import java.io.Serializable;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashSet;
import java.util.List;
public class DateUtil implements Serializable {
private static final long serialVersionUID = 1L;
public static final String DATE_PATTERN_01 = "yyyy-MM-dd";
public static final String DATE_PATTERN_02 = "yyyyMMdd";
public static final String DATE_PATTERN_03 = "yyyy-MM-dd HH:mm:ss:SSS";
public static final String DATE_PATTERN_04 = "yy-MM-dd";
public static final String DATE_PATTERN_05 = "yyyyMMddHHmmssSSS";
public static final String DATE_PATTERN_06 = "yyyy-MM-dd HH:mm";
public static final String DATE_PATTERN_07 = "yyyy";
public static final String DATE_PATTERN_08 = "yyyy-MM-dd HH:mm:ss";
public static final String DATE_PATTERN_09 = "yyyyMMddHHmmss";
public static final String DATE_PATTERN_10 = "yyyy-MM-dd HH:mm:ss";
public static final String DATE_PATTERN_11 = "yyMMddHHmm";
public static final String DATE_PATTERN_12 = "yyyy-MM-dd";
public static final String DATE_PATTERN_13 = "yyyy/MM/dd HH:mm";
public static final String DATE_PATTERN_14 = "yyyy-MM-dd HH:mm";
public static final String DATE_PATTERN_15 = "yyyy/MM/dd";
public static final String DATE_PATTERN_16 = "HH:mm";
/**
* 简短日期格式:yyyyMMddHHmmss
*/
public static final String DATE_FORMAT_SHORT_YYYYMMDDHHMMSS = "yyyyMMddHHmmss";
/**
* 简短日期格式:yyyyMMddHHmm
*/
public static final String DATE_FORMAT_SHORT_YYYYMMDDHHMM = "yyyyMMddHHmm";
/**
* 简短日期格式:yyyyMMddHH
*/
public static final String DATE_FORMAT_SHORT_YYYYMMDDHH = "yyyyMMddHH";
/**
* 简短日期格式:yyyyMMdd
*/
public static final String DATE_FORMAT_SHORT_YYYYMMDD = "yyyyMMdd";
/**
* 简短日期格式长度YYYYMMDDHHMMSS
*/
private static final int SHORT_YYYYMMDDHHMMSS_LENGTH = 14;
/**
* 简短日期格式长度YYYYMMDDHHMM
*/
private static final int SHORT_YYYYMMDDHHMM_LENGTH = 12;
/**
* 简短日期格式长度YYYYMMDDHH
*/
private static final int SHORT_YYYYMMDDHH_LENGTH = 10;
/**
* 日期上限值
*/
private static final Integer DATE_LIMIT = 99991231;
/**
* 日期格式集合
*/
private static HashSet datePatternSet;
static {
datePatternSet = new HashSet();
datePatternSet.add(DATE_PATTERN_01);
datePatternSet.add(DATE_PATTERN_02);
datePatternSet.add(DATE_PATTERN_03);
datePatternSet.add(DATE_PATTERN_04);
datePatternSet.add(DATE_PATTERN_05);
datePatternSet.add(DATE_PATTERN_06);
datePatternSet.add(DATE_PATTERN_07);
datePatternSet.add(DATE_PATTERN_08);
datePatternSet.add(DATE_PATTERN_09);
datePatternSet.add(DATE_PATTERN_10);
datePatternSet.add(DATE_PATTERN_11);
datePatternSet.add(DATE_PATTERN_16);
}
/**
* 根据指定的格式把日期型转化为字符串
*
* @param pDate
* Date 日期型
* @param datePattern
* Date 格式
* @return String 结果
*/
public static String getDateString(Date pDate, String datePattern) {
if (StringUtil.isEmpty(datePattern) || !datePatternSet.contains(datePattern)) {
// throw new IllegalArgumentException("Date Pattern error!");
return "";
}
if (pDate == null) {
// throw new IllegalArgumentException("inputed Date error!");
return "";
}
SimpleDateFormat dateFormat = new SimpleDateFormat(datePattern);
return dateFormat.format(pDate);
}
/**
* 根据指定的格式把字符串转换为日期型
*
* @param str
* String 字符串
* @param datePattern 格式
* @return Date 日期型
*/
public static Date getDateByString(String str, String datePattern) {
if (StringUtil.isEmpty(datePattern) || !datePatternSet.contains(datePattern)) {
return null;
}
if (StringUtil.isEmpty(str)) {
return null;
}
SimpleDateFormat dateFormat = new SimpleDateFormat(datePattern);
try {
