BSTree* bst = new BSTree();
for(int i = 0;i < 5;i++)
{
bst->insert(a[i]);
}
cout<<"前序遍历:";
bst->preOrder(bst->getRoot());
cout<<endl;
cout<<"中序遍历:";
bst->inOrder(bst->getRoot());
cout<<endl;
cout<<"后序遍历:";
bst->postOrder(bst->getRoot());
cout<<endl;
cout<<"广度遍历:";
bst->printFromTopToBottom(bst->getRoot());
cout<<endl;
BSTNode* max_node = bst->minimum(bst->getRoot());
cout<<"当前树中最小的结点键值key = "<<max_node->key;
cout<<endl;
BSTNode* min_node = bst->maximum(bst->getRoot());
cout<<"当前树中最大的结点键值key = "<<min_node->key;
cout<<endl;
int key;
cin>>key;
cout<<"查找"<<key<<"是否存在树中:";
BSTNode* search_key = bst->search(key,bst->getRoot());
if(search_key != NULL)
{
cout<<search_key->key;
}
else
{
cout<<"不存在!";
}
cout<<endl;
cout<<"查找"<<key<<"的前驱结点为:";
BSTNode* pre_node = bst->precessor(key,bst->getRoot());
if(pre_node != NULL)
{
cout<<pre_node->key;
}
else
{
cout<<"不存在!";
}
cout<<endl;
cout<<"查找"<<key<<"的后继结点为:";
BSTNode* post_node = bst->successor(key,bst->getRoot());
if(post_node != NULL)
{
cout<<post_node->key;
}
else
{
cout<<"不存在!";
}
cout<<endl;
/*
cout<<"删除"<<key<<"的中序遍历为:";
BSTNode* root = bst->getRoot();
bst->remove(key,root);
bst->inOrder(root);
cout<<endl;
bst->insert(2);
bst->inOrder(root);
cout<<endl;
*/
// key = ;
cout<<"删除"<<key<<"的中序遍历为:";
BSTNode* root = bst->getRoot();
bst->remove(key,root);
bst->inOrder(root);
cout<<endl;
return 0;
}
实现方式二:
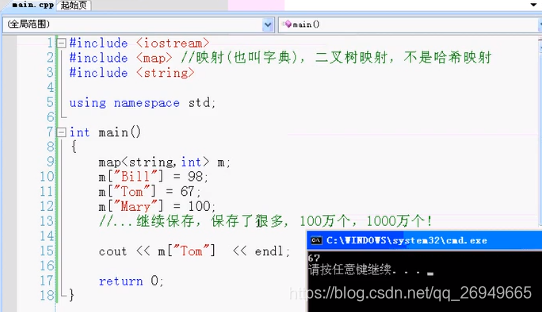