如何一次创建多个线程:
#include<stdio.h>
#include<unistd.h>
#include<malloc.h>
#include<pthread.h>
#define MAX_THREAD_NUM 10
void* thread_fun(void*arg)
{
int i = *(int*)arg;
printf("[%d]thread start up.\n",i);
pthread_exit(0);
}
int main()
{
pthread_t tid[MAX_THREAD_NUM];
for(int i=0;i<MAX_THREAD_NUM;++i)
{
pthread_create(&tid[i],NULL,thread_fun,&i);
sleep(1);
}
for(int i=0;i<MAX_THREAD_NUM;++i)
{
pthread_join(tid[i],NULL);
}
return 0;
}
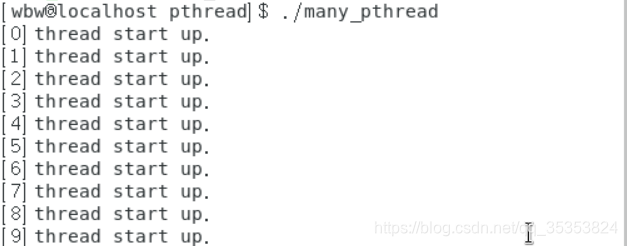
上述程序用for 循环创建了多个线程,并将参数传递给线程。sleep(1)函数一定要加上,不然得不到想要的效果。sleep()函数的等待并不释放cpu资源。
多线程的同步之互斥量:
#include<stdio.h>
#include<unistd.h>
#include<malloc.h>
#include<pthread.h>
//pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_mutex_t mutex;
int count = 0;
void* thread_fun1(void* arg)
{
pthread_mutex_lock(&mutex);
count = 10;
sleep(3);
printf("This is thread fun1.count = %d\n",count);
pthread_mutex_unlock(&mutex);
}
void* thread_fun2(void* arg)
{
pthread_mutex_lock(&mutex);
count = 100;
printf("This is thread fun2.count = %d\n",count);
pthread_mutex_unlock(&mutex);
}
int main()
{
pthread_mutex_init(&mutex, NULL);
pthread_t tid1,tid2;
pthread_create(&tid1,NULL,thread_fun1,NULL);
sleep(1);
pthread_create(&tid2,NULL,thread_fun2,NULL);
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_mutex_destroy(&mutex);
return 0;
}

现在对上述的互斥量的实验程序进行分析:首先在main()函数中创建了两个线程,先执行线程1,再执行线程2.线程1对count加互斥所,此时线程2就不能访问count。只有当沉睡时间过后线程1对互斥量解锁之后,线程2才能加锁修改count的值并访问,最后解锁。
trylock()函数:同样也是对线程加锁(非阻塞的)加锁成功或者是不成功都会有返回值,立即处理。
#include<stdio.h>
#include<unistd.h>
#include<malloc.h>
#include<pthread.h>
//pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_mutex_t mutex;
int count = 0;
void* thread_fun1(void* arg)
{
pthread_mutex_lock(&mutex);
count = 10;
// sleep(3);
printf("This is thread fun1.count = %d\n",count);
pthread_mutex_unlock(&mutex);
}
void* thread_fun2(void* arg)
{
sleep(1);
int ret = pthread_mutex_trylock(&mutex);
if(ret == 0)
{
printf("Try lock mutex success.\n");
count = 100;
printf("This is thread fun2.count = %d\n",count);
pthread_mutex_unlock(&mutex);
}
else
printf("Try lock mutex fail. ret = %d\n",ret);
}
int main()
{
pthread_mutex_init(&mutex, NULL);
pthread_t tid1,tid2;
pthread_create(&tid1,NULL,thread_fun1,NULL);
sleep(1);
pthread_create(&tid2,NULL,thread_fun2,NULL);
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_mutex_destroy(&mutex);
return 0;
}

如果注释掉thread_fun1()函数中的pthread_mutex_unlock(&mutex),则会打印“Try lock mutex fail.”并返回打开失败的错误类型。
多进程同步之条件量:
#include<stdio.h>
#include<unistd.h>
#include<malloc.h>
#include<pthread.h>
//pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_mutex_t mutex;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
int count = 0;
void* thread_fun1(void* arg)
{
pthread_mutex_lock(&mutex);
count = 10;
//sleep(3);
printf("aaaaaaaaaaaaa.\n");
pthread_cond_wait(&cond, &mutex);//pthread_mutex_unclock().
printf("bbbbbbbbbbbbb.\n");
printf("This is thread fun1.count = %d\n",count);
//pthread_mutex_unlock(&mutex);
}
void* thread_fun2(void* arg)
{
sleep(1);
int ret = pthread_mutex_trylock(&mutex);
if(ret == 0)
{
printf("Try lock mutex success.\n");
count = 100;
printf("This is thread fun2.count = %d\n",count);
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&cond);
}
else
printf("Try lock mutex fail.ret = %d\n",ret);
}
int main()
{
pthread_mutex_init(&mutex, NULL);
pthread_t tid1,tid2;
pthread_create(&tid1,NULL,thread_fun1,NULL);
sleep(1);
pthread_create(&tid2,NULL,thread_fun2,NULL);
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
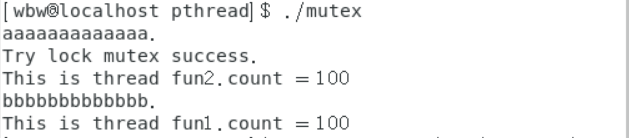
学会pthread_cond_wait()函数和pthread_cond_signal()函数的用法,理解这一对函数是怎么实现线程之间的同步的。当线程执行到
pthread_cond_wait()函数,会阻塞。直到pthread_cond_signal()函数执行唤醒原来的线程继续执行。线程1中有没有pthread_mutex_unlock(&mutex)函数似乎对实验结果没啥影响???
