喝酒不骑马的Android自学日记(4)-简易计算器的实现***2015年12月12日
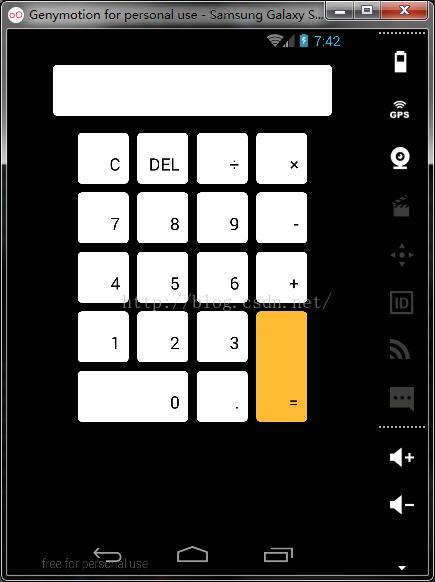
计算器分为两个大部分:
一、UI设计
1.布局设计
分为显示区和按钮区。整体运用线性垂直布局。EditText是第一行,充当显示的功能。往下,每一行有4个钮,每一行用线性水平布局,与EditText行距设置为20dp。没行四个钮居中,钮间距用layout_marginLeft设置为10dp。长宽都为60dp。行与行间距用layout_marginTop设置为10dp。第三行第四行由于有个等号键,所以三四行算做一行,整体用水平线性,123和0&.用线性垂直布局。
2.样式设计
在drawble里自定样式。
新建一个white_bg.xml文件
radius是设置边角为半径为5dp的圆角。
solid color设置填充色。
新建一个white_selector.xml文件
设置按钮在按下和抬起时不同颜色。当state_pressed="true"时,为灰色。当然了,这里的gray_bg也是我创建的颜色。当按钮不在按下状态时,为白色。
在button按钮里,设置背景颜色为创建的文件
android:background="@drawable/white_selector"
设置button中显示的数字&字母位置,靠右下
android:gravity="right|bottom"
设置完后,在测试中会发现过于贴着右下,所以设置与右边和下边的边距
android:paddingBottom="10dp"
android:paddingRight="10dp"
关于界面UI的设计基本完成。
二、逻辑设计
初始化button和edittext。注册监听。
分析一下这些按钮。数字0~9和point键,都是按下去后,仅在显示屏显示内容,不进行相关运算和删除操作。所以
et_input.setText(str + ((Button) v).getText());//实现点一下就显示一下的功能
对于四个运算符按钮,设置
et_input.setText(str + " " + ((Button) v).getText() + " ");
在左右加上两个空格的意义是为了之后的判断。
对于delete按钮
if (str != null && !str.equals("")) {
//substring用法:从第x个字符开始,截取y长度的内容。
et_input.setText(str.substring(0, str.length() - 1));
}
判断下,如果编辑器不为空,则通过截取字符串长度-1的方式实现delete功能。
clear按钮
et_input.setText("");//清屏
最后的等号按钮
因为设计不同的运算,所以创建一个方法private void getResult()
如果显示屏里没有内容,则不进行任何操作。
如果显示屏里没有空格,也就是说,没有运算符,也不进行任何操作。
然后定义三个String类型,分别截取运算符两边的数字和运算符本身。
String s1 = exp.substring(0, exp.indexOf(" "));//截取运算符前面的字符
String op = exp.substring(exp.indexOf(" ") + 1, exp.indexOf(" ") + 2);//截取运算符
String s2 = exp.substring(exp.indexOf(" ") + 3);//截取运算符后面的字符
indexOf的方法是返回 String 对象内第一次出现子字符串的字符位置。这样就知道了哪两个数字,以及要进行什么运算。
通过判断运算符,来对两边的数字进行相对应的运算。
判断数字是否有小数点,如果没有小数点,就强制转化为整形。
if (!s1.equals("") && s2.equals("")) {
et_input.setText(exp);//这种情况是一个运算符,后面只跟着数字,这种情况不运算。
这样的话第一个数字相当于0。
最后,如果都为空,则显示空
当进行完等号运算后,再次输入数字会代替原来的结果。这个问题怎么解决呢?
