1默认构造函数和有参构造函数
1.1构造函数分开声明定义
#include<iostream>
using namespace std;
class Person {
public:
Person();
Person(int newAge);
Person(const Person& p);
~Person();
int m_age;
};
Person::Person()
{
m_age = 0;
cout << "默认构造函数" << endl;
}
Person::Person(int newAge)
{
m_age = newAge;
cout << "有参构造函数" ;
}
Person::Person(const Person& p)
{
m_age = p.m_age;
cout << "拷贝构造函数";
}
Person::~Person()
{
cout << "析构函数" << endl;
}
void test01()
{
Person p1;
Person p2(1);
cout << "P2的年龄为" << p2.m_age << endl;
p2.m_age = 10;
Person p3(p2);
cout << "P3的年龄为" << p3.m_age << endl;
Person p4 = Person(100);
cout << "P4的年龄为" << p4.m_age << endl;
}
int main()
{
test01();
return 0;
}
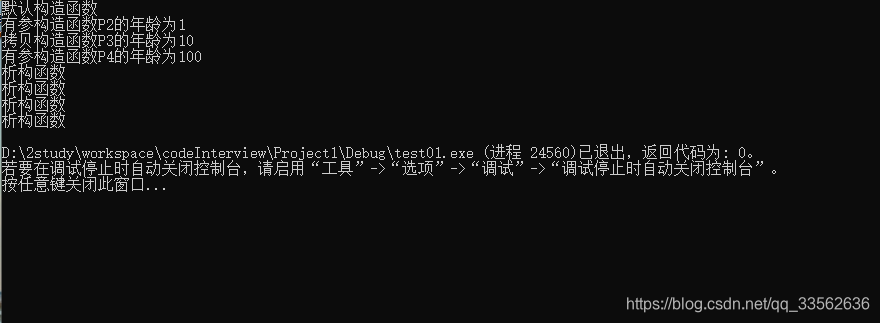
1.2构造函数一块声明定义
#include<iostream>
using namespace std;
class Person {
public:
Person(int newAge=NULL);
Person(const Person& p);
~Person();
int m_age;
};
Person::Person(int newAge)
{
if (newAge == NULL)
{
m_age = 0;
cout << "默认构造函数" << endl;
}
else {
m_age = newAge;
cout << "有参构造函数";
}
}
Person::Person(const Person& p)
{
m_age = p.m_age;
cout << "拷贝构造函数";
}
Person::~Person()
{
cout << "析构函数" << endl;
}
void test01()
{
Person p1;
Person p2(1);
cout << "P2的年龄为" << p2.m_age << endl;
p2.m_age = 10;
Person p3(p2);
cout << "P3的年龄为" << p3.m_age << endl;
Person p4 = Person(100);
cout << "P4的年龄为" << p4.m_age << endl;
}
int main()
{
test01();
return 0;
}
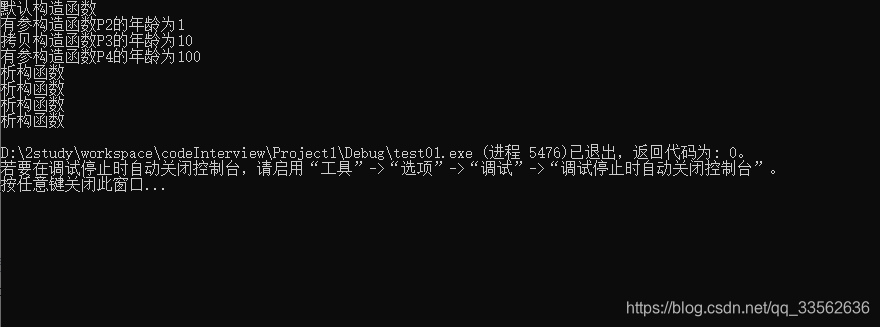
1.3相关知识
1.对象所占据的内存空间只是用于存放数据成员,函数成员不在每一个对象中存储副本,每个函数的代码在内存中只占据一份空间。
2.如果有的函数成员需要被频繁调用,而且代码比较简单,则这个函数可以定义为内联函数,关键字为inline,如打印函数。
3.如果类中声明了构造函数(无论是否有参),编译器便不会再为之生成隐含的构造函数。
4.如果希望程序在对象被删除之前的时刻自动的完成某些事情,可以把他们写到析构函数中。