<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>旋转切换的轮播图</title>
<style>
body {
background: #000;
}
.container {
width: 200px;
height: 200px;
border-radius: 50%;
outline: 5px solid #fff;
margin: 50px auto;
display: flex;
justify-content: center;
overflow: hidden;
}
.inner {
width: 400px;
height: 400px;
border-radius: 50%;
flex-shrink: 0;
margin-top: 100px;
position: relative;
}
.inner img {
width: 200px;
height: 200px;
border-radius: 50%;
position: absolute;
margin-left: 100px;
margin-top: -100px;
transform-origin: center 300px;
}
.inner img:nth-child(1) {
transform: rotate(0deg);
}
.inner img:nth-child(2) {
transform: rotate(60deg);
}
.inner img:nth-child(3) {
transform: rotate(120deg);
}
.inner img:nth-child(4) {
transform: rotate(180deg);
}
.inner img:nth-child(5) {
transform: rotate(240deg);
}
.inner img:nth-child(6) {
transform: rotate(300deg);
}
@keyframes rotation {
12.8205128205%,
16.6666666667% {
transform: rotate(-60deg);
}
29.4871794872%,
33.3333333333% {
transform: rotate(-120deg);
}
46.1538461538%,
50% {
transform: rotate(-180deg);
}
62.8205128205%,
66.6666666667% {
transform: rotate(-240deg);
}
79.4871794872%,
83.3333333333% {
transform: rotate(-300deg);
}
96.1538461538%,
100% {
transform: rotate(-360deg);
}
}
.inner {
animation: rotation 7.8s ease-in infinite;
}
</style>
</head>
<body>
<div class="container">
<div class="inner">
<img src="https://picsum.photos/id/51/600" />
<img src="https://picsum.photos/id/52/600" />
<img src="https://picsum.photos/id/53/600" />
<img src="https://picsum.photos/id/54/600" />
<img src="https://picsum.photos/id/55/600" />
<img src="https://picsum.photos/id/56/600" />
</div>
</div>
</body>
</html>
<!--
@use 'sass:math';
body {
background: #000;
}
$size: 200px; //大小
$n: 6; // 图片个数
$pDeg: 360deg / $n; //每个扇形的角度
$r: $size/2;
$R: $r/math.sin($pDeg/2);
$innerSize: $R * 2;
.container {
width: $size;
height: $size;
border-radius: 50%;
outline: 5px solid #fff;
margin: 50px auto;
display: flex;
justify-content: center;
overflow: hidden;
}
.inner {
width: $innerSize;
height: $innerSize;
border-radius: 50%;
flex-shrink: 0;
margin-top: $r;
position: relative;
img {
width: $size;
height: $size;
border-radius: 50%;
position: absolute;
margin-left: $r;
margin-top: -$r;
transform-origin: center #{$R + $r};
@for $i from 1 through $n {
&:nth-child(#{$i}) {
transform: rotate($pDeg * ($i - 1));
}
}
}
}
$u: 1 / $n * 100%; //动画每个阶段经历的时间百分比
$rotateDuration: 0.3s; //每张图片旋转的时间
$stopDuration: 1s; //每张图片停留的时间
$duration: ($rotateDuration + $stopDuration) * $n;
$backPercent: $rotateDuration/($rotateDuration + $stopDuration) * $u;
@keyframes rotation {
@for $i from 1 through $n {
$p: $u * $i;
$deg: $pDeg * $i;
#{$p - $backPercent},
#{$p} {
transform: rotate(-$deg);
}
}
}
.inner {
animation: rotation $duration ease-in infinite;
}
-->
7. 旋转切换的轮播图
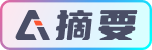