1布局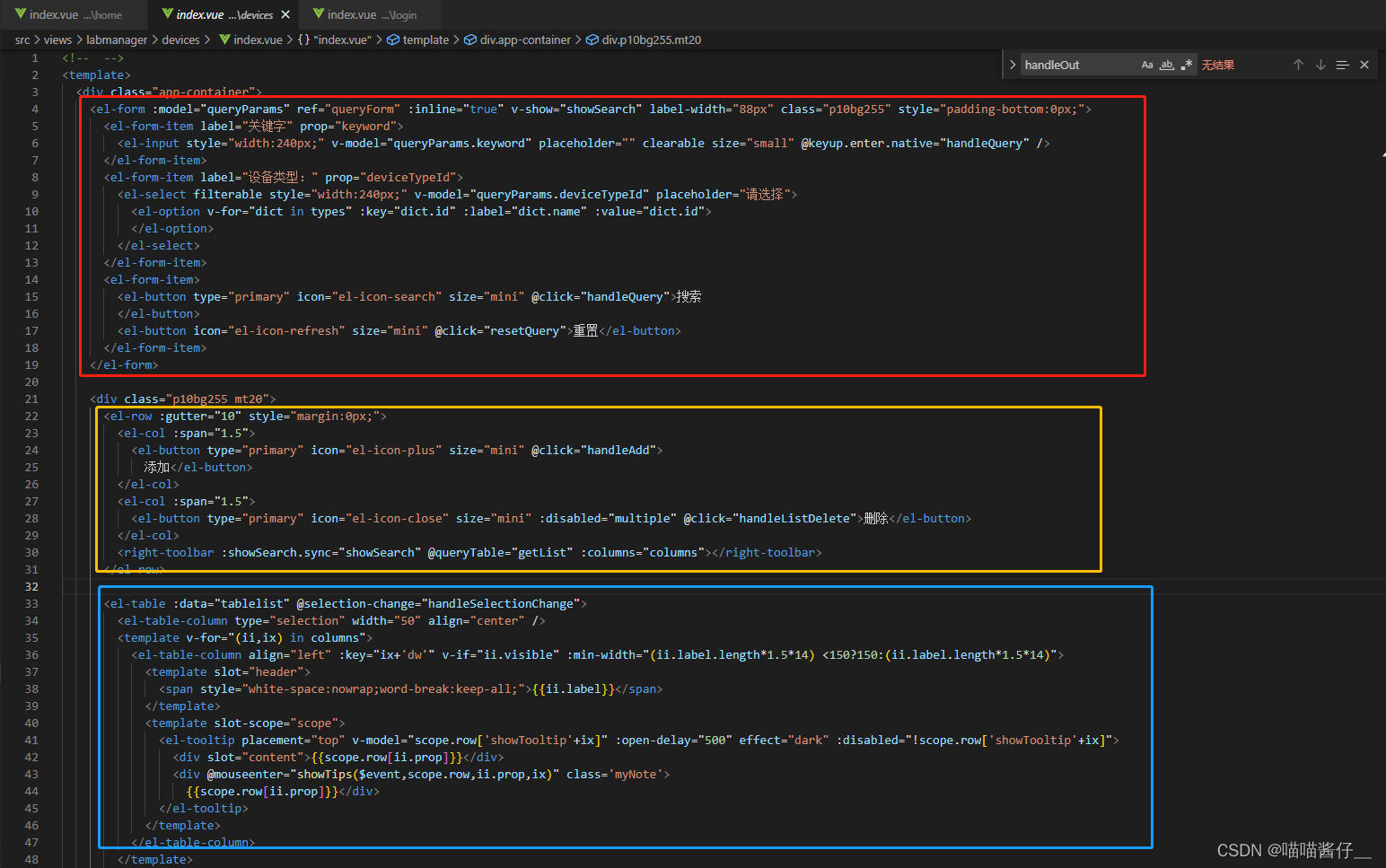
data(){
return{
queryParams: {
pageNum: 1,
pageSize: 10,
keyword: undefined,
deviceTypeId: undefined,
},
total: 0,
tablelist: [],
types: [],
}
},
mounted() {
this.getList()
deviceTypeList().then(res => {
console.log(res)
this.types = res.data
})
},
methods:{
handleQuery() {
this.getList()
},
resetQuery() {
this.resetForm("queryForm");
this.handleQuery();
},
getList() {
// 获取分页列表
devicePage(this.queryParams).then((res) => {
this.tablelist = res.data.list || []
this.total = res.data.total || 0
})
},
}
2 多选框
获取id:
<el-table :data="tablelist" @selection-change="handleSelectionChange">
<el-table-column type="selection" width="50" align="center" />
</el-table-column>
</el-table>
// 多选框选中数据
handleSelectionChange(selection) {
this.ids = selection.map(item => item.id);
this.single = selection.length != 1;
this.multiple = !selection.length;
},
multiple控制是否展示批量删除按钮
<el-button type="primary" icon="el-icon-close" size="mini" :disabled="multiple" @click="handleListDelete">删除</el-button>
2.2 批量删除和 删除用的一个方法
<el-button type="primary" icon="el-icon-close" size="mini" :disabled="multiple" @click="handleListDelete">批量删除</el-button>
<el-button size="mini" type="text" icon="el-icon-delete" @click="handleListDelete(scope.row)">删除</el-button>
handleListDelete(e) {
let that = this
this.$confirm('是否确认删除该设备?', "警告", {
confirmButtonText: "确定",
cancelButtonText: "取消",
type: "warning"
}).then(function () {
return deviceDeleteBatch(('id' in e) ? [e.id] : that.ids);
}).then(() => {
this.getList();
this.msgSuccess("删除成功");
})
},
3 跳转详情页
http://localhost:8080/#/lab/dev/info?id=123
两种跳转:
1 还在当前二级路由 router.push
2 跳新的一页 window.open
【问题描述】
路由模式从 hash 模式换为 history 模式 window.open() 不能跳转了
【解决】
借助 this.$router.resolve() 方法 给路径拼接上 #/ 。
const { href } = this.$router.resolve({path:`/home/?aaa=${ev.aaa}&bbb=${ev.bbb}`})
window.open(href, '_blank') // _blank 打开新的页面
2 vue 路由跳转方式的几种方法
4 编辑
<el-button size="mini" type="text" icon="el-icon-edit" @click="handleEdit(scope.row)">编辑</el-button>
handleEdit(e) {
this.$router.push({ path: '/lab/dev/update', query: { id: e.id } })
},
http://localhost:8080/#/lab/dev/update?id=123
5 添加
<el-button type="primary" icon="el-icon-plus" size="mini" @click="handleAdd"> 添加</el-button>
handleAdd() {
this.$router.push({ path: '/lab/dev/add' })
},
http://localhost:8080/#/lab/dev/add
5.1 时间
<el-form-item label="出厂日期:">
<el-date-picker style="width:100%;" v-model="form.productionTime" type="date" format="yyyy-MM-dd" value-format="yyyy-MM-dd" placeholder="选择出厂日期:">
</el-date-picker>
</el-form-item>
5.2 上传图片
<el-form-item label="照片:" prop="photo">
<el-upload class="avatar-uploader" action="" :auto-upload="false" ref="upload" :show-file-list="false" accept="image/*" :on-change="imageChange">
<img v-if="imgurl" :src="imgurl" class="avatar">
