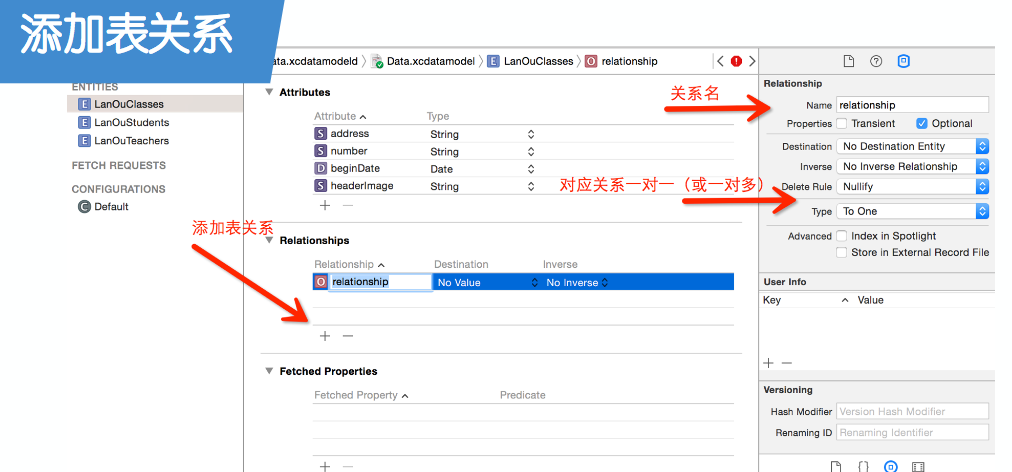
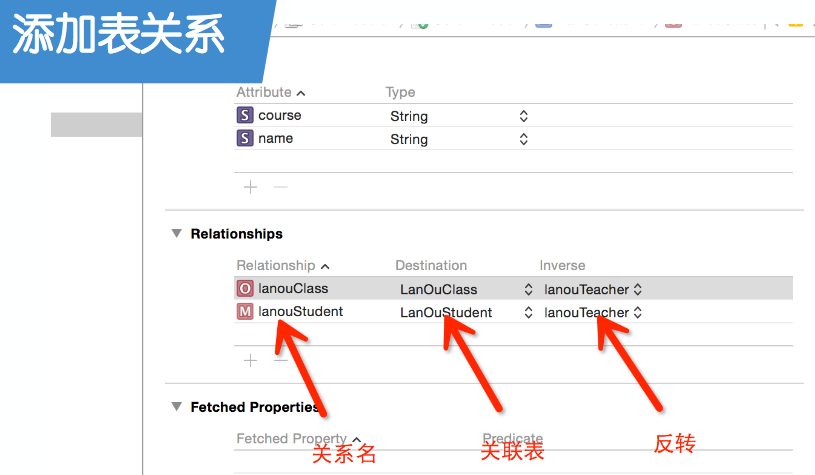
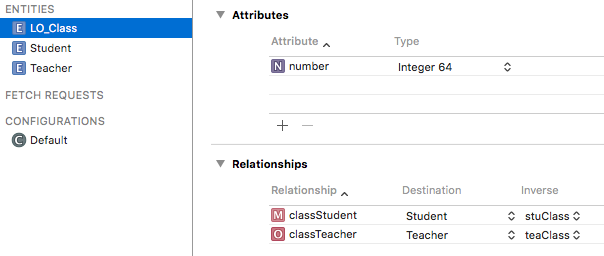
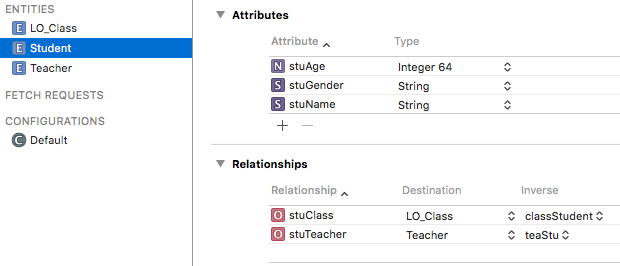
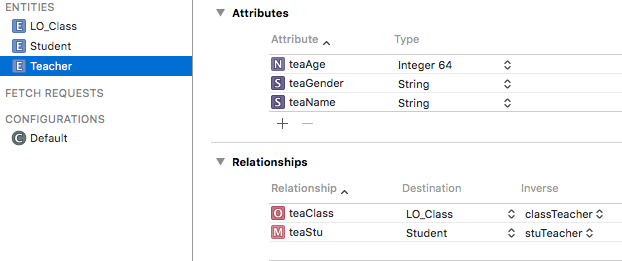
-barButtonItemClicked方法里
//Class
NSEntityDescription*classDescription = [NSEntityDescriptionentityForName:@"LO_Class"inManagedObjectContext:self.objectContext];
LO_Class*myClass = [[LO_Classalloc]initWithEntity:classDescriptioninsertIntoManagedObjectContext:self.objectContext];
intnumber =arc4random() % 100;
myClass.number= [NSNumbernumberWithInteger:number];
//Teacher
NSEntityDescription*teacherDescription = [NSEntityDescriptionentityForName:@"Teacher"inManagedObjectContext:self.objectContext];
Teacher*teacher = [[Teacheralloc]initWithEntity:teacherDescriptioninsertIntoManagedObjectContext:self.objectContext];
teacher.teaName=@"萌萌";
teacher.teaAge= [NSNumbernumberWithInteger:18];
teacher.teaGender=@"男";
//将老师和班级建立连接
myClass.classTeacher= teacher;
teacher.teaClass= myClass;
//Student
inta =arc4random() % 50 + 30;
for(inti = 0; i < a; i++) {
NSEntityDescription*studentDescription = [NSEntityDescriptionentityForName:@"Student"inManagedObjectContext:self.objectContext];
Student*student = [[Studentalloc]initWithEntity:studentDescriptioninsertIntoManagedObjectContext:self.objectContext];
student.stuName= [NSStringstringWithFormat:@"王震威%d号",i];
student.stuAge= [NSNumbernumberWithInteger:18];
NSArray*arr = @[@"男",@"女",@"王德亮"];
intgender =arc4random() % arr.count;
student.stuGender= arr[gender];
//学生和班级,老师建立联系
student.stuClass= myClass;
student.stuTeacher= teacher;
//让班级数据和学生建立一个一对多的关系,多次进行添加
[myClassaddClassStudentObject:student];
//让老师数据和学生建立一个一对多的关系,多次进行添加
[teacheraddTeaStuObject:student];
}
总结
CoreData数据库框架的实现核心是持久化存储栈
与CoreData数据库框架的所有交互都是通过NSManagedObjectContext完成的
NSManagedObjectContext中的数据是缓存在内存中的副本,要想达到持久化目的必须更新保存
代码:
#import"ViewController.h"
#import"Student.h"
#import"Teacher.h"
#import"LO_Class.h"
#import
#import"AppDelegate.h"
@interfaceViewController()
@property(nonatomic,strong)UITableView*tableView;