return dateFormat.parse(str);
} catch (ParseException pe) {
pe.printStackTrace();
}
return null;
}
/**
* 获取格式YYYYMMDD的当前系统时间的字符串
*
* @return 日期字符串
*/
public static String getShortSysDateString() {
Date date = new Date(System.currentTimeMillis());
return DateUtil.format(date, DATE_FORMAT_SHORT_YYYYMMDD);
}
/**
* 获取表示当前系统时间的前一天的YYYYMMDD格式的字符串
*
* @return 日期字符串
*/
public static String getPreviousDate() {
SimpleDateFormat dateFormat = new SimpleDateFormat(DATE_PATTERN_02);
Calendar calendar = new GregorianCalendar();
calendar.set(Calendar.DAY_OF_MONTH, calendar.get(Calendar.DAY_OF_MONTH) - 1);
return dateFormat.format(calendar.getTime());
}
/**
* 把字符串转换为日期
*
* @param dateStr 日期字符串
* @return 结果日期
*/
public static Date parse(String dateStr) {
Date date = null;
if (StringUtil.isNotEmpty(dateStr)) {
String shortDateStr = dateStr.replaceAll("\\-|\\ |\\.|\\:|\\/", "");
String formatStr = "";
// 如果月份或日期格式为XXXX/X/X转为XXXX/0X/0X,没有0加0
int shortDateStrLen = shortDateStr.length();
if (shortDateStrLen == 7 || shortDateStrLen == 6) {
String[] strarr = {};
if (dateStr.indexOf("-") > 0) {
strarr = dateStr.split("-");
} else if (dateStr.indexOf("/") > 0) {
strarr = dateStr.split("/");
}
boolean firstAdd = false;
if (strarr[1].length() == 1) {
shortDateStr = strarr[0] + "0" + strarr[1] + strarr[2];
firstAdd = true;
}
if (strarr[2].length() == 1) {
if (firstAdd) {
shortDateStr = strarr[0] + "0" + strarr[1] + "0" + strarr[2];
} else {
shortDateStr = strarr[0] + strarr[1] + "0" + strarr[2];
}
}
} else if (shortDateStrLen == 13) {
long dateLong = Long.parseLong(shortDateStr);
return new Date(dateLong);
}
switch (shortDateStrLen) {
case SHORT_YYYYMMDDHHMMSS_LENGTH:
formatStr = DATE_FORMAT_SHORT_YYYYMMDDHHMMSS;
break;
case SHORT_YYYYMMDDHHMM_LENGTH:
formatStr = DATE_FORMAT_SHORT_YYYYMMDDHHMM;
break;
case SHORT_YYYYMMDDHH_LENGTH:
formatStr = DATE_FORMAT_SHORT_YYYYMMDDHH;
break;
default:
formatStr = DATE_FORMAT_SHORT_YYYYMMDD;
break;
}
date = parse(shortDateStr, formatStr);
}
return date;
}
/**
* 把字符串格式化为日期类型
*
* @param dateStr 日期字符串
* @param format 格式
* @return 日期类型结果
*/
public static Date parse(String dateStr, String format) {
Date date = null;
if (StringUtil.isNotEmpty(dateStr)) {
DateFormat formatter = new SimpleDateFormat(format);
try {
date = formatter.parse(dateStr);
} catch (ParseException ex) {
}
}
return date;
}
/**
* 把日期格式化为字符串
*
* @param date 原始日期
* @param parttern 格式
* @return 日期字符串
*/
public static String format(Date date, String parttern) {
String value = "";
if (date != null) {
try {
SimpleDateFormat dateFormat = new SimpleDateFormat(parttern);
value = dateFormat.format(date);
} catch (Exception ex) {
}
}
return value;
}
// /**
// * 把日期格式化为字符串
// *
// * @param date 原始日期
// * @param sparttern 格式
// * @return 日期字符串
// */
// public static String formatAny(Date date, String sparttern) {
// String value = "";
// String parttern = StringUtil.replace(sparttern, "yy/mm", "yy/MM");
// parttern = StringUtil.replace(parttern, "yy-mm", "yy-MM");
// parttern = StringUtil.replace(parttern, "hh", "HH");
// parttern = StringUtil.replace(parttern, "h", "HH");
// parttern = StringUtil.replace(parttern, "ii", "mm");
// parttern = StringUtil.replace(parttern, "i", "mm");
// if (date != null) {
// try {
// SimpleDateFormat dateFormat = new SimpleDateFormat(parttern);
// value = dateFormat.format(date);
// } catch (Exception ex) {
// }
// }
// return value;