设置个boolean类型标记clear_flag
当摁下回车后,标记变为true。
在其他按钮按下时,进行判断
if (clear_flag) {
clear_flag = false;
str = "";
et_input.setText("");
}
et_input.setText(str + ((Button) v).getText());
如果clear_flag为true,则清空显示屏,然后显示输入的内容。
三、程序调试
四、程序存在的缺陷
这个计算机功能简单。
因为截取运算符和两边数字的逻辑原因,只能运算两个数字之间的运算逻辑。不能一次性运算发杂的式子。同时,没有纠错功能。当输入格式不规范时,比如“5++++”,这时摁回车会导致程序崩溃。原因也是因为对于字符串的截取问题。
代码:
activity_main.xml:
ashen.xml:
gray_bg.xml:
orange_bg:
orange_selector.xml:
white_bg.xml:
white_selector.xml:
MainActivity.java:
计算器分为两个大部分:
一、UI设计
1.布局设计
分为显示区和按钮区。整体运用线性垂直布局。EditText是第一行,充当显示的功能。往下,每一行有4个钮,每一行用线性水平布局,与EditText行距设置为20dp。没行四个钮居中,钮间距用layout_marginLeft设置为10dp。长宽都为60dp。行与行间距用layout_marginTop设置为10dp。第三行第四行由于有个等号键,所以三四行算做一行,整体用水平线性,123和0&.用线性垂直布局。
2.样式设计
在drawble里自定样式。
新建一个white_bg.xml文件
<span style="font-size:18px;"><shape xmlns:android="http://schemas.android.com/apk/res/android">
<corners android:radius="5dp" />
<solid android:color="@android:color/white"/>
</shape></span>
radius是设置边角为半径为5dp的圆角。
solid color设置填充色。
新建一个white_selector.xml文件
<span style="font-size:18px;"><selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/gray_bg" android:state_pressed="true"/>
<item android:drawable="@drawable/white_bg"/>
</selector></span>
设置按钮在按下和抬起时不同颜色。当state_pressed="true"时,为灰色。当然了,这里的gray_bg也是我创建的颜色。当按钮不在按下状态时,为白色。
在button按钮里,设置背景颜色为创建的文件
android:background="@drawable/white_selector"
设置button中显示的数字&字母位置,靠右下
android:gravity="right|bottom"
设置完后,在测试中会发现过于贴着右下,所以设置与右边和下边的边距
android:paddingBottom="10dp"
android:paddingRight="10dp"
关于界面UI的设计基本完成。
二、逻辑设计
初始化button和edittext。注册监听。
分析一下这些按钮。数字0~9和point键,都是按下去后,仅在显示屏显示内容,不进行相关运算和删除操作。所以
et_input.setText(str + ((Button) v).getText());//实现点一下就显示一下的功能
对于四个运算符按钮,设置
et_input.setText(str + " " + ((Button) v).getText() + " ");
在左右加上两个空格的意义是为了之后的判断。
对于delete按钮
if (str != null && !str.equals("")) {
//substring用法:从第x个字符开始,截取y长度的内容。
et_input.setText(str.substring(0, str.length() - 1));
}
判断下,如果编辑器不为空,则通过截取字符串长度-1的方式实现delete功能。
clear按钮
et_input.setText("");//清屏
最后的等号按钮
因为设计不同的运算,所以创建一个方法private void getResult()
如果显示屏里没有内容,则不进行任何操作。
如果显示屏里没有空格,也就是说,没有运算符,也不进行任何操作。
然后定义三个String类型,分别截取运算符两边的数字和运算符本身。
String s1 = exp.substring(0, exp.indexOf(" "));//截取运算符前面的字符
String op = exp.substring(exp.indexOf(" ") + 1, exp.indexOf(" ") + 2);//截取运算符
String s2 = exp.substring(exp.indexOf(" ") + 3);//截取运算符后面的字符
indexOf的方法是返回 String 对象内第一次出现子字符串的字符位置。这样就知道了哪两个数字,以及要进行什么运算。
通过判断运算符,来对两边的数字进行相对应的运算。
判断数字是否有小数点,如果没有小数点,就强制转化为整形。
if (!s1.equals("") && s2.equals("")) {
et_input.setText(exp);//这种情况是一个运算符,后面只跟着数字,这种情况不运算。
这样的话第一个数字相当于0。
最后,如果都为空,则显示空
当进行完等号运算后,再次输入数字会代替原来的结果。这个问题怎么解决呢?
