原视频链接:https://search.bilibili.com/all?keyword=BV1xs411Q799&from_source=nav_search_new
030 文件系统:介绍一个高大上的东西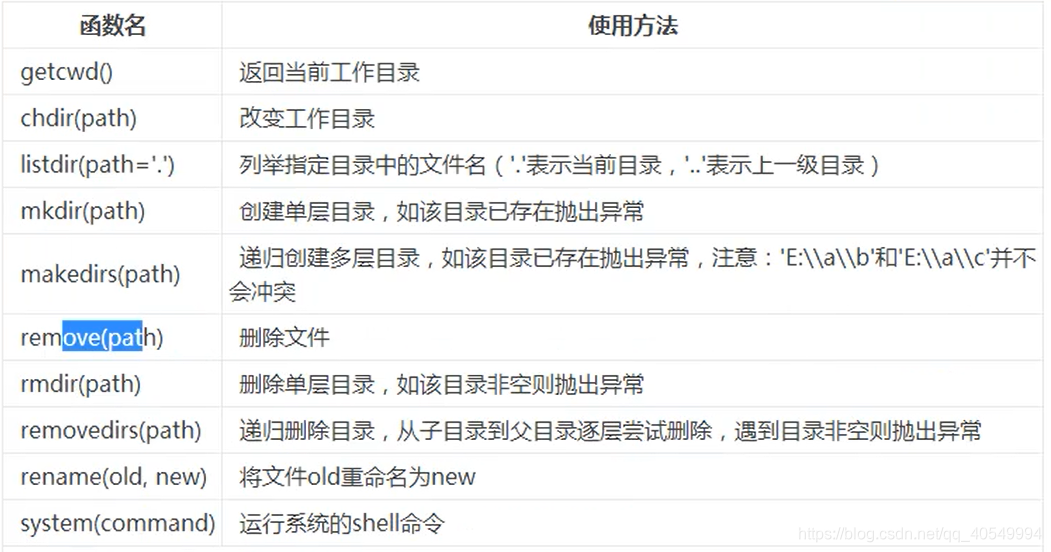
import os
import random
secret = random.randint(1,10)
print(os.getcwd())
# D:\Py_Doc 显示工作目录
print(os.listdir(os.getcwd()))
# ['.idea', 'TEST.py', 'venv'] 显示工作目录下的文件名
import os
import time
print(os.path.basename('E:\\asd\\asd\\123.txt'))
# 123.txt 去掉路径,返回文件名
print(os.path.dirname('E:\\asd\\asd\\123.txt'))
# E:\asd\asd 去掉文件名,返回路径
print(os.path.join('A', 'B', 'C'))
# A\B\C 组合成文件名
print(os.path.split('E:\\asd\\asd\\123.txt'))
# ('E:\\asd\\asd', '123.txt') 分割路劲和文件名
print(os.path.splitext('E:\\asd\\asd\\123.txt'))
# ('E:\\asd\\asd\\123', '.txt') 将扩展名拿出来
print(os.path.getatime('F:\\boy_1.txt'))
# 1588345310.7322388 浮点秒数
print(time.gmtime(os.path.getatime('F:\\boy_1.txt')))
# time.struct_time(tm_year=2020, tm_mon=5, tm_mday=1, tm_hour=15, tm_min=1, tm_sec=50, tm_wday=4, tm_yday=122, tm_isdst=0) 用gmtime()将时间转换为世界时
print(time.localtime(os.path.getatime('F:\\boy_1.txt')))
# time.struct_time(tm_year=2020, tm_mon=5, tm_mday=1, tm_hour=23, tm_min=1, tm_sec=50, tm_wday=4, tm_yday=122, tm_isdst=0) 用localtime()将时间转换为北京时间
031 永久储存:腌制一缸美味的泡菜
import pickle
my_list = [123, 3.14, '小甲鱼', ['anthor list']]
pickle_file = open('F:\\my_list.pkl', 'wb')
pickle.dump(my_list, pickle_file)
pickle_file.close()
# 这样就在F盘创建了一个my_list.pkl文件,但是这是个二进制文,打开可能会是乱码
pickle_file = open('F:\\my_list.pkl', 'rb')
my_list2 = pickle.load(pickle_file)
print(my_list2)
# [123, 3.14, '小甲鱼', ['anthor list']] 用load方法读取pkl文件内容
032 异常处理:你不可能总是对的 1
Python标准异常总结:异常
AssertionError: assert 语句报出的异常
my = ['This is it.']
