最近的项目中用到了对比图,为了以后不再到处找资料,特记录下对比图的做法。
原理
对比图实际上是一组横向的柱状图,以x轴为度量,y轴为维度,与echarts官方给出的示例不同的是,一般x轴左右侧都需要是正值。这样的话可以建立三个坐标系来实现:第一个坐标系对应左侧部分(x轴设置inverse),第二个坐标系对应右侧部分,两个坐标系均隐藏y轴,第三个坐标系用来显示y轴(y轴一般放在左侧,视情况也可以放右侧)同时隐藏x轴。如图所示
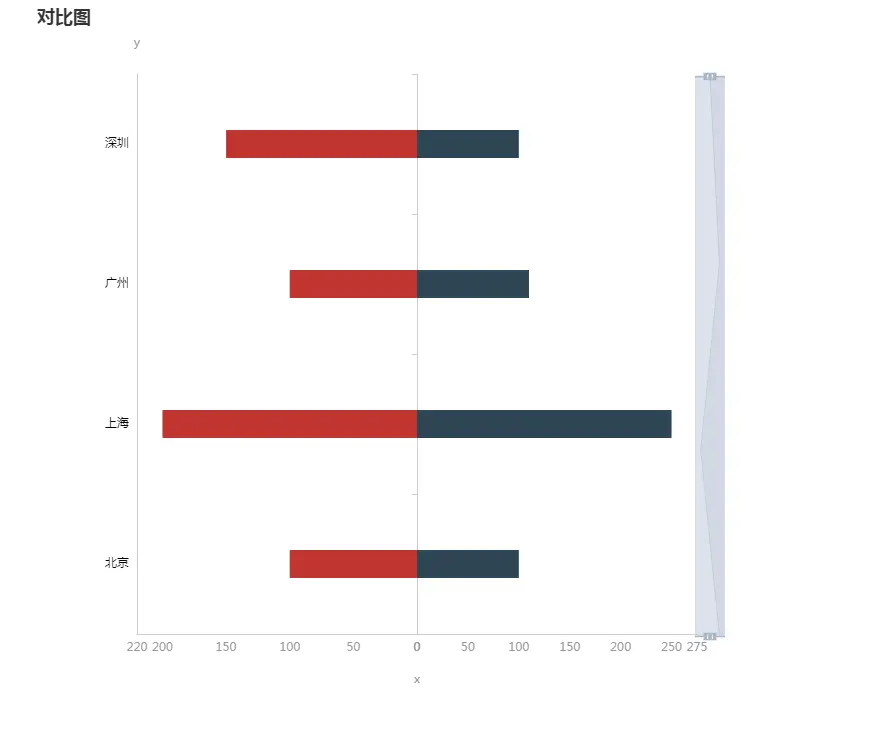
对比图
代码
const chartDom = document.getElementById('chart');
const myChart = echarts.init(chartDom);
const yData = ['北京', '上海', '广州', '深圳'];
const option = {
title: {
text: '对比图'
},
tooltip: {},
grid: [
{ // 左侧对应坐标系
show: false,
left: '15%',
top: '10%',
bottom: '10%',
width: '40%'
},
{ // 右侧对应坐标系
show: false,
left: '55%',
top: '10%',
bottom: '10%',
width: '40%'
},
{ // 用来显示的坐标系
show: false,
left: '15%',
top: '10%',
bottom: '10%',
width: '80%'
}
],
// x轴是度量,y轴是维度
xAxis: [
{ // 左侧轴
type: 'value',
inverse: true,
min: 0,
max: val => {
return Math.floor((val.max) * 1.1);
},
axisLine: {
show: true,
lineStyle: {
color: '#ccc'
}
},
axisTick: {
show: false
},
axisLabel: {
show: true,
textStyle: {
color: '#999',
fontSize: 12
}
},
splitLine: {
show: false
}
},
{ // 右侧轴
type: 'value',
gridIndex: 1,
min: 0,
max: val => {
return Math.floor((val.max) * 1.1);
},
axisLine: {
show: true,
lineStyle: {
color: '#ccc'
}
},
axisTick: {
show: false
},
axisLabel: {
show: true,
textStyle: {
color: '#999',
fontSize: 12
}
},
splitLine: {
show: false
}
},
{ // 最左侧y轴对应x轴
gridIndex: 2,
name: 'x',
nameLocation: 'center',
nameGap: 40, // 与坐标轴距离
nameTextStyle: {
color: '#999999',
fontSize: 12
},
axisLine: {
show: false
}
}
],
yAxis: [
{
type: 'category',
position: 'right',
axisLine: {
show: false
},
axisTick: {
show: false
},
axisLabel: {
show: false,
margin: 0
},
data: yData
},
{
gridIndex: 1,
type: 'category',
position: 'left',
axisLine: {
show: true,
lineStyle: {
color: '#ccc'
}
},
axisTick: {
show: true
},
axisLabel: {
show: false
},
data: yData
},
{
name: 'y',
gridIndex: 2,
type: 'category',
position: 'left',
nameLocation: 'end',
nameGap: 25, // 与坐标轴距离
nameTextStyle: {
color: '#999999',
fontSize: 12
},
axisLine: {
show: true,
lineStyle: {
color: '#ccc'
}
},
axisTick: {
show: false
},
axisLabel: {
show: true,
textStyle: {
color: '#000',
fontSize: 12
}
},
data: yData
}
],
dataZoom: [
{
type: 'slider',
yAxisIndex: [0, 1, 2],
filterMode: 'filter',
minSpan: 20
},
{
type: 'inside',
yAxisIndex: [0, 1, 2],
filterMode: 'filter',
minSpan: 20
}
],
series: [
{
name: '男',
type: 'bar',
xAxisIndex: 0,
yAxisIndex: 0,
barMaxWidth: '20%',
data: [100, 200, 100, 150]
},
{
name: '女',
type: 'bar',
xAxisIndex: 1,
yAxisIndex: 1,
barMaxWidth: '20%',
data: [100, 250, 110, 100]
}
]
};
myChart.setOption(option);
对比图中还加入了datazoom,将三个y轴联动起来。
最后有一个美中不足的地方,鼠标移入时tooltip暂时只能显示某一侧的内容,如果再增加坐标系或许能够解决...