设置个boolean类型标记clear_flag
当摁下回车后,标记变为true。
在其他按钮按下时,进行判断
if (clear_flag) {
clear_flag = false;
str = "";
et_input.setText("");
}
et_input.setText(str + ((Button) v).getText());
如果clear_flag为true,则清空显示屏,然后显示输入的内容。
三、程序调试
四、程序存在的缺陷
这个计算机功能简单。
因为截取运算符和两边数字的逻辑原因,只能运算两个数字之间的运算逻辑。不能一次性运算发杂的式子。同时,没有纠错功能。当输入格式不规范时,比如“5++++”,这时摁回车会导致程序崩溃。原因也是因为对于字符串的截取问题。
代码:
activity_main.xml:
<span style="font-size:18px;"><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<EditText
android:id="@+id/et_input"
android:layout_width="match_parent"
android:layout_height="60dip"
android:background="@drawable/white_bg"
android:editable="false"
android:gravity="right|bottom"
android:textSize="40sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:gravity="center_horizontal"
android:orientation="horizontal">
<Button
android:id="@+id/bt_clear"
android:layout_width="60dp"
android:layout_height="60dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="C"
android:textSize="20sp" />
<Button
android:id="@+id/bt_delete"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="DEL"
android:textSize="20sp" />
<Button
android:id="@+id/bt_divide"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="÷"
android:textSize="20sp" />
<Button
android:id="@+id/bt_mutiply"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="×"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:gravity="center_horizontal"
android:orientation="horizontal">
<Button
android:id="@+id/bt_7"
android:layout_width="60dp"
android:layout_height="60dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="7"
android:textSize="20sp" />
<Button
android:id="@+id/bt_8"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="8"
android:textSize="20sp" />
<Button
android:id="@+id/bt_9"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="9"
android:textSize="20sp" />
<Button
android:id="@+id/bt_minus"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="-"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:gravity="center_horizontal"
android:orientation="horizontal">
<Button
android:id="@+id/bt_4"
android:layout_width="60dp"
android:layout_height="60dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="4"
android:textSize="20sp" />
<Button
android:id="@+id/bt_5"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="5"
android:textSize="20sp" />
<Button
android:id="@+id/bt_6"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="6"
android:textSize="20sp" />
<Button
android:id="@+id/bt_plus"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="+"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:gravity="center_horizontal"
android:orientation="horizontal">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/bt_1"
android:layout_width="60dp"
android:layout_height="60dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="1"
android:textSize="20sp" />
<Button
android:id="@+id/bt_2"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="2"
android:textSize="20sp" />
<Button
android:id="@+id/bt_3"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="3"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:orientation="horizontal">
<Button
android:id="@+id/bt_0"
android:layout_width="130dp"
android:layout_height="60dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="0"
android:textSize="20sp" />
<Button
android:id="@+id/bt_point"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_marginLeft="10dp"
android:background="@drawable/white_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="."