@property(nonatomic,strong)NSManagedObjectContext*objectContext;
@property(nonatomic,strong)NSMutableArray*dataArray;
@end
@implementationViewController
- (void)viewDidLoad {
[superviewDidLoad];
self.dataArray= [NSMutableArrayarray];
[selfsetTableView];
[selfappdelegate];
[selfsearchAll];
}
- (void)setTableView{
self.tableView= [[UITableViewalloc]initWithFrame:[UIScreenmainScreen].boundsstyle:(UITableViewStylePlain)];
[self.viewaddSubview:self.tableView];
self.tableView.dataSource=self;
self.tableView.delegate=self;
[self.tableViewregisterClass:[UITableViewCellclass]forCellReuseIdentifier:@"cell"];
self.tableView.backgroundColor= [UIColorredColor];
}
-(void)appdelegate{
AppDelegate*app = (AppDelegate*)[UIApplicationsharedApplication].delegate;
self.objectContext= app.managedObjectContext;
self.navigationItem.rightBarButtonItem= [[UIBarButtonItemalloc]initWithBarButtonSystemItem:(UIBarButtonSystemItemAdd)target:selfaction:@selector(rightBarAction)];
}
- (void)rightBarAction{
//Class
NSEntityDescription*classDescription = [NSEntityDescriptionentityForName:@"LO_Class"inManagedObjectContext:self.objectContext];
LO_Class*myClass = [[LO_Classalloc]initWithEntity:classDescriptioninsertIntoManagedObjectContext:self.objectContext];
intnumber =arc4random() % 100;
myClass.number= [NSNumbernumberWithInteger:number];
//Teacher
NSEntityDescription*teacherDescription = [NSEntityDescriptionentityForName:@"Teacher"inManagedObjectContext:self.objectContext];
Teacher*teacher = [[Teacheralloc]initWithEntity:teacherDescriptioninsertIntoManagedObjectContext:self.objectContext];
teacher.teaName=@"萌萌";
teacher.teaAge= [NSNumbernumberWithInteger:18];
teacher.teaGender=@"男";
//将老师和班级建立连接
myClass.classTeacher= teacher;
teacher.teaClass= myClass;
//Student
inta =arc4random() % 50 + 30;
for(inti = 0; i < a; i++) {
NSEntityDescription*studentDescription = [NSEntityDescriptionentityForName:@"Student"inManagedObjectContext:self.objectContext];
Student*student = [[Studentalloc]initWithEntity:studentDescriptioninsertIntoManagedObjectContext:self.objectContext];
student.stuName= [NSStringstringWithFormat:@"王震威%d号",i];
