一
C++ primerplus
第六章分支语句是看到一个案例,
char ch;
cout << "Typr , and I shell repeat.\n";
cin.get(ch);
while (ch != '.') {
if (ch == '\n')
cout << ch;
else
cout << ch +1;
cin.get(ch);
}
cout << "\n Please excuse thr slight confusion.\n";
其中我们将cout<<ch+1
替换为cout<<++ch
之后会出现两个不同的
-
ch+1
-
++ch
可以看到一个为字符前进以为一个将char
转换为整型,看书加上自己目前的理解觉得是cout
输出参数会自动根据内容进行输出调整,所以将前者递增识别为char
的自增,将后者识别为整型相加,最后的结果就会将'c'
进行输出.
’A’与"A"的区别为字符串A相当于’A\0’在字符‘A’后加了一个空字符
二
EOF语句,重置文件尾
函数与数组
void(int[] arr,int n)
其中arr表示数组指针,通过访问arr数组第一个值的指针从而访问整个数组
书中实例对这一情况进行讲解
int cookies[8] = {1,2,4,8,16,32,64,128};
void sum_arr(arr,8)
访问容量8的数组,数组Σ为255;
void sum_arr(arr,3);
访问同一个数组,数组容量为3,结果为7
void sum_arr(arr+4,4);
,数组容量为4,计算后面四个数字的Σ.结果为240
上面三个可以发现arr在函数中作为数组第一个指针进行传递
函数指针
double besty(int);
double pam(int);
void estimate(int,double(*pf)(int));
int main()
{
using namespace std;
int code;
cout << "how many code you have : ";
cin >> code;
cout << "here's betsy's estimate:\n";
estimate(code, besty);
cout << "here's pam's estimate:\n";
estimate(code, pam);
return 0;
}
double besty(int ins) {
return 0.05 * ins;
}
double pam(int ins) {
return 0.03 * ins + 0.0004 * ins * ins;
}
void estimate(int lines, double (*pf)(int)) {
using namespace std;
cout << lines << "lines will take ";
cout << (*pf)(lines) << "hours(s) \n";
}
通过只想函数指针地址调用函数
引用变量用作函数参数
第八章 函数探幽 引用变量 感觉还是不太明白,暂时将自己的理解记录后面纠正
书中实例,通过使用引用变量,指针和实参进行参数互换,其中使用引用进行数值互换
void swapr(int& a, int& b) {
int tmp;
tmp = a;
a = b;
b = tmp;
}
void swapv(int a, int b) {
int tmp;
tmp = a;
a = b;
b = tmp;
}
void swap(int* a, int* b) {
int tmp;
tmp = *a;
*a = *b;
*b = tmp;
}
void swapv(int a, int b);
void swap(int* a, int* b);
void swapr(int& a, int& b);
int main()
{
int wallent1 = 300;
int wallent2 = 350;
swapv(wallent1, wallent2);
cout << "wallent1 = $" << wallent1 << " wallent2 = $ " << wallent2;
swap(&wallent1, &wallent2);
cout << "wallent1 = $" << wallent1 << " wallent2 = $ " << wallent2;
swapr(wallent1, wallent2)
cout << "wallent1 = $" << wallent1 << " wallent2 = $ " << wallent2;
return 0;
}
- 当我们使用引用变量进行数值互换是,此时
wallent1
,wallent2
作为a,b
的一个变量名称传入,简化为int& a = wallent1
,此时a,b
作为形参进行数值互换
可以看到在数值互换结束后,wallent1的值与a的值的地址一致 - 如果将值直接传入函数中,a新建临时
int
变量wallent1的值,在函数关系中只是对a,b
的数值进行互换与传入值无关
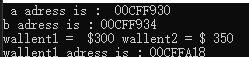
可以看到a,b
与wallent1,wallentr2
指向的地址不同.
字符串、向量和数组
string s = "hiYa";//拷贝初始化,将'hiYa'的值拷贝到s中
string s2("hiYa");//直接初始化
string s3(10,'c');//直接初始化,与vector(10,'c')表达意思一致创建指定数量的‘c'
vector<unsigned > scores {11,0};//定义一个容量为11,数值为0的vector 直接初始化
unsigned grade;
while(cin>>grade){
if(grade<=100)
++scores[grade/10];//等价于 auto ind = grade/10; scores[ind] = scores[ind]+1; 相当于取出指定位置数值自加
}
C++ Primer Part5.4
课后习题:5.4.1 读取若干string并查找连续重复出现的单词,输出重复出现的最大次数,如果不存在,输出一条信息说明任何单词都没有连续出现过
之前刷Leetcode见过类似的题目,忘记了当时怎么做的只能重新写一遍 ,感觉还是需要优化一下,整个代码体现的思路有一些混乱,后面再优化一下吧。
int main() {
using namespace std;
string s;//中间值
int count=0;//最终值
int tmp = 1;//中间值,起始量为1
string sStr;//字符串最终值
vector<string> strings {"how","now","cow","cow"};//定义对象集合
s = strings[0];//以第一个为第一次循环的判定对象
for (int i = 1; i < strings.size(); ++i) {
if(strings[i]==s){
++tmp;//如果相等,数量自加
} else
{
if (tmp > count&&tmp>=2)//如果与记录的字符串中间值不一致,且tmp>2,交换对象,count赋值
{
count = tmp;
sStr=s;
s = strings[i];
tmp = 1;
} else{//如果数量没有超过现有的总和,单纯交换中间值
tmp=1;
s=strings[i];
}
}
//使用for循环或while循环这种判定方式会遗漏最后一个数组值
if(strings.size()-1==i&&strings[i]==s) {if(tmp>count&&tmp>=2) {count = tmp;sStr=strings[i];} }
}
//如果对象集合内无重复对象
if(count==0&&s==strings[strings.size()-1]){cout<<"对象集合中无重复对象"<<endl;}
else{
cout<<count<<sStr<<endl;
}
return 0;
}
C++ Primer Part 6.1 函数基础
局部静态对象(local static object):在程序执行路径第一次经过对象定义语句时初始化,并且直到程序终止才被销毁,在此期间即使对象所在的函数结束执行也不会对它有影响。
size_t count_calls(){
static size_t ctr = 0;
return ++ctr;
}
int main() {
using namespace std;
for (size_t i = 0; i != 10; ++i) {
cout<<count_calls()<<endl;
}
return 0;
}