State
const Counter = {
template:'<div>{{count}}</div>',
computed:{
count(){
return this.$store.state.count
}
}
}
Getters
const store = new Vuex.Store({
state:{
todos:[
{id:1,text:'...',done:true},
{id:2,text:'...',done:false},
]
},
getters: {
doneTodos:state =>{
return state.todos.filter(todo=>todo.done)
}
}
})
Mutations
- 更改Vuex的store中的状态的唯一的方法是提交mutation
const store = new Vuex.Store({
state:{
count: 1
},
mutations:{
increment(state){
state.count++;
}
}
})
store.commit('increment')
Actions
- action提交的是mutation,而不是直接变更状态
- action可以包含任意异步操作
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment(state){
state.count++;
}
},
actions: {
increment(context){
context.commit('increment')
}
}
})
Modules
- 面对复杂的应用程序,当管理的状态比较多时,我们需要将Vuex的store对象分割成模块(modules)
const moduleA = {
state:{},
mutations: {},
actions: {},
getters:{},
}
const moduleB = {
state:{},
mutations: {},
actions: {},
}
const store = new Vuex.Store({
modules: {
a:moduleA,
b:moduleB
}
})
Vuex的示例之使用state
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Vuex</title>
<script src="../node_modules/vue/dist/vue.js"></script>
<script src="../node_modules/vuex/dist/vuex.min.js"></script>
</head>
<body>
<div id="app">
<h2>{{msg}}</h2>
<counter></counter>
</div>
<script>
const counter ={
template:`<div>{{count}}</div>`,
computed:{
count(){
return this.$store.state.count;
}
}
}
const storeVuex =new Vuex.Store({
state:{
count:10
}
});
new Vue({
el:"#app",
store:storeVuex,
data:{
msg:'Vuex的使用'
},
components: {
counter
}
})
</script>
</body>
</html>
对比不使用vuex
<div id="app">
<h2>{{msg}}</h2>
<counter v-bind:count="count"></counter>
</div>
<script>
const counter ={
template:`<div>{{count}}</div>`,
props:['count']
}
new Vue({
el:"#app",
data:{
count: 15,
msg:'Vuex的使用'
},
components: {
counter
}
})
</script>
运用Vuex中的mutation改变Vuex实例的状态
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Vuex</title>
<script src="../node_modules/vue/dist/vue.js"></script>
<script src="../node_modules/vuex/dist/vuex.min.js"></script>
</head>
<body>
<div id="app">
<h2>{{msg}}</h2>
<a href="javascript:;" @click="add">click</a>
<counter ></counter>
</div>
<script>
const counter ={
template:`<div>{{count}}</div>`,
computed:{
count(){
return this.$store.state.count;
}
}
}
const storeVuex =new Vuex.Store({
state:{
count:10
},
mutations: {
increment(state){
return state.count++;
}
}
});
new Vue({
el:"#app",
store:storeVuex,
data:{
msg:'Vuex的使用'
},
components: {
counter
},
methods:{
add(){
this.$store.commit('increment')
}
}
})
</script>
</body>
</html>
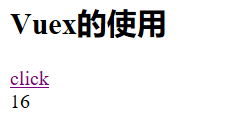
使用action
const storeVuex =new Vuex.Store({
state:{
count:10
},
mutations: {
increment(state, num){
return state.count = num;
}
},
actions:{
incrementAction(context, num){
context.commit("increment", num);
}
}
});
new Vue({
el:"#app",
store:storeVuex,
data:{
msg:'Vuex的使用'
},
components: {
counter
},
methods:{
add(){
this.$store.dispatch('incrementAction', 5)
}
}
})
getter的使用实例
<body>
<div id="app">
<h2>{{msg}}</h2>
<a href="javascript:;" @click="add">click</a>
<counter ></counter>
</div>
<script>
const counter ={
template:`<div>
<div>点击数量:{{count}}</div>
<div>用户名:{{userName}}</div>
</div>`,
computed:{
count(){
return this.$store.state.count;
},
userName(){
return this.$store.getters.userName;
}
}
}
const storeVuex =new Vuex.Store({
state:{
count:10,
name:'jack'
},
mutations: {
increment(state, num){
return state.count = num;
}
},
actions:{
incrementAction(context, num){
context.commit("increment", num);
}
},
getters:{
userName(state){
return state.name + ',Hello'
}
}
});
new Vue({
el:"#app",
store:storeVuex,
data:{
msg:'Vuex的使用'
},
components: {
counter
},
methods:{
add(){
this.$store.dispatch('incrementAction', 5)
}
}
})
</script>
</body>