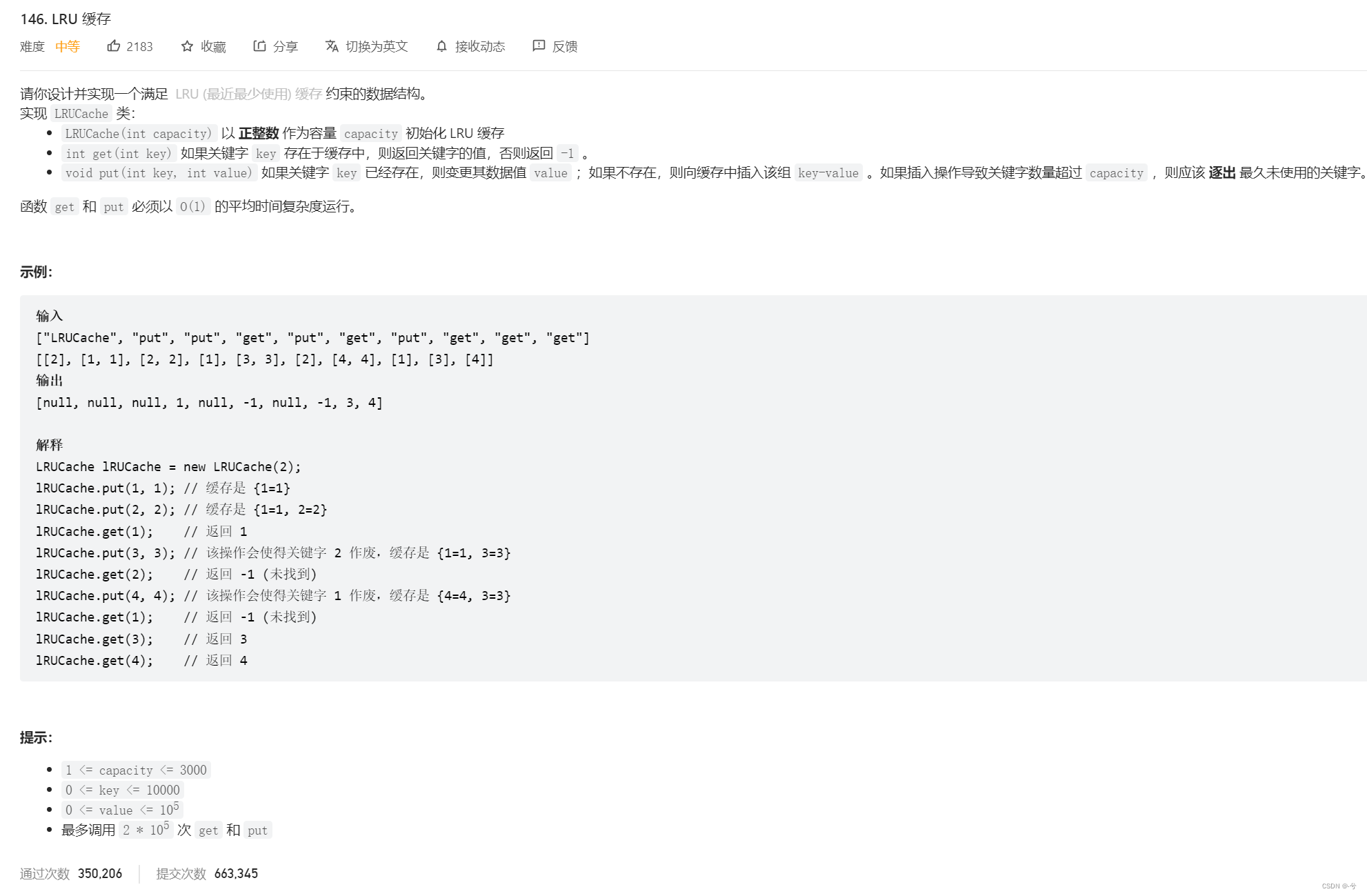
结果
class LRUCache {
public:
struct node { //双向链表
int key, value;
node *front, *next;
node () {
key = 0, value = 0;
front = nullptr, next = nullptr;
}
node (int k, int v) {
key = k, value = v;
front = nullptr, next = nullptr;
}
};
node *l, //临时头节点,一直指向双向链表的头节点
*r; //临时尾节点,双向链表尾节点一直指向此节点
unordered_map<int, node *> m; //哈希表
int ma, //容量上限
now; //当前的节点个数
LRUCache(int capacity) {
ma = capacity, now = 0;
l = new node();
r = new node();
l->next = r;
r->front = l;
}
void push_front(node *p) { //将目标值放到最前面
if (p->next) { //如果p存在
p->front->next = p->next;
p->next->front = p->front;
}
//将p调整到双向链表的头部
p->next = l->next;
l->next->front = p;
p->front = l;
l->next = p;
}
int get(int key) {
if (m.count(key)) {
push_front(m[key]);
return m[key]->value;
}
return -1;
}
void put(int key, int value) {
if (m.count(key)) {
m[key]->value = value;
push_front(m[key]);
return ;
}
node *p = new node(key, value);
push_front(p);
m[key] = p;
++now;
if (now > ma) {
--now;
//删掉r->front这个节点
node *tmp = r->front; //临时尾节点的上一个节点
tmp->front->next = r;
r->front = tmp->front;
m.erase(tmp->key);
delete tmp;
}
}
};
/**
* Your LRUCache object will be instantiated and called as such:
* LRUCache* obj = new LRUCache(capacity);
* int param_1 = obj->get(key);
* obj->put(key,value);
*/