android:textSize="20sp" />
</LinearLayout>
</LinearLayout>
<Button
android:id="@+id/bt_equal"
android:layout_width="60dp"
android:layout_height="130dp"
android:layout_marginLeft="10dp"
android:background="@drawable/orange_selector"
android:gravity="right|bottom"
android:paddingBottom="10dp"
android:paddingRight="10dp"
android:text="="
android:textSize="20sp" />
</LinearLayout>
</LinearLayout></span>
ashen.xml:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<corners android:radius="5dp" />
<solid android:color="#a3a22e"/>
</shape></span>
gray_bg.xml:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<corners android:radius="5dp" />
<solid android:color="@android:color/darker_gray"/>
</shape></span>
orange_bg:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<corners android:radius="5dp" />
<solid android:color="@android:color/holo_orange_light"/>
</shape></span>
orange_selector.xml:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/ashen" android:state_pressed="true"/>
<item android:drawable="@drawable/orange_bg"/>
</selector></span>
white_bg.xml:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<corners android:radius="5dp" />
<solid android:color="@android:color/white"/>
</shape></span>
white_selector.xml:
<span style="font-size:18px;"><?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/gray_bg" android:state_pressed="true"/>
<item android:drawable="@drawable/white_bg"/>
</selector></span>
MainActivity.java:
<span style="font-size:18px;">package com.example.martin.calculatordemo;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity implements View.OnClickListener {
Button bt_1;//数字键1
Button bt_2;//数字键2
Button bt_3;//数字键3
Button bt_4;//数字键4
Button bt_5;//数字键5
Button bt_6;//数字键6
Button bt_7;//数字键7
Button bt_8;//数字键8
Button bt_9;//数字键9
Button bt_0;//数字键0
Button bt_point;//小数点键
Button bt_clear;//清屏键
Button bt_plus;//加号键
Button bt_multiply;//乘号键
Button bt_delete;//删除键
Button bt_minus;//减法键
Button bt_divide;//除号键
Button bt_equal;//等号键
EditText et_input;//显示输入内容的显示屏
boolean clear_flag;//清空标识
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//舒适化这些按键
bt_0 = (Button) findViewById(R.id.bt_0);
bt_1 = (Button) findViewById(R.id.bt_1);
bt_2 = (Button) findViewById(R.id.bt_2);
bt_3 = (Button) findViewById(R.id.bt_3);
bt_4 = (Button) findViewById(R.id.bt_4);
bt_5 = (Button) findViewById(R.id.bt_5);
bt_6 = (Button) findViewById(R.id.bt_6);
bt_7 = (Button) findViewById(R.id.bt_7);
bt_8 = (Button) findViewById(R.id.bt_8);
bt_9 = (Button) findViewById(R.id.bt_9);
bt_plus = (Button) findViewById(R.id.bt_plus);
bt_minus = (Button) findViewById(R.id.bt_minus);
bt_multiply = (Button) findViewById(R.id.bt_mutiply);
bt_divide = (Button) findViewById(R.id.bt_divide);
bt_clear = (Button) findViewById(R.id.bt_clear);
bt_delete = (Button) findViewById(R.id.bt_delete);
bt_equal = (Button) findViewById(R.id.bt_equal);
bt_point = (Button) findViewById(R.id.bt_point);
//以上是实例化按钮
et_input = (EditText) findViewById(R.id.et_input);
bt_0.setOnClickListener(this);
bt_1.setOnClickListener(this);
bt_2.setOnClickListener(this);
bt_3.setOnClickListener(this);
bt_4.setOnClickListener(this);
