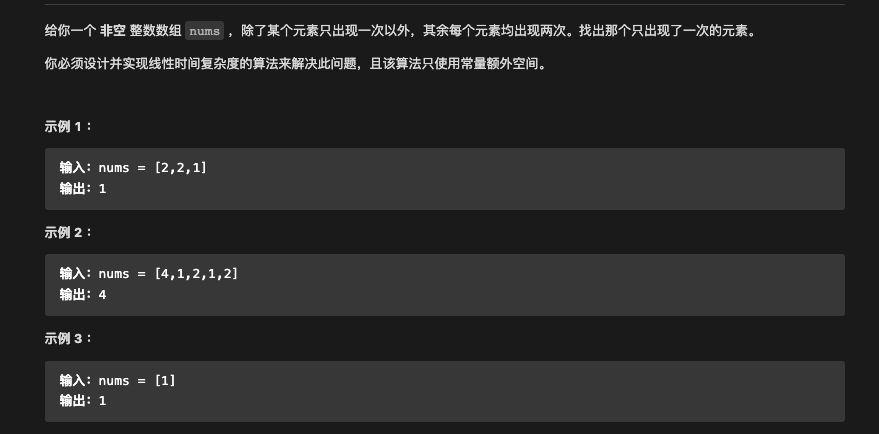
第一种解题思路:
双重循环记录每种数字出现的次数,找到出现次数为1的数字即可,优点是好理解,缺点是运行效率低
public static int SingleNumber_1(int[] nums)
{
if (nums.Length <= 1)
return nums[0];
int length = nums.Length;
//记录某一数字出现的 次数
int count = 0;
//最后查找出来的结果
int result = 0;
for (int i = 0; i < length; i++)
{
//获取要查找的数字
int target = nums[i];
count = 1;
for (int j = 0; j < length; j++)
{
//不比较自身位置的数字
if (j != i)
{
if (target == nums[j])
count = 2;
}
}
if (count == 1)
{
result = nums[i];
break;
}
}
return result;
}
第二种解题思路:
使用异或运算,异或的原理是两个数相同为false,不同为true
异或运算,相异为真,相同为假,所以 a^a = 0 ;0^a = a
因为异或运算 满足交换律 a^b^a = a^a^b = b 所以数组经过异或运算,单独的值就剩下了
比如例子[4,1,2,1,2]异或运算可以变为这样----4^1^2^1^2 = 4^1^1^2^2 = 4^0^0 = 4
优点是运算效率高,缺点是理解起来有点难
//只出现一次的数字
public static int SingleNumber_2(int[] nums)
{
//使用异或运算
int length = nums.Length;
int result = 0;
for (int i = 0; i < length; i++)
{
result ^= nums[i];
}
return result;
}