系列文章
SpringBoot 01 —— HelloSpringBoot、yaml配置、数据校验、多环境切换
SpringBoot 02 —— Web简单探究、员工管理系统
SpringBoot 03 —— Spring Security
SpringBoot 04 —— Shiro
SpringBoot 05 —— Swagger
SpringBoot 06 —— 异步任务、邮件任务、定时任务
SpringBoot 07 —— 分布式:Dubbo+Zookeeper
1、异步任务
当我们在网页中发起某个请求,而请求处理需要耗费一定时间时,我们常常会用异步任务来完成。这其实类似于多线程处理,我们会先给用户一个反馈,然后在后台进行处理。
未使用异步任务
1、service/AysnService.java
@RestController
public class AsyncController {
@Autowired
AsyncService asyncService;
@RequestMapping("/request")
public String request(){
asyncService.hello();//会睡眠三秒,页面持续转圈。
return "处理完毕!";
}
}
2、controller/AsyncController.java
@RestController
public class AsyncController {
@Autowired
AsyncService asyncService;
@RequestMapping("/request")
public String request(){
asyncService.hello();//会睡眠三秒,页面持续转圈。
return "处理完毕!";
}
}
3、启动后,要3秒才能得到结果。
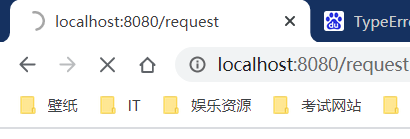
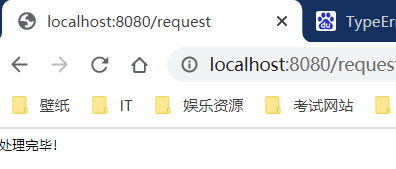
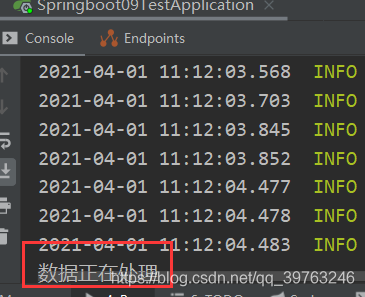
使用异步任务
会开启一个线程池,用另一个线程执行异步方法。
1、在启动程序开启异步
@EnableAsync //开启异步
@SpringBootApplication
public class Springboot09TestApplication {
public static void main(String[] args) {
SpringApplication.run(Springboot09TestApplication.class, args);
}
}
2、把方法改为异步方法
@Service
public class AsyncService {
@Async//异步方法
public void hello(){
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("数据正在处理");
}
}
3、再次运行后,立即得到反馈,后台会继续处理数据。
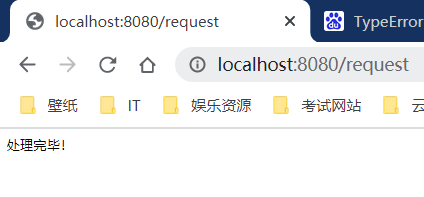
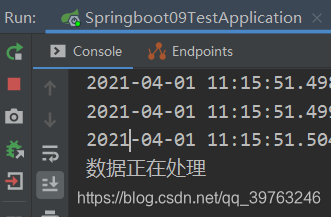
2、邮件任务
1、导入邮件启动器
<!-- 里面就导入了javax.mail -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
2、开启邮箱POP3/SMTP服务
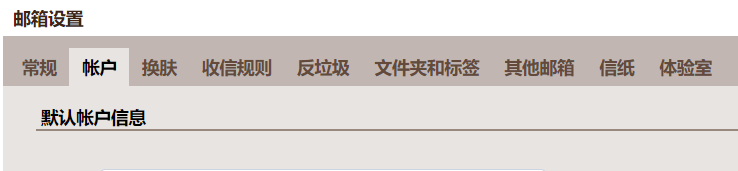
开启后,会得到一个密码

3、application.properties配置邮件服务
# 发送方邮箱
spring.mail.username=xxxx@qq.com
# POP3/SMTP服务 服务器邮箱的密码
spring.mail.password=这里填自己获取到的密码
# 邮件主机 QQ邮箱
spring.mail.host=smtp.qq.com