student.stuAge= [NSNumbernumberWithInteger:18];
NSArray*arr = @[@"男",@"女",@"王德亮"];
intgender =arc4random() % arr.count;
student.stuGender= arr[gender];
//学生和班级,老师建立联系
student.stuClass= myClass;
student.stuTeacher= teacher;
//让班级数据和学生建立一个一对多的关系,多次进行添加
[myClassaddClassStudentObject:student];
//让老师数据和学生建立一个一对多的关系,多次进行添加
[teacheraddTeaStuObject:student];
}
//很重要,完成save后才保存到数据库
NSError*error =nil;
[self.objectContextsave:&error];
if(error ==nil) {
NSLog(@"保存正确");
[self.dataArrayaddObject:myClass];
NSIndexPath*indexPath = [NSIndexPathindexPathForRow:self.dataArray.count-1inSection:0];
[self.tableViewinsertRowsAtIndexPaths:@[indexPath]withRowAnimation:(UITableViewRowAnimationFade)];
}
}
- (void)searchAll{
// Fetch ——CoreData 系统封装好的
NSFetchRequest*fetchRequest = [[NSFetchRequestalloc]init];
NSEntityDescription*entity = [NSEntityDescriptionentityForName:@"LO_Class"inManagedObjectContext:self.objectContext];
[fetchRequestsetEntity:entity];
//使用谓词查询,里面类似于SQL查询语句中的where条件
// LO_Class *myClass = [LO_Class new];
// NSPredicate *predicate = [NSPredicate predicateWithFormat:@"studentClass = %@", myClass];
// [fetchRequest setPredicate:predicate];
//排序(升降序)
NSSortDescriptor*sortDescriptor = [[NSSortDescriptoralloc]initWithKey:@"number"ascending:YES];
[fetchRequestsetSortDescriptors:[NSArrayarrayWithObjects:sortDescriptor,nil]];
NSError*error =nil;
NSArray*fetchedObjects = [self.objectContextexecuteFetchRequest:fetchRequesterror:&error];
if(error ==nil) {
[self.dataArraysetArray:fetchedObjects];
[self.tableViewreloadData];
}
}
//tableView必须实现方法
- (UITableViewCell*)tableView:(UITableView*)tableView cellForRowAtIndexPath:(NSIndexPath*)indexPath{
UITableViewCell*cell = [tableViewdequeueReusableCellWithIdentifier:@"cell"];
LO_Class*class =self.dataArray[indexPath.row];
Teacher*teacher = class.classTeacher;
cell.textLabel.text= [NSStringstringWithFormat:@"%@班级的%@老师",class.number,teacher.teaName];
returncell;
}
- (NSInteger)tableView:(UITableView*)tableView numberOfRowsInSection:(NSInteger)section{
returnself.dataArray.count;
}
@end