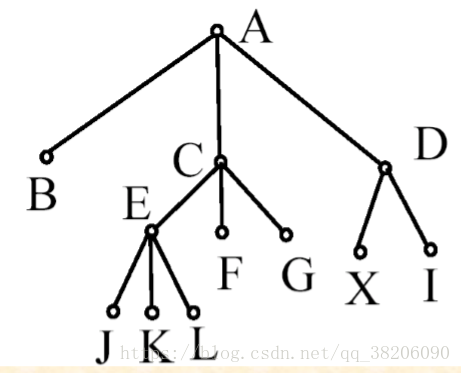
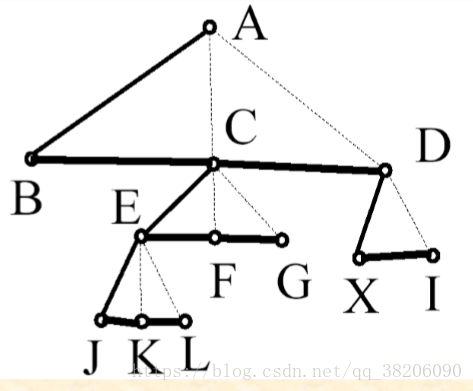
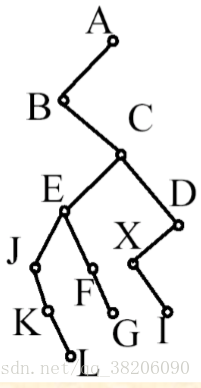
#include<bits/stdc++.h>
#define INF 99999999
using namespace std;
/*
树:
树是一种特殊的图,这种图是连通的,并且边数恰好比顶点数少一,即:树集 = { G=(V,E) :|V|=0 或 G连通且|E|=|V|-1}
*/
/*
默认0是根结点
*/
class Tree
{
public:
int vn;
int **matrix;
Tree(){ vn = 0; matrix = NULL; }
};
typedef class Node
{
public:
Node *left;
Node *right;
int val;
Node(){ left = right = NULL; }
Node(int tval){ val = tval; left = right = NULL; }
} *BinTree;
Tree *createTree()
{
int n, m, i, j, from, to;
cin >> n >> m;
Tree *tree = new Tree();
tree->vn = n;
tree->matrix = new int*[n];
for(i = 0; i < n; i++)
{
tree->matrix[i] = new int[n];
fill(tree->matrix[i], tree->matrix[i] + n, INF);
}
for(i = 0; i < m; i++)
{
cin >> from >> n;
for(j = 0; j < n; j++)
{
cin >> to;
tree->matrix[from][to] = 1;
}
}
return tree;
}
BinTree TreeToBinTree(Tree *tree)
{
int n = tree->vn;
int i, j, cnt = 0;
BinTree *arr = new BinTree[n];
for(int i = 0; i < n; i++)
arr[i] = new Node(i);
for(i = 0; i < n; i++)
{
int from = -1;
for(j = 0; j < n; j++)
if(tree->matrix[i][j] == 1)
{
if(from != -1)
arr[from]->right = arr[j];
else
arr[i]->left = arr[j];
from = j;
}
}
return arr[0];
}
void print_preOrder_recursion(BinTree root)
{
if(root != NULL)
{
cout << root->val << " ";
print_preOrder_recursion(root->left);
print_preOrder_recursion(root->right);
}
}
void print_inOrder_recursion(BinTree root)
{
if(root != NULL)
{
print_inOrder_recursion(root->left);
cout << root->val << " ";
print_inOrder_recursion(root->right);
}
}
int main()
{
Tree *tree = createTree();
BinTree root = TreeToBinTree(tree);
print_preOrder_recursion(root);
cout << endl;
print_inOrder_recursion(root);
return 0;
}
/*
树:
//第一行是结点个数N和非叶结点个数M,下面M行数据:非叶结点编号、当前结点的孩子个数、孩子结点编号...
12 4
0 3 1 2 3
2 3 4 5 6
3 2 10 11
4 3 7 8 9
pre: 0 1 2 4 7 8 9 5 6 3 10 11
in: 1 7 8 9 4 5 6 2 10 11 3 0
*/