bt_5.setOnClickListener(this);
bt_6.setOnClickListener(this);
bt_7.setOnClickListener(this);
bt_8.setOnClickListener(this);
bt_9.setOnClickListener(this);
bt_plus.setOnClickListener(this);
bt_point.setOnClickListener(this);
bt_equal.setOnClickListener(this);
bt_delete.setOnClickListener(this);
bt_divide.setOnClickListener(this);
bt_clear.setOnClickListener(this);
bt_minus.setOnClickListener(this);
bt_multiply.setOnClickListener(this);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onClick(View v) {
String str = et_input.getText().toString();
switch (v.getId()) {
//数字键0~9和小数点键的特点是,点一下就在显示屏上显示。
case R.id.bt_0:
case R.id.bt_1:
case R.id.bt_2:
case R.id.bt_3:
case R.id.bt_4:
case R.id.bt_5:
case R.id.bt_6:
case R.id.bt_7:
case R.id.bt_8:
case R.id.bt_9:
case R.id.bt_point:
if (clear_flag) {
clear_flag = false;
str = "";
et_input.setText("");
}
et_input.setText(str + ((Button) v).getText());//实现点一下就显示一下的功能
break;
//运算符的功能
case R.id.bt_plus:
case R.id.bt_minus:
case R.id.bt_mutiply:
case R.id.bt_divide:
if (clear_flag) {
clear_flag = false;
str = "";
et_input.setText("");
}
et_input.setText(str + " " + ((Button) v).getText() + " ");
break;
case R.id.bt_delete:
if (clear_flag) {
clear_flag = false;
str = "";
et_input.setText("");
} else if (str != null && !str.equals("")) {
//substring用法:从第x个字符开始,截取y长度的内容。
et_input.setText(str.substring(0, str.length() - 1));
}
break;
case R.id.bt_clear:
clear_flag = false;
str = "";
et_input.setText("");//清屏
break;
case R.id.bt_equal:
getResult();
break;
}
}
//运算结果
private void getResult() {
String exp = et_input.getText().toString();
if (exp == null || exp.equals("")) {
return;//如果为空,则返回
}
if (!exp.contains(" ")) {
//String.contains()方法用法实例教程, 返回true,当且仅当此字符串包含指定的char值序列
return;
}
if (clear_flag) {//判断,如果点完等号,再点等号,为了避免清屏,则赋值false
clear_flag = false;
return;
}
clear_flag = true;
double result = 0;
//indexOf 方法,返回 String 对象内第一次出现子字符串的字符位置。
String s1 = exp.substring(0, exp.indexOf(" "));//截取运算符前面的字符
String op = exp.substring(exp.indexOf(" ") + 1, exp.indexOf(" ") + 2);//截取运算符
String s2 = exp.substring(exp.indexOf(" ") + 3);//截取运算符后面的字符
if (!s1.equals("") && !s2.equals("")) {
double d1 = Double.parseDouble(s1);
double d2 = Double.parseDouble(s2);
if (op.equals("+")) {
result = d1 + d2;
} else if (op.equals("-")) {
result = d1 - d2;
} else if (op.equals("×")) {
result = d1 * d2;
} else if (op.equals("÷")) {
if (d2 == 0) {//如果分母为0,则结果为0
result = 0;
} else {
result = d1 / d2;
}
}
if (!s1.contains(".") && !s2.contains(".")&&!op.equals("÷")) {//判断有没有小数点
int r = (int) result;//如果没有小数点,强制转换成整形
et_input.setText(r + "");
} else {
et_input.setText(result + "");
}
} else if (!s1.equals("") && s2.equals("")) {
et_input.setText(exp);//这种情况是一个运算符,后面只跟着数字,这种情况不运算。
} else if (s1.equals("") && !s2.equals("")) {
double d2 = Double.parseDouble(s2);
if (op.equals("+")) {
result = 0 + d2;
} else if (op.equals("-")) {
result = 0 - d2;
} else if (op.equals("×")) {
result = 0;
} else if (op.equals("÷")) {
result = 0;
}
if (!s2.contains(".")) {
int r = (int) result;
et_input.setText(r + "");
} else {
et_input.setText(result + "");
}
} else {
et_input.setText("");//如果都是空,就显示为空
}
}
}</span>