- 二叉树是递归定义的,每个节点在其子树上都是根节点,都有左右子树
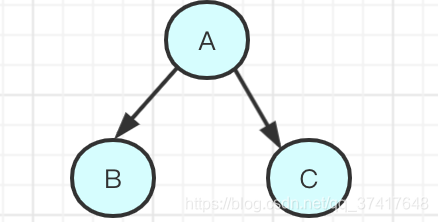
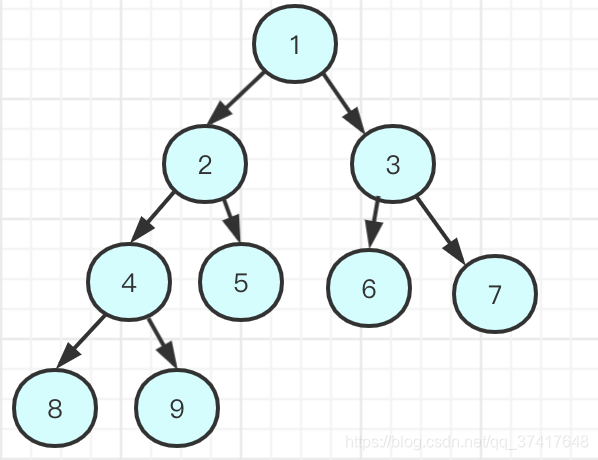
访问节点操作发生在第一次经过节点时
,根
左右,就是前序遍历访问节点操作发生在第二次经过节点时
,左根
右,就是中序遍历访问节点操作发生在第三次经过节点时
,左右根
,就是后序遍历- 所谓的遍历,就是对树中的每个节点仅仅做一次访问操作,
对根节点访问顺序
,形成了遍历顺序 - 先序:1 2 4 8 9 5 3 6 7
中序:8 4 9 2 5 1 6 3 7
后序:8 9 4 5 2 6 7 3 1 - 递归遍历 java 代码
public class Test {
public static void main(String[] args) {
TreeNode root = createTree();
preOrderRe(root);
System.out.println();
inOrderRe(root);
System.out.println();
postOrderRe(root);
System.out.println();
}
public static TreeNode createTree() {
TreeNode[] nodes = new TreeNode[10];
int i, l, r;
for (i = 1; i < 10; i++) {
nodes[i] = new TreeNode(i);
}
for (i = 1; i <= 9; i++) {
l = 2*i;
r = 2*i + 1;
if (l < 10) {
nodes[i].left = nodes[l];
}
if (r < 10) {
nodes[i].right = nodes[r];
}
}
return nodes[1];
}
public static void preOrderRe(TreeNode root) {
if (root == null) {
return;
}
root.show();
preOrderRe(root.left);
preOrderRe(root.right);
}
public static void inOrderRe(TreeNode root) {
if (root == null) {
return;
}
inOrderRe(root.left);
root.show();
inOrderRe(root.right);
}
public static void postOrderRe(TreeNode root) {
if (root == null) {
return;
}
postOrderRe(root.left);
postOrderRe(root.right);
root.show();
}
}
class TreeNode {
int value;
TreeNode left;
TreeNode right;
TreeNode(int value) {
this.value = value;
}
public void show() {
System.out.print(value + " ");
}
}
- 非递归遍历 java 代码
public static void preOrder(TreeNode root) {
Stack<TreeNode> stack = new Stack<>();
TreeNode cur = root;
while (!stack.isEmpty() || cur != null) {
if (cur != null) {
cur.show();
stack.push(cur);
cur = cur.left;
} else {
cur = stack.pop();
cur = cur.right;
}
}
}
public static void inOrder(TreeNode root) {
Stack<TreeNode> stack = new Stack<>();
TreeNode cur = root;
while (!stack.isEmpty() || cur != null) {
if (cur != null) {
stack.push(cur);
cur = cur.left;
} else {
cur = stack.pop();
cur.show();
cur = cur.right;
}
}
}
public static void postOrder(TreeNode root) {
Stack<TreeNode> stack = new Stack<>();
TreeNode cur = root, pre = null;
while (cur != null || !stack.isEmpty()) {
if (cur != null) {
stack.push(cur);
cur = cur.left;
} else {
cur = stack.peek();
if (cur.right == null || cur.right == pre) {
stack().pop().show();
pre = cur;
cur = null;
} else {
cur = cur.right;
}
}
}
}
public static void levelOrder(TreeNode root) {
if (root == null) {
return;
}
LinkedList<TreeNode> queue = new LinkedList<>();
queue.add(root);
while (!queue.isEmpty()) {
root = queue.poll();
root.show();
if (root.left != null) {
queue.add(root.left);
}
if (root.right != null) {
queue.add(root.right);
}
}
}
参考1
参考2