<i v-else class="el-icon-plus avatar-uploader-icon"></i>
</el-upload>
<div>支持jpg和png格式,小于2M,建议比例16:9</div>
</el-form-item>
传递流给后台,所以不用action,使用on-change
imageChange: function (res, fileList) {
const file = fileList[fileList.length - 1].raw;
const fileSize = file.size;
let fileExtension = '';
let fileName = '';
if (file.name.lastIndexOf('.') > -1) {
fileExtension = file.name.slice(file.name.lastIndexOf('.') + 1);
fileName = file.name.split('.')[0];
}
if (!fileSize) {
this.$message.error('无效的文件,请重新选择!');
return;
}
if (fileSize / 1024 / 1024 > 2) {
this.$message.error('上传文件大小不能超过 2MB!');
return;
}
if (fileExtension != 'jpg' && fileExtension != 'png' && fileExtension != 'jpeg') {
this.$message.error('支持jpg和png格式!');
return;
}
let params = new FormData();
params.append('fileName', file);
let that = this
const reader = new FileReader() // 创建读取文件对象
reader.readAsDataURL(file) // 发起异步请求,读取文件
reader.onload = function () { // 文件读取完成后
that.imgurl = reader.result
}
uploadFileToFast(params).then((res) => {
console.log(res);
this.form.photo = res;
this.msgSuccess("上传成功");
}).catch((e) => {
});
this.$refs.upload.clearFiles();
},
接口:
export function uploadFileToFast(params, config, cancelFun) {
// 上传文件
return request({
url: '/file/uploadFileToFast',
method: 'post',
headers: {
"Content-Type": "multipart/form-data",
Authorization: getToken()
},
data: params,
onUploadProgress: config,
cancelToken:cancelFun
})
}
5.3 上传附件
<el-form-item label="上传附件:">
<Upload @fileback="fileBack"></Upload>
</el-form-item>
<el-form-item label="已传附件:" v-if="form.files.length">
<div v-for="(ii,ix) in form.files" :key="ix" class="flx">
<i class="el-icon-s-order"></i>
<span style="margin-left:15px">{{ii.name}}</span>
<i class="el-icon-close" style="margin-left:15px" @click="deleteFile(ii,ix)"></i>
</div>
</el-form-item>
fileBack (value) {
this.form.files.push(value);
},
deleteFile (i, x) {
this.form.files.splice(x, 1)
},
上传组件
<template>
<div>
<el-upload action="#" :limit="1" :on-change="fileChange" :auto-upload="false" ref="files">
<div>
<el-button size="small" :type="title==='点击上传'?'primary':'text'">{{title}}</el-button>
</div>
</el-upload>
<div class="fl">
<el-progress :percentage="processNum" style="width: 200px;" v-if="processNum">
</el-progress>
<i class="el-icon-close" style="margin-left:15px" v-if="processNum"
@click="cancleUpload()"></i>
</div>
</div>
</template>
<script>
import { uploadFileToFast } from '@/api/utils.js';
export default {
name: 'singleUpload',
data() {
return {
processNum: 0,
source: undefined
}
},
props: {
folderId: {
type: Number,
default: 0
},
title: {
type: String,
default: '点击上传'
},
limit:{
type:String,
default:''
}
},
computed: {
},
methods: {
fileChange(res, fileList) {
const file = fileList[fileList.length - 1].raw;
const fileSize = file.size;
let fileExtension = '';
let fileName = '';