# QQ邮箱 需要开启加密验证(这里其实是一个Map键值对 "mail.smtp.ssl.enable":"true"
spring.mail.properties.mail.smtp.ssl.enable=true
4、编程(两个方法可写在Controller里面,这里是为了方便测试)
@SpringBootTest
class Springboot09TestApplicationTests {
@Autowired
JavaMailSenderImpl mailSender;
@Test
void simpleMail() {
//发送简单邮件
SimpleMailMessage mailMessage = new SimpleMailMessage();
mailMessage.setSubject("同学,你好~");//主题
mailMessage.setText("Java 路上持续学习!!");//内容
mailMessage.setTo("xxxx@qq.com");//发给谁(可写多个)
mailMessage.setFrom("xxxx@qq.com");//由谁发出
mailSender.send(mailMessage);
}
@Test
void mimeMail() throws MessagingException {
//发送复杂邮件
MimeMessage mimeMessage = mailSender.createMimeMessage();//加参数可从文件流读取
// true 支持多文件上传
MimeMessageHelper messageHelper = new MimeMessageHelper(mimeMessage, true);
messageHelper.setSubject("同学,你好~plus版");//主题
//支持解析HTML格式的内容
messageHelper.setText("<h1> Java 路上持续学习!!plus版 </h1>", true);
//附件(文件名、File对象)
messageHelper.addAttachment("1.png", new File("C:\\Users\\11427\\Desktop\\qq.png"));
messageHelper.addAttachment("2.png", new File("C:\\Users\\11427\\Desktop\\qq.png"));
messageHelper.setTo("xxxx@qq.com");//发给谁
messageHelper.setFrom("xxxx@qq.com");//由谁发
mailSender.send(mimeMessage);
}
}
效果:
-
简单邮件
-
复杂邮件
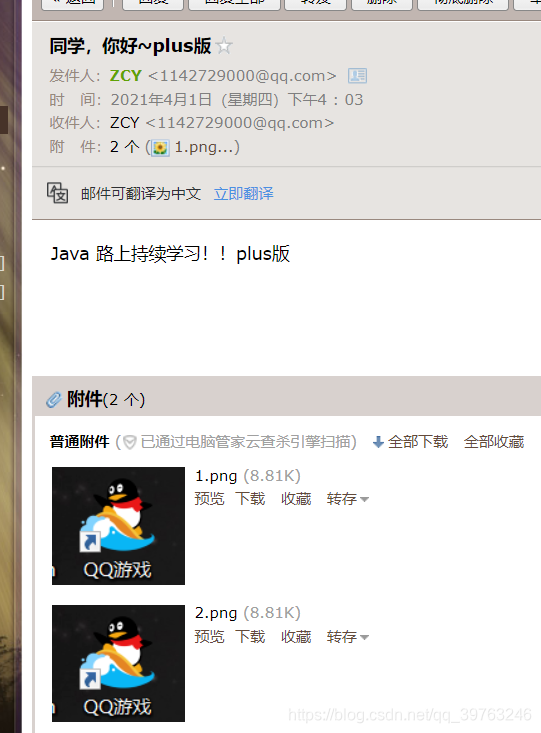
3、定时任务
顾名思义,在指定时间执行方法。
1、开启定时功能
@EnableAsync//打开异步功能
@EnableScheduling//打开定时功能
@SpringBootApplication
public class Springboot09TestApplication {
public static void main(String[] args) {
SpringApplication.run(Springboot09TestApplication.class, args);
}
}
2、编写定时方法
@Service
public class ScheduledService {
/**在一个特定的事件执行这个方法(有点像Timer)
* 这里会用到cron表达式,具体方法可百度,一般情况下要什么时间都可直接去网上找
* https://cron.qqe2.com/ 可查询具体怎么写
* 这里简单说明:秒 分 时 日 月 周几
*/
@Scheduled(cron = "* * * * * ?")//每秒执行一次
public void hello(){
System.out.println("执行了一次方法!");
}
}