primer C++笔记
二分搜索算法
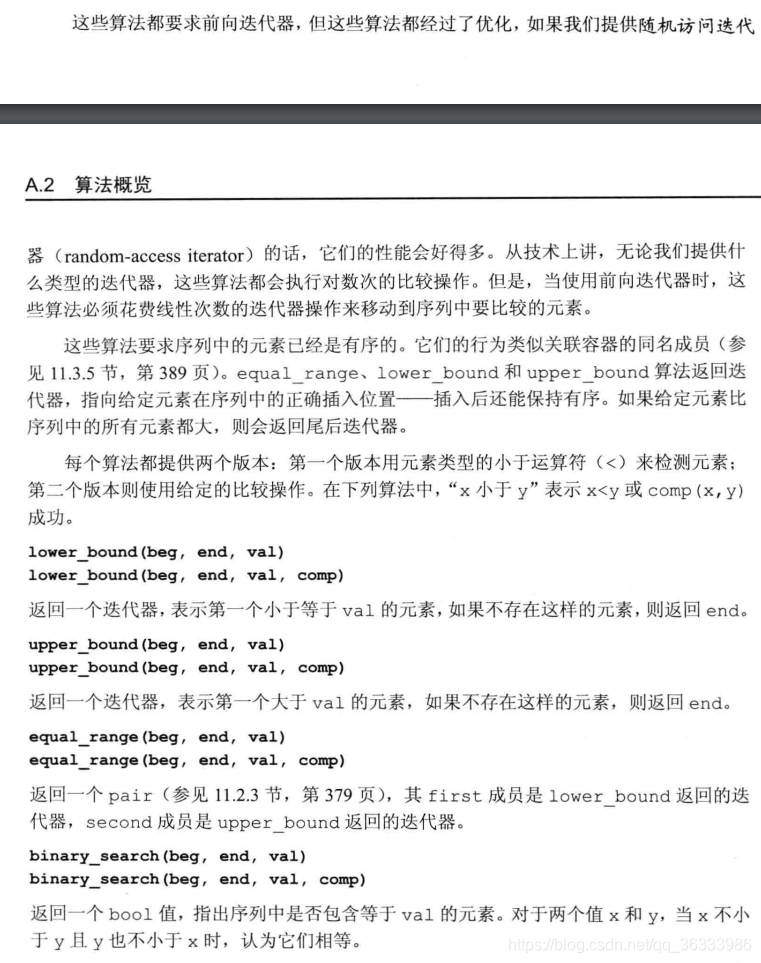
#include <iostream>
#include <stdlib.h>
#include <vector>
#include <algorithm>
#include <iterator>
using namespace std;
template<class ForwardIt, class T, class Compare = std::less<>>
ForwardIt binary_find(ForwardIt first, ForwardIt last, const T& value, Compare comp = {})
{
first = std::lower_bound(first, last, value, comp);
return first != last && !comp(value, *first) ? first : last;
}
void test01()
{
std::vector<int> data = { 1, 1, 2, 3, 3, 3, 3, 4, 4, 4, 5, 5, 6 };
auto lower = std::lower_bound(data.begin(), data.end(), 4);
auto upper = std::upper_bound(data.begin(), data.end(), 4);
std::copy(lower, upper, std::ostream_iterator<int>(std::cout, " "));
std::cout << '\n';
data = { 1, 2, 4, 6, 9, 10 };
auto it = binary_find(data.cbegin(), data.cend(), 4);
if (it != data.cend())
std::cout << *it << " found at index " << std::distance(data.cbegin(), it);
}
struct S
{
int number;
char name;
bool operator< (const S& s) const { return number < s.number; }
};
void test02()
{
std::vector<S> vec = { {1,'A'}, {2,'B'}, {2,'C'}, {2,'D'}, {4,'G'}, {3,'F'} };
S value = { 2, '?' };
auto p = std::equal_range(vec.begin(), vec.end(), value);
for (auto i = p.first; i != p.second; ++i)
std::cout << i->name << ' ';
struct Comp
{
bool operator() (const S& s, int i) const { return s.number < i; }
bool operator() (int i, const S& s) const { return i < s.number; }
};
auto p2 = std::equal_range(vec.begin(), vec.end(), 2, Comp{});
for (auto i = p2.first; i != p2.second; ++i)
std::cout << i->name << ' ';
}
void test03()
{
std::vector<int> haystack{ 1, 3, 4, 5, 9 };
std::vector<int> needles{ 1, 2, 3 };
for (auto needle : needles) {
std::cout << "Searching for " << needle << '\n';
if (std::binary_search(haystack.begin(), haystack.end(), needle)) {
std::cout << "Found " << needle << '\n';
}
else {
std::cout << "no dice!\n";
}
}
}