assert len(my) < 1
# Traceback (most recent call last):
# File "D:/Py_Doc/TEST.py", line 2, in <module>
# assert len(my) < 1
# AssertionError
IndexError: 索引超出序列的范围
my = [1, 2, 3]
print(my[3])
# Traceback (most recent call last):
# File "D:/Py_Doc/TEST.py", line 2, in <module>
# print(my[3])
# IndexError: list index out of range
KeyError: 字典中查找一个不存在的关键字
my = {'one': 1, 'two': 2}
print(my['three'])
# Traceback (most recent call last):
# File "D:/Py_Doc/TEST.py", line 2, in <module>
# print(my['three'])
# KeyError: 'three'
033 异常处理:你不可能总是对的 2
try:
my = {'one': 1, 'two': 2}
print(my['three'])
except KeyError:
print('字典索引错误')
# 字典索引错误
try:
my = {'one': 1, 'two': 2}
print(my['three'])
except KeyError as reason:
print('字典索引错误\n错误的原因是:' + str(reason))
# 字典索引错误
# 错误的原因是:'three'
try:
my = {'one': 1, 'two': 2}
print(my['three'])
except KeyError as reason:
print('字典索引错误\n错误的原因是:' + str(reason))
finally:
print('try结束')
# 字典索引错误
# 错误的原因是:'three'
# try结束
raise KeyError
# Traceback (most recent call last):
# File "D:/Py_Doc/TEST.py", line 30, in <module>
# raise KeyError
# KeyError
try后面可以跟多个except,出错的时候会在except里找匹配的错误,然后运行相关的语句
try语句中一旦出现异常,则不会继续运行下面的语句
raise: 直接引出错误
034 丰富的else语句以及简洁的with语句
while与if搭配:如果while中没有完成循环就被break语句中断,就会运行if中的内容。
关注 – with open(‘文件名’, ‘打开方式’) as f: ;-- 句式
035 图形用户界面入门:EasyGui
对象 = 属性 + 方法
OO:Object Oriented
继承:子类自动共享父类之间数据和方法的机制
class MyList(list):
pass
list1 = MyList()
list1.append(1)
list1.append(8)
list1.append(3)
print(list1)
# [1, 8, 3]
多态
class A:
def fun(self):
print('I\'m A')
class B:
def fun(self):
print('I\'m B')
a = A()
b = B()
a.fun()
b.fun()
# I'm A
# I'm B
037 类和对象:面向对象编程
self是什么
class Ball:
def setName(self, name):
self.name = name
def kick(self):
print('My name is %s' % self.name)
a = Ball()
a.setName('Ball A')
b = Ball()
b.setName('Ball B')
a.kick()
b.kick()
私有变量的定义:Python中只需要在变量名或者函数名前加上两个下划线,那这个变量或者函数就会变为私有的了
class Name():
__name = '小甲鱼'
def getName(self):
print(self.__name)
p = Name()
print(p.__name)
# 报错,__name是私有变量,不可在class外访问
p.getName()
# 小甲鱼 getName()是class内函数
038 类和对象:继承
在语句class ABC(abc)
中,abc称作基类,父类或者超类;ABC称作子类
class Parent():
def hello(self):
print('It\'s Parents')
class Child(Parent):
pass
a = Child()
a.hello()
# It's Parents
如果子类中定义与父类同名的方法或属性,则会自动覆盖父类对应的方法或属性
class Parent():
def hello(self):
print('It\'s Parents')
class Child(Parent):
def hello(self):
print('It\'s Child')
a = Child()
a.hello()
# It's Child
多重继承:class ABC(abc, qwe, asd)
同时继承多个类
039 类和对象:拾遗
组合:把多个类的实例化放到新类里面,那就不用继承多个类了
class Turtle:
def __init__(self, x):
self.num = x
class Fish:
def __init__(self, x):
self.num = x
class Pool:
def __init__(self, x, y):
self.turtle = x
self.fish = y
def print_num(self):
print('There are %d turtles and %d fish in the po0l' % (self.turtle, self.fish))
pool = Pool(1, 10)
pool.print_num()
Mix-in:混入
class C():
count = 0
a = C()
b = C()
c = C()
print(a.count)
print(b.count)
print(c.count)
# 0 0 0
c.count += 10
print(a.count)
print(b.count)
print(c.count)
# 0 0 10
C.count += 100
print(a.count)
print(b.count)
print(c.count)
# 100 100 10
如果属性名字跟方法名字相同,属性会覆盖方法
class C():
def x(self):
print('I\'m X')
c = C()
c.x()
# I'm X
c.x = 10
print(c.x)
# 10
c.x()
# 报错 TypeError: 'int' object is not callable
绑定:Python严格要求方法需要有实例才能被调用
class CC:
def setXY(self, x, y):
self.x = x
self.y = y
def printXY(self):
print(self.x, self.y)
dd = CC()
print(dd.__dict__)
print(CC.__dict__)
# {}
# {'__module__': '__main__', 'setXY': <function CC.setXY at 0x037D1AD8>, 'printXY': <function CC.printXY at 0x037D1A90>, '__dict__': <attribute '__dict__' of 'CC' objects>, '__weakref__': <attribute '__weakref__' of 'CC' objects>, '__doc__': None}
dd.setXY(4, 5)
print(dd.__dict__)
print(CC.__dict__)
# {'x': 4, 'y': 5}
# {'__module__': '__main__', 'setXY': <function CC.setXY at 0x03381AD8>, 'printXY': <function CC.printXY at 0x03381A90>, '__dict__': <attribute '__dict__' of 'CC' objects>, '__weakref__': <attribute '__weakref__' of 'CC' objects>, '__doc__': None}
# 请注意,setXY之后的CC__dict__和没有setXY之前是一样的。
del CC
dd.setXY(1, 2)
dd.printXY()
# 1 2
这种情况就要归功于self
,在dd.self(4, 5)
的时候,实际上语句应该是dd.self(dd, 4, 5)
,所以说4,5这两个属性是只属于实例对象dd的,跟类本身CC无关。最后删除了CC这个类之后,dd.printXY
还能运行的原因也是类似,类中定义的属性和方法也是静态变量,就算类本身被删除,这些静态变量还依然是存放在之前的实例化对象中的。