The Rotation Game
Time Limit: 15000MS | Memory Limit: 150000K | |
Total Submissions: 6062 | Accepted: 2039 |
Description
The rotation game uses a # shaped board, which can hold 24 pieces of square blocks (see Fig.1). The blocks are marked with symbols 1, 2 and 3, with exactly 8 pieces of each kind.
Initially, the blocks are placed on the board randomly. Your task is to move the blocks so that the eight blocks placed in the center square have the same symbol marked. There is only one type of valid move, which is to rotate one of the four lines, each consisting of seven blocks. That is, six blocks in the line are moved towards the head by one block and the head block is moved to the end of the line. The eight possible moves are marked with capital letters A to H. Figure 1 illustrates two consecutive moves, move A and move C from some initial configuration.
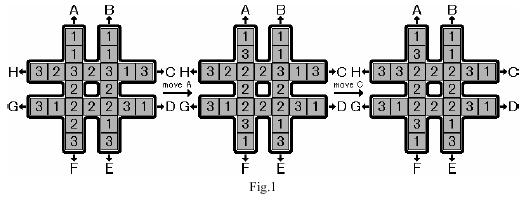
Initially, the blocks are placed on the board randomly. Your task is to move the blocks so that the eight blocks placed in the center square have the same symbol marked. There is only one type of valid move, which is to rotate one of the four lines, each consisting of seven blocks. That is, six blocks in the line are moved towards the head by one block and the head block is moved to the end of the line. The eight possible moves are marked with capital letters A to H. Figure 1 illustrates two consecutive moves, move A and move C from some initial configuration.
Input
The input consists of no more than 30 test cases. Each test case has only one line that contains 24 numbers, which are the symbols of the blocks in the initial configuration. The rows of blocks are listed from top to bottom. For each row the blocks are listed from left to right. The numbers are separated by spaces. For example, the first test case in the sample input corresponds to the initial configuration in Fig.1. There are no blank lines between cases. There is a line containing a single `0' after the last test case that ends the input.
Output
For each test case, you must output two lines. The first line contains all the moves needed to reach the final configuration. Each move is a letter, ranging from `A' to `H', and there should not be any spaces between the letters in the line. If no moves are needed, output `No moves needed' instead. In the second line, you must output the symbol of the blocks in the center square after these moves. If there are several possible solutions, you must output the one that uses the least number of moves. If there is still more than one possible solution, you must output the solution that is smallest in dictionary order for the letters of the moves. There is no need to output blank lines between cases.
Sample Input
1 1 1 1 3 2 3 2 3 1 3 2 2 3 1 2 2 2 3 1 2 1 3 3 1 1 1 1 1 1 1 1 2 2 2 2 2 2 2 2 3 3 3 3 3 3 3 3 0
Sample Output
AC 2 DDHH 2
题目大意:
给你一个游戏初始状态(从上到下,从左到右顺序给出),其中包含两个横轴,两个竖轴,你可以进行向上转,向下转,向左转,向右转操作(一次旋转一个横轴或竖轴),你的任务是找到一个最短的操作序列,使中间3*3的方格中只有一种数字(除了中央的那个空方格),如果有多个长度相等的序列,输出字典序最小的那个,如果不需要操作,输出"No moves needed"。然后还要输出中间区域的数字。
数据保证游戏中只有1,2,3这几个数字且每个出现8次。
于是我们大吼一声,这不就是搜索吗?
那么问题来了,本题一共有24个格子,状态数即有个C(24,8)*C(16,8)=735471*12870个,BFS肯定是要MLE的,这时就要用到在空间上完爆BFS的迭代加深搜索了,再加上这里状态判重太难,输入保证有解,时间限制又给了15s,简直是天助。
1.加上一个估价函数H()=中间8个格子中最多的数字凑成答案的最理想步数剪枝即可,(当前步数+H()后大于深度限 制,那么无论怎么转也不能在深度限制下凑出答案。)
2.在实现时手工打出8种旋转方案,便于保证字典序。
#include<cstdio>
#include<iostream>
#include<cstring>
#include<algorithm>
using namespace std;
#define MAXN 7
#define MAXM
#define INF 0x3f3f3f3f
typedef long long int LL;
const int Input_rule[8][8]={
{0,0,0,0,0,0,0,0},
{2,3,5,0,0,0,0,0},
{2,3,5,0,0,0,0,0},
{7,1,2,3,4,5,6,7},
{2,3,5,0,0,0,0,0},
{7,1,2,3,4,5,6,7},
{2,3,5,0,0,0,0,0},
{2,3,5,0,0,0,0,0},
};
const int rule[9][3]={
{0,0,0},
{3,1,0},
{5,1,0},
{3,0,1},
{5,0,1},
{5,1,1},
{3,1,1},
{5,0,0},
{3,0,0}
};
const int back[9]={0,6,5,8,7,2,1,4,3};
const char opp[9]={'0','A','B','C','D','E','F','G','H'};
int map[MAXN+5][MAXN+5];
char road[100];int cnt=0;
int N;
int h()
{
int num[5];
memset(num,0,sizeof(num));
for(int i=3;i<=5;++i)
for(int j=3;j<=5;++j)
if(!(i==4&&j==4))++num[map[i][j]];
int Smax=-INF;
for(int i=1;i<=3;++i)
Smax=max(Smax,num[i]);
return 8-Smax;
}
void rotate(int now)
{
bool CorR=rule[now][1],dir=rule[now][2];
if(CorR)
{
int col=rule[now][0];
if(dir==0)
{
for(int i=0;i<N;++i)
map[i][col]=map[i+1][col];
map[N][col]=map[0][col];
map[0][col]=0;
}
else
{
for(int i=N+1;i>1;--i)
map[i][col]=map[i-1][col];
map[1][col]=map[N+1][col];
map[N+1][col]=0;
}
}
else
{
int row=rule[now][0];
if(dir==0)
{
for(int i=0;i<N;++i)
map[row][i]=map[row][i+1];
map[row][N]=map[row][0];
map[row][0]=0;
}
else
{
for(int i=N+1;i>1;--i)
map[row][i]=map[row][i-1];
map[row][1]=map[row][N+1];
map[row][N+1]=0;
}
}
}
bool dfs(int dep,int &Maxdep)
{
if(dep+h()>Maxdep)return 0;
if(h()==0)return 1;
for(int i=1;i<9;++i)
{
rotate(i);
road[++cnt]=opp[i];
if(dfs(dep+1,Maxdep))return 1;
--cnt;
rotate(back[i]);
}
return 0;
}
int main()
{
N=7;
int i,j,n;
while(~scanf("%d",&map[1][3])&&map[1][3])
{
cnt=0;
n=2;
i=1;
while(i<=N)
{
j=Input_rule[i][n++];
scanf("%d",&map[i][j]);
if(n>Input_rule[i][0])
{
n-=Input_rule[i][0];
++i;
}
}
int ans;
for(ans=0;;++ans)
if(dfs(0,ans))break;
if(ans==0)puts("No moves needed");
else
{
for(i=1;i<=cnt;++i)
putchar(road[i]);
puts("");
}
printf("%d\n",map[3][3]);
}
}