设置优先级
package com.yuzhenc.thread;
public class Test04 {
public static void main(String[] args) {
TestThread4 testThread4 = new TestThread4();
testThread4.setPriority(1);
TestThread5 testThread5 = new TestThread5();
Thread thread = new Thread(testThread5);
thread.setPriority(10);
testThread4.start();
thread.start();
}
}
class TestThread4 extends Thread {
@Override
public void run() {
for (int i = 0; i < 10000; i++) {
System.out.println(i);
}
}
}
class TestThread5 implements Runnable {
@Override
public void run() {
for (int i = 10000; i < 20000; i++) {
System.out.println(i);
}
}
}
join
package com.yuzhenc.thread;
public class Test06 {
public static void main(String[] args) throws InterruptedException {
for (int i = 0; i < 10; i++) {
System.out.println("main----"+i);
if (i == 6) {
TestThread6 testThread6 = new TestThread6("子线程");
testThread6.start();
testThread6.join();
}
}
}
}
class TestThread6 extends Thread {
public TestThread6(String name) {
super(name);
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
System.out.println(this.getName()+"----"+i);
}
}
}
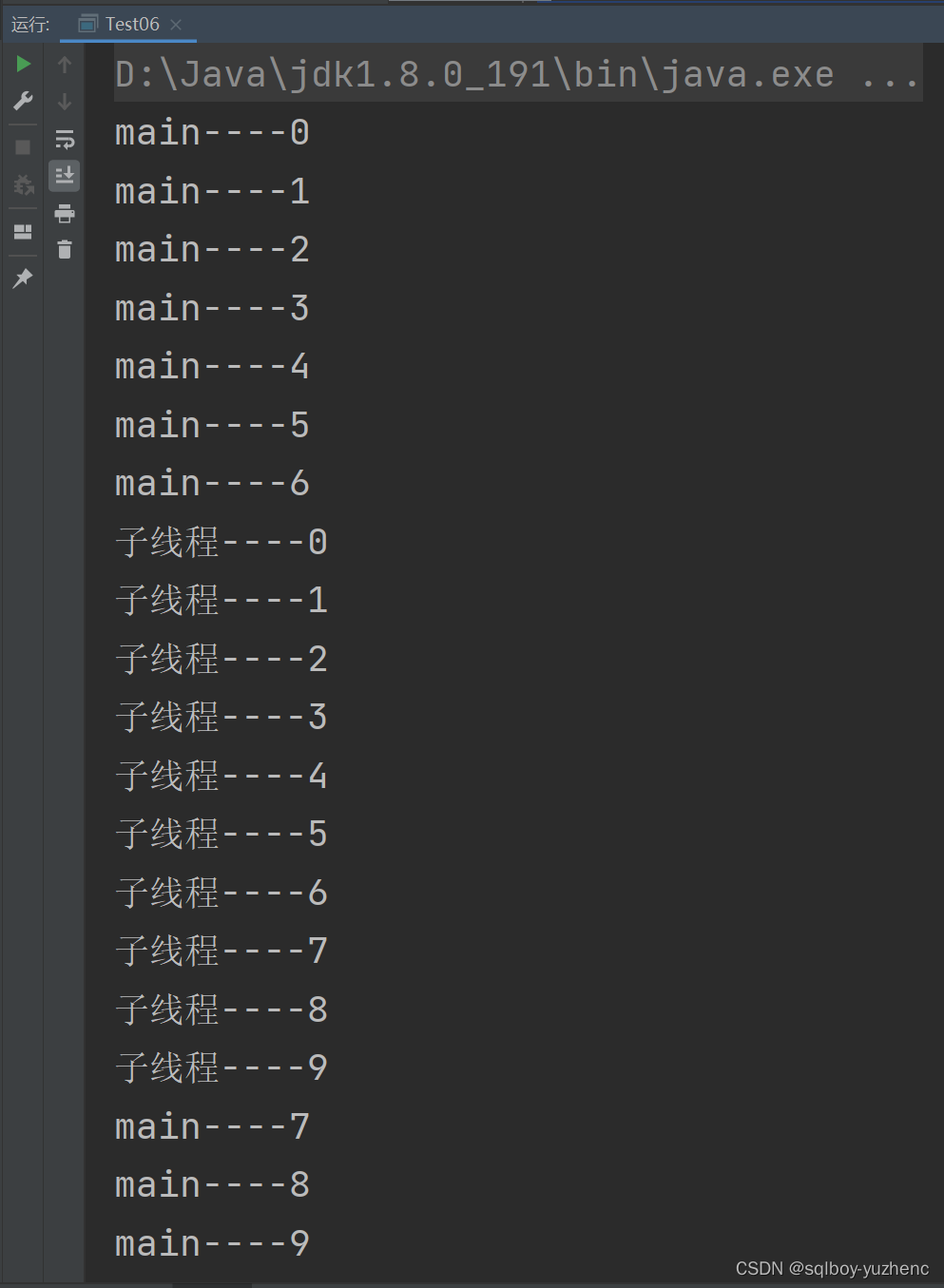
sleep
package com.yuzhenc.thread;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Test07 {
public static void main(String[] args) {
DateFormat df = new SimpleDateFormat("HH:mm:ss");
while(true) {
Date d = new Date();
System.out.println(df.format(d));
try {
Thread.sleep(1000);
} catch (InterruptedException interruptedException) {
System.out.println(interruptedException.getStackTrace());
}
}
}
}
setDaemon
package com.yuzhenc.thread;
public class Test08 {
public static void main(String[] args) {
TestThread7 thread7 = new TestThread7();
thread7.setDaemon(true);
thread7.start();
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
}
}
class TestThread7 extends Thread{
@Override
public void run() {
for (int i = 10; i < 1000; i++) {
System.out.println(i);
}
}
}