1.学习目标:
for 语句while 语句
if 语句
case 语句
expect 语句
exit,break,continue
2.exit break continue退出命令的区别
exit直接退出当前脚本
break仅仅退出本次循环
continue 退出本次循环进行下一次循环
vim file.sh
#!/bin/bash
for NUM in {1..5}
do
if
[ "$NUM" -eq 3 ]
then
$1
fi
echo $NUM
done
echo hello linux!!!
3.for语句
示例:
vim file.sh
#!/bin/bash
for NUM in {1..5}
do
echo $NUM
done
4.while语句
while true 条件为真就执行
do
done
练习:编写脚本当负载超过80%时发送邮件给超级用户
vim mail_warning.sh
#!/bin/bash
DISK_NUM=`df -h | awk '/\/$/{print $5}' | awk -F "%" '{print $1}'`
#TTY=`ps $$|awk '/bash$/{print $2}'`
while true
do
[ "$DISK_NUM" -ge "80" ]&&{
echo "Your / will full !!" |mail -s warning root
}
sleep 1
done
5.if语句
格式:if
then
elif
then
...
else
fi
if语句应用举例
#!/bin/bash
if
[ "$1" = "a" ]
then
echo '$1' is a
elif
[ "$1" = "a" ]
then
echo '$1' is b
elif
[ "$1" = "a" ]
then
echo '$1' is c
else
echo unknow $1
fi
练习:
写一个脚本file_check.sh,检测文件是否存在,如果存在,并判断文件类型
#!/bin/bash
if
[ "$#" -ne 1 ]
then
echo "Please input a file follow script!!"
elif
[ ! -e "$1" ]
then
echo "$1 is not exist !!"
elif
[ -L "$1" ]
then
echo $1 is a link
elif
[ -S "$1" ]
then
echo $1 is a socket
elif
[ -b "$1" ]
then
echo $1 is a block
elif
[ -c "$1" ]
then
echo $1 is a common
else
echo unknown $1
fi
编写脚本check_file.sh用函数的方法实现检测文件是否存在,如果存在,并判断文件类型
#!/bin/bash
Check_file()
{
if
[ "$1" "$2" ]
then
echo "$2" is $3
exit 0
fi
}
if
[ "$#" -ne "1" ]
then
echo "Please input a file follow script!!"
elif
[ ! -e "$1" ]
then
echo "$1 is not exist !!"
else
Check_file -S $1 socket
Check_file -b $1 block
Check_file -c $1 character
Check_file -L $1 link
Check_file -d $1 directory
fi
编写一个脚本:建立用户当出现以下情况报错:
1 文件数量不对报错2 文件不存在
3 文件行数差异
4 用户存在显示用户存在,但不改变此用户密码
5 当用户不存在建立用户并设定相应密码
#!/bin/bash
################## Check Rule ##################
if
[ "$#" -ne "2" ]
then
echo "ERROR1:Please input userfile and passfile follow the script!!"
exit 1
elif
[ ! -e "$1" ]
then
echo "ERROR2:Sorry, $1 is not exist !!"
exit 1
elif
[ ! -e "$2" ]
then
echo "ERROR3:Sorry, $2 is not exist !!"
exit 1
elif
USERFILE_LINE=`awk 'BEGIN{N=0}{N++}END{print N}' $1`
PASSFILE_LINE=`awk 'BEGIN{N=0}{N++}END{print N}' $2`
[ ! "$USERFILE_LINE" -eq "$PASSFILE_LINE" ]
then
echo "ERROR4:$1's line is different from $2's line !!"
exit 1
fi
################CREATE USER################
for LINE_NUM in `seq 1 $USERFILE_LINE`
do
USERNAME=`sed -n ${LINE_NUM}p $1`
PASSWORD=`sed -n ${LINE_NUM}p $2`
useradd $USERNAME &&{
echo $PASSWORD | passwd --stdin $USERNAME
}
done
6.case语句
格式:
case
word1)
action1
;;
word2)
action2
;;
......
*)
action_last
esac
示例:
#!/bin/bash
case $1 in
westos)
echo linux
;;
linux)
echo westos
;;
*)
echo "Error: input westos or linux after script !!"
esac
练习:
写一个脚本,当$1是cat,输出为dog,当$1 是dog,输出为cat如果是其他的,输出为"please input cat or dog foolow scripts !!"
方法一:
if语句
方法二:
case语句:
#!/bin/bash
case $1 in
dog)
echo cat
;;
cat)
echo dog
;;
*)
echo error
esac
case和if的比较
case效率更高
7.expect
expect是自动应答命令用于交互式命令的自动执行expect是一种自动交互语言,能实现在shell脚本中为scp和ssh等自动输入密码自动登录。
spawn是expect中的监控程序,其运行后台会监控命令提出的交互问题
send 发送问题答案给交互命令
"\r" 表示回车
exp_continue 表示当问题不存在时继续回答下面的问题
expect eof 表示问题回答完毕退出expect环境
interact 表示问题回答完毕退出expect环境;//交互模式,用户会永远停在远程服务器上面
set NAME [lindex $argv n] 定义变量
命令在:
/usr/bin/expect示例:
#!/bin/bash
read -p "What's your name: " NAME
read -p "How old are you: " AGE
read -p "Where are you from: " CITY
read -p "Are you happy: " FEEL
echo "$NAME is $AGE and come from $CITY ; She feel $FEEL "
编写一个问题脚本
yum install expect -y
#!/usr/bin/expect
set timeout 2
spawn /mnt/ask.sh
expect "name:"
send "name\r"
expect "old:"
send "18\r"
expect "from:"
send "chain\r"
expect "happy:"
send "happy\r"
expect eof
用expect自动回答问题脚本中的问题
自动匹配问题中的关键字作答
#!/usr/bin/expect
set timeout 2
spawn /mnt/ask.sh
expect {
name { send "girl\r";exp_continue }
old { send "18\r";exp_continue }
from { send "chain\r";exp_continue }
happy { send "happy\r" }
}
expect eof
用跟在脚本后的字符串回答问题
#!/usr/bin/expect
set timeout 2
set NAME [lindex $argv 0] #在expect中表示exp文件后的第一串字符,和shell中的$1相似
set AGE [lindex $argv 1]
set CITY [lindex $argv 2]
set FEEL [lindex $argv 3]
spawn /mnt/ask.sh
expect {
name { send "$NAME\r";exp_continue }
old { send "$AGE\r";exp_continue }
from { send "$CITY\r";exp_continue }
happy { send "$FEEL\r" }
}
expect eof
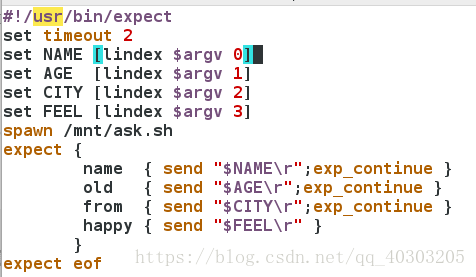