if (file.name.lastIndexOf('.') > -1) {
fileExtension = file.name.slice(file.name.lastIndexOf('.') + 1);
fileName = file.name.substring(0, file.name.lastIndexOf("."))
}
if (!fileSize) {
this.$message.error('无效的文件,请重新选择!');
this.$refs.files.clearFiles();
return;
}
if (this.limit != '') {
if (fileExtension != 'png' && fileExtension != 'jpg' && fileExtension != 'mp4') {
this.$message.error('只允许上传png,jpg,mp4');
this.$refs.files.clearFiles();
return
}
}
let params = new FormData();
params.append('fileName', file);
this.processNum = 1
let _processCallback = (progressEvent) => {
this.processNum = (progressEvent.loaded / progressEvent.total * 100 | 0)
if (this.processNum >= 100) {
this.processNum = 99
}
}
this.source = this.$axios.CancelToken.source();
uploadFileToFast(params, _processCallback, this.source.token).then((res) => {
this.processNum = 0
let fileP = {
name: fileName,
size: this.filterSize(fileSize),
type: fileExtension,
url: res,
};
if (this.folderId) {
fileP.folderId = this.folderId
}
this.$emit('fileback', fileP)
this.msgSuccess("上传成功");
}).catch((e) => {
this.processNum = 0
if (this.$axios.isCancel(e)) {
} else {
// 处理错误
this.$message.error("上传失败");
}
});
this.$refs.files.clearFiles();
},
cancleUpload() {
if (this.source) {
this.source.cancel('取消上传');
this.source = undefined
}
}
}
}
</script>
<style>
</style>
接口:
export function uploadFileToFast(params, config, cancelFun) {
// 上传文件
return request({
url: '/file/uploadFileToFast',
method: 'post',
headers: {
"Content-Type": "multipart/form-data",
Authorization: getToken()
},
data: params,
onUploadProgress: config,
cancelToken:cancelFun
})
}
5.4 取消按钮
cancel () { this.$router.go(-1) },
6 下拉框多选
<el-form-item label="实验室" prop="labId">
<el-select filterable style="width:240px;" v-model="queryParams.labId" placeholder="全部" multiple clearable size="small">
<el-option v-for="dict in labs" :key="dict.id" :label="dict.name" :value="dict.id" />
</el-select>
</el-form-item>
multiple clearable
7 枚举
<el-form-item label="课程状态" prop="lessonStatus">
<el-select filterable style="width:240px;" v-model="queryParams.lessonStatus" placeholder="全部" clearable size="small">
<el-option v-for="dict in lessonStatuses" :key="dict.id" :label="dict.name" :value="dict.id" />
</el-select>
</el-form-item>
lessonStatuses: [
{ id: 0, name: '未开始' },
{ id: 1, name: '进行中' },
{ id: 2, name: '已结束' }
],
8导入
<el-col :span="1.5">
<el-upload action="#" :limit="1" :auto-upload="false" :show-file-list="false" accept=".csv, application/vnd.ms-excel, application/vnd.openxmlformats-officedocument.spreadsheetml.sheet" ref="upload" :on-change="fileUpdateChange">
<el-button type="primary" icon="el-icon-upload2" size="mini">导入
</el-button>
</el-upload>
</el-col>
<el-col :span="1.5">
<el-button type="primary" icon="el-icon-download" size="mini" @click="handleOut">
导出
</el-button>
</el-col>
<el-col :span="1.5">
<el-button type="primary" icon="el-icon-download" size="mini" @click="handleDownTemplate">
下载模板</el-button>
</el-col>
fileUpdateChange(res, fileList) {
const file = fileList[fileList.length - 1].raw;
const fileSize = file.size;
let fileExtension = '';
let fileName = '';
if (file.name.lastIndexOf('.') > -1) {
fileExtension = file.name.slice(file.name.lastIndexOf('.') + 1);
fileName = file.name.split('.')[0];
}
if (!fileSize) {
this.$message.error('无效的文件,请重新选择!');
return;
}
if (fileSize / 1024 / 1024 > 20) {
this.$message.error('上传文件大小不能超过 10MB!');
return;
}
if (fileExtension != 'xls' && fileExtension != 'xlsx') {
this.$message.error('必须为excel文件!');
return;
}
let params = new FormData();
params.append('fileName', file);
const loading = this.$loading({
lock: true,
text: 'Loading',
background: 'rgba(0, 0, 0, 0.7)'
});
uploadFileToFast(params).then((res) => {
console.log(res);
loading.close()
appointmentImport({ url: res }).then(e => {
this.msgSuccess(e.data);
})
});
this.$refs.upload.clearFiles();
},
export function uploadFileToFast(params, config, cancelFun) {
// 上传文件
return request({
url: '/file/uploadFileToFast',
method: 'post',
headers: {
"Content-Type": "multipart/form-data",
Authorization: getToken()
},
data: params,
onUploadProgress: config,
cancelToken:cancelFun
})
}
9 导出
<el-col :span="1.5">
<el-button type="primary" icon="el-icon-download" size="mini" @click="handleOut">
导出
</el-button>
</el-col>
后台做导出,只需要a链接跳转即可
handleOut() {
let that = this
this.$confirm('确认要导出教学计划吗?', "", {
confirmButtonText: "确定",
cancelButtonText: "取消",
type: "warning"
}).then(() => {
var a = document.createElement("a");
let url = this.setParam(process.env.BASE_API + '/appointment/exportappointment', this.queryParams)
a.setAttribute("href", url);
a.setAttribute("target", "_blank");
a.click();
})
},
10 下载模板
<el-col :span="1.5">
<el-button type="primary" icon="el-icon-download" size="mini" @click="handleDownTemplate">
下载模板</el-button>
</el-col>
handleDownTemplate() {
this.$confirm('确认要下载该模板吗?', "", {
confirmButtonText: "确定",
cancelButtonText: "取消",
type: "warning"
}).then(() => {
var a = document.createElement("a");
let downUrl = process.env.BASE_API + '/appointment/exportAppointmentExcel'
a.setAttribute("href", downUrl);
a.setAttribute("target", "_blank");
a.click();
})
},
11 查看详情 ,编辑
11.1 radio
<el-form-item label="是否关联课程:">
<el-radio v-model="form.relation" :label="1">是</el-radio>
<el-radio v-model="form.relation" :label="0"> 否</el-radio>
</el-form-item>
11.2 VUE插件vue-treeselect的使用
首先需要安装:
npm install --save @riophae/vue-treeselect
<el-form-item label="作业班级:" prop="classIds">
<treeselect showSearch v-model="form.classIds" :options="classIds"
:disable-branch-nodes='true' :multiple="true"
:normalizer="normalizer" placeholder="全部" />
</el-form-item>
11.3 隐藏显示