执行pthread_cond_wait()函数时临界区就会解锁!!!
pthread_cond_wait()方法是:在阻塞之时,自动解锁。该方法在遇到pthread_cond_signal()时,唤醒等待的wait()方法,但是不直接执行wait()其后的语句,而是接着原先pthread_cond_signal()其后的方法继续执行,直到遇到pthread_mutex_lock()锁时,此时,转到wait()其后的方法执行。从这个观点来看最后一个例子,就显得比较可疑。。。
下面的场景,你将体会到pthread_cond_broadcast()函数的作用:
#include<iostream>
#include<unistd.h>
#include<stdio.h>
#include<pthread.h>
#define MAX_SIZE 5
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
void* pthread_fun(void* arg)
{
int i = *(int *)arg;
printf("[%d]thread start up.\n",i);
pthread_mutex_lock(&mutex);
printf("[%d]thread wait......\n",i);
pthread_cond_wait(&cond,&mutex);
printf("[%d] thread wake up.\n",i);
pthread_mutex_unlock(&mutex);
}
int main()
{
pthread_t tid[MAX_SIZE];
for(int i=0;i<MAX_SIZE;++i)
{
pthread_create(&tid[i], NULL, pthread_fun, &i);
sleep(1);
}
sleep(3);
pthread_cond_broadcast(&cond);//唤醒多个阻塞的线程
for(int i=0;i<MAX_SIZE;++i)
{
pthread_join(tid[i], NULL);
}
return 0;
}
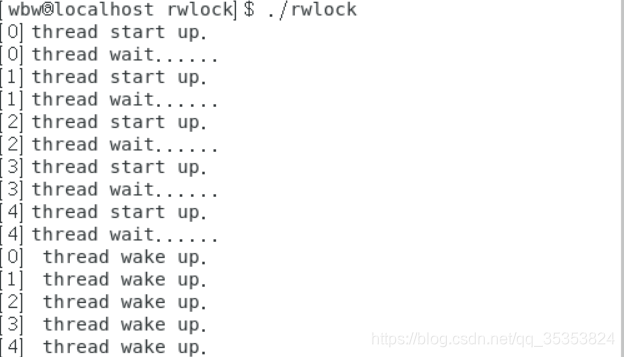
条件量的一个简单的应用:用两个线程轮流交换数完1-10,一个进程数奇数,一个进程数偶数。
#include<stdio.h>
#include<unistd.h>
#include<pthread.h>
#define MAX_COUNT 10
static int count = 1;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t is_odd = PTHREAD_COND_INITIALIZER;
pthread_cond_t is_even = PTHREAD_COND_INITIALIZER;
void* A_fun(void *arg)
{
pthread_mutex_lock(&mutex);
while(count <= MAX_COUNT)
{
if(count % 2 == 1)
{
printf("In A fun : %d\n", count++);
pthread_cond_signal(&is_even);
}
else
{
pthread_cond_wait(&is_odd, &mutex);
}
}
pthread_mutex_unlock(&mutex);
}
void* B_fun(void *arg)
{
pthread_mutex_lock(&mutex);
while(count <= MAX_COUNT)
{
if(count % 2 == 0)
{
printf("In B fun : %d\n", count++);
pthread_cond_signal(&is_odd);
}
else
{
pthread_cond_wait(&is_even, &mutex);
}
}
pthread_mutex_unlock(&mutex);
}
int main()
{
pthread_t tid1, tid2;
pthread_create(&tid2, NULL, B_fun, NULL);
sleep(5);
pthread_create(&tid1, NULL, A_fun, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
}
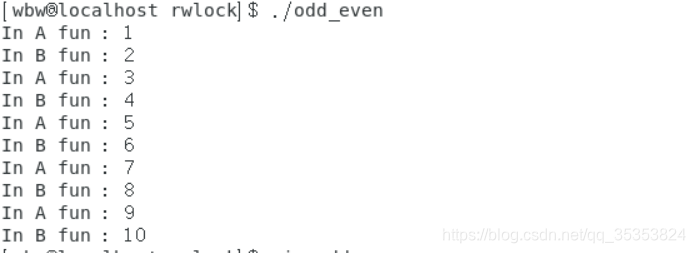
从代码可以看出,线程2先执行,过了5秒之后,线程1 才执行。程序执行的结果与两个进程的先后没有关系,符合编写程序的本意。
条件量是不是只能在互斥锁形成的临界区设置???