继承
- 在java中使用extends关键字来表示继承关系,例如:
class Engineer {
protected int no;
protected String name;
protected String enterTime;
public Engineer() {
super();
}
public Engineer(int no, String name, String enterTime) {
super();
this.no = no;
this.name = name;
this.enterTime = enterTime;
}
public void showInfo() {
System.out.println("[编号:" + no + ",姓名:" + name + ",入职时间:" + enterTime + "]");
}
}
class SoftEngineer extends Engineer {
private String type;
public SoftEngineer() {
super();
}
public SoftEngineer(int no, String name, String enterTime, String type) {
super(no, name, enterTime);
this.type = type;
}
@Override
public void showInfo() {
System.out.println("[编号:" + no + ",姓名:" + name + ",入职时间:" + enterTime + ",语言类型:" + type + "]");
}
public void coding() {
System.out.println(no + "号软件工程师" + name + ",正在使用" + type + "语言编写项目。");
}
}
class TestEngineer extends Engineer {
private String level;
public TestEngineer() {
super();
}
public TestEngineer(int no, String name, String enterTime, String level) {
super(no, name, enterTime);
this.level = level;
}
@Override
public void showInfo() {
System.out.println("[编号:" + no + ",姓名:" + name + ",入职时间:" + enterTime + ",等级:" + level + "]");
}
public void test() {
System.out.println(no + "号" + level + "测试工程师" + name + ",正在测试项目");
}
}
- 继承后的初始化顺序:父类属性->父类构造方法->子类属性->子类构造方法
- 有些父类成员不能继承
- 不能继承父类private成员
- 不能继承子类与父类不在同包时,默认访问权限的成员
- 不能继承构造方法
访问修饰符
访问修饰符 | 本类 | 同包 | 子类 | 其他 |
---|
private | √ | | | |
默认(friendly) | √ | √ | | |
protected | √ | √ | √ | |
public | √ | √ | √ | √ |
方法重载
- 在同一个类中定义的多个同名方法,参数列表不同(数据类型,数量,顺序)——两同一不同。
- 调用重载方法时,Java编译器能通过检查调用的方法的参数类型和个数选择一个恰当的方法。
- 方法重载通常用于创建完成一组任务相似但参数的类型或参数的个数不同的方法。
- 如,上例中构造方法的重载。
方法重写
- 方法重写的规则
- 在继承关系的子类中
- 重写的方法名、参数、返回值类型必须和父类相同
- 不能比父类更严格
- 私有的方法不能继承因而也无法重写
- 如,上例两个子类中的showInfo()方法
| 位置 | 方法名 | 参数列表 | 返回值 | 访问修饰符 |
---|
方法重写 | 子类 | 相同 | 相同 | 相同 | 不能比父类更严格 |
方法重载 | 同类 | 相同 | 不同 | 无关 | 无关 |
向上转型(多态)
- 向上转型,JAVA中的一种调用方式。向上转型是对父类的对象的方法的扩充,即父类的对象可访问子类从父类中继承来的和子类复写父类的方法。
- 例子:
public class Demo01 {
public static void main(String[] args) {
Artist a = new Artist();
Piano p = new Piano();
a.make(p);
}
}
class Artist{
public void make(Instrument instrument) {
System.out.print("音乐家正在");
instrument.play();
}
}
abstract class Instrument {
protected String brand;
protected double weight;
public Instrument() {
super();
}
public Instrument(String brand, double weight) {
super();
this.brand = brand;
this.weight = weight;
}
public abstract void play();
}
class Piano extends Instrument {
protected double size;
public Piano() {
super();
}
public Piano(String brand, double weight, double size) {
super(brand, weight);
this.size = size;
}
@Override
public void play(){
System.out.println("演奏钢琴");
}
}
class Violin extends Instrument {
protected double length;
public Violin() {
super();
}
public Violin(String brand, double weight, double length) {
super(brand, weight);
this.length = length;
}
@Override
public void play(){
System.out.println("演奏小提琴");
}
}
public class Demo02 {
public static void main(String[] args) {
Scanner reader = new Scanner(System.in);
Print print = null;
while(true){
System.out.println("请选择打印机:1、黑白打印机,2、彩色打印机");
switch (reader.next()) {
case "1":
print = new hbPrint();
break;
case "2":
print = new csPrint();
break;
}
if(print != null){
break;
} else {
System.out.println("输入有误");
}
}
System.out.println("请输入打印内容:");
String str = reader.next();
System.out.println("打印结果:");
print.printText(str);
}
}
abstract class Print{
abstract void printText(String str);
}
class hbPrint extends Print{
@Override
void printText(String str) {
System.out.println(str);
}
}
class csPrint extends Print{
@Override
void printText(String str) {
System.err.println(str);
}
}
执行效果测试
public class Demo04 {
public static void main(String[] args) {
Base base = new Child();
base.showName();
}
}
class Base{
public String name;
public Base() {
super();
System.out.println(1);
this.name = "张三";
}
public Base(String name) {
super();
System.out.println(2);
this.name = name;
}
public void showName(){
System.out.println(name);
}
}
class Child extends Base{
public Child(){
super("李四");
System.out.println(3);
}
}
- 猜测可能的输出结果:
- 预测结果:
name="李四"
- 运行结果:
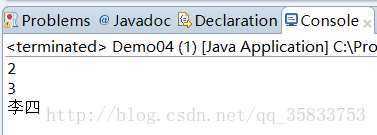
- 结论:
- 使用向上转型(父类引用指向子类对象)时,子类重写的方法会覆盖父类中的方法
- 子类中的无参构造方法可以调用父类中重载的有参构造方法
- ……