1. 分类
* 基本(值)类型
* String: 任意字符串
* Number: 任意的数字
* boolean: true/false
* undefined: undefined
* null: null
* 对象(引用)类型
* Object: 任意对象
* Function: 一种特别的对象(可以执行)
* Array: 一种特别的对象(数值下标, 内部数据是有序的)
2. 判断
* typeof:
* 可以判断: undefined/ 数值 / 字符串 / 布尔值 / function
* 不能判断: null与object object与array
* instanceof:
* 判断对象的具体类型
* ===
* 可以判断: undefined, null
var a
console.log(a, typeof a, typeof a==='undefined',a===undefined ) // undefined 'undefined' true true
console.log(undefined==='undefined')
a = 4
console.log(typeof a==='number')
a = 'atguigu'
console.log(typeof a==='string')
a = true
console.log(typeof a==='boolean')
a = null
console.log(typeof a, a===null) // 'object'
console.log('-----------------')
//2. 对象
var b1 = {
b2: [1, 'abc', console.log],
b3: function () {
console.log('b3')
return function () {
return 'xfzhang'
}
}
}
console.log(b1 instanceof Object, b1 instanceof Array) // true false
console.log(b1.b2 instanceof Array, b1.b2 instanceof Object) // true true
console.log(b1.b3 instanceof Function, b1.b3 instanceof Object) // true true
console.log(typeof b1.b2, '-------') // 'object'
console.log(typeof b1.b3==='function') // true
console.log(typeof b1.b2[2]==='function')
b1.b2[2](4)
console.log(b1.b3()())
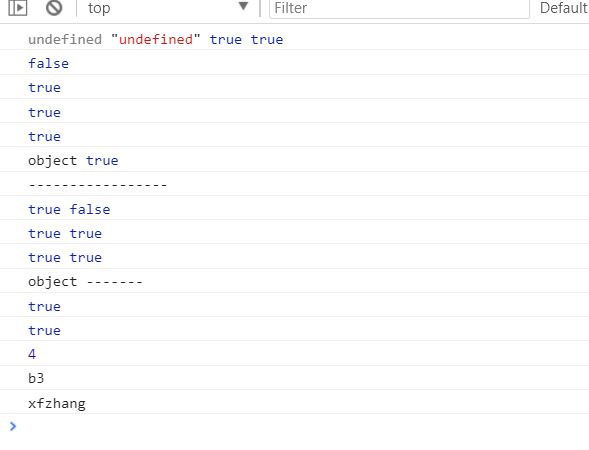
1. instanceof是如何判断的?
* 表达式: A instanceof B
* 如果B函数的显式原型对象在A对象的原型链上, 返回true, 否则返回false
2. Function是通过new自己产生的实例
/*
案例1
*/
function Foo() { }
var f1 = new Foo()
console.log(f1 instanceof Foo) // true
console.log(f1 instanceof Object) // true
/*
案例2
*/
console.log(Object instanceof Function) // true
console.log(Object instanceof Object) // true
console.log(Function instanceof Function) // true
console.log(Function instanceof Object) // true
function Foo() {}
console.log(Object instanceof Foo) // false