// }
/**
* 更改日期表示格式
*
* @param value 日期字符串
* @param format 格式
* @return 日期字符串
*/
public static String changeDateFormat(String value, String format) {
Date date = parse(value);
return format(date, format);
}
/**
* 增加年数
*
* @param date 原始日期
* @param yearAmount 要增加的年数
* @return 增加后的日期
*/
public static Date addYears(Date date, int yearAmount) {
return add(date, Calendar.YEAR, yearAmount);
}
/**
* 获取当前系统时间
*
* @return Date 当前时刻
*/
public static Date getSysDate() {
return new Date(System.currentTimeMillis());
}
/**
* 获取当前日期格式yyyyMMddHHmmss
*
* @return 日期字符串
*/
public static String getSysDateString() {
Date date = new Date(System.currentTimeMillis());
return format(date, DATE_FORMAT_SHORT_YYYYMMDDHHMMSS);
}
/**
* 获取yyyy-MM-dd格式当前日期
*
* @return 日期字符串
*/
public static String getSysDateString2() {
Date date = new Date(System.currentTimeMillis());
return format(date, DATE_PATTERN_12);
}
/**
* 获取参数日期为周几
*
* @param date
* @return 周几
*/
public static String getWeekDay(Date date) {
String weekDay = "";
if (date == null) {
return weekDay;
}
SimpleDateFormat formatter = new SimpleDateFormat("E");
weekDay = formatter.format(date);
return weekDay;
}
/**
* 获取2个参数日期的相差日数
*
* @param firstDate
* date 起始日期
* @param secendDate
* date 结束日期
* @return Integer (firstDate-secendDate) 相差日数
*/
public static Integer getRemainder(Date firstDate, Date secendDate) {
if (firstDate == null || secendDate == null) {
return null;
}
Date firstYmdDate = getDateByString(getDateString(firstDate, DATE_PATTERN_01), DATE_PATTERN_01);
Date secendYmdDate = getDateByString(getDateString(secendDate, DATE_PATTERN_01), DATE_PATTERN_01);
long dayRemainder = (firstYmdDate.getTime() - secendYmdDate.getTime()) / (24 * 60 * 60 * 1000);
return new Integer((int) dayRemainder);
}
/**
* 日期的加法
*
* @param date 原始日期
* @param field 日期单位
* @param amount 要增加的数值
* @return Date 结果日期
*/
private static Date add(Date date, int field, int amount) {
if (date == null) {
return null;
}
Calendar calendar = GregorianCalendar.getInstance();
calendar.setTime(date);
calendar.add(field, amount);
return calendar.getTime();
}
/**
* 日期增加天数
*
* @param date
* 原日期
* @param dayAmount
* 增加的天数
* @return Date 结果日期
*/
public static Date addDays(Date date, int dayAmount) {
return add(date, Calendar.DATE, dayAmount);
}
/**
* 日期增加月数
*
* @param date
* 原日期
* @param monthAmount
* 增加的月数
* @return Date 结果日期
*/
public static Date addMonths(Date date, int monthAmount) {
return add(date, Calendar.MONTH, monthAmount);
}
/**
* 把原Date转换为yyyy/MM/dd HH:mm:ss格式的Date
*
* @param date
* 原Date
* @return yyyy/MM/dd HH:mm:ss格式的Date
*/
public static Date toDateFullStr(String date) {
if (null == date) return null;
try {
return parse(DATE_PATTERN_03, date);
} catch (Exception e) {
return null;
}
}
/**
* 获取给定Date的yyyy-MM-dd格式日期
*
* @param date 原始日期
* @return Date 转换后的日期
*/
public static Date getDateByDatetime(Date date) {
if (date == null) {
return null;
}
return getDateByString(getDateString(date, DATE_PATTERN_01), DATE_PATTERN_01);
}
/**
* 检查日期字符串是否符合yyyy格式
*
* @param strDate 日期字符串
* @return boolean 是/否
*/
public static boolean isValidateDate(String strDate) {
try {
if (!StringUtil.isNumeric(strDate)) {
return false;
}
SimpleDateFormat sdf = new SimpleDateFormat(DATE_PATTERN_07);
sdf.setLenient(false);
sdf.parse(strDate);
return true;
} catch (ParseException e) {
return false;
}
}
/**
* 检查日期字符串是否符合yyyy-MM-dd格式
*
* @param strDate 日期字符串
* @return boolean 是/否
*/
public static boolean isValidateDateFYYYYMMDD(String strDate) {
try {
SimpleDateFormat sdf = new SimpleDateFormat(DATE_PATTERN_01);
sdf.setLenient(false);
sdf.parse(strDate);
return true;
} catch (ParseException e) {
return false;
}
}
/**
* 检查日期字符串是否符合YYYYMMDD格式1
*
* @param strDate 日期字符串
* @return boolean 是/否
*/
public static boolean isValidateDateYYYYMMDD(String strDate) {
try {
SimpleDateFormat sdf = new SimpleDateFormat(DATE_PATTERN_02);
sdf.setLenient(false);
sdf.parse(strDate);
return true;
} catch (ParseException e) {
return false;
}
}
/**
* 检查日期是否合法
*
* @param date
* 日期字符串
* @return boolean 是/否
*/
public static boolean checkDate(Date date) {
String strDate = getDateString(date, DATE_PATTERN_02);
Date systemDate = getDateByDatetime(getSysDate());
String beforStrDate = getDateString(addYears(systemDate, -1), DATE_PATTERN_02);
String afterStrDate = getDateString(addYears(systemDate, 5), DATE_PATTERN_02);
if (strDate.compareTo(beforStrDate) < 0 || strDate.compareTo(afterStrDate) > 0) {
return false;
}
return true;
}
/**
* 获取给定日期的当月的最后一天
*
* @param date 给定日期
* @return Date 当月最后一天
*/
public static Date getMonthLastDay(Date date) {
Calendar calendar = GregorianCalendar.getInstance();
calendar.setTime(date);
String dateStr = getDateString(date, DATE_PATTERN_02);
String lastDay = dateStr.substring(0, 6) + String.valueOf(calendar.getActualMaximum(Calendar.DATE));
return getDateByString(lastDay, DATE_PATTERN_02);
}
/**
* 获取当月的第一天
*
* @param date 给定日期
* @return Date 当月第一天
*/
public static Date getMonthFirstDay(Date date) {
Calendar calendar = GregorianCalendar.getInstance();
calendar.setTime(date);
String dateStr = DateUtil.getDateString(date, DateUtil.DATE_PATTERN_02);
String lastDay = dateStr.substring(0, 6) + String.valueOf(calendar.getActualMinimum(Calendar.DATE));
return DateUtil.getDateByString(lastDay, DateUtil.DATE_PATTERN_02);
}
/**
* 未知方法
* //TODO 添加方法功能描述
* @param date
*/
@SuppressWarnings("deprecation")
public static void lastDaySecond(Date date) {
if (date != null) {
date.setHours(23);
date.setMinutes(59);
date.setSeconds(59);
}
}
/**
* 未知方法
* //TODO 添加方法功能描述
* @return
*/
public static boolean isApplicationEffOut() {
// Date today = DateUtil.getSysDate();
// String applicationEffOutDate = "2013/08/01";
// Date applicationEffOutDatedt = DateUtil.getDateByString(applicationEffOutDate, DateUtil.DATE_PATTERN_01);
// if (today.after(applicationEffOutDatedt))
// {
// return true;
// }
// else
// {
// return false;
// }
return false;
}
/**
* 计算日期天数的间隔
*
* @param d1 起始日期
* @param d2 结束日期
* @return int 间隔日数
*/
public static int getBetweenDayNumber(Date d1, Date d2) {
long dayNumber = 0L;
try {
dayNumber = (d2.getTime() - d1.getTime()) / 86400000L;
} catch (Exception e) {
e.printStackTrace();
}
return (int) dayNumber;
}
/**
* 计算字符串型日期间隔
*
* @param date1 起始日期字符串
* @param date2 结束日期字符串
* @return int 间隔日数
*/
public static int getBetweenDayNumber(String date1, String date2) {
long dayNumber = 0L;
try {
Date d1 = parse(date1);
Date d2 = parse(date2);
dayNumber = (d2.getTime() - d1.getTime()) / 86400000L;
} catch (Exception e) {
e.printStackTrace();
}
return (int) dayNumber;
}
/**
* 给日期字符串增加指定的天数
*
* @param date 原始日期
* @param day 要增加的日数
* @return String 日期字符串
*/
public static String addDate(String date, int day) {
String resultString = date;
try {
Date d = parse(date);
Calendar cal = Calendar.getInstance();
cal.setTime(d);
cal.add(5, day);
resultString = convertDateToString(cal.getTime());
} catch (Exception localException) {
}
return resultString;
}
/**
* 判断前一个Date与后一个Date的天数差是否在指定天数以内
*
* @param fromDate
* 开始日期
* @param thisDate
* 结束日期
* @param day
* 天数差
* @return 是/否
*/
public static boolean betweenDate(Date fromDate, Date thisDate, Integer day) {
try {
Date toDate = addDays(fromDate, day);
if (fromDate.compareTo(thisDate) <= 0 && thisDate.compareTo(toDate) <= 0) return true;
return false;
} catch (Exception e) {
return false;
}
}
/**
* Date->String转换
*
* @param todayDate 原始日期
* @return String 日期字符串
*/
public static String convertDateToString(Date todayDate) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String tempStr = sdf.format(todayDate);
return tempStr;
}
/**
* 计算第二个日期和第一个日期的年份和天数差值组成的列表</br>
* 例:DateUtil.getBetweenYearNumber("2016-06-02", "2017-06-05") 返回 [1, 3]
*
* @param date1 第一个日期字符串
* @param date2 第二个日期字符串
* @return List<Integer> 结果
*/
public static List<Integer> getBetweenYearNumber(String date1, String date2) {
List result = new ArrayList();
result = getBetweenYearNumber(parse(date1), parse(date2));
return result;
}
/**
* 计算第二个日期和第一个日期的年份和天数差值组成的列表</br>
* 例:DateUtil.getBetweenYearNumber("2016-06-02", "2017-06-05") 返回 [1, 3]
*
* @param date1 第一个日期
* @param date2 第二个日期
* @return List<Integer> 结果
*/
public static List<Integer> getBetweenYearNumber(Date date1, Date date2) {
List result = new ArrayList();
Calendar cal = Calendar.getInstance();
cal.setTime(date1);
int y1 = cal.get(1);
int m1 = cal.get(2);
int d1 = cal.get(5);
cal.setTime(date2);
int y2 = cal.get(1);
int m2 = cal.get(2);
int d2 = cal.get(5);
if ((m2 > m1) || ((m2 >= m1) && (d2 >= d1))) {
result.add(Integer.valueOf(y2 - y1));
cal.set(y2, m1, d1);
Date temp = cal.getTime();
result.add(Integer.valueOf(getBetweenDayNumber(temp, date2)));
} else {
result.add(Integer.valueOf(y2 - y1 - 1));
cal.set(y2 - 1, m1, d1);
Date temp = cal.getTime();
result.add(Integer.valueOf(getBetweenDayNumber(temp, date2)));
}
return result;
}
/**
* 给日期字符串增加指定的年份值
*
* @param date 日期字符串
* @param year 要增加的年数
* @return String 结果日期字符串
*/
public static String addYear(String date, int year) {
String resultString = date;
try {
Date d = parse(date);
Calendar cal = Calendar.getInstance();
cal.setTime(d);
cal.add(1, year);
resultString = convertDateToString(cal.getTime());
} catch (Exception localException) {
}
return resultString;
}
/**
* 获取当年的2月份的天数
*
* @param issueDate 给定的日期字符串
* @param cDate 指定日期
* @return int 当年的二月份的天数
*/
public static int getNumFor2_29(String issueDate, String cDate) {
int leapYear = 0;
try {
Date dIssueDate = parse(issueDate);
Date dCDate = parse(cDate);
List listInt = getBetweenYearNumber(dIssueDate, dCDate);
int dayCount = 365 * ((Integer) listInt.get(0)).intValue() + ((Integer) listInt.get(1)).intValue();
int accrueDays = getBetweenDayNumber(issueDate, cDate);
leapYear = accrueDays - dayCount;
Calendar calend = Calendar.getInstance();
calend.setTime(dCDate);
Calendar calmid = Calendar.getInstance();
calmid.setTime(dCDate);
calmid.add(5, -1 * ((Integer) listInt.get(1)).intValue());
if ((calmid.get(2) == 1) && (calmid.get(5) == 29)) {
leapYear++;
} else {
Calendar caladdy = Calendar.getInstance();
caladdy.set(calmid.get(1), calmid.get(2), calmid.get(5));
caladdy.add(1, 1);
Calendar caladdy2 = Calendar.getInstance();
caladdy2.set(calmid.get(1), calmid.get(2), calmid.get(5));
caladdy2.add(5, 365);
if (caladdy.getTimeInMillis() != caladdy2.getTimeInMillis()) {
int y1 = calmid.get(1);
int y2 = calend.get(1);
Calendar cal28_y1 = Calendar.getInstance();
cal28_y1.set(y1, 1, 28);
cal28_y1.add(5, 1);
if (cal28_y1.get(2) == 1) {
if ((!cal28_y1.after(cal28_y1)) && (calend.after(cal28_y1))) {
leapYear++;
}
}
if (y1 != y2) {
cal28_y1.set(y2, 1, 28);
cal28_y1.add(5, 1);
if (cal28_y1.get(2) == 1) {
if ((calmid.before(cal28_y1)) && (calend.after(cal28_y1))) {
leapYear++;
}
}
}
}
}
} catch (Exception localException) {
}
return leapYear;
}
/**
* 按照指定格式增加日期
*
* @param date 原始日期字符串
* @param payment_freq 增加数量
* @param payment_unit 日期单位:年Y/月M/日D
* @return String 处理后的日期字符串
* @throws ParseException
* @throws Exception
*/
public static String addDate(String date, int payment_freq, String payment_unit) throws ParseException, Exception {
SimpleDateFormat sdf = new SimpleDateFormat(DATE_PATTERN_01);
Date d = sdf.parse(date);
Calendar cal = Calendar.getInstance();
cal.setTime(d);
if (("D").equals(payment_unit))
cal.add(5, payment_freq);
else if (("M").equals(payment_unit))
cal.add(2, payment_freq);
else if (("Y").equals(payment_unit))
cal.add(1, payment_freq);
else {
throw new Exception();
}
return sdf.format(cal.getTime());
}
/**
* 说明:比较两个日期大小,Date格式<br>
* 备注:date1>date2 返回1 ,date1 = date2 返回0 , date1 <date2 返回 -1<br>
*
* @param date1
* 日期1
* @param date2
* 日期2
* @return 比较结果
*/
public static int CompareDate(Date date1, Date date2) {
if (date1.getTime() > date2.getTime()) {
return 1;
} else if (date1.getTime() == date2.getTime()) {
return 0;
} else {
return -1;
}
}
/**
* 字符串类型日期比较
*
* @param d1 第一个日期字符串
* @param d2 第二个日期字符串
* @return int 0表示相等/-1表示小于/1表示大于
* @throws ParseException
*/
public static int compareDate(String d1, String d2) throws ParseException {
if (d1 == null && d2 == null) {
return 0;
} else if (d1 == null) {
return -1;
} else if (d2 == null) {
return 1;
} else {
// SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
// Date date1 = sdf.parse(d1);
// Date date2 = sdf.parse(d2);
// if (date1.getTime() > date2.getTime())
// return 1;
// else if (date1.getTime() == date2.getTime()) {
// return 0;
// }
return d1.compareTo(d2);
}
// return -1;
}
/**
* 计算第二个日期和第一个日期的年份和天数差值组成的列表</br>
* 例:DateUtil.getBetweenYearNumber("2016-06-02", "2017-06-05") 返回 [1, 3]
*
* @param date1 日期1
* @param date2 日期2
* @return List<Integer>结果
*/
public static List<Integer> getBetweenYearNumberBackward(String date1, String date2) {
List result = new ArrayList();
result = getBetweenYearNumberBackward(parse(date1), parse(date2));
return result;
}
/**
* 计算第二个日期和第一个日期的年份和天数差值组成的列表</br>
* 例:DateUtil.getBetweenYearNumber("2016-06-02", "2017-06-05") 返回 [1, 3]
*
* @param date1 日期1
* @param date2 日期2
* @return List<Integer>结果
*/
public static List<Integer> getBetweenYearNumberBackward(Date date1, Date date2) {
List result = new ArrayList();
Calendar cal = Calendar.getInstance();
cal.setTime(date1);
int y1 = cal.get(1);
int m1 = cal.get(2);
int d1 = cal.get(5);
cal.setTime(date2);
int y2 = cal.get(1);
int m2 = cal.get(2);
int d2 = cal.get(5);
if ((m2 > m1) || ((m2 >= m1) && (d2 >= d1))) {
result.add(Integer.valueOf(y2 - y1));
cal.set(y1, m2, d2);
Date temp = cal.getTime();
result.add(Integer.valueOf(getBetweenDayNumber(date1, temp)));
} else {
result.add(Integer.valueOf(y2 - y1 - 1));
cal.set(y1 + 1, m2, d2);
Date temp = cal.getTime();
result.add(Integer.valueOf(getBetweenDayNumber(date1, temp)));
}
return result;
}
/**
* 计算一个日期加上payment_freq*payment_unit后的日期 payment_freq 收付息周期
*
* @param d
* @param payment_freq
* @param payment_unit
* @return Date
*/
public static Date addDate(Date d, int payment_freq, String payment_unit) {
// throws NeedDealException
Calendar cal = Calendar.getInstance();
cal.setTime(d);
if (("D").equals(payment_unit)) {
cal.add(Calendar.DAY_OF_MONTH, payment_freq);
} else if (("M").equals(payment_unit)) {
cal.add(Calendar.MONTH, payment_freq);
} else if (("Y").equals(payment_unit)) {
cal.add(Calendar.YEAR, payment_freq);
} else {
// throw new NeedDealException("不支持的日期单位");
}
return cal.getTime();
}
/**
* 将字符串类型的转换成Date类型
*
* @param dateStr
* 字符串类型的日期 yyyy-MM-dd
* @return Date类型的日期
* @throws Exception 所有异常
*/
public static Date convertStringToDate(String dateStr) throws Exception {
// 返回的日期
Date resultDate;
try {
// 日期格式转换
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
resultDate = sdf.parse(dateStr);
} catch (ParseException e) {
throw new Exception("数据转换异常");
}
return resultDate;
}
/**
* 获取给定日期的当月的最后一天
*
* @param todayDate 参照日期
* @return 当月的最后一天
*/
public static Date getLastDay(Date todayDate) {
Calendar calendar = Calendar.getInstance();
// 把平台日期赋值给Calendar
calendar.setTime(todayDate);
calendar.set(Calendar.YEAR, calendar.get(Calendar.YEAR));
calendar.set(Calendar.MONTH, calendar.get(Calendar.MONTH));
int endday = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
calendar.set(Calendar.DAY_OF_MONTH, endday);
return calendar.getTime();
}
/**
* 将Date转换成String
*
* @param todayDate
* 当前日期,Date类型
* @param format
* 转换格式的类型
* @return 转换成字符串类型
*/
public static String convertDateToString(Date todayDate, String format) {
// 日期格式转换
SimpleDateFormat sdf = new SimpleDateFormat(format);
// 返回的字符串
String tempStr = sdf.format(todayDate);
return tempStr;
}
/**
* Convert the passed integer value to date with default format yyyMMdd.
*
* @param iDate
* the integer value of date. Ex: 20071003.
* @return Date the converted date. Date format of 2007/10/03.
*/
public static Date convertIntegerToDate(Integer iDate) {
if (null == iDate) {
return null;
}
String sDate;
Date baseDate;
// logic to make sure that the year does not exceed the limit of 9999.
if (iDate > DATE_LIMIT) {
// iDate = DateFormatDef.EXPIRY_DATE_INT;
iDate = DATE_LIMIT;
}
sDate = String.valueOf(iDate);
baseDate = parse(DATE_PATTERN_02, sDate);
return baseDate;
}
/**
* @param date
* the util Date. Ex: Date of 2007/10/04.
* @return iDate the integer value of the date. Ex: 20071004.
*/
public static Integer convertDateToInteger(Date date) {
if (null == date) {
return null;
}
String sDate = convertDateToString(date);
return Integer.parseInt(sDate);
}
/**
* Returns the Maximum data by comparing two dates.
*
* @param date1
* The first date to be comapred
* @param date2
* The second date to be compared
* @return Date The maximum date of two dates
*/
public static Date getMaxDate(Date date1, Date date2) {
int value = date1.compareTo(date2);
if (value < 0) {
return date2;
} else if (value > 0) {
return date1;
}
return date1;
}
/**
* Method to convert the date to MMDD format.
*
* @param dateBeforeFmt 原始日期
* @return String in MMDD format
*/
public static String toMMDDStrFormat(Date dateBeforeFmt) {
if (dateBeforeFmt == null) {
return null;
}
// Create an instance of Calendar class.
Calendar calendar = Calendar.getInstance();
calendar.setTime(dateBeforeFmt);
// Holds the current system time in MMDD format.
StringBuffer currentTime = new StringBuffer();
// get the month and date.
String strMonth = String.valueOf(calendar.get(Calendar.MONTH) + 1);
String strDay = String.valueOf(calendar.get(Calendar.DAY_OF_MONTH));
// If month length is equal to 1, preffix 0 to it.
if (strMonth.length() == 1) {
currentTime.append(0);
}
// Prefix month.
currentTime.append(strMonth);
// If day length is equal to 1, preffix 0 to it.
if (strDay.length() == 1) {
currentTime.append(0);
}
// Prefix day.
currentTime.append(strDay);
// return the current time in MMDD format.
return String.valueOf(currentTime);
}
/**
* 根据起始时间、结束时间生成半小时的时间数组
*/
public static List<String> getTimes(String begin,String end){
String[] allTimes = {"00:00","00:30","01:00","01:30","02:00","02:30","03:00","03:30","04:00","04:30","05:00","05:30",
"06:00","06:30","07:00","07:30","08:00","08:30","09:00","09:30","10:00","10:30","11:00","11:30",
"12:00","12:30","13:00","13:30","14:00","14:30","15:00","15:30","16:00","16:30","17:00","17:30",
"18:00","18:30","19:00","19:30","20:00","20:30","21:00","21:30","22:00","22:30","23:00","23:30"};
List<String> times = new ArrayList<>();
for(int i=0;i<allTimes.length;i++){
if(allTimes[i].compareTo(begin)>=0 && allTimes[i].compareTo(end)<=0){
times.add(allTimes[i]);
}
}
return times;
}
/**
*
* 获取格式化后的字符串日期
* @param pDate
* @param datePattern
* @return
*/
public static String getStrDate(Date pDate, String datePattern) {
if (StringUtil.isEmpty(datePattern)) {
return "";
}
if (pDate == null) {
return "";
}
SimpleDateFormat dateFormat = new SimpleDateFormat(datePattern);
return dateFormat.format(pDate);
}
}
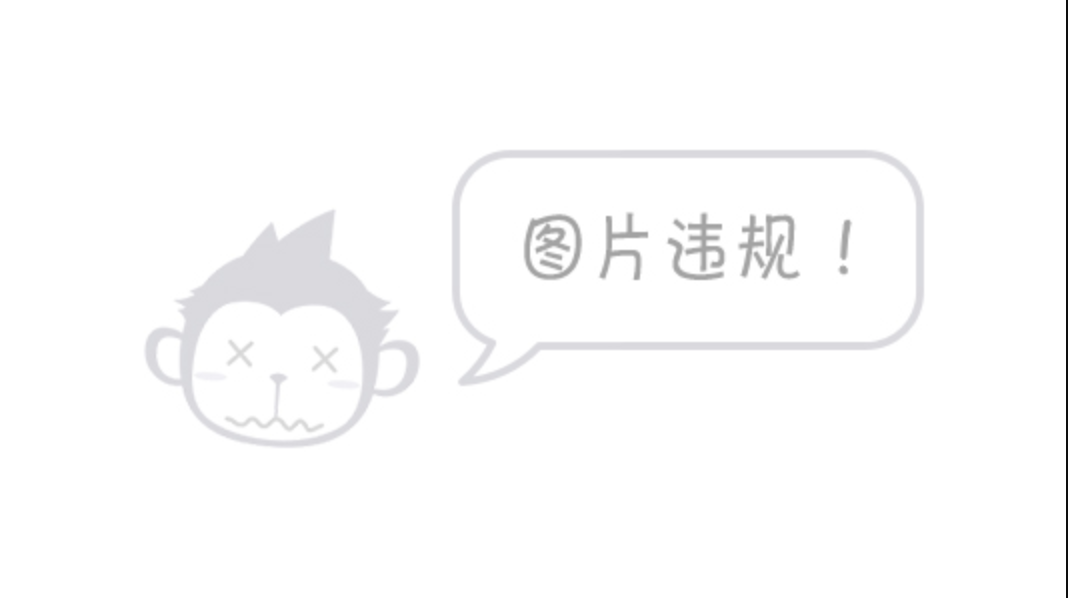
